kdeui
KRuler Class Reference
A ruler widget. More...
#include <kruler.h>
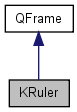
Public Types | |
enum | MetricStyle { Custom = 0, Pixel, Inch, Millimetres, Centimetres, Metres } |
Public Slots | |
void | slotEndOffset (int) |
void | slotNewOffset (int) |
void | slotNewValue (int) |
Public Member Functions | |
int | bigMarkDistance () const |
QString | endLabel () const |
int | endOffset () const |
KRuler (Orientation orient, int widgetWidth, QWidget *parent=0, const char *name=0, WFlags f=0) | |
KRuler (Orientation orient, QWidget *parent=0, const char *name=0, WFlags f=0) | |
KRuler (QWidget *parent=0, const char *name=0) | |
int | length () const |
bool | lengthFixed () const |
int | littleMarkDistance () const |
int | maxValue () const |
int | mediumMarkDistance () const |
int | minValue () const |
int | offset () const |
double | pixelPerMark () const |
void | setBigMarkDistance (int) |
void | setEndLabel (const QString &) |
void | setLength (int) |
void | setLengthFixed (bool fix) |
void | setLittleMarkDistance (int) |
void | setMaxValue (int) |
void | setMediumMarkDistance (int) |
void | setMinValue (int) |
void | setOffset (int offset) |
void | setPixelPerMark (double rate) |
void | setRange (int min, int max) |
void | setRulerMetricStyle (KRuler::MetricStyle) |
void | setShowBigMarks (bool) |
void | setShowEndLabel (bool) |
void | setShowEndMarks (bool) |
void | setShowLittleMarks (bool) |
void | setShowMediumMarks (bool) |
void | setShowPointer (bool) |
void | setShowTinyMarks (bool) |
void | setTinyMarkDistance (int) |
void | setValue (int) |
void | setValuePerBigMark (int) KDE_DEPRECATED |
void | setValuePerLittleMark (int) KDE_DEPRECATED |
void | setValuePerMediumMark (int) KDE_DEPRECATED |
bool | showBigMarks () const |
bool | showEndLabel () const |
bool | showEndMarks () const |
bool | showLittleMarks () const |
bool | showMediumMarks () const |
bool | showPointer () const |
bool | showTinyMarks () const |
void | slideDown (int count=1) |
void | slideUp (int count=1) |
int | tinyMarkDistance () const |
int | value () const |
~KRuler () | |
Protected Member Functions | |
virtual void | drawContents (QPainter *) |
virtual void | virtual_hook (int id, void *data) |
Properties | |
int | bigMarkDistance |
bool | lengthFixed |
int | littleMarkDistance |
int | maxValue |
int | mediumMarkDistance |
int | minValue |
double | pixelPerMark |
bool | showBigMarks |
bool | showEndLabel |
bool | showLittleMarks |
bool | showMediumMarks |
bool | showPointer |
bool | showTinyMarks |
int | tinyMarkDistance |
int | value |
Detailed Description
A ruler widget.The vertical ruler looks similar to this:
meters inches ------ <--- end mark ---> ------ -- - -- <---little mark---> -- -- - -- --- --- <---medium mark - -- -- -- tiny mark----> - -- ---- -- - ---- <-----big mark -- -- - |>-- <--ruler pointer--> |>--
There are tiny marks, little marks, medium marks, and big marks along the ruler.
To receive mouse clicks or mouse moves, the class has to be overloaded.
For performance reasons, the public methods don't call QWidget::repaint(). (Slots do, see documentation below.) All the changed settings will be painted once after leaving to the main event loop. For performance painting the slot methods should be used, they do a fast QWidget::repaint() call after changing the values. For setting multiple values like minValue(), maxValue(), offset() etc. using the public methods is recommended so the widget will be painted only once when entering the main event loop.
A ruler widget.
Definition at line 71 of file kruler.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
KRuler::KRuler | ( | QWidget * | parent = 0 , |
|
const char * | name = 0 | |||
) |
The style (or look) of the ruler.
Constructs a horizontal ruler.
Definition at line 94 of file kruler.cpp.
KRuler::KRuler | ( | Orientation | orient, | |
QWidget * | parent = 0 , |
|||
const char * | name = 0 , |
|||
WFlags | f = 0 | |||
) |
Constructs a ruler with orientation orient
.
parent
, name
and f
are passed to QFrame. The default look is a raised widget but may be changed with the inherited QFrame methods.
- Parameters:
-
orient Orientation of the ruler. parent Will be handed over to QFrame. name Will be handed over to QFrame. f Will be handed over to QFrame.
