KConfig
#include <KConfig>
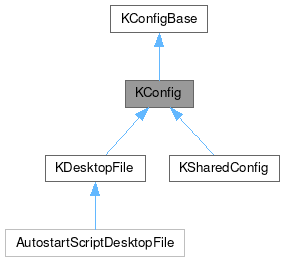
Public Types | |
enum | OpenFlag { IncludeGlobals = 0x01 , CascadeConfig = 0x02 , SimpleConfig = 0x00 , NoCascade = IncludeGlobals , NoGlobals = CascadeConfig , FullConfig = IncludeGlobals | CascadeConfig } |
typedef QFlags< OpenFlag > | OpenFlags |
![]() | |
enum | AccessMode { NoAccess , ReadOnly , ReadWrite } |
enum | WriteConfigFlag { Persistent = 0x01 , Global = 0x02 , Localized = 0x04 , Notify = 0x08 | Persistent , Normal = Persistent } |
typedef QFlags< WriteConfigFlag > | WriteConfigFlags |
Static Public Member Functions | |
static QString | mainConfigName () |
static void | setMainConfigName (const QString &str) |
Protected Member Functions | |
KCONFIGCORE_NO_EXPORT | KConfig (KConfigPrivate &d) |
void | deleteGroupImpl (const QString &groupName, WriteConfigFlags flags=Normal) override |
const KConfigGroup | groupImpl (const QString &groupName) const override |
KConfigGroup | groupImpl (const QString &groupName) override |
bool | hasGroupImpl (const QString &groupName) const override |
bool | isGroupImmutableImpl (const QString &groupName) const override |
void | virtual_hook (int id, void *data) override |
Protected Attributes | |
KConfigPrivate *const | d_ptr |
Detailed Description
The central class of the KDE configuration data system.
Quickstart:
Get the default application config object via KSharedConfig::openConfig().
Load a specific configuration file:
Load the configuration of a specific component:
In general it is recommended to use KSharedConfig instead of creating multiple instances of KConfig to avoid the overhead of separate objects or concerns about synchronizing writes to disk even if the configuration object is updated from multiple code paths. KSharedConfig provides a set of open methods as counterparts for the KConfig constructors.
Member Typedef Documentation
◆ OpenFlags
typedef QFlags< OpenFlag > KConfig::OpenFlags |
Member Enumeration Documentation
◆ OpenFlag
enum KConfig::OpenFlag |
Determines how the system-wide and user's global settings will affect the reading of the configuration.
If CascadeConfig is selected, system-wide configuration sources are used to provide defaults for the settings accessed through this object, or possibly to override those settings in certain cases.
If IncludeGlobals is selected, the kdeglobals configuration is used as additional configuration sources to provide defaults. Additionally selecting CascadeConfig will result in the system-wide kdeglobals sources also getting included.
Note that the main configuration source overrides the cascaded sources, which override those provided to addConfigSources(), which override the global sources. The exception is that if a key or group is marked as being immutable, it will not be overridden.
Note that all values other than IncludeGlobals and CascadeConfig are convenience definitions for the basic mode. Do not combine them with anything.
- See also
- OpenFlags
Constructor & Destructor Documentation
◆ KConfig() [1/3]
|
explicit |
Creates a KConfig object to manipulate a configuration file for the current application.
If an absolute path is specified for file
, that file will be used as the store for the configuration settings. If a non-absolute path is provided, the file will be looked for in the standard directory specified by type. If no path is provided, a default configuration file will be used based on the name of the main application component.
mode
determines whether the user or global settings will be allowed to influence the values returned by this object. See OpenFlags for more details.
- Note
- You probably want to use KSharedConfig::openConfig instead.
- Parameters
-
file the name of the file. If an empty string is passed in and SimpleConfig is passed in for the OpenFlags, then an in-memory KConfig object is created which will not write out to file nor which requires any file in the filesystem at all. mode how global settings should affect the configuration options exposed by this KConfig object type The standard directory to look for the configuration file in
Definition at line 241 of file kconfig.cpp.
◆ KConfig() [2/3]
KConfig::KConfig | ( | const QString & | file, |
const QString & | backend, | ||
QStandardPaths::StandardLocation | type = QStandardPaths::GenericConfigLocation ) |
Creates a KConfig object using the specified backend. If the backend can not be found or loaded, then the standard configuration parser is used as a fallback.
- Parameters
-
file the file to be parsed backend the backend to load type where to look for the file if an absolute path is not provided
- Since
- 4.1
- Deprecated
- since 6.3, use other constructor
Definition at line 251 of file kconfig.cpp.
◆ ~KConfig()
|
override |
Definition at line 267 of file kconfig.cpp.
◆ KConfig() [3/3]
|
explicitprotected |
Definition at line 262 of file kconfig.cpp.
Member Function Documentation
◆ accessMode()
|
overridevirtual |
configuration object state
- Reimplemented from superclass.
Implements KConfigBase.
Definition at line 830 of file kconfig.cpp.
◆ addConfigSources()
void KConfig::addConfigSources | ( | const QStringList & | sources | ) |
extra config files
Adds the list of configuration sources to the merge stack.
Currently only files are accepted as configuration sources.
The first entry in sources
is treated as the most general and will be overridden by the second entry. The settings in the final entry in sources
will override all the other sources provided in the list.
The settings in sources
will also be overridden by the sources provided by any previous calls to addConfigSources().
The settings in the global configuration sources will be overridden by the sources provided to this method (
- See also
- IncludeGlobals). All the sources provided to any call to this method will be overridden by any files that cascade from the source provided to the constructor (
- CascadeConfig), which will in turn be overridden by the source provided to the constructor.
Note that only the most specific file, ie: the file provided to the constructor, will be written to by this object.
The state is automatically updated by this method, so there is no need to call reparseConfiguration().
- Parameters
-
sources A list of extra config sources.
Definition at line 836 of file kconfig.cpp.
◆ additionalConfigSources()
QStringList KConfig::additionalConfigSources | ( | ) | const |
Returns a list of the additional configuration sources used in this object.
Definition at line 848 of file kconfig.cpp.
◆ checkUpdate()
Ensures that the configuration file contains a certain update.
If the configuration file does not contain the update id
as contained in updateFile
, kconf_update is run to update the configuration file.
If you install config update files with critical fixes you may wish to use this method to verify that a critical update has indeed been performed to catch the case where a user restores an old config file from backup that has not been updated yet.
- Parameters
-
id the update to check updateFile the file containing the update
Definition at line 533 of file kconfig.cpp.
◆ copyTo()
Copies all entries from this config object to a new config object that will save itself to file
.
The configuration will not actually be saved to file
until the returned object is destroyed, or sync() is called on it.
Do not forget to delete the returned KConfig object if config
was 0.
- Parameters
-
file the new config object will save itself to config if not 0, copy to the given KConfig object rather than creating a new one
- Returns
config
if it was set, otherwise a new KConfig object
Definition at line 544 of file kconfig.cpp.
◆ deleteGroupImpl()
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 932 of file kconfig.cpp.
◆ entryMap()
Returns a map (tree) of entries in a particular group.
The entries are all returned as strings.
- Parameters
-
aGroup The group to get entries from.
- Returns
- A map of entries in the group specified, indexed by key. The returned map may be empty if the group is empty, or not found.
- See also
- QMap
Definition at line 384 of file kconfig.cpp.
◆ groupImpl() [1/2]
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 908 of file kconfig.cpp.
◆ groupImpl() [2/2]
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 903 of file kconfig.cpp.
◆ groupList()
|
overridevirtual |
◆ hasGroupImpl()
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 971 of file kconfig.cpp.
◆ isConfigWritable()
bool KConfig::isConfigWritable | ( | bool | warnUser | ) |
Whether the configuration can be written to.
If warnUser
is true and the configuration cannot be written to (ie: this method returns false
), a warning message box will be shown to the user telling them to contact their system administrator to get the problem fixed.
The most likely cause for this method returning false
is that the user does not have write permission for the configuration file.
- Parameters
-
warnUser whether to show a warning message to the user if the configuration cannot be written to
- Returns
- true if the configuration can be written to, false if the configuration cannot be written to
Definition at line 949 of file kconfig.cpp.
◆ isDirty()
bool KConfig::isDirty | ( | ) | const |
Returns true if sync has any changes to write out.
- Since
- 4.12
Definition at line 527 of file kconfig.cpp.
◆ isGroupImmutableImpl()
|
overrideprotectedvirtual |
- Parameters
-
groupName name of group
Implements KConfigBase.
Definition at line 897 of file kconfig.cpp.
◆ isImmutable()
|
overridevirtual |
immutability
- Reimplemented from superclass.
Implements KConfigBase.
Definition at line 891 of file kconfig.cpp.
◆ locale()
QString KConfig::locale | ( | ) | const |
◆ locationType()
QStandardPaths::StandardLocation KConfig::locationType | ( | ) | const |
Returns the standard location enum passed to the constructor.
Used by KSharedConfig.
- Since
- 5.0
Definition at line 1045 of file kconfig.cpp.
◆ mainConfigName()
|
static |
◆ markAsClean()
|
overridevirtual |
◆ name()
QString KConfig::name | ( | ) | const |
Returns the filename used to store the configuration.
Definition at line 562 of file kconfig.cpp.
◆ openFlags()
KConfig::OpenFlags KConfig::openFlags | ( | ) | const |
◆ readDefaults()
bool KConfig::readDefaults | ( | ) | const |
- Returns
true
if the system-wide defaults will be read instead of the user's settings
Definition at line 885 of file kconfig.cpp.
◆ reparseConfiguration()
void KConfig::reparseConfiguration | ( | ) |
Updates the state of this object to match the persistent storage.
Note that if this object has pending changes, this method will call sync() first so as not to lose those changes.
Definition at line 649 of file kconfig.cpp.
◆ setLocale()
bool KConfig::setLocale | ( | const QString & | aLocale | ) |
Sets the locale to aLocale
.
The global locale is used by default.
- Note
- If set to the empty string, no locale will be matched. This effectively disables reading translated entries.
- Returns
true
if locale was changed,false
if the call had no effect (eg:aLocale
was already the current locale for this object)
Definition at line 869 of file kconfig.cpp.
◆ setMainConfigName()
|
static |
◆ setReadDefaults()
void KConfig::setReadDefaults | ( | bool | b | ) |
defaults
When set, all readEntry calls return the system-wide (default) values instead of the user's settings.
This is off by default.
- Parameters
-
b whether to read the system-wide defaults instead of the user's settings
Definition at line 879 of file kconfig.cpp.
◆ sync()
|
overridevirtual |
◆ virtual_hook()
|
overrideprotectedvirtual |
Virtual hook, used to add new "virtual" functions while maintaining binary compatibility.
Unused in this class.
Reimplemented from KConfigBase.
Definition at line 1051 of file kconfig.cpp.
Member Data Documentation
◆ d_ptr
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:04:35 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.