palapeli/libpala
#include <Pala/Slicer>
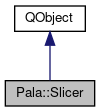
Public Types | |
enum | SlicerFlag { NoFlags = 0x0, AllowFullTransparency = 0x1 } |
Public Member Functions | |
Slicer (QObject *parent=0, const QVariantList &args=QVariantList()) | |
virtual | ~Slicer () |
SlicerFlags | flags () const |
QList< const Pala::SlicerMode * > | modes () const |
bool | process (Pala::SlicerJob *job) |
QMap< QByteArray, const Pala::SlicerProperty * > | properties () const |
QList< const Pala::SlicerProperty * > | propertyList () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
void | addMode (Pala::SlicerMode *mode) |
void | addProperty (const QByteArray &key, Pala::SlicerProperty *property) |
virtual bool | run (Pala::SlicerJob *job)=0 |
void | setFlags (SlicerFlags flags) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Representation of a slicing algorithm.
This class represents a slicing algorithm. It has to be subclassed by slicing plugin developers. Subclasses need to implement
- the constructor (where the slicer's properties and, if used, the modes have to be instantiated)
- the run() method (where the actual slicing is performed).
Additionally, the class must be flagged as entry point into the plugin, with the following code:
In the last line, put inside the string literal the file name of the plugin library (e.g. myslicer is the name for a library libmyslicer.so on unixoid systems, or myslicer.dll on Windows systems).
Member Enumeration Documentation
Behavioral flags of a slicer.
These flags can be used to programmatically configure the behavior of libpala for a single slicer. You should only set the slicer's flags once in the constructor, and not modify it later. (The latter might cause unexpected behavior.)
- See also
- setFlags
Constructor & Destructor Documentation
|
explicit |
Constructs a new Slicer object.
In any subclass, the constructor signature has to be the same (due to the way the plugin loader works). The arguments should be passed to this constructor and ignored by the subclass implementation, as their format might change without notice in future versions.
Definition at line 36 of file slicer.cpp.
|
virtual |
Deletes this slicer, and all properties and modes which have been added with addProperty() and addMode().
Definition at line 43 of file slicer.cpp.
Member Function Documentation
|
protected |
Add an operation mode to this slicer.
The slicer will take care of destructing the given Pala::SlicerMode instance when it is destructed. You may use modes e.g. if your slicer includes different slicing algorithms at once which might need a different set of properties (see Pala::SlicerMode documentation for details). If you choose not to use modes, just ignore this function and all other functions concerning modes.
- Warning
- It is not safe to add new modes outside the constructor of a Pala::Slicer subclass.
- Since
- libpala 1.2 (KDE SC 4.6)
Definition at line 90 of file slicer.cpp.
|
protected |
Add the given property to the property list of this slicer.
The slicer will take care of destructing the given Pala::SlicerProperty instance when it is destructed. Use this method in the subclass constructors to fill the slicer with properties. Properties let the user control how the slicing is done.
- Warning
- It is not safe to add new properties outside the constructor of a Pala::Slicer subclass.
Definition at line 73 of file slicer.cpp.
Pala::Slicer::SlicerFlags Pala::Slicer::flags | ( | ) | const |
Definition at line 68 of file slicer.cpp.
QList< const Pala::SlicerMode * > Pala::Slicer::modes | ( | ) | const |
- Since
- libpala 1.2 (KDE SC 4.6)
Definition at line 50 of file slicer.cpp.
bool Pala::Slicer::process | ( | Pala::SlicerJob * | job | ) |
Definition at line 111 of file slicer.cpp.
QMap< QByteArray, const Pala::SlicerProperty * > Pala::Slicer::properties | ( | ) | const |
- Deprecated:
- because sorting order is not right
Definition at line 55 of file slicer.cpp.
QList< const Pala::SlicerProperty * > Pala::Slicer::propertyList | ( | ) | const |
- Since
- libpala 1.2 (KDE SC 4.6)
Definition at line 63 of file slicer.cpp.
|
protectedpure virtual |
The slicing algorithm.
Implement the slicing algorithm in this method. The slicing algorithm should always respect the current values of the slicer's properties, as defined through the addProperty() method.
- Returns
- whether the operation has been completed successfully
- See also
- Pala::SlicerJob
|
protected |
- See also
- Pala::Slicer::SlicerFlags
Definition at line 106 of file slicer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:19:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.