marble
#include <MarbleModel.h>
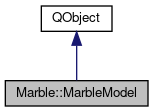
Public Slots | |
void | assignFillColors (const QString &filePath) |
void | clearPersistentTileCache () |
void | setPersistentTileCacheLimit (quint64 kiloBytes) |
void | setTrackedPlacemark (const GeoDataPlacemark *placemark) |
void | updateProperty (const QString &property, bool value) |
Signals | |
void | creatingTilesStart (TileCreator *, const QString &name, const QString &description) |
void | homeChanged (const GeoDataCoordinates &newHomePoint) |
void | themeChanged (QString mapTheme) |
void | trackedPlacemarkChanged (const GeoDataPlacemark *placemark) |
void | workOfflineChanged () |
Public Member Functions | |
MarbleModel (QObject *parent=0) | |
virtual | ~MarbleModel () |
void | addGeoDataFile (const QString &filename) |
void | addGeoDataString (const QString &data, const QString &key="data") |
void | assignNewStyle (const QString &filePath, GeoDataStyle *style) |
BookmarkManager * | bookmarkManager () |
MarbleClock * | clock () |
const MarbleClock * | clock () const |
QDateTime | clockDateTime () const |
int | clockSpeed () const |
int | clockTimezone () const |
HttpDownloadManager * | downloadManager () |
const HttpDownloadManager * | downloadManager () const |
ElevationModel * | elevationModel () |
const ElevationModel * | elevationModel () const |
FileManager * | fileManager () |
QAbstractItemModel * | groundOverlayModel () |
const QAbstractItemModel * | groundOverlayModel () const |
void | home (qreal &lon, qreal &lat, int &zoom) const |
QTextDocument * | legend () |
GeoSceneDocument * | mapTheme () |
const GeoSceneDocument * | mapTheme () const |
QString | mapThemeId () const |
quint64 | persistentTileCacheLimit () const |
QAbstractItemModel * | placemarkModel () |
const QAbstractItemModel * | placemarkModel () const |
QItemSelectionModel * | placemarkSelectionModel () |
const Planet * | planet () const |
QString | planetId () const |
QString | planetName () const |
qreal | planetRadius () const |
const PluginManager * | pluginManager () const |
PluginManager * | pluginManager () |
PositionTracking * | positionTracking () const |
void | removeGeoData (const QString &key) |
RoutingManager * | routingManager () |
const RoutingManager * | routingManager () const |
void | setClockDateTime (const QDateTime &datetime) |
void | setClockSpeed (int speed) |
void | setClockTimezone (int timeInSec) |
void | setHome (qreal lon, qreal lat, int zoom=1050) |
void | setHome (const GeoDataCoordinates &homePoint, int zoom=1050) |
void | setLegend (QTextDocument *document) |
void | setMapThemeId (const QString &mapThemeId) |
void | setWorkOffline (bool workOffline) |
SunLocator * | sunLocator () |
const SunLocator * | sunLocator () const |
const GeoDataPlacemark * | trackedPlacemark () const |
GeoDataTreeModel * | treeModel () |
const GeoDataTreeModel * | treeModel () const |
quint64 | volatileTileCacheLimit () const |
bool | workOffline () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Properties | |
QString | mapThemeId |
bool | workOffline |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
The data model (not based on QAbstractModel) for a MarbleWidget.
This class provides a data storage and indexer that can be displayed in a MarbleWidget. It contains 3 different datatypes: tiles which provide the background, vectors which provide things like country borders and coastlines and placemarks which can show points of interest, such as cities, mountain tops or the poles.
The tiles provide the background of the image and can be for instance height and depth fields, magnetic strength, topographic data or anything else that is area based.
The vectors provide things like country borders and coastlines. They are stored in separate files and can be added or removed at anytime.
The placemarks contain points of interest, such as cities, mountain tops or the poles. These are sorted by size (for cities) and category (capitals, other important cities, less important cities, etc) and are displayed with different color or shape like square or round.
- See also
- MarbleWidget
Definition at line 97 of file MarbleModel.h.
Constructor & Destructor Documentation
|
explicit |
Construct a new MarbleModel.
- Parameters
-
parent the parent widget
Definition at line 169 of file MarbleModel.cpp.
|
virtual |
Definition at line 206 of file MarbleModel.cpp.
Member Function Documentation
void Marble::MarbleModel::addGeoDataFile | ( | const QString & | filename | ) |
Handle file loading into the treeModel.
- Parameters
-
filename the file to load
Definition at line 725 of file MarbleModel.cpp.
Handle raw data loading into the treeModel.
- Parameters
-
data the raw data to load key the name to remove this raw data later
Definition at line 731 of file MarbleModel.cpp.
|
slot |
Definition at line 754 of file MarbleModel.cpp.
void Marble::MarbleModel::assignNewStyle | ( | const QString & | filePath, |
GeoDataStyle * | style | ||
) |
Definition at line 417 of file MarbleModel.cpp.
