KConfigDialogManager
#include <KConfigDialogManager>
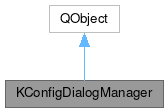
Signals | |
void | settingsChanged () |
void | widgetModified () |
Public Slots | |
void | setDefaultsIndicatorsVisible (bool enabled) |
void | updateSettings () |
void | updateWidgets () |
void | updateWidgetsDefault () |
Protected Member Functions | |
QByteArray | getCustomProperty (const QWidget *widget) const |
QByteArray | getCustomPropertyChangedSignal (const QWidget *widget) const |
QByteArray | getUserProperty (const QWidget *widget) const |
QByteArray | getUserPropertyChangedSignal (const QWidget *widget) const |
void | init (bool trackChanges) |
bool | parseChildren (const QWidget *widget, bool trackChanges) |
QVariant | property (QWidget *w) const |
void | setProperty (QWidget *w, const QVariant &v) |
void | setupWidget (QWidget *widget, KConfigSkeletonItem *item) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Static Protected Member Functions | |
static void | initMaps () |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
Provides a means of automatically retrieving, saving and resetting KConfigSkeleton based settings in a dialog.
The KConfigDialogManager class provides a means of automatically retrieving, saving and resetting basic settings. It also can emit signals when settings have been changed (settings were saved) or modified (the user changes a checkbox from on to off).
The object names of the widgets to be managed have to correspond to the names of the configuration entries in the KConfigSkeleton object plus an additional "kcfg_" prefix. For example a widget with the object name "kcfg_MyOption" would be associated to the configuration entry "MyOption".
The widget classes of Qt and KDE Frameworks are supported out of the box, for other widgets see below:
- Using Custom Widgets
Custom widget classes are supported if they have a Q_PROPERTY defined for the property representing the value edited by the widget. By default the property is used for which "USER true" is set. For using another property, see below.
Example:
A class ColorEditWidget is used in the settings UI to select a color. The color value is set and read as type QColor. For that it has a definition of the value property similar to this:
Q_PROPERTY(...)T qobject_cast(QObject *object)And of course it has the definition and implementation of the respective read & write methods and the notify signal. This class then can be used directly with KConfigDialogManager and does not need further setup.
- Using Other Properties than The USER Property
- To use a widget's property that is not the USER property, the property to use can be selected by setting onto the widget instance a property with the key "kcfg_property" and as the value the name of the property:
- Configuring Classes to use Other Properties Globally
Alternatively a non-USER property can be defined for a widget class globally by registering it for the class in the KConfigDialogManager::propertyMap(). This global registration has lower priority than any "kcfg_property" property set on a class instance though, so the latter overrules this global setting. Note: setting the property in the propertyMap affects any instances of that widget class in the current application, so use only when needed and prefer instead the "kcfg_property" property. Especially with software with many libraries and 3rd-party plugins in one process there is a chance of conflicting settings.
Example:
If the ColorEditWidget has another property redColor defined by
and this one should be used in the settings, call somewhere in the code before using the settings:
static QHash< QString, QByteArray > * propertyMap()Retrieve the map between widgets class names and the USER properties used for the configuration value...Definition kconfigdialogmanager.cpp:94
- Using Different Signals than The NOTIFY Signal
If some non-default signal should be used, e.g. because the property to use does not have a NOTIFY setting, for a given widget instance the signal to use can be set by a property with the key "kcfg_propertyNotify" and as the value the signal signature. This will take priority over the signal noted by NOTIFY for the chosen property as well as the content of KConfigDialogManager::changedMap(). Since 5.32.
Example:
If for a class OtherColorEditWidget there was no NOTIFY set on the USER property, but some signal colorSelected(QColor) defined which would be good enough to reflect the settings change, defined by
Q_SIGNALSQ_SIGNALSthe signal to use would be defined by this:
Definition at line 131 of file kconfigdialogmanager.h.
Constructor & Destructor Documentation
◆ KConfigDialogManager()
KConfigDialogManager::KConfigDialogManager | ( | QWidget * | parent, |
KCoreConfigSkeleton * | conf ) |
Constructor.
- Parameters
-
parent Dialog widget to manage conf Object that contains settings
Definition at line 31 of file kconfigdialogmanager.cpp.
◆ ~KConfigDialogManager()
|
overridedefault |
Destructor.
Member Function Documentation
◆ addWidget()
Add additional widgets to manage.
- Parameters
-
widget Additional widget to manage, including all its children
Definition at line 109 of file kconfigdialogmanager.cpp.
◆ getCustomProperty()
|
protected |
Find the property to use for a widget by querying the "kcfg_property" property of the widget.
Like a widget can use a property other than the USER property.
- Since
- 4.3
Definition at line 418 of file kconfigdialogmanager.cpp.
◆ getCustomPropertyChangedSignal()
|
protected |
Find the changed signal of the property to use for a widget by querying the "kcfg_propertyNotify" property of the widget.
Like a widget can use a property change signal other than the one for USER property, if there even is one.
- Since
- 5.32
Definition at line 450 of file kconfigdialogmanager.cpp.
◆ getUserProperty()
|
protected |
Finds the USER property name using Qt's MetaProperty system, and caches it in the property map (the cache could be retrieved by propertyMap() ).
Definition at line 385 of file kconfigdialogmanager.cpp.
◆ getUserPropertyChangedSignal()
|
protected |
Finds the changed signal of the USER property using Qt's MetaProperty system.
- Since
- 5.32
Definition at line 431 of file kconfigdialogmanager.cpp.
◆ hasChanged()
bool KConfigDialogManager::hasChanged | ( | ) | const |
Returns whether the current state of the known widgets are different from the state in the config object.
Definition at line 535 of file kconfigdialogmanager.cpp.
◆ init()
|
protected |
- Parameters
-
trackChanges - If any changes by the widgets should be tracked set true. This causes the emitting the modified() signal when something changes. TODO:
- Returns
- bool - True if any setting was changed from the default.
Definition at line 100 of file kconfigdialogmanager.cpp.
◆ initMaps()
|
staticprotected |
Initializes the property maps.
Definition at line 43 of file kconfigdialogmanager.cpp.
◆ isDefault()
bool KConfigDialogManager::isDefault | ( | ) | const |
Returns whether the current state of the known widgets are the same as the default state in the config object.
Definition at line 557 of file kconfigdialogmanager.cpp.
◆ parseChildren()
Recursive function that finds all known children.
Goes through the children of widget and if any are known and not being ignored, stores them in currentGroup. Also checks if the widget should be disabled because it is set immutable.
- Parameters
-
widget - Parent of the children to look at. trackChanges - If true then tracks any changes to the children of widget that are known.
- Returns
- bool - If a widget was set to something other than its default.
Definition at line 179 of file kconfigdialogmanager.cpp.
◆ property()
Retrieve a property.
Definition at line 501 of file kconfigdialogmanager.cpp.
◆ propertyMap()
|
static |
Retrieve the map between widgets class names and the USER properties used for the configuration values.
Definition at line 94 of file kconfigdialogmanager.cpp.
◆ setDefaultsIndicatorsVisible
|
slot |
Show or hide an indicator when settings have changed from their default value.
Update all widgets to show or hide the indicator accordingly.
- Since
- 5.73
Definition at line 351 of file kconfigdialogmanager.cpp.
◆ setProperty()
Set a property.
Definition at line 463 of file kconfigdialogmanager.cpp.
◆ settingsChanged
|
signal |
One or more of the settings have been saved (such as when the user clicks on the Apply button).
This is only emitted by updateSettings() whenever one or more setting were changed and consequently saved.
◆ setupWidget()
|
protected |
Setup secondary widget properties.
Definition at line 114 of file kconfigdialogmanager.cpp.
◆ updateSettings
|
slot |
Traverse the specified widgets, saving the settings of all known widgets in the settings object.
Example use: User clicks Ok or Apply button in a configure dialog.
Definition at line 356 of file kconfigdialogmanager.cpp.
◆ updateWidgets
|
slot |
Traverse the specified widgets, sets the state of all known widgets according to the state in the settings object.
Example use: Initialisation of dialog. Example use: User clicks Reset button in a configure dialog.
Definition at line 304 of file kconfigdialogmanager.cpp.
◆ updateWidgetsDefault
|
slot |
Traverse the specified widgets, sets the state of all known widgets according to the default state in the settings object.
Example use: User clicks Defaults button in a configure dialog.
Definition at line 343 of file kconfigdialogmanager.cpp.
◆ widgetModified
|
signal |
If retrieveSettings() was told to track changes then if any known setting was changed this signal will be emitted.
Note that a settings can be modified several times and might go back to the original saved state. hasChanged() will tell you if anything has actually changed from the saved values.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:13:01 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.