KJob
#include <KJob>
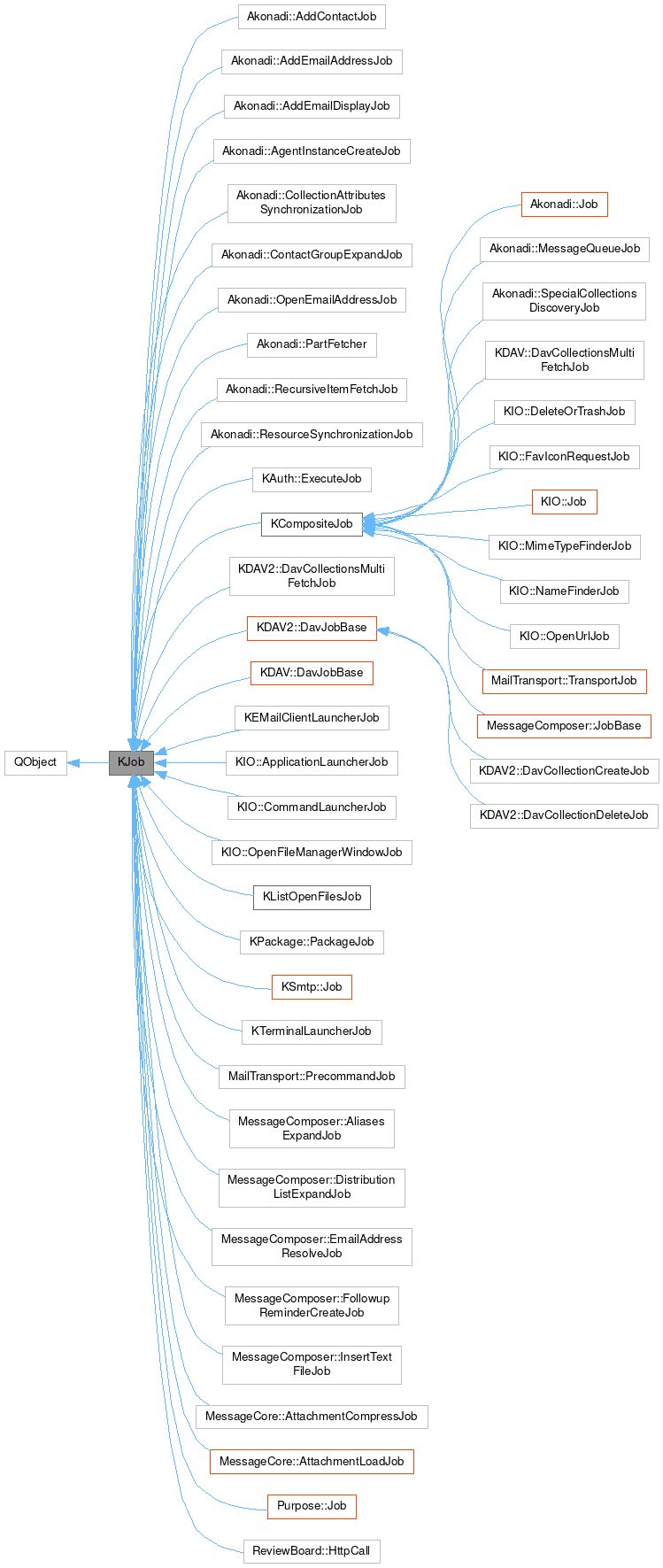
Public Types | |
enum | { NoError = 0 , KilledJobError = 1 , UserDefinedError = 100 } |
typedef QFlags< Capability > | Capabilities |
enum | Capability { NoCapabilities = 0x0000 , Killable = 0x0001 , Suspendable = 0x0002 } |
enum | KillVerbosity { Quietly , EmitResult } |
enum | Unit { Bytes = 0 , Files , Directories , Items , UnitsCount } |
Properties | |
Capabilities | capabilities |
int | error |
QString | errorString |
QString | errorText |
ulong | percent |
![]() | |
objectName | |
Signals | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=QPair< QString, QString >(), const QPair< QString, QString > &field2=QPair< QString, QString >()) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &message) |
void | percentChanged (KJob *job, unsigned long percent) |
void | processedAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmountChanged (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &message) |
Public Slots | |
bool | kill (KJob::KillVerbosity verbosity=KJob::Quietly) |
bool | resume () |
bool | suspend () |
Public Member Functions | |
KJob (QObject *parent=nullptr) | |
~KJob () override | |
Capabilities | capabilities () const |
qint64 | elapsedTime () const |
int | error () const |
virtual QString | errorString () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isFinishedNotificationHidden () const |
bool | isStartedWithExec () const |
bool | isSuspended () const |
unsigned long | percent () const |
Q_SCRIPTABLE qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setFinishedNotificationHidden (bool hide=true) |
void | setUiDelegate (KJobUiDelegate *delegate) |
virtual Q_SCRIPTABLE void | start ()=0 |
Q_SCRIPTABLE qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
KCOREADDONS_NO_EXPORT | KJob (KJobPrivate &dd, QObject *parent) |
virtual bool | doKill () |
virtual bool | doResume () |
virtual bool | doSuspend () |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | isFinished () const |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setProgressUnit (Unit unit) |
void | setTotalAmount (Unit unit, qulonglong amount) |
void | startElapsedTimer () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
std::unique_ptr< KJobPrivate > const | d_ptr |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The base class for all jobs.
For all jobs created in an application, the code looks like
(other connects, specific to the job)
