KTextTemplate::Parser
#include <KTextTemplate/Parser>
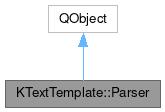
Public Member Functions | |
Parser (const QList< Token > &tokenList, QObject *parent) | |
~Parser () override | |
QSharedPointer< Filter > | getFilter (const QString &name) const |
bool | hasNextToken () const |
void | invalidBlockTag (const Token &token, const QString &command, const QStringList &stopAt={}) |
NodeList | parse (Node *parent, const QString &stopAt) |
NodeList | parse (Node *parent, const QStringList &stopAt={}) |
NodeList | parse (TemplateImpl *parent, const QStringList &stopAt={}) |
void | removeNextToken () |
void | skipPast (const QString &tag) |
Token | takeNextToken () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
void | prependToken (const Token &token) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The Parser class processes a string template into a tree of nodes.
For application developers, this class is internal.
For template tag authors it may be necessary to advance the parser and process contained tags if the tag works in a tag – endtag fashion.
Constructor & Destructor Documentation
◆ Parser()
◆ ~Parser()
|
override |
Destructor.
Definition at line 96 of file parser.cpp.
Member Function Documentation
◆ getFilter()
QSharedPointer< Filter > Parser::getFilter | ( | const QString & | name | ) | const |
Returns the filter object called name
or an invalid object if no filter with that name is loaded.
Definition at line 138 of file parser.cpp.
◆ hasNextToken()
bool Parser::hasNextToken | ( | ) | const |
Returns whether the parser has another token to process.
Definition at line 253 of file parser.cpp.
◆ invalidBlockTag()
void Parser::invalidBlockTag | ( | const Token & | token, |
const QString & | command, | ||
const QStringList & | stopAt = {} ) |
Definition at line 271 of file parser.cpp.
◆ parse() [1/3]
◆ parse() [2/3]
NodeList Parser::parse | ( | Node * | parent, |
const QStringList & | stopAt = {} ) |
Advance the parser, using parent
as the parent of new Nodes.
The parser will stop if it encounters a tag which is contained in the list stopAt
.
For example, the {% if %}
tag would stopAt both {% endif %}
and {% else %}
tags.
- See also
- AbstractNodeFactory::getNode
Definition at line 160 of file parser.cpp.
◆ parse() [3/3]
NodeList KTextTemplate::Parser::parse | ( | TemplateImpl * | parent, |
const QStringList & | stopAt = {} ) |
This is an overloaded method.
- See also
- parse.
◆ prependToken()
|
protected |
Puts the token token
to the front of the list to be processed by the parser.
Definition at line 285 of file parser.cpp.
◆ removeNextToken()
void Parser::removeNextToken | ( | ) |
Deletes the next token available to the parser.
Definition at line 265 of file parser.cpp.
◆ skipPast()
void Parser::skipPast | ( | const QString & | tag | ) |
Advances the parser to the tag tag
.
This method is similar to parse, but it does not create nodes for tags encountered.
Definition at line 128 of file parser.cpp.
◆ takeNextToken()
Token Parser::takeNextToken | ( | ) |
Returns the next token to be processed by the parser.
This can be examined in template tag implementations to determine why parsing stopped.
For example, if the {% if %}
tag, parsing may stop at an {% else %}
tag, in which case parsing should be restarted, or it could stop at an {% endif %}
tag, in which case parsing is finished for that node.
Definition at line 259 of file parser.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:49:34 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.