BookmarkManager * Marble::MarbleModel::bookmarkManager | ( | ) |
return instance of BookmarkManager
Definition at line 213 of file MarbleModel.cpp.
|
slot |
Definition at line 562 of file MarbleModel.cpp.
MarbleClock * Marble::MarbleModel::clock | ( | ) |
Definition at line 537 of file MarbleModel.cpp.
const MarbleClock * Marble::MarbleModel::clock | ( | ) | const |
Definition at line 542 of file MarbleModel.cpp.
QDateTime Marble::MarbleModel::clockDateTime | ( | ) | const |
Definition at line 690 of file MarbleModel.cpp.
int Marble::MarbleModel::clockSpeed | ( | ) | const |
Definition at line 695 of file MarbleModel.cpp.
int Marble::MarbleModel::clockTimezone | ( | ) | const |
Definition at line 710 of file MarbleModel.cpp.
|
signal |
Signal that the MarbleModel has started to create a new set of tiles.
- Parameters
-
HttpDownloadManager * Marble::MarbleModel::downloadManager | ( | ) |
Return the downloadmanager to load missing tiles.
- Returns
- the HttpDownloadManager instance.
Definition at line 466 of file MarbleModel.cpp.
const HttpDownloadManager * Marble::MarbleModel::downloadManager | ( | ) | const |
Definition at line 471 of file MarbleModel.cpp.
ElevationModel * Marble::MarbleModel::elevationModel | ( | ) |
Definition at line 853 of file MarbleModel.cpp.
const ElevationModel * Marble::MarbleModel::elevationModel | ( | ) | const |
Definition at line 858 of file MarbleModel.cpp.
FileManager * Marble::MarbleModel::fileManager | ( | ) |
Definition at line 517 of file MarbleModel.cpp.
QAbstractItemModel * Marble::MarbleModel::groundOverlayModel | ( | ) |
Definition at line 497 of file MarbleModel.cpp.
const QAbstractItemModel * Marble::MarbleModel::groundOverlayModel | ( | ) | const |
Definition at line 502 of file MarbleModel.cpp.
void Marble::MarbleModel::home | ( | qreal & | lon, |
qreal & | lat, | ||
int & | zoom | ||
) | const |
get the home point
- Parameters
-
lon the longitude of the home point. lat the latitude of the home point. zoom the default zoom level of the home point.
Definition at line 446 of file MarbleModel.cpp.
|
signal |
QTextDocument * Marble::MarbleModel::legend | ( | ) |
Definition at line 715 of file MarbleModel.cpp.
GeoSceneDocument * Marble::MarbleModel::mapTheme | ( | ) |
Definition at line 228 of file MarbleModel.cpp.
const GeoSceneDocument * Marble::MarbleModel::mapTheme | ( | ) | const |
Definition at line 233 of file MarbleModel.cpp.
QString Marble::MarbleModel::mapThemeId | ( | ) | const |
Return the name of the current map theme.
- Returns
- the identifier of the current MapTheme. To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: maptheme = "earth/bluemarble/bluemarble.dgml"
quint64 Marble::MarbleModel::persistentTileCacheLimit | ( | ) | const |
Returns the limit in kilobytes of the persistent (on hard disc) tile cache.
- Returns
- the limit of persistent tile cache in kilobytes.
Definition at line 557 of file MarbleModel.cpp.
QAbstractItemModel * Marble::MarbleModel::placemarkModel | ( | ) |
Definition at line 487 of file MarbleModel.cpp.
const QAbstractItemModel * Marble::MarbleModel::placemarkModel | ( | ) | const |
Definition at line 492 of file MarbleModel.cpp.
QItemSelectionModel * Marble::MarbleModel::placemarkSelectionModel | ( | ) |
Definition at line 507 of file MarbleModel.cpp.
const Planet * Marble::MarbleModel::planet | ( | ) | const |
Returns the planet object for the current map.
- Returns
- the planet object for the current map
Definition at line 644 of file MarbleModel.cpp.
QString Marble::MarbleModel::planetId | ( | ) | const |
Definition at line 532 of file MarbleModel.cpp.
QString Marble::MarbleModel::planetName | ( | ) | const |
Definition at line 527 of file MarbleModel.cpp.
qreal Marble::MarbleModel::planetRadius | ( | ) | const |
Definition at line 522 of file MarbleModel.cpp.
const PluginManager * Marble::MarbleModel::pluginManager | ( | ) | const |
Definition at line 634 of file MarbleModel.cpp.
PluginManager * Marble::MarbleModel::pluginManager | ( | ) |
Definition at line 639 of file MarbleModel.cpp.
PositionTracking * Marble::MarbleModel::positionTracking | ( | ) | const |
Definition at line 512 of file MarbleModel.cpp.
void Marble::MarbleModel::removeGeoData | ( | const QString & | key | ) |
Remove the file or raw data from the treeModel.
- Parameters
-
key either the file name or the key for raw data
Definition at line 736 of file MarbleModel.cpp.
RoutingManager * Marble::MarbleModel::routingManager | ( | ) |
Definition at line 675 of file MarbleModel.cpp.
const RoutingManager * Marble::MarbleModel::routingManager | ( | ) | const |
Definition at line 680 of file MarbleModel.cpp.
void Marble::MarbleModel::setClockDateTime | ( | const QDateTime & | datetime | ) |
Definition at line 685 of file MarbleModel.cpp.
void Marble::MarbleModel::setClockSpeed | ( | int | speed | ) |
Definition at line 700 of file MarbleModel.cpp.
void Marble::MarbleModel::setClockTimezone | ( | int | timeInSec | ) |
Definition at line 705 of file MarbleModel.cpp.
void Marble::MarbleModel::setHome | ( | qreal | lon, |
qreal | lat, | ||
int | zoom = 1050 |
||
) |
Set the home point.
- Parameters
-
lon the longitude of the new home point. lat the latitude of the new home point. zoom the default zoom level for the new home point.
Definition at line 452 of file MarbleModel.cpp.
void Marble::MarbleModel::setHome | ( | const GeoDataCoordinates & | homePoint, |
int | zoom = 1050 |
||
) |
Set the home point.
- Parameters
-
homePoint the new home point. zoom the default zoom level for the new home point.
Definition at line 459 of file MarbleModel.cpp.
void Marble::MarbleModel::setLegend | ( | QTextDocument * | document | ) |
Definition at line 720 of file MarbleModel.cpp.
void Marble::MarbleModel::setMapThemeId | ( | const QString & | mapThemeId | ) |
Set a new map theme to use.
- Parameters
-
mapThemeId the identifier of the new map theme
This function sets the map theme, i.e. combination of tile set and color scheme to use. If the map theme is not previously used, some basic tiles are created and a progress dialog is shown.
The ID of the new maptheme. To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: maptheme = "earth/bluemarble/bluemarble.dgml"
If the sourcefile of data matches any in the currentDatasets then there is no need to parse the file again just update the style i.e. <brush> and <pen> values of already parsed file. assignNewStyle() does that
data->colors() are the colorMap values from dgml file. If this is not empty then we are supposed to assign every placemark a different style by giving it a color from colorMap values based on color index of that placemark. See assignFillColors() for details. So, we need to send an empty style to fileManeger otherwise the FileLoader::createFilterProperties() will overwrite the parsed value of color index ( GeoDataPolyStyle::d->m_colorIndex ).
if already parsed sourcefile doesn't contains colorMap then assignNewStyle otherwise assignFillColors.
Definition at line 247 of file MarbleModel.cpp.
|
slot |
Set the limit of the persistent (on hard disc) tile cache.
- Parameters
-
bytes The limit in kilobytes, 0 means no limit.
Definition at line 607 of file MarbleModel.cpp.
|
slot |
Change the placemark tracked by this model.
Definition at line 623 of file MarbleModel.cpp.
void Marble::MarbleModel::setWorkOffline | ( | bool | workOffline | ) |
Definition at line 843 of file MarbleModel.cpp.
SunLocator * Marble::MarbleModel::sunLocator | ( | ) |
Definition at line 547 of file MarbleModel.cpp.
const SunLocator * Marble::MarbleModel::sunLocator | ( | ) | const |
Definition at line 552 of file MarbleModel.cpp.
|
signal |
Signal that the map theme has changed, and to which theme.
- Parameters
-
mapTheme the identifier of the new map theme.
- See also
- mapTheme
- setMapTheme
const GeoDataPlacemark * Marble::MarbleModel::trackedPlacemark | ( | ) | const |
Returns the placemark being tracked by this model or 0 if no placemark is currently tracked.
Definition at line 629 of file MarbleModel.cpp.
|
signal |
Emitted when the placemark tracked by this model has changed.
- See also
- setTrackedPlacemark(), trackedPlacemark()
GeoDataTreeModel * Marble::MarbleModel::treeModel | ( | ) |
Return the list of Placemarks as a QAbstractItemModel *.
- Returns
- a list of all Placemarks in the MarbleModel.
Definition at line 477 of file MarbleModel.cpp.
const GeoDataTreeModel * Marble::MarbleModel::treeModel | ( | ) | const |
Definition at line 482 of file MarbleModel.cpp.
|
slot |
Definition at line 741 of file MarbleModel.cpp.
quint64 Marble::MarbleModel::volatileTileCacheLimit | ( | ) | const |
Returns the limit of the volatile (in RAM) tile cache.
- Returns
- the cache limit in kilobytes
bool Marble::MarbleModel::workOffline | ( | ) | const |
|
signal |
Property Documentation
|
readwrite |
Definition at line 103 of file MarbleModel.h.
|
readwrite |
Definition at line 104 of file MarbleModel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:13:45 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.