kdeui
KLed Class Reference
An LED widget. More...
#include <kled.h>
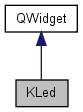
Public Types | |
enum | Look { Flat, Raised, Sunken } |
enum | Shape { Rectangular, Circular } |
enum | State { Off, On } |
Public Slots | |
void | off () |
void | on () |
void | toggle () |
Public Member Functions | |
QColor | color () const |
int | darkFactor () const |
KLed (const QColor &col, KLed::State state, KLed::Look look, KLed::Shape shape, QWidget *parent=0, const char *name=0) | |
KLed (const QColor &col, QWidget *parent=0, const char *name=0) | |
KLed (QWidget *parent=0, const char *name=0) | |
Look | look () const |
virtual QSize | minimumSizeHint () const |
void | setColor (const QColor &color) |
void | setDarkFactor (int darkfactor) |
void | setLook (Look look) |
void | setShape (Shape s) |
void | setState (State state) |
Shape | shape () const |
virtual QSize | sizeHint () const |
State | state () const |
void | toggleState () KDE_DEPRECATED |
~KLed () | |
Protected Member Functions | |
int | ensureRoundLed () |
bool | paintCachedPixmap () |
void | paintEvent (QPaintEvent *) |
virtual void | paintFlat () |
virtual void | paintRect () |
virtual void | paintRectFrame (bool raised) |
virtual void | paintRound () |
virtual void | paintSunken () |
virtual void | virtual_hook (int id, void *data) |
Properties | |
QColor | color |
int | darkFactor |
Look | look |
Shape | shape |
State | state |
Detailed Description
An LED widget.Displays a round or rectangular light emitting diode.
It is configurable to five colors, the two on/off states and three styles (or "looks");
It may display itself in a performant flat view, a round view with light spot or a round view sunken in the screen.

KDE LED Widget
Definition at line 45 of file kled.h.
Member Enumeration Documentation
Displays a flat, round or sunken LED.
Displaying the LED flat is less time and color consuming, but not so nice to see.
The sunken LED itself is (certainly) smaller than the round LED because of the 3 shading circles and is most time consuming. Makes sense for LED > 15x15 pixels.
Timings:
( AMD K5/133, Diamond Stealth 64 PCI Graphics, widgetsize 29x29 )
- flat Approximately 0.7 msec per paint
- round Approximately 2.9 msec per paint
- sunken Approximately 3.3 msec per paint
LED look.
Constructor & Destructor Documentation
KLed::KLed | ( | QWidget * | parent = 0 , |
|
const char * | name = 0 | |||
) |
KLed::KLed | ( | const QColor & | col, | |
KLed::State | state, | |||
KLed::Look | look, | |||
KLed::Shape | shape, | |||
QWidget * | parent = 0 , |
|||
const char * | name = 0 | |||
) |
Constructor with the ledcolor, ledstate, ledlook, the parent widget, and the name.
Differs from above only in the parameters, which configure all settings.
Member Function Documentation
int KLed::darkFactor | ( | ) | const |
int KLed::ensureRoundLed | ( | ) | [protected] |
QSize KLed::minimumSizeHint | ( | void | ) | const [virtual] |
void KLed::off | ( | ) | [slot] |
Sets the state of the widget to Off.
The widget will be painted immediately.
- See also:
- on() toggle() toggleState() setState()
void KLed::on | ( | ) | [slot] |
Sets the state of the widget to On.
The widget will be painted immediately.
- See also:
- off() toggle() toggleState() setState()
bool KLed::paintCachedPixmap | ( | ) | [protected] |
void KLed::paintEvent | ( | QPaintEvent * | ) | [protected] |
void KLed::paintFlat | ( | ) | [protected, virtual] |
void KLed::paintRect | ( | ) | [protected, virtual] |
void KLed::paintRectFrame | ( | bool | raised | ) | [protected, virtual] |
void KLed::paintRound | ( | ) | [protected, virtual] |
void KLed::paintSunken | ( | ) | [protected, virtual] |
void KLed::setColor | ( | const QColor & | color | ) |
Set the color of the widget.
The Color is shown with the KLed::On state. The KLed::Off state is shown with QColor.dark() method
The widget calls the update() method, so it will be updated when entering the main event loop.
- See also:
- Color
- Parameters:
-
color New color of the LED. Sets the LED color.
void KLed::setDarkFactor | ( | int | darkfactor | ) |
Sets the factor to darken the LED in OFF state.
Same as QColor::dark(). "darkfactor should be greater than 100, else the LED gets lighter in OFF state. Defaults to 300.
- See also:
- QColor
- Parameters:
-
darkfactor sets the factor to darken the LED. sets the factor to darken the LED.
void KLed::setLook | ( | Look | look | ) |
Sets the color of the widget.
The Color is shown with the KLed::On state. darkcolor is explicidly used for the off state of the LED. Normally you don't have to use this method, the setColor(const QColor& color) is sufficient for the task.
The widget calls the update() method, so it will be updated when entering the main event loop.
- See also:
- Color setColor()
- Parameters:
-
color New color of the LED used for on state. darkcolor Dark color of the LED used for off state. Sets the light and dark LED color. void setColor(const QColor& color, const QColor& darkcolor); Sets the look of the widget.
- See also:
- Look
- Parameters:
-
look New look of the LED. Sets LED look.
void KLed::setShape | ( | KLed::Shape | s | ) |
void KLed::setState | ( | State | state | ) |
Shape KLed::shape | ( | ) | const |
QSize KLed::sizeHint | ( | void | ) | const [virtual] |
State KLed::state | ( | ) | const |
void KLed::toggle | ( | ) | [slot] |
void KLed::toggleState | ( | ) |
Toggle the state of the LED from Off to On and vice versa.
The widget will be repainted when returning to the main event loop. Toggles LED on->off / off->on.
- Deprecated:
- , use toggle() instead.
void KLed::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Property Documentation
KLed::Look KLed::look [read, write] |
KLed::Shape KLed::shape [read, write] |
KLed::State KLed::state [read, write] |
The documentation for this class was generated from the following files: