KCompletion
#include <KCompletion>
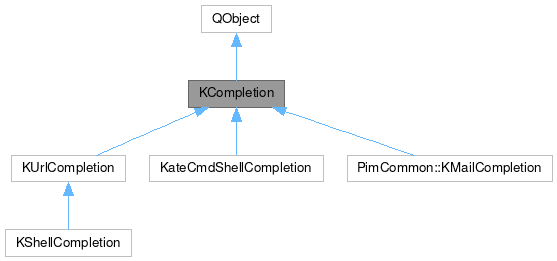
Public Types | |
enum | CompletionMode { CompletionNone = 1 , CompletionAuto , CompletionMan , CompletionShell , CompletionPopup , CompletionPopupAuto } |
enum | CompOrder { Sorted , Insertion , Weighted } |
using | SorterFunction = std::function<void(QStringList &)> |
Properties | |
bool | ignoreCase |
QStringList | items |
CompOrder | order |
![]() | |
objectName | |
Signals | |
void | match (const QString &item) |
void | matches (const QStringList &matchlist) |
void | multipleMatches () |
Public Slots | |
void | addItem (const QString &item) |
void | addItem (const QString &item, uint weight) |
virtual void | clear () |
void | insertItems (const QStringList &items) |
virtual QString | makeCompletion (const QString &string) |
QString | nextMatch () |
QString | previousMatch () |
void | removeItem (const QString &item) |
virtual void | setItems (const QStringList &itemList) |
Protected Member Functions | |
virtual void | postProcessMatch (QString *match) const |
virtual void | postProcessMatches (KCompletionMatches *matches) const |
virtual void | postProcessMatches (QStringList *matchList) const |
void | setShouldAutoSuggest (bool shouldAutosuggest) |
void | setSorterFunction (SorterFunction sortFunc) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A generic class for completing QStrings.
This class offers easy use of "auto completion", "manual completion" or "shell completion" on QString objects. A common use is completing filenames or URLs (see KUrlCompletion()). But it is not limited to URL-completion – everything should be completable! The user should be able to complete email addresses, telephone numbers, commands, SQL queries... Every time your program knows what the user can type into an edit field, you should offer completion. With KCompletion, this is very easy, and if you are using a line edit widget (KLineEdit), it is even easier. Basically, you tell a KCompletion object what strings should be completable and, whenever completion should be invoked, you call makeCompletion(). KLineEdit and (an editable) KComboBox even do this automatically for you.
KCompletion offers the completed string via the signal match() and all matching strings (when the result is ambiguous) via the method allMatches().
Notice: auto completion, shell completion and manual completion work slightly differently:
- auto completion always returns a complete item as match. When more than one matching item is available, it will deliver just the first one (depending on sorting order). Iterating over all matches is possible via nextMatch() and previousMatch().
- popup completion works in the same way, the only difference being that the completed items are not put into the edit widget, but into a separate popup box.
- manual completion works the same way as auto completion, except that it is not invoked automatically while the user is typing, but only when the user presses a special key. The difference of manual and auto completion is therefore only visible in UI classes. KCompletion needs to know whether to deliver partial matches (shell completion) or whole matches (auto/manual completion), therefore KCompletion::CompletionMan and KCompletion::CompletionAuto have the exact same effect in KCompletion.
- shell completion works like "tab completion" in a shell: when multiple matches are available, the longest possible string of all matches is returned (i.e. only a partial item). Iterating over all matching items (complete, not partial) is possible via nextMatch() and previousMatch().
As an application programmer, you do not normally have to worry about the different completion modes; KCompletion handles that for you, according to the setting setCompletionMode(). The default setting is globally configured by the user and read from completionMode().
A short example:
In shell-completion mode, this will be "carp"; in auto-completion mode it will be "carp\@cs.tu-berlin.de", as that is alphabetically smaller. If setOrder was set to Insertion, "carpdjih\@sp.zrz.tu-berlin.de" would be completed in auto-completion mode, as that was inserted before "carp\@cs.tu-berlin.de".
You can dynamically update the completable items by removing and adding them whenever you want. For advanced usage, you could even use multiple KCompletion objects. E.g. imagine an editor like kwrite with multiple open files. You could store items of each file in a different KCompletion object, so that you know (and tell the user) where a completion comes from.
- Note
- KCompletion does not work with strings that contain 0x0 characters (unicode null), as this is used internally as a delimiter.
You may inherit from KCompletion and override makeCompletion() in special cases (like reading directories or urls and then supplying the contents to KCompletion, as KUrlCompletion does), but this is usually not necessary.
Definition at line 117 of file kcompletion.h.
Member Typedef Documentation
◆ SorterFunction
using KCompletion::SorterFunction = std::function<void(QStringList &)> |
The sorter function signature.
Deriving classes may provide custom sorting logic via the setSorterFunction method.
- Since
- 5.88
Definition at line 176 of file kcompletion.h.
Member Enumeration Documentation
◆ CompletionMode
This enum describes the completion mode used for by the KCompletion class.
- Since
- 5.0
Definition at line 131 of file kcompletion.h.
◆ CompOrder
Constants that represent the order in which KCompletion performs completion lookups.
Enumerator | |
---|---|
Sorted | Use alphabetically sorted order or custom sorter logic. |
Insertion | Use order of insertion. |
Weighted | Use weighted order. |
Definition at line 163 of file kcompletion.h.
Property Documentation
◆ ignoreCase
|
readwrite |
Definition at line 120 of file kcompletion.h.
◆ items
|
readwrite |
Definition at line 121 of file kcompletion.h.
◆ order
|
readwrite |
Definition at line 119 of file kcompletion.h.
Constructor & Destructor Documentation
◆ KCompletion()
KCompletion::KCompletion | ( | ) |
Constructor, nothing special here :)
Definition at line 122 of file kcompletion.cpp.
◆ ~KCompletion()
|
override |
Destructor, nothing special here, either.
Definition at line 128 of file kcompletion.cpp.
Member Function Documentation
◆ addItem [1/2]
Adds an item to the list of available completions.
Resets the current item state (previousMatch() and nextMatch() won't work the next time they are called).
- Parameters
-
item the item to add
Definition at line 201 of file kcompletion.cpp.
◆ addItem [2/2]
Adds an item to the list of available completions.
Resets the current item state (previousMatch() and nextMatch() won't work the next time they are called).
Sets the weight of the item to weight
or adds it to the current weight if the item is already available. The weight has to be greater than 1 to take effect (default weight is 1).
- Parameters
-
item the item to add weight the weight of the item, default is 1
Definition at line 211 of file kcompletion.cpp.
◆ allMatches() [1/2]
QStringList KCompletion::allMatches | ( | ) |
Returns a list of all items matching the last completed string.
It might take some time if you have a lot of items.
- Returns
- a list of all matches for the last completed string.
- See also
- substringCompletion
Definition at line 374 of file kcompletion.cpp.
◆ allMatches() [2/2]
QStringList KCompletion::allMatches | ( | const QString & | string | ) |
Returns a list of all items matching string
.
- Parameters
-
string the string to match
- Returns
- the list of all matches
Definition at line 402 of file kcompletion.cpp.
◆ allWeightedMatches() [1/2]
KCompletionMatches KCompletion::allWeightedMatches | ( | ) |
Returns a list of all items matching the last completed string.
It might take some time if you have a lot of items. The matches are returned as KCompletionMatches, which also keeps the weight of the matches, allowing you to modify some matches or merge them with matches from another call to allWeightedMatches(), and sort the matches after that in order to have the matches ordered correctly.
- Returns
- a list of all completion matches
- See also
- substringCompletion
Definition at line 388 of file kcompletion.cpp.
◆ allWeightedMatches() [2/2]
KCompletionMatches KCompletion::allWeightedMatches | ( | const QString & | string | ) |
Returns a list of all items matching string
.
- Parameters
-
string the string to match
- Returns
- a list of all matches
Definition at line 413 of file kcompletion.cpp.
◆ clear
|
virtualslot |
Removes all inserted items.
Reimplemented in PimCommon::KMailCompletion.
Definition at line 252 of file kcompletion.cpp.
◆ completionMode()
KCompletion::CompletionMode KCompletion::completionMode | ( | ) | const |
Returns the current completion mode.
- Returns
- the current completion mode, default is CompletionPopup
- See also
- setCompletionMode
- CompletionMode
Definition at line 350 of file kcompletion.cpp.
◆ hasMultipleMatches()
bool KCompletion::hasMultipleMatches | ( | ) | const |
Returns true when more than one match is found.
- Returns
- true if there is more than one match
- See also
- multipleMatches
Definition at line 436 of file kcompletion.cpp.
◆ ignoreCase()
bool KCompletion::ignoreCase | ( | ) | const |
Returns whether KCompletion acts case insensitively or not.
Default is false (case sensitive).
- Returns
- true if the case will be ignored
- See also
- setIgnoreCase
Definition at line 151 of file kcompletion.cpp.
◆ insertItems
|
slot |
Inserts items
into the list of possible completions.
It does the same as setItems(), but without calling clear() before.
- Parameters
-
items the items to insert
Definition at line 163 of file kcompletion.cpp.
◆ isEmpty()
bool KCompletion::isEmpty | ( | ) | const |
Returns true if the completion object contains no entries.
Definition at line 183 of file kcompletion.cpp.
◆ items()
QStringList KCompletion::items | ( | ) | const |
Returns a list of all items inserted into KCompletion.
This is useful if you need to save the state of a KCompletion object and restore it later.
- Note
- When order() == Weighted, then every item in the stringlist has its weight appended, delimited by a colon. E.g. an item "www.kde.org" might look like "www.kde.org:4", where 4 is the weight. This is necessary so that you can save the items along with its weighting on disk and load them back with setItems(), restoring its weight as well. If you really don't want the appended weightings, call setOrder( KCompletion::Insertion ) before calling items().
- Returns
- a list of all items
- See also
- setItems
Definition at line 175 of file kcompletion.cpp.
◆ lastMatch()
Returns the last match.
Might be useful if you need to check whether a completion is different from the last one.
- Returns
- the last match. QString() is returned when there is no last match.
Definition at line 477 of file kcompletion.cpp.
◆ makeCompletion
Attempts to find an item in the list of available completions that begins with string
.
Will either return the first matching item (if there is more than one match) or QString(), if no match is found.
In the latter case, a sound will be emitted, depending on soundsEnabled(). If a match is found, it will be emitted via the signal match().
If this is called twice or more with the same string while no items were added or removed in the meantime, all available completions will be emitted via the signal matches(). This happens only in shell-completion mode.
- Parameters
-
string the string to complete
- Returns
- the matching item, or QString() if there is no matching item.
- See also
- substringCompletion
Reimplemented in PimCommon::KMailCompletion, KShellCompletion, KUrlCompletion, and KateCmdShellCompletion.
Definition at line 262 of file kcompletion.cpp.
◆ match
This signal is emitted when a match is found.
In particular, makeCompletion(), previousMatch() and nextMatch() all emit this signal; makeCompletion() will only emit it when a match is found, but the other methods will always emit it (and so may emit it with an empty string).
- Parameters
-
item the matching item, or QString() if there were no more matching items.
◆ matches
|
signal |
This signal is emitted by makeCompletion() in shell-completion mode when the same string is passed to makeCompletion() multiple times in a row.
- Parameters
-
matchlist the list of all matching items
◆ multipleMatches
|
signal |
This signal is emitted when calling makeCompletion() and more than one matching item is found.
- See also
- hasMultipleMatches
◆ nextMatch
|
slot |
Returns the next item from the list of matching items.
When reaching the last item, the list is rotated, so it will return the first match and a sound is emitted (depending on soundsEnabled()).
- Returns
- the next item from the list of matching items. When there is no match, QString() is returned and a sound is emitted.
Definition at line 445 of file kcompletion.cpp.
◆ order()
KCompletion::CompOrder KCompletion::order | ( | ) | const |
Returns the completion order.
- Returns
- the current completion order.
- See also
- setOrder
Definition at line 139 of file kcompletion.cpp.
◆ postProcessMatch()
This method is called after a completion is found and before the matching string is emitted.
You can override this method to modify the string that will be emitted. This is necessary e.g. in KUrlCompletion(), where files with spaces in their names are shown escaped ("filename\ with\ spaces"), but stored unescaped inside KCompletion. Never delete that pointer!
Default implementation does nothing.
- Parameters
-
match the match to process
- See also
- postProcessMatches
Definition at line 189 of file kcompletion.cpp.
◆ postProcessMatches() [1/2]
|
protectedvirtual |
This method is called before a list of all available completions is emitted via matches().
You can override this method to modify the found items before match() or matches() are emitted. Never delete that pointer!
Default implementation does nothing.
- Parameters
-
matches the matches to process
- See also
- postProcessMatch
Definition at line 197 of file kcompletion.cpp.
◆ postProcessMatches() [2/2]
|
protectedvirtual |
This method is called before a list of all available completions is emitted via matches().
You can override this method to modify the found items before match() or matches() are emitted. Never delete that pointer!
Default implementation does nothing.
- Parameters
-
matchList the matches to process
- See also
- postProcessMatch
Reimplemented in PimCommon::KMailCompletion.
Definition at line 193 of file kcompletion.cpp.
◆ previousMatch
|
slot |
Returns the next item from the list of matching items.
When reaching the beginning, the list is rotated so it will return the last match and a sound is emitted (depending on soundsEnabled()).
- Returns
- the next item from the list of matching items. When there is no match, QString() is returned and a sound is emitted.
Definition at line 483 of file kcompletion.cpp.
◆ removeItem
Removes an item from the list of available completions.
Resets the current item state (previousMatch() and nextMatch() won't work the next time they are called).
- Parameters
-
item the item to remove
Definition at line 242 of file kcompletion.cpp.
◆ setCompletionMode()
|
virtual |
Sets the completion mode.
- Parameters
-
mode the completion mode
- See also
- CompletionMode
Definition at line 344 of file kcompletion.cpp.
◆ setIgnoreCase()
|
virtual |
Setting this to true makes KCompletion behave case insensitively.
E.g. makeCompletion("CA"); might return "carp\@cs.tu-berlin.de". Default is false (case sensitive).
- Parameters
-
ignoreCase true to ignore the case
- See also
- ignoreCase
Definition at line 145 of file kcompletion.cpp.
◆ setItems
|
virtualslot |
Sets the list of items available for completion.
Removes all previous items.
- Note
- When order() == Weighted, then the weighting is looked up for every item in the stringlist. Every item should have ":number" appended, where number is an unsigned integer, specifying the weighting. If you don't like this, call setOrder(KCompletion::Insertion) before calling setItems().
- Parameters
-
itemList the list of items that are available for completion
- See also
- items
Definition at line 157 of file kcompletion.cpp.
◆ setOrder()
KCompletion offers three different ways in which it offers its items:
- in the order of insertion
- sorted alphabetically
- weighted
Choosing weighted makes KCompletion perform an implicit weighting based on how often an item is inserted. Imagine a web browser with a location bar, where the user enters URLs. The more often a URL is entered, the higher priority it gets.
- Note
- Setting the order to sorted only affects new inserted items, already existing items will stay in the current order. So you probably want to call setOrder(Sorted) before inserting items if you want everything sorted.
Default is insertion order.
- Parameters
-
order the new order
- See also
- order
Definition at line 132 of file kcompletion.cpp.
◆ setShouldAutoSuggest()
|
protected |
Deriving classes may set this property and control whether the auto-suggestion should be displayed for the last completion operation performed.
Applies for CompletionPopupAuto and CompletionAuto modes.
- Since
- 5.87
Definition at line 356 of file kcompletion.cpp.
◆ setSorterFunction()
|
protected |
Sets a custom function to be used to sort the matches.
Can be set to nullptr to use the default sorting logic.
Applies for CompOrder::Sorted mode.
- Since
- 5.88
Definition at line 368 of file kcompletion.cpp.
◆ setSoundsEnabled()
|
virtual |
Enables/disables emitting a sound when.
- makeCompletion() can't find a match
- there is a partial completion (= multiple matches in Shell-completion mode)
- nextMatch() or previousMatch() hit the last possible match and the list is rotated
KNotifyClient() is used to emit the sounds.
- Parameters
-
enable true to enable sounds
- See also
- soundsEnabled
Definition at line 424 of file kcompletion.cpp.
◆ shouldAutoSuggest()
bool KCompletion::shouldAutoSuggest | ( | ) | const |
Informs the caller if they should display the auto-suggestion for the last completion operation performed.
Applies for CompletionPopupAuto and CompletionAuto modes. Defaults to true, but deriving classes may set it to false in special cases via "setShouldAutoSuggest".
- Returns
- true if auto-suggestion should be displayed for the last completion operation performed.
- Since
- 5.87
Definition at line 362 of file kcompletion.cpp.
◆ soundsEnabled()
bool KCompletion::soundsEnabled | ( | ) | const |
Tells you whether KCompletion will emit sounds on certain occasions.
Default is enabled.
- Returns
- true if sounds are enabled
- See also
- setSoundsEnabled
Definition at line 430 of file kcompletion.cpp.
◆ substringCompletion()
QStringList KCompletion::substringCompletion | ( | const QString & | string | ) | const |
Returns a list of all completion items that contain the given string
.
- Parameters
-
string the string to complete
- Returns
- a list of items which contain
text
as a substring, i.e. not necessarily at the beginning.
- See also
- makeCompletion
Definition at line 319 of file kcompletion.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:16:24 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.