Definition at line 104 of file kruler.cpp.
KRuler::KRuler | ( | Orientation | orient, | |
int | widgetWidth, | |||
QWidget * | parent = 0 , |
|||
const char * | name = 0 , |
|||
WFlags | f = 0 | |||
) |
Constructs a ruler with orientation orient
and initial width widgetWidth
.
The width sets the fixed width of the widget. This is useful if you want to draw the ruler bigger or smaller than the default size. Note: The size of the marks doesn't change. parent
, name
and f
are passed to QFrame.
- Parameters:
-
orient Orientation of the ruler. widgetWidth Fixed width of the widget. parent Will be handed over to QFrame. name Will be handed over to QFrame. f Will be handed over to QFrame.
Definition at line 118 of file kruler.cpp.
KRuler::~KRuler | ( | ) |
Member Function Documentation
int KRuler::bigMarkDistance | ( | ) | const [inline] |
Returns the distance between big marks.
void KRuler::drawContents | ( | QPainter * | p | ) | [protected, virtual] |
QString KRuler::endLabel | ( | ) | const |
Definition at line 374 of file kruler.cpp.
int KRuler::endOffset | ( | ) | const |
Definition at line 512 of file kruler.cpp.
int KRuler::length | ( | ) | const |
Definition at line 481 of file kruler.cpp.
bool KRuler::lengthFixed | ( | ) | const |
int KRuler::littleMarkDistance | ( | ) | const [inline] |
Returns the distance between little marks.
int KRuler::maxValue | ( | ) | const [inline] |
Returns the maximal value of the ruler pointer.
int KRuler::mediumMarkDistance | ( | ) | const [inline] |
int KRuler::minValue | ( | ) | const [inline] |
Returns the minimal value of the ruler pointer.
int KRuler::offset | ( | ) | const [inline] |
double KRuler::pixelPerMark | ( | ) | const [inline] |
Returns the number of pixels between two base marks.
void KRuler::setBigMarkDistance | ( | int | dist | ) |
Sets distance between big marks.
For English (inches) or metric styles it is twice the medium mark distance.
Definition at line 224 of file kruler.cpp.
void KRuler::setEndLabel | ( | const QString & | label | ) |
Sets the label this is drawn at the beginning of the visible part of the ruler to label
.
Definition at line 360 of file kruler.cpp.
void KRuler::setLength | ( | int | length | ) |
Sets the length of the ruler, i.e.
the difference between the begin mark and the end mark of the ruler.
Same as (width() - offset())
when the length is not locked, it gets adjusted with the length of the widget.
Definition at line 465 of file kruler.cpp.
void KRuler::setLengthFixed | ( | bool | fix | ) |
Locks the length of the ruler, i.e.
the difference between the two end marks doesn't change when the widget is resized.
- Parameters:
-
fix fixes the length, if true
Definition at line 491 of file kruler.cpp.
void KRuler::setLittleMarkDistance | ( | int | dist | ) |
Sets the distance between little marks.
The default value is 1 in the metric system and 2 in the English (inches) system.
Definition at line 206 of file kruler.cpp.
void KRuler::setMaxValue | ( | int | value | ) |
Sets the maximum value of the ruler pointer (default is 100).
This method calls update() so that the widget is painted after leaving to the main event loop.
Definition at line 172 of file kruler.cpp.
void KRuler::setMediumMarkDistance | ( | int | dist | ) |
Sets the distance between medium marks.
For English (inches) styles it defaults to twice the little mark distance. For metric styles it defaults to five times the little mark distance.
Definition at line 215 of file kruler.cpp.
void KRuler::setMinValue | ( | int | value | ) |
Sets the minimal value of the ruler pointer (default is 0).
This method calls update() so that the widget is painted after leaving to the main event loop.
Definition at line 163 of file kruler.cpp.
void KRuler::setOffset | ( | int | offset | ) |
Sets the ruler slide offset.
This is like slideup() or slidedown() with an absolute offset from the start of the ruler.
- Parameters:
-
offset Number of pixel to move the ruler up or left from the beginning
Definition at line 503 of file kruler.cpp.
void KRuler::setPixelPerMark | ( | double | rate | ) |
Sets the number of pixels between two base marks.
Calling this method stretches or shrinks your ruler.
For pixel display ( MetricStyle) the value is 10.0 marks per pixel ;-) For English (inches) it is 9.0, and for centimetres ~2.835 -> 3.0 . If you want to magnify your part of display, you have to adjust the mark distance here
. Notice: The double type is only supported to give the possibility of having some double values. It should be used with care. Using values below 10.0 shows visible jumps of markpositions (e.g. 2.345). Using whole numbers is highly recommended. To use int
values use setPixelPerMark((int)your_int_value); default: 1 mark per 10 pixels
Definition at line 457 of file kruler.cpp.
void KRuler::setRange | ( | int | min, | |
int | max | |||
) |
Sets minimum and maximum values of the ruler pointer.
