KMime::Headers::ContentType
#include <kmime_headers.h>
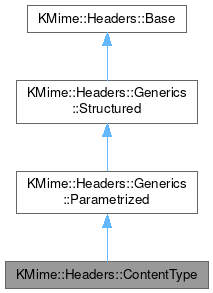
Public Member Functions | |
QByteArray | as7BitString (bool withHeaderType=true) const override |
QByteArray | boundary () const |
QByteArray | charset () const |
void | clear () override |
QByteArray | id () const |
bool | isEmpty () const override |
bool | isHTMLText () const |
bool | isImage () const |
bool | isMediatype (const char *mediatype) const |
bool | isMimeType (const char *mimeType) const |
bool | isMultipart () const |
bool | isPartial () const |
bool | isPlainText () const |
bool | isSubtype (const char *subtype) const |
bool | isText () const |
QByteArray | mediaType () const |
QByteArray | mimeType () const |
QString | name () const |
int | partialCount () const |
int | partialNumber () const |
void | setBoundary (const QByteArray &s) |
void | setCharset (const QByteArray &s) |
void | setId (const QByteArray &s) |
void | setMimeType (const QByteArray &mimeType) |
void | setName (const QString &s, const QByteArray &cs) |
void | setPartialParams (int total, int number) |
QByteArray | subType () const |
![]() | |
QByteArray | as7BitString (bool withHeaderType=true) const override |
void | clear () override |
bool | hasParameter (const QString &key) const |
bool | isEmpty () const override |
QString | parameter (const QString &key) const |
void | setParameter (const QString &key, const QString &value) |
![]() | |
QString | asUnicodeString () const override |
void | from7BitString (const char *s, size_t len) override |
void | from7BitString (const QByteArray &s) override |
void | fromUnicodeString (const QString &s, const QByteArray &b) override |
![]() | |
Base () | |
virtual | ~Base () |
bool | is (const char *t) const |
bool | isMimeHeader () const |
QByteArray | rfc2047Charset () const |
void | setRFC2047Charset (const QByteArray &cs) |
virtual const char * | type () const |
Protected Member Functions | |
bool | parse (const char *&scursor, const char *const send, bool isCRLF=false) override |
![]() | |
bool | parse (const char *&scursor, const char *const send, bool isCRLF=false) override |
![]() | |
QByteArray | typeIntro () const |
Additional Inherited Members | |
![]() | |
typedef QList< KMime::Headers::Base * > | List |
Detailed Description
Represents a "Content-Type" header.
- See also
- RFC 2045, section 5.
Definition at line 976 of file kmime_headers.h.
Member Function Documentation
◆ as7BitString()
|
overridevirtual |
Returns the encoded header.
- Parameters
-
withHeaderType Specifies whether the header-type should be included.
Implements KMime::Headers::Base.
Definition at line 1633 of file kmime_headers.cpp.
◆ boundary()
QByteArray KMime::Headers::ContentType::boundary | ( | ) | const |
Returns the boundary (for multipart containers).
Definition at line 1742 of file kmime_headers.cpp.
◆ charset()
QByteArray KMime::Headers::ContentType::charset | ( | ) | const |
Returns the charset for the associated MIME entity.
Definition at line 1729 of file kmime_headers.cpp.
◆ clear()
|
overridevirtual |
◆ id()
QByteArray KMime::Headers::ContentType::id | ( | ) | const |
Returns the identifier of the associated MIME entity.
Definition at line 1760 of file kmime_headers.cpp.
◆ isEmpty()
|
overridevirtual |
Checks if this header contains any data.
Implements KMime::Headers::Base.
Definition at line 1623 of file kmime_headers.cpp.
◆ isHTMLText()
bool KMime::Headers::ContentType::isHTMLText | ( | ) | const |
Returns true if the associated MIME entity is a HTML file.
Definition at line 1713 of file kmime_headers.cpp.
◆ isImage()
bool KMime::Headers::ContentType::isImage | ( | ) | const |
Returns true if the associated MIME entity is an image.
Definition at line 1717 of file kmime_headers.cpp.
◆ isMediatype()
bool KMime::Headers::ContentType::isMediatype | ( | const char * | mediatype | ) | const |
Tests if the media type equals mediatype
.
Definition at line 1681 of file kmime_headers.cpp.
