KArchive
#include <KArchive>
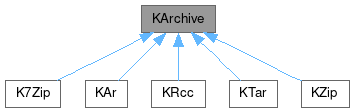
Public Member Functions | |
bool | addLocalDirectory (const QString &path, const QString &destName) |
bool | addLocalFile (const QString &fileName, const QString &destName) |
virtual bool | close () |
QIODevice * | device () const |
const KArchiveDirectory * | directory () const |
QString | errorString () const |
QString | fileName () const |
bool | finishWriting (qint64 size) |
bool | isOpen () const |
QIODevice::OpenMode | mode () const |
virtual bool | open (QIODevice::OpenMode mode) |
bool | prepareWriting (const QString &name, const QString &user, const QString &group, qint64 size, mode_t perm=0100644, const QDateTime &atime=QDateTime(), const QDateTime &mtime=QDateTime(), const QDateTime &ctime=QDateTime()) |
bool | writeData (const char *data, qint64 size) |
bool | writeData (QByteArrayView data) |
bool | writeDir (const QString &name, const QString &user=QString(), const QString &group=QString(), mode_t perm=040755, const QDateTime &atime=QDateTime(), const QDateTime &mtime=QDateTime(), const QDateTime &ctime=QDateTime()) |
bool | writeFile (const QString &name, QByteArrayView data, mode_t perm=0100644, const QString &user=QString(), const QString &group=QString(), const QDateTime &atime=QDateTime(), const QDateTime &mtime=QDateTime(), const QDateTime &ctime=QDateTime()) |
bool | writeSymLink (const QString &name, const QString &target, const QString &user=QString(), const QString &group=QString(), mode_t perm=0120755, const QDateTime &atime=QDateTime(), const QDateTime &mtime=QDateTime(), const QDateTime &ctime=QDateTime()) |
Protected Member Functions | |
KArchive (const QString &fileName) | |
KArchive (QIODevice *dev) | |
virtual bool | closeArchive ()=0 |
virtual bool | createDevice (QIODevice::OpenMode mode) |
virtual bool | doFinishWriting (qint64 size)=0 |
virtual bool | doPrepareWriting (const QString &name, const QString &user, const QString &group, qint64 size, mode_t perm, const QDateTime &atime, const QDateTime &mtime, const QDateTime &ctime)=0 |
virtual bool | doWriteData (const char *data, qint64 size) |
virtual bool | doWriteDir (const QString &name, const QString &user, const QString &group, mode_t perm, const QDateTime &atime, const QDateTime &mtime, const QDateTime &ctime)=0 |
virtual bool | doWriteSymLink (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm, const QDateTime &atime, const QDateTime &mtime, const QDateTime &ctime)=0 |
KArchiveDirectory * | findOrCreate (const QString &path) |
virtual bool | openArchive (QIODevice::OpenMode mode)=0 |
virtual KArchiveDirectory * | rootDir () |
void | setDevice (QIODevice *dev) |
void | setErrorString (const QString &errorStr) |
void | setRootDir (KArchiveDirectory *rootDir) |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
KArchive is a base class for reading and writing archives.
generic class for reading/writing archives
Definition at line 40 of file karchive.h.
Constructor & Destructor Documentation
◆ KArchive() [1/2]
|
explicitprotected |
Base constructor (protected since this is a pure virtual class).
- Parameters
-
fileName is a local path (e.g. "/tmp/myfile.ext"), from which the archive will be read from, or into which the archive will be written, depending on the mode given to open().
Definition at line 115 of file karchive.cpp.
◆ KArchive() [2/2]
|
explicitprotected |
Base constructor (protected since this is a pure virtual class).
- Parameters
-
dev the I/O device where the archive reads its data Note that this can be a file, but also a data buffer, a compression filter, etc. For a file in writing mode it is better to use the other constructor though, to benefit from the use of QSaveFile when saving.
Definition at line 126 of file karchive.cpp.
◆ ~KArchive()
|
virtual |
Definition at line 135 of file karchive.cpp.
Member Function Documentation
◆ addLocalDirectory()
Writes a local directory into the archive, including all its contents, recursively.
Calls addLocalFile for each file to be added.
It will also add a path
that is a symbolic link to a directory. The symbolic link will be dereferenced and the content of the directory it is pointing to added recursively. However, symbolic links under path
will be stored as is.