And handleResult is usually at least:
With the synchronous interface the code looks like
Subclasses must implement start(), which should trigger the execution of the job (although the work should be done asynchronously). errorString() should also be reimplemented by any subclasses that introduce new error codes.
- Note
- KJob and its subclasses are meant to be used in a fire-and-forget way. Jobs will delete themselves when they finish using deleteLater() (although this behaviour can be changed), so a job instance will disappear after the next event loop run.
Member Typedef Documentation
◆ Capabilities
typedef QFlags< Capability > KJob::Capabilities |
Stores a combination of Capability values.
Member Enumeration Documentation
◆ anonymous enum
◆ Capability
enum KJob::Capability |
- See also
- Capabilities
Enumerator | |
---|---|
NoCapabilities | None of the capabilities exist. |
Killable | The job can be killed. |
Suspendable | The job can be suspended. |
◆ KillVerbosity
◆ Unit
enum KJob::Unit |
Describes the unit used in the methods that handle reporting the job progress info.
- See also
- totalAmount
Property Documentation
◆ capabilities
|
read |
◆ error
◆ errorString
◆ errorText
◆ percent
Constructor & Destructor Documentation
◆ KJob() [1/2]
|
explicit |
◆ ~KJob()
◆ KJob() [2/2]
Member Function Documentation
◆ capabilities()
KJob::Capabilities KJob::capabilities | ( | ) | const |
Returns the capabilities of this job.
- Returns
- the capabilities that this job supports
- See also
- setCapabilities()
◆ description
|
signal |
Emitted to display general description of this job.
A description has a title and two optional fields which can be used to complete the description.
Examples of titles are "Copying", "Creating resource", etc. The fields of the description can be "Source" with an URL, and, "Destination" with an URL for a "Copying" description.
- Parameters
-
job the job that emitted this signal title the general description of the job field1 first field (localized name and value) field2 second field (localized name and value)
◆ doKill()
|
protectedvirtual |
Aborts this job quietly.
This simply kills the job, no error reporting or job deletion should be involved.
- Returns
- true if the operation is supported and succeeded, false otherwise
Reimplemented in Akonadi::Job, KIO::Job, KIO::SimpleJob, and MailTransport::PrecommandJob.
◆ doResume()
|
protectedvirtual |
Resumes this job.
