KCharSelect Class Reference
from PyKDE4.kdeui import *
Detailed Description
Character selection widget
This widget allows the user to select a character of a specified font and to browse Unicode information
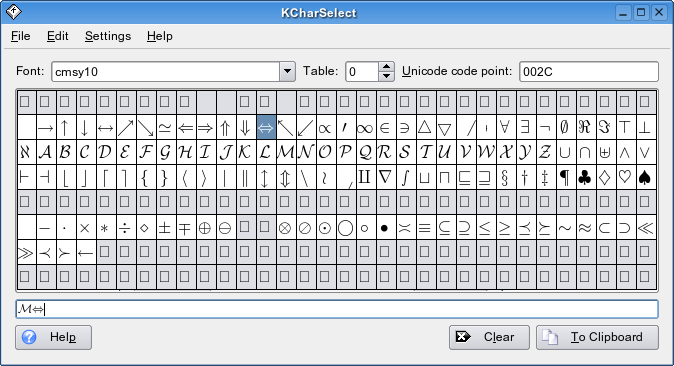
"Character Selection Widget"
You can specify the font whose characters should be displayed via setCurrentFont(). Using the Controls argument in the contructor you can create a compact version of KCharSelect if there is not enough space and if you don't need all features.
KCharSelect displays one Unicode block at a time and provides categorized access to them. Unicode character names and further details, including cross references, are displayed. Additionally, there is a search to find characters.
To get the current selected character, use the currentChar() method. You can set the character which should be displayed with setCurrentChar().
Enumerations | |
Control | { SearchLine, FontCombo, FontSize, BlockCombos, CharacterTable, DetailBrowser, HistoryButtons, AllGuiElements } Typesafe wrapper: Controls |
Signals | |
charSelected (QChar c) | |
currentCharChanged (QChar c) | |
currentFontChanged (QFont font) | |
displayedCharsChanged () | |
Methods | |
__init__ (self, QWidget parent, KCharSelect.Controls controls=KCharSelect.AllGuiElements) | |
__init__ (self, QWidget parent, KActionCollection collection, KCharSelect.Controls controls=KCharSelect.AllGuiElements) | |
charSelected (self, QChar c) | |
QChar | currentChar (self) |
currentCharChanged (self, QChar c) | |
QFont | currentFont (self) |
currentFontChanged (self, QFont font) | |
[QChar] | displayedChars (self) |
displayedCharsChanged (self) | |
setCurrentChar (self, QChar c) | |
setCurrentFont (self, QFont font) | |
QSize | sizeHint (self) |
Method Documentation
__init__ | ( | self, | ||
QWidget | parent, | |||
KCharSelect.Controls | controls=KCharSelect.AllGuiElements | |||
) |
- Deprecated:
__init__ | ( | self, | ||
QWidget | parent, | |||
KActionCollection | collection, | |||
KCharSelect.Controls | controls=KCharSelect.AllGuiElements | |||
) |
Constructor. controls can be used to show a custom set of widgets.
The widget uses the following actions: - KStandardActions.find() (edit_find) - KStandardActions.back() (go_back) - KStandardActions.forward() (go_forward)
If you provide a KActionCollection, this will be populated with the above actions, which you can then manually trigger or place in menus and toolbars.
- Parameters:
-
parent the parent widget for this KCharSelect (see QWidget documentation) collection if this is not null, KCharSelect will place its actions into this collection controls selects the visible controls on the KCharSelect widget
- Since:
- 4.2
charSelected | ( | self, | ||
QChar | c | |||
) |
A character is selected to be inserted somewhere.
- Parameters:
-
c the selected character
- Signal syntax:
QObject.connect(source, SIGNAL("charSelected(const QChar&)"), target_slot)
QChar currentChar | ( | self ) |
Returns the currently selected character.
currentCharChanged | ( | self, | ||
QChar | c | |||
) |
The current character is changed.
- Parameters:
-
c the new character
- Signal syntax:
QObject.connect(source, SIGNAL("currentCharChanged(const QChar&)"), target_slot)
QFont currentFont | ( | self ) |
Returns the currently displayed font.
currentFontChanged | ( | self, | ||
QFont | font | |||
) |
A new font is selected or the font size changed.
- Parameters:
-
font the new font
- Signal syntax:
QObject.connect(source, SIGNAL("currentFontChanged(const QFont&)"), target_slot)
[QChar] displayedChars | ( | self ) |
Returns a list of currently displayed characters.
displayedCharsChanged | ( | self ) |
The currently displayed characters are changed (search results or block).
- Signal syntax:
QObject.connect(source, SIGNAL("displayedCharsChanged()"), target_slot)
setCurrentChar | ( | self, | ||
QChar | c | |||
) |
Highlights the character c. If the character is not displayed, the block is changed.
- Parameters:
-
c the character to highlight
setCurrentFont | ( | self, | ||
QFont | font | |||
) |
Sets the font which is displayed to font
- Parameters:
-
font the display font for the widget
QSize sizeHint | ( | self ) |
Reimplemented.
Enumeration Documentation
Control |
Shows the search widgets
- Note:
- It is necessary to wrap members of this enumeration in a
Controls
instance when passing them to a method as group of flags. For example:Controls( SearchLine | FontCombo)
- Enumerator:
-
SearchLine = 0x01 FontCombo = 0x02 FontSize = 0x04 BlockCombos = 0x08 CharacterTable = 0x10 DetailBrowser = 0x20 HistoryButtons = 0x40 AllGuiElements = 65535