- Parameters
-
path full path to an existing local directory, to be added to the archive. destName the resulting name (or relative path) of the file in the archive.
Definition at line 348 of file karchive.cpp.
◆ addLocalFile()
Writes a local file into the archive.
The main difference with writeFile, is that this method minimizes memory usage, by not loading the whole file into memory in one go.
If fileName
is a symbolic link, it will be written as is, i.e. it will not be resolved before.
- Parameters
-
fileName full path to an existing local file, to be added to the archive. destName the resulting name (or relative path) of the file in the archive.
Definition at line 262 of file karchive.cpp.
◆ close()
|
virtual |
Closes the archive.
Inherited classes might want to reimplement closeArchive instead.
- Returns
- true if close succeeded without problems
- See also
- open
Definition at line 214 of file karchive.cpp.
◆ closeArchive()
|
protectedpure virtual |
◆ createDevice()
|
protectedvirtual |
◆ device()
QIODevice * KArchive::device | ( | ) | const |
◆ directory()
const KArchiveDirectory * KArchive::directory | ( | ) | const |
If an archive is opened for reading, then the contents of the archive can be accessed via this function.
- Returns
- the directory of the archive
Definition at line 256 of file karchive.cpp.
◆ doFinishWriting()
|
protectedpure virtual |
◆ doPrepareWriting()
|
protectedpure virtual |
This virtual method must be implemented by subclasses.
Depending on the archive type not all metadata might be used.
- Parameters
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file. Use 0100644 if you don't have any more specific permissions to set. atime time the file was last accessed mtime modification time of the file ctime time of last status change
- See also
- prepareWriting
◆ doWriteData()
|
protectedvirtual |
Write data into the current file.
Called by writeData.
- Parameters
-
data a pointer to the data size the size of the chunk
- Returns
true
if successful,false
otherwise
- See also
- writeData
- Since
- 6.0
Reimplemented in K7Zip, and KZip.
Definition at line 422 of file karchive.cpp.
◆ doWriteDir()
|
protectedpure virtual |
Write a directory to the archive.
This virtual method must be implemented by subclasses.
Depending on the archive type not all metadata might be used.
- Parameters
-
name the name of the directory user the user that owns the directory group the group that owns the directory perm permissions of the directory. Use 040755 if you don't have any other information. atime time the file was last accessed mtime modification time of the file ctime time of last status change
- See also
- writeDir
◆ doWriteSymLink()
|
protectedpure virtual |
Writes a symbolic link to the archive.
This virtual method must be implemented by subclasses.
- Parameters
-
name name of symbolic link target target of symbolic link user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime time of last status change
- See also
- writeSymLink
◆ errorString()
QString KArchive::errorString | ( | ) | const |
◆ fileName()
QString KArchive::fileName | ( | ) | const |
The name of the archive file, as passed to the constructor that takes a fileName, or an empty string if you used the QIODevice constructor.
- Returns
- the name of the file, or QString() if unknown
Definition at line 640 of file karchive.cpp.
◆ findOrCreate()
|
protected |
Ensures that path
exists, create otherwise.
This handles e.g. tar files missing directory entries, like mico-2.3.0.tar.gz :)
- Parameters
-
path the path of the directory
- Returns
- the directory with the given
path
Definition at line 529 of file karchive.cpp.
◆ finishWriting()
bool KArchive::finishWriting | ( | qint64 | size | ) |
Call finishWriting after writing the data.
- Parameters
-
size the size of the file
- See also
- prepareWriting()
Definition at line 477 of file karchive.cpp.
◆ isOpen()
bool KArchive::isOpen | ( | ) | const |
Checks whether the archive is open.
- Returns
- true if the archive is opened
Definition at line 635 of file karchive.cpp.
◆ mode()
QIODevice::OpenMode KArchive::mode | ( | ) | const |
Returns the mode in which the archive was opened.
- Returns
- the mode in which the archive was opened (QIODevice::ReadOnly or QIODevice::WriteOnly)
- See also
- open()
Definition at line 625 of file karchive.cpp.
◆ open()
|
virtual |
Opens the archive for reading or writing.
Inherited classes might want to reimplement openArchive instead.
- Parameters
-
mode may be QIODevice::ReadOnly or QIODevice::WriteOnly
- See also
- close
Definition at line 141 of file karchive.cpp.
◆ openArchive()
|
protectedpure virtual |
Opens an archive for reading or writing.
Called by open.