This method calls update() so that the widget is painted after leaving to the main event loop.
Definition at line 181 of file kruler.cpp.
void KRuler::setRulerMetricStyle | ( | KRuler::MetricStyle | style | ) |
Sets up the necessary tasks for the provided styles.
A convenience method.
Definition at line 380 of file kruler.cpp.
void KRuler::setShowBigMarks | ( | bool | show | ) |
void KRuler::setShowEndLabel | ( | bool | show | ) |
Show/hide number values of the little marks.
Default is false
. Show/hide number values of the medium marks.
Default is false
. Show/hide number values of the big marks.
Default is false
. Show/hide number values of the end marks.
Default is false
.
Definition at line 343 of file kruler.cpp.
void KRuler::setShowEndMarks | ( | bool | show | ) |
void KRuler::setShowLittleMarks | ( | bool | show | ) |
void KRuler::setShowMediumMarks | ( | bool | show | ) |
void KRuler::setShowPointer | ( | bool | show | ) |
void KRuler::setShowTinyMarks | ( | bool | show | ) |
void KRuler::setTinyMarkDistance | ( | int | dist | ) |
Sets the distance between tiny marks.
This is mostly used in the English system (inches) with distance of 1.
Definition at line 197 of file kruler.cpp.
void KRuler::setValue | ( | int | value | ) |
Sets the value of the ruler pointer.
The value is indicated by painting the ruler pointer at the corresponding position. This method calls update() so that the widget is painted after leaving to the main event loop.
Definition at line 190 of file kruler.cpp.
void KRuler::setValuePerBigMark | ( | int | ) |
- Deprecated:
- This method has no effect other than an update. Do not use.
Definition at line 337 of file kruler.cpp.
void KRuler::setValuePerLittleMark | ( | int | ) |
- Deprecated:
- This method has no effect other than an update. Do not use.
Definition at line 325 of file kruler.cpp.
void KRuler::setValuePerMediumMark | ( | int | ) |
- Deprecated:
- This method has no effect other than an update. Do not use.
Definition at line 331 of file kruler.cpp.
bool KRuler::showBigMarks | ( | ) | const |
bool KRuler::showEndLabel | ( | ) | const |
bool KRuler::showEndMarks | ( | ) | const |
Definition at line 303 of file kruler.cpp.
bool KRuler::showLittleMarks | ( | ) | const |
bool KRuler::showMediumMarks | ( | ) | const |
bool KRuler::showPointer | ( | ) | const |
bool KRuler::showTinyMarks | ( | ) | const |
void KRuler::slideDown | ( | int | count = 1 |
) |
Sets the number of pixels by which the ruler may slide down or right.
The number of pixels moved is realive to the previous position. The Method makes sense for updating a ruler, which is working with a scrollbar.
This doesn't affect the position of the ruler pointer. Only the visible part of the ruler is moved.
- Parameters:
-
count Number of pixel moving up or left relative to the previous position
Definition at line 531 of file kruler.cpp.
void KRuler::slideUp | ( | int | count = 1 |
) |
Sets the number of pixels by which the ruler may slide up or left.
The number of pixels moved is realive to the previous position. The Method makes sense for updating a ruler, which is working with a scrollbar.
This doesn't affect the position of the ruler pointer. Only the visible part of the ruler is moved.
- Parameters:
-
count Number of pixel moving up or left relative to the previous position
Definition at line 522 of file kruler.cpp.
void KRuler::slotEndOffset | ( | int | offset | ) | [slot] |
Definition at line 578 of file kruler.cpp.
void KRuler::slotNewOffset | ( | int | _offset | ) | [slot] |
Sets the ruler marks to a new position.
The pointer is NOT updated. QWidget::repaint() is called afterwards.
Definition at line 567 of file kruler.cpp.
void KRuler::slotNewValue | ( | int | _value | ) | [slot] |
Sets the pointer to a new position.
The offset is NOT updated. QWidget::repaint() is called afterwards.
Definition at line 541 of file kruler.cpp.
int KRuler::tinyMarkDistance | ( | ) | const [inline] |
Returns the distance between tiny marks.
int KRuler::value | ( | ) | const [inline] |
void KRuler::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 739 of file kruler.cpp.
Property Documentation
The documentation for this class was generated from the following files: