Plasma::WindowThumbnail
#include <windowthumbnail.h>
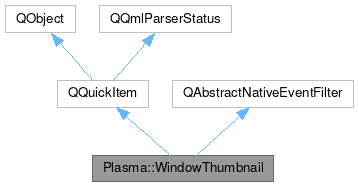
Properties | |
qreal | paintedHeight |
qreal | paintedWidth |
bool | thumbnailAvailable |
QML_ELEMENTuint | winId |
![]() | |
activeFocus | |
activeFocusOnTab | |
antialiasing | |
baselineOffset | |
childrenRect | |
clip | |
containmentMask | |
enabled | |
focus | |
height | |
implicitHeight | |
implicitWidth | |
opacity | |
parent | |
rotation | |
scale | |
smooth | |
state | |
transformOrigin | |
visible | |
width | |
x | |
y | |
z | |
![]() | |
objectName | |
Signals | |
void | paintedSizeChanged () |
void | thumbnailAvailableChanged () |
void | winIdChanged () |
Public Member Functions | |
WindowThumbnail (QQuickItem *parent=nullptr) | |
bool | isTextureProvider () const override |
bool | nativeEventFilter (const QByteArray &eventType, void *message, qintptr *result) override |
qreal | paintedHeight () const |
qreal | paintedWidth () const |
void | resetWinId () |
void | setWinId (uint32_t winId) |
QSGTextureProvider * | textureProvider () const override |
bool | thumbnailAvailable () const |
QSGNode * | updatePaintNode (QSGNode *oldNode, UpdatePaintNodeData *updatePaintNodeData) override |
uint32_t | winId () const |
![]() | |
QQuickItem (QQuickItem *parent) | |
Qt::MouseButtons | acceptedMouseButtons () const const |
bool | acceptHoverEvents () const const |
bool | acceptTouchEvents () const const |
void | activeFocusChanged (bool) |
bool | activeFocusOnTab () const const |
void | activeFocusOnTabChanged (bool) |
bool | antialiasing () const const |
void | antialiasingChanged (bool) |
qreal | baselineOffset () const const |
void | baselineOffsetChanged (qreal) |
QBindable< qreal > | bindableHeight () |
QBindable< qreal > | bindableWidth () |
QBindable< qreal > | bindableX () |
QBindable< qreal > | bindableY () |
virtual QRectF | boundingRect () const const |
QQuickItem * | childAt (qreal x, qreal y) const const |
QList< QQuickItem * > | childItems () const const |
QRectF | childrenRect () |
void | childrenRectChanged (const QRectF &) |
bool | clip () const const |
void | clipChanged (bool) |
virtual QRectF | clipRect () const const |
QObject * | containmentMask () const const |
void | containmentMaskChanged () |
virtual bool | contains (const QPointF &point) const const |
QCursor | cursor () const const |
void | dumpItemTree () const const |
void | enabledChanged () |
void | ensurePolished () |
bool | filtersChildMouseEvents () const const |
Flags | flags () const const |
void | focusChanged (bool) |
void | forceActiveFocus () |
void | forceActiveFocus (Qt::FocusReason reason) |
void | grabMouse () |
QSharedPointer< QQuickItemGrabResult > | grabToImage (const QSize &targetSize) |
void | grabTouchPoints (const QList< int > &ids) |
bool | hasActiveFocus () const const |
bool | hasFocus () const const |
qreal | height () const const |
void | heightChanged () |
qreal | implicitHeight () const const |
void | implicitHeightChanged () |
qreal | implicitWidth () const const |
void | implicitWidthChanged () |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
bool | isAncestorOf (const QQuickItem *child) const const |
bool | isEnabled () const const |
bool | isFocusScope () const const |
bool | isVisible () const const |
bool | keepMouseGrab () const const |
bool | keepTouchGrab () const const |
QPointF | mapFromGlobal (const QPointF &point) const const |
QPointF | mapFromItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapFromScene (const QPointF &point) const const |
QRectF | mapRectFromItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectFromScene (const QRectF &rect) const const |
QRectF | mapRectToItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectToScene (const QRectF &rect) const const |
QPointF | mapToGlobal (const QPointF &point) const const |
QPointF | mapToItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapToScene (const QPointF &point) const const |
QQuickItem * | nextItemInFocusChain (bool forward) |
qreal | opacity () const const |
void | opacityChanged () |
void | parentChanged (QQuickItem *) |
QQuickItem * | parentItem () const const |
void | polish () |
void | resetAntialiasing () |
void | resetHeight () |
void | resetWidth () |
qreal | rotation () const const |
void | rotationChanged () |
qreal | scale () const const |
void | scaleChanged () |
QQuickItem * | scopedFocusItem () const const |
void | setAcceptedMouseButtons (Qt::MouseButtons buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActiveFocusOnTab (bool) |
void | setAntialiasing (bool) |
void | setBaselineOffset (qreal) |
void | setClip (bool) |
void | setContainmentMask (QObject *mask) |
void | setCursor (const QCursor &cursor) |
void | setEnabled (bool) |
void | setFiltersChildMouseEvents (bool filter) |
void | setFlag (Flag flag, bool enabled) |
void | setFlags (Flags flags) |
void | setFocus (bool focus, Qt::FocusReason reason) |
void | setFocus (bool) |
void | setHeight (qreal) |
void | setImplicitHeight (qreal) |
void | setImplicitWidth (qreal) |
void | setKeepMouseGrab (bool keep) |
void | setKeepTouchGrab (bool keep) |
void | setOpacity (qreal) |
void | setParentItem (QQuickItem *parent) |
void | setRotation (qreal) |
void | setScale (qreal) |
void | setSize (const QSizeF &size) |
void | setSmooth (bool) |
void | setState (const QString &) |
void | setTransformOrigin (TransformOrigin) |
void | setVisible (bool) |
void | setWidth (qreal) |
void | setX (qreal) |
void | setY (qreal) |
void | setZ (qreal) |
QSizeF | size () const const |
bool | smooth () const const |
void | smoothChanged (bool) |
void | stackAfter (const QQuickItem *sibling) |
void | stackBefore (const QQuickItem *sibling) |
QString | state () const const |
void | stateChanged (const QString &) |
TransformOrigin | transformOrigin () const const |
void | transformOriginChanged (TransformOrigin) |
void | ungrabMouse () |
void | ungrabTouchPoints () |
void | unsetCursor () |
void | update () |
QQuickItem * | viewportItem () const const |
void | visibleChanged () |
qreal | width () const const |
void | widthChanged () |
QQuickWindow * | window () const const |
void | windowChanged (QQuickWindow *window) |
qreal | x () const const |
void | xChanged () |
qreal | y () const const |
void | yChanged () |
qreal | z () const const |
void | zChanged () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
void | itemChange (ItemChange change, const ItemChangeData &data) override |
void | releaseResources () override |
![]() | |
virtual bool | childMouseEventFilter (QQuickItem *item, QEvent *event) |
virtual void | classBegin () override |
virtual void | componentComplete () override |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual bool | event (QEvent *ev) override |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
virtual void | geometryChange (const QRectF &newGeometry, const QRectF &oldGeometry) |
bool | heightValid () const const |
virtual void | hoverEnterEvent (QHoverEvent *event) |
virtual void | hoverLeaveEvent (QHoverEvent *event) |
virtual void | hoverMoveEvent (QHoverEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
bool | isComponentComplete () const const |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | mouseUngrabEvent () |
virtual void | touchEvent (QTouchEvent *event) |
virtual void | touchUngrabEvent () |
void | updateInputMethod (Qt::InputMethodQueries queries) |
virtual void | updatePolish () |
virtual void | wheelEvent (QWheelEvent *event) |
bool | widthValid () const const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | Flag |
enum | ItemChange |
enum | TransformOrigin |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
Bottom | |
BottomLeft | |
BottomRight | |
Center | |
typedef | Flags |
ItemAcceptsDrops | |
ItemAcceptsInputMethod | |
ItemActiveFocusHasChanged | |
ItemAntialiasingHasChanged | |
ItemChildAddedChange | |
ItemChildRemovedChange | |
ItemClipsChildrenToShape | |
ItemDevicePixelRatioHasChanged | |
ItemEnabledHasChanged | |
ItemHasContents | |
ItemIsFocusScope | |
ItemIsViewport | |
ItemObservesViewport | |
ItemOpacityHasChanged | |
ItemParentHasChanged | |
ItemRotationHasChanged | |
ItemSceneChange | |
ItemVisibleHasChanged | |
Left | |
Right | |
Top | |
TopLeft | |
TopRight | |
![]() | |
typedef | QObjectList |
Detailed Description
Renders a thumbnail for the window specified by the winId
property.
This declarative item is able to render a live updating thumbnail for the window specified by the given winId
property. If it is not possible to get the thumbnail, the window's icon is rendered instead or in case that the window Id is invalid a generic fallback icon is used.
The thumbnail does not necessarily fill out the complete geometry as the thumbnail gets scaled keeping the aspect ratio. This means the thumbnail gets rendered into the center of the item's geometry.
Note: live updating thumbnails are only implemented on the X11 platform. On X11 a running compositor is not required as this item takes care of redirecting the window. For technical reasons the window's frame is not included on X11.
If the window closes, the thumbnail does not get destroyed, which allows to have a window close animation.
Example usage:
Import Statement
- Version
- 2.0
Definition at line 68 of file windowthumbnail.h.
Property Documentation
◆ paintedHeight
|
read |
Definition at line 74 of file windowthumbnail.h.
◆ paintedWidth
|
read |
Definition at line 73 of file windowthumbnail.h.
◆ thumbnailAvailable
|
read |
Definition at line 75 of file windowthumbnail.h.
◆ winId
|
readwrite |
Definition at line 72 of file windowthumbnail.h.
Constructor & Destructor Documentation
◆ WindowThumbnail()
|
explicit |
Definition at line 137 of file windowthumbnail.cpp.
◆ ~WindowThumbnail()
|
override |
Definition at line 164 of file windowthumbnail.cpp.
Member Function Documentation
◆ isTextureProvider()
|
overridevirtual |
Reimplemented from QQuickItem.
Definition at line 990 of file windowthumbnail.cpp.
◆ itemChange()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 172 of file windowthumbnail.cpp.
◆ nativeEventFilter()
|
overridevirtual |
Implements QAbstractNativeEventFilter.
Definition at line 354 of file windowthumbnail.cpp.
◆ paintedHeight()
qreal Plasma::WindowThumbnail::paintedHeight | ( | ) | const |
Definition at line 311 of file windowthumbnail.cpp.
◆ paintedWidth()
qreal Plasma::WindowThumbnail::paintedWidth | ( | ) | const |
Definition at line 306 of file windowthumbnail.cpp.
◆ releaseResources()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 210 of file windowthumbnail.cpp.
◆ resetWinId()
void Plasma::WindowThumbnail::resetWinId | ( | ) |
Definition at line 301 of file windowthumbnail.cpp.
◆ setWinId()
void Plasma::WindowThumbnail::setWinId | ( | uint32_t | winId | ) |
Definition at line 278 of file windowthumbnail.cpp.
◆ textureProvider()
|
overridevirtual |
Reimplemented from QQuickItem.
Definition at line 995 of file windowthumbnail.cpp.
◆ thumbnailAvailable()
bool Plasma::WindowThumbnail::thumbnailAvailable | ( | ) | const |
Definition at line 316 of file windowthumbnail.cpp.
◆ updatePaintNode()
|
overridevirtual |
Reimplemented from QQuickItem.
Definition at line 321 of file windowthumbnail.cpp.
◆ winId()
uint32_t Plasma::WindowThumbnail::winId | ( | ) | const |
Definition at line 273 of file windowthumbnail.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:47 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.