KDirWatch
#include <KDirWatch>
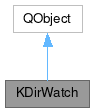
Public Types | |
enum | Method { INotify , Stat , QFSWatch } |
enum | WatchMode { WatchDirOnly = 0 , WatchFiles = 0x01 , WatchSubDirs = 0x02 } |
typedef QFlags< WatchMode > | WatchModes |
Signals | |
void | created (const QString &path) |
void | deleted (const QString &path) |
void | dirty (const QString &path) |
Public Slots | |
void | setCreated (const QString &path) |
void | setDeleted (const QString &path) |
void | setDirty (const QString &path) |
Public Member Functions | |
KDirWatch (QObject *parent=nullptr) | |
~KDirWatch () override | |
void | addDir (const QString &path, WatchModes watchModes=WatchDirOnly) |
void | addFile (const QString &file) |
bool | contains (const QString &path) const |
QDateTime | ctime (const QString &path) const |
bool | event (QEvent *event) override |
Method | internalMethod () const |
bool | isStopped () |
void | removeDir (const QString &path) |
void | removeFile (const QString &file) |
bool | restartDirScan (const QString &path) |
void | startScan (bool notify=false, bool skippedToo=false) |
bool | stopDirScan (const QString &path) |
void | stopScan () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static bool | exists () |
static KDirWatch * | self () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Class for watching directory and file changes.
Watch directories and files for changes. The watched directories or files don't have to exist yet.
When a watched directory is changed, i.e. when files therein are created or deleted, KDirWatch will emit the signal dirty().
When a watched, but previously not existing directory gets created, KDirWatch will emit the signal created().
When a watched directory gets deleted, KDirWatch will emit the signal deleted(). The directory is still watched for new creation.
When a watched file is changed, i.e. attributes changed or written to, KDirWatch will emit the signal dirty().
Scanning of particular directories or files can be stopped temporarily and restarted. The whole class can be stopped and restarted. Directories and files can be added/removed from the list in any state.
The implementation uses the INOTIFY functionality on LINUX. As a last resort, a regular polling for change of modification times is done; the polling interval is a global config option: DirWatch/PollInterval and DirWatch/NFSPollInterval for NFS mounted directories. The choice of implementation can be adjusted by the user, with the key [DirWatch] PreferredMethod={Stat|QFSWatch|inotify}
- See also
- self()
Definition at line 55 of file kdirwatch.h.
Member Typedef Documentation
◆ WatchModes
typedef QFlags< WatchMode > KDirWatch::WatchModes |
Stores a combination of WatchMode values.
Definition at line 72 of file kdirwatch.h.
Member Enumeration Documentation
◆ Method
enum KDirWatch::Method |
Definition at line 209 of file kdirwatch.h.
◆ WatchMode
enum KDirWatch::WatchMode |
Available watch modes for directory monitoring.
- See also
- WatchModes
Enumerator | |
---|---|
WatchDirOnly | Watch just the specified directory. |
WatchFiles | Watch also all files contained by the directory. |
WatchSubDirs | Watch also all the subdirs contained by the directory. |
Definition at line 64 of file kdirwatch.h.
Constructor & Destructor Documentation
◆ KDirWatch()
|
explicit |
Constructor.
Scanning begins immediately when a dir/file watch is added.
- Parameters
-
parent the parent of the QObject (or nullptr
for parent-less KDataTools)
Definition at line 1656 of file kdirwatch.cpp.
◆ ~KDirWatch()
|
override |
Member Function Documentation
◆ addDir()
void KDirWatch::addDir | ( | const QString & | path, |
WatchModes | watchModes = WatchDirOnly ) |
Adds a directory to be watched.
The directory does not have to exist. When watchModes
is set to WatchDirOnly (the default), the signals dirty(), created(), deleted() can be emitted, all for the watched directory. When watchModes
is set to WatchFiles, all files in the watched directory are watched for changes, too. Thus, the signals dirty(), created(), deleted() can be emitted. When watchModes
is set to WatchSubDirs, all subdirs are watched using the same flags specified in watchModes
(symlinks aren't followed). If the path
points to a symlink to a directory, the target directory is watched instead. If you want to watch the link, use addFile()
.
- Parameters
-
path the path to watch watchModes watch modes
- See also
- KDirWatch::WatchMode
Definition at line 1674 of file kdirwatch.cpp.
◆ addFile()
void KDirWatch::addFile | ( | const QString & | file | ) |
Adds a file to be watched.
If it's a symlink to a directory, it watches the symlink itself.
- Parameters
-
file the file to watch
Definition at line 1685 of file kdirwatch.cpp.
◆ contains()
bool KDirWatch::contains | ( | const QString & | path | ) | const |
Check if a directory is being watched by this KDirWatch instance.
- Parameters
-
path the directory to check
- Returns
- true if the directory is being watched
Definition at line 1768 of file kdirwatch.cpp.
◆ created
|
signal |
Emitted when a file or directory (being watched explicitly) is created.
This is not emitted when creating a file is created in a watched directory.
- Parameters
-
path the path of the file or directory
◆ ctime()
Returns the time the directory/file was last changed.
- Parameters
-
path the file to check
- Returns
- the date of the last modification
Definition at line 1698 of file kdirwatch.cpp.
◆ deleted
|
signal |
Emitted when a file or directory is deleted.
The object is still watched for new creation.
- Parameters
-
path the path of the file or directory
◆ dirty
|
signal |
Emitted when a watched object is changed.
For a directory this signal is emitted when files therein are created or deleted. For a file this signal is emitted when its size or attributes change.
When you watch a directory, changes in the size or attributes of contained files may or may not trigger this signal to be emitted depending on which backend is used by KDirWatch.
The new ctime is set before the signal is emitted.
- Parameters
-
path the path of the file or directory
◆ event()
|
overridevirtual |
Trivial override.
See QObject::event.
Reimplemented from QObject.
Definition at line 1834 of file kdirwatch.cpp.
◆ exists()
|
static |
Returns true if there is an instance of KDirWatch.
- Returns
- true if there is an instance of KDirWatch.
- See also
- KDirWatch::self()
Definition at line 1651 of file kdirwatch.cpp.
◆ internalMethod()
KDirWatch::Method KDirWatch::internalMethod | ( | ) | const |
Returns the preferred internal method to watch for changes.
Definition at line 1801 of file kdirwatch.cpp.
◆ isStopped()
bool KDirWatch::isStopped | ( | ) |
Is scanning stopped?
After creation of a KDirWatch instance, this is false.
- Returns
- true when scanning stopped
Definition at line 1755 of file kdirwatch.cpp.
◆ removeDir()
void KDirWatch::removeDir | ( | const QString & | path | ) |
Removes a directory from the list of scanned directories.
If specified path is not in the list this does nothing.
- Parameters
-
path the path of the dir to be removed from the list
Definition at line 1709 of file kdirwatch.cpp.
◆ removeFile()
void KDirWatch::removeFile | ( | const QString & | file | ) |
Removes a file from the list of watched files.
If specified path is not in the list this does nothing.
- Parameters
-
file the file to be removed from the list
Definition at line 1716 of file kdirwatch.cpp.
◆ restartDirScan()
bool KDirWatch::restartDirScan | ( | const QString & | path | ) |
Restarts scanning for specified path.
It doesn't notify about the changes (by emitting a signal). The ctime value is reset.
Call it when you are finished with big operations on that path, and when you have refreshed that path.
- Parameters
-
path the path to restart scanning
- Returns
- true if the
path
is being watched, otherwise false
- See also
- stopDirScan()
Definition at line 1734 of file kdirwatch.cpp.
◆ self()
|
static |
The KDirWatch instance usually globally used in an application.
It is automatically deleted when the application exits.
However, you can create an arbitrary number of KDirWatch instances aside from this one - for those you have to take care of memory management.
This function returns an instance of KDirWatch. If there is none, it will be created.
- Returns
- a KDirWatch instance
Definition at line 1644 of file kdirwatch.cpp.
◆ setCreated
|
slot |
Emits created().
- Parameters
-
path the path of the file or directory
Definition at line 1784 of file kdirwatch.cpp.
◆ setDeleted
|
slot |
Emits deleted().
- Parameters
-
path the path of the file or directory
Definition at line 1795 of file kdirwatch.cpp.
◆ setDirty
|
slot |
Emits dirty().
- Parameters
-
path the path of the file or directory
Definition at line 1790 of file kdirwatch.cpp.
◆ startScan()
void KDirWatch::startScan | ( | bool | notify = false, |
bool | skippedToo = false ) |
Starts scanning of all dirs in list.
- Parameters
-
notify If true, all changed directories (since stopScan() call) will be notified for refresh. If notify is false, all ctimes will be reset (except those who are stopped, but only if skippedToo
is false) and changed dirs won't be notified. You can start scanning even if the list is empty. First call should be called withfalse
or else all directories in list will be notified.skippedToo if true, the skipped directories (scanning of which was stopped with stopDirScan() ) will be reset and notified for change. Otherwise, stopped directories will continue to be unnotified.
Definition at line 1760 of file kdirwatch.cpp.
◆ stopDirScan()
bool KDirWatch::stopDirScan | ( | const QString & | path | ) |
Stops scanning the specified path.
The path
is not deleted from the internal list, it is just skipped. Call this function when you perform an huge operation on this directory (copy/move big files or many files). When finished, call restartDirScan(path).
- Parameters
-
path the path to skip
- Returns
- true if the
path
is being watched, otherwise false
- See also
- restartDirScan()
Definition at line 1723 of file kdirwatch.cpp.
◆ stopScan()
void KDirWatch::stopScan | ( | ) |
Stops scanning of all directories in internal list.
The timer is stopped, but the list is not cleared.
Definition at line 1747 of file kdirwatch.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:00 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.