- Returns
- true if the operation is supported and succeeded, false otherwise
Reimplemented in KIO::CopyJob, KIO::Job, KIO::SimpleJob, and KIO::TransferJob.
◆ doSuspend()
|
protectedvirtual |
Suspends this job.
- Returns
- true if the operation is supported and succeeded, false otherwise
Reimplemented in KIO::CopyJob, KIO::Job, and KIO::SimpleJob.
◆ elapsedTime()
qint64 KJob::elapsedTime | ( | ) | const |
The number of milliseconds the job has been running for.
Starting from the last start()
call.
Sub-classes must call startElapsedTimer() from their start()
implementation, to get elapsedTime()
measurement. Otherwise this method will always return 0.
The time when paused is excluded.
- Since
- 6.8
◆ emitPercent()
|
protected |
◆ emitResult()
|
protected |
Utility function to emit the result signal, and end this job.
It first notifies the observers to hide the progress for this job using the finished() signal.
- Note
- Deletes this job using deleteLater().
- See also
- result()
- finished()
◆ emitSpeed()
|
protected |
◆ error()
int KJob::error | ( | ) | const |
◆ errorString()
|
virtual |
A human-readable error message.
This provides a translated, human-readable description of the error. Only call if error is not 0.
Subclasses should implement this to create a translated error message from the error code and error text. For example:
- Returns
- a translated error message, providing error() is 0
Reimplemented in Akonadi::Job, and KIO::Job.
◆ errorText()
QString KJob::errorText | ( | ) | const |
Returns the error text if there has been an error.
Only call if error is not 0.
This is usually some extra data associated with the error, such as a URL. Use errorString() to get a human-readable, translated message.
- Returns
- a string to help understand the error
◆ exec()
bool KJob::exec | ( | ) |
Executes the job synchronously.
This will start a nested QEventLoop internally. Nested event loop can be dangerous and can have unintended side effects, you should avoid calling exec() whenever you can and use the asynchronous interface of KJob instead.
Should you indeed call this method, you need to make sure that all callers are reentrant, so that events delivered by the inner event loop don't cause non-reentrant functions to be called, which usually wreaks havoc.
Note that the event loop started by this method does not process user input events, which means your user interface will effectively be blocked. Other events like paint or network events are still being processed. The advantage of not processing user input events is that the chance of accidental reentrance is greatly reduced. Still you should avoid calling this function.
- Returns
- true if the job has been executed without error, false otherwise
◆ finished
|
signal |
Emitted when the job is finished, in any case.
It is used to notify observers that the job is terminated and that progress can be hidden.
Since 5.75 this signal is guaranteed to be emitted exactly once.
This is a private signal, it can't be emitted directly by subclasses of KJob, use emitResult() instead.
In general, to be notified of a job's completion, client code should connect to result() rather than finished(), so that kill(Quietly) is indeed quiet. However if you store a list of jobs and they might get killed silently, then you must connect to this instead of result(), to avoid dangling pointers in your list.
- Parameters
-
job the job that emitted this signal
- See also
- result
◆ infoMessage
Emitted to display state information about this job.
Examples of message are "Resolving host", "Connecting to host...", etc.
- Parameters
-
job the job that emitted this signal message the info message
◆ isAutoDelete()
bool KJob::isAutoDelete | ( | ) | const |
◆ isFinished()
|
protected |
Returns if the job has been finished and has emitted the finished() signal.
- Returns
- if the job has been finished
- See also
- finished()
- Since
- 5.75
◆ isFinishedNotificationHidden()
bool KJob::isFinishedNotificationHidden | ( | ) | const |
Whether to not show a finished notification when a job's finished signal is emitted.
- See also
- setFinishedNotificationHidden()
- Since
- 5.92
◆ isStartedWithExec()
bool KJob::isStartedWithExec | ( | ) | const |
Returns true
if this job was started with exec(), which starts