◆ isMimeType()
bool KMime::Headers::ContentType::isMimeType | ( | const char * | mimeType | ) | const |
Tests if the mime type is mimeType
.
Definition at line 1699 of file kmime_headers.cpp.
◆ isMultipart()
bool KMime::Headers::ContentType::isMultipart | ( | ) | const |
Returns true if the associated MIME entity is a multipart container.
Definition at line 1721 of file kmime_headers.cpp.
◆ isPartial()
bool KMime::Headers::ContentType::isPartial | ( | ) | const |
Returns true if the associated MIME entity contains partial data.
- See also
- partialNumber(), partialCount()
Definition at line 1725 of file kmime_headers.cpp.
◆ isPlainText()
bool KMime::Headers::ContentType::isPlainText | ( | ) | const |
Returns true if the associated MIME entity is a plain text.
Definition at line 1709 of file kmime_headers.cpp.
◆ isSubtype()
bool KMime::Headers::ContentType::isSubtype | ( | const char * | subtype | ) | const |
Tests if the mime sub-type equals subtype
.
Definition at line 1688 of file kmime_headers.cpp.
◆ isText()
bool KMime::Headers::ContentType::isText | ( | ) | const |
Returns true if the associated MIME entity is a text.
Definition at line 1705 of file kmime_headers.cpp.
◆ mediaType()
QByteArray KMime::Headers::ContentType::mediaType | ( | ) | const |
Returns the media type (first part of the mimetype).
Definition at line 1656 of file kmime_headers.cpp.
◆ mimeType()
QByteArray KMime::Headers::ContentType::mimeType | ( | ) | const |
Returns the mimetype.
Definition at line 1651 of file kmime_headers.cpp.
◆ name()
QString KMime::Headers::ContentType::name | ( | ) | const |
Returns the name of the associated MIME entity.
Definition at line 1750 of file kmime_headers.cpp.
◆ parse()
|
overrideprotectedvirtual |
This method parses the raw header and needs to be implemented in every sub-class.
- Parameters
-
scursor Pointer to the start of the data still to parse. send Pointer to the end of the data. isCRLF true if input string is terminated with a CRLF.
Implements KMime::Headers::Generics::Structured.
Definition at line 1791 of file kmime_headers.cpp.
◆ partialCount()
int KMime::Headers::ContentType::partialCount | ( | ) | const |
Returns the total number of parts in a multi-part set.
- See also
- isPartial(), partialNumber()
Definition at line 1777 of file kmime_headers.cpp.
◆ partialNumber()
int KMime::Headers::ContentType::partialNumber | ( | ) | const |
Returns the position of this part in a multi-part set.
- See also
- isPartial(), partialCount()
Definition at line 1768 of file kmime_headers.cpp.
◆ setBoundary()
void KMime::Headers::ContentType::setBoundary | ( | const QByteArray & | s | ) |
Sets the multipart container boundary.
Definition at line 1746 of file kmime_headers.cpp.
◆ setCharset()
void KMime::Headers::ContentType::setCharset | ( | const QByteArray & | s | ) |
Sets the charset.
Definition at line 1738 of file kmime_headers.cpp.
◆ setId()
void KMime::Headers::ContentType::setId | ( | const QByteArray & | s | ) |
Sets the identifier.
Definition at line 1764 of file kmime_headers.cpp.
◆ setMimeType()
void KMime::Headers::ContentType::setMimeType | ( | const QByteArray & | mimeType | ) |
Sets the mimetype.
- Parameters
-
mimeType The new mimetype.
Definition at line 1676 of file kmime_headers.cpp.
◆ setName()
void KMime::Headers::ContentType::setName | ( | const QString & | s, |
const QByteArray & | cs ) |
Sets the name to s
using charset cs
.
Definition at line 1754 of file kmime_headers.cpp.
◆ setPartialParams()
void KMime::Headers::ContentType::setPartialParams | ( | int | total, |
int | number ) |
Sets parameters of a partial MIME entity.
- Parameters
-
total The total number of entities in the multi-part set. number The number of this entity in a multi-part set.
Definition at line 1786 of file kmime_headers.cpp.
◆ subType()
QByteArray KMime::Headers::ContentType::subType | ( | ) | const |
Returns the mime sub-type (second part of the mimetype).
Definition at line 1666 of file kmime_headers.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:20:12 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.