KNewPasswordDialog Class Reference
from PyKDE4.kdeui import *
Inherits: KDialog → QDialog → QWidget → QObject
Detailed Description
A password input dialog.
This dialog asks the user to enter a password.
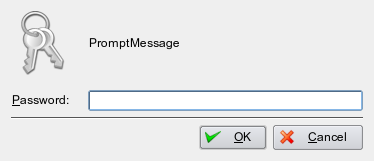
"KDE Password Dialog"
Usage Exemple
KNewPasswordDialog *dlg = new KNewPasswordDialog( parent ); dlg->setPrompt( i18n( "Enter a password" ); connect( dlg, SIGNAL( newPassword( const QString& ) ) , this, SLOT( setPassword( const QString &) ) ); connect( dlg, SIGNAL( rejected() ) , this, SLOT( slotCancel() ) ); dlg->show();
KNewPasswordDialog dlg( parent ); dlg.setPrompt( i18n( "Enter a password" ); if( dlg.exec() ) setPassword( dlg.password() );
Signals | |
newPassword (QString password) | |
Methods | |
__init__ (self, QWidget parent=0) | |
accept (self) | |
bool | allowEmptyPasswords (self) |
bool | checkAndGetPassword (self, QString pwd) |
bool | checkPassword (self, QString a0) |
int | maximumPasswordLength (self) |
int | minimumPasswordLength (self) |
newPassword (self, QString password) | |
QString | password (self) |
int | passwordStrengthWarningLevel (self) |
QPixmap | pixmap (self) |
QString | prompt (self) |
int | reasonablePasswordLength (self) |
setAllowEmptyPasswords (self, bool allowed) | |
setMaximumPasswordLength (self, int maxLength) | |
setMinimumPasswordLength (self, int minLength) | |
setPasswordStrengthWarningLevel (self, int warningLevel) | |
setPixmap (self, QPixmap a0) | |
setPrompt (self, QString prompt) | |
setReasonablePasswordLength (self, int reasonableLength) |
Method Documentation
__init__ | ( | self, | ||
QWidget | parent=0 | |||
) |
Constructs a password dialog.
- Parameters:
-
parent Passed to lower level constructor.
accept | ( | self ) |
- Internal:
bool allowEmptyPasswords | ( | self ) |
Allow empty passwords?
- Returns:
- true if minimumPasswordLength() == 0
bool checkAndGetPassword | ( | self, | ||
QString | pwd | |||
) |
Checks input password. If the password is right, returns true and fills pwd with the password. Otherwise returns false and pwd will be null.
- Since:
- 4.2
bool checkPassword | ( | self, | ||
QString | a0 | |||
) |
Virtual function that can be overridden to provide password checking in derived classes. It should return true if the password is valid, false otherwise.
int maximumPasswordLength | ( | self ) |
Maximum acceptable password length.
int minimumPasswordLength | ( | self ) |
Minimum acceptable password length.
newPassword | ( | self, | ||
QString | password | |||
) |
The dialog has been accepted, and the new password is password
- Signal syntax:
QObject.connect(source, SIGNAL("newPassword(const QString&)"), target_slot)
QString password | ( | self ) |
Returns the password entered.
- Note:
- Only has meaningful data after accept has been called if you want to access the password from a subclass use checkAndGetPassword()
int passwordStrengthWarningLevel | ( | self ) |
Password strength level below which a warning is given
QPixmap pixmap | ( | self ) |
Returns the pixmap that appears next to the prompt in the dialog
QString prompt | ( | self ) |
Returns the password prompt.
int reasonablePasswordLength | ( | self ) |
Password length that is expected to be reasonably safe.
setAllowEmptyPasswords | ( | self, | ||
bool | allowed | |||
) |
Allow empty passwords? - Default: true
same as setMinimumPasswordLength( allowed ? 0 : 1 )
setMaximumPasswordLength | ( | self, | ||
int | maxLength | |||
) |
Maximum acceptable password length.
- Parameters:
-
maxLength: The new maximum password length.
setMinimumPasswordLength | ( | self, | ||
int | minLength | |||
) |
Minimum acceptable password length.
Default: 0
- Parameters:
-
minLength: The new minimum password length
setPasswordStrengthWarningLevel | ( | self, | ||
int | warningLevel | |||
) |
Set the password strength level below which a warning is given Value is in the range 0 to 99. Empty passwords score 0; non-empty passwords score up to 100, depending on their length and whether they contain numbers, mixed case letters and punctuation.
Default: 1 - warn if the password has no discernable strength whatsoever
- Parameters:
-
warningLevel: The level below which a warning should be given.
setPixmap | ( | self, | ||
QPixmap | a0 | |||
) |
Sets the pixmap that appears next to the prompt in the dialog. The default pixmap represent a simple key.
the recommended size is KIconLoader.SizeHuge
setPrompt | ( | self, | ||
QString | prompt | |||
) |
Sets the password prompt.
setReasonablePasswordLength | ( | self, | ||
int | reasonableLength | |||
) |
Password length that is expected to be reasonably safe.
Used to compute the strength level
Default: 8 - the standard UNIX password length
- Parameters:
-
reasonableLength: The new reasonable password length.