- Parameters
-
mode may be QIODevice::ReadOnly or QIODevice::WriteOnly
◆ prepareWriting()
bool KArchive::prepareWriting | ( | const QString & | name, |
const QString & | user, | ||
const QString & | group, | ||
qint64 | size, | ||
mode_t | perm = 0100644, | ||
const QDateTime & | atime = QDateTime(), | ||
const QDateTime & | mtime = QDateTime(), | ||
const QDateTime & | ctime = QDateTime() ) |
Here's another way of writing a file into an archive: Call prepareWriting(), then call writeData() as many times as wanted then call finishWriting( totalSize ).
For tar.gz files, you need to know the size before hand, it is needed in the header! For zip files, size isn't used.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file atime time the file was last accessed mtime modification time of the file ctime time of last status change
Definition at line 461 of file karchive.cpp.
◆ rootDir()
|
protectedvirtual |
Retrieves or create the root directory.
The default implementation assumes that openArchive() did the parsing, so it creates a dummy rootdir if none was set (write mode, or no '/' in the archive). Reimplement this to provide parsing/listing on demand.
- Returns
- the root directory
Definition at line 517 of file karchive.cpp.
◆ setDevice()
|
protected |
Can be called by derived classes in order to set the underlying device.
Note that KArchive will -not- own the device, it must be deleted by the derived class.
Definition at line 609 of file karchive.cpp.
◆ setErrorString()
|
protected |
Sets error description.
- Parameters
-
errorStr error description
- Since
- 5.29
Definition at line 482 of file karchive.cpp.
◆ setRootDir()
|
protected |
Derived classes call setRootDir from openArchive, to set the root directory after parsing an existing archive.
Definition at line 618 of file karchive.cpp.
◆ virtual_hook()
|
protectedvirtual |
Definition at line 1055 of file karchive.cpp.
◆ writeData() [1/2]
bool KArchive::writeData | ( | const char * | data, |
qint64 | size ) |
Write data into the current file - to be called after calling prepareWriting.
- Parameters
-
data a pointer to the data size the size of the chunk
- Returns
true
if successful,false
otherwise
Definition at line 412 of file karchive.cpp.
◆ writeData() [2/2]
bool KArchive::writeData | ( | QByteArrayView | data | ) |
Overload for writeData(const char *, qint64);.
- Since
- 6.0
Definition at line 417 of file karchive.cpp.
◆ writeDir()
bool KArchive::writeDir | ( | const QString & | name, |
const QString & | user = QString(), | ||
const QString & | group = QString(), | ||
mode_t | perm = 040755, | ||
const QDateTime & | atime = QDateTime(), | ||
const QDateTime & | mtime = QDateTime(), | ||
const QDateTime & | ctime = QDateTime() ) |
If an archive is opened for writing then you can add new directories using this function.
KArchive won't write one directory twice.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters
-
name the name of the directory user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime time of last status change
Definition at line 438 of file karchive.cpp.
◆ writeFile()
bool KArchive::writeFile | ( | const QString & | name, |
QByteArrayView | data, | ||
mode_t | perm = 0100644, | ||
const QString & | user = QString(), | ||
const QString & | group = QString(), | ||
const QDateTime & | atime = QDateTime(), | ||
const QDateTime & | mtime = QDateTime(), | ||
const QDateTime & | ctime = QDateTime() ) |
Writes a new file into the archive.
The archive must be opened for writing first.
The necessary parent directories are created automatically if needed. For instance, writing "mydir/test1" does not require creating the directory "mydir" first.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be written out.
- Parameters
-
name the name of the file data the data to write perm permissions of the file user the user that owns the file group the group that owns the file atime time the file was last accessed mtime modification time of the file ctime time of last status change
- Since
- 6.0
Definition at line 383 of file karchive.cpp.
◆ writeSymLink()
bool KArchive::writeSymLink | ( | const QString & | name, |
const QString & | target, | ||
const QString & | user = QString(), | ||
const QString & | group = QString(), | ||
mode_t | perm = 0120755, | ||
const QDateTime & | atime = QDateTime(), | ||
const QDateTime & | mtime = QDateTime(), | ||
const QDateTime & | ctime = QDateTime() ) |
Writes a symbolic link to the archive if supported.
The archive must be opened for writing.
- Parameters
-
name name of symbolic link target target of symbolic link user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime time of last status change
Definition at line 449 of file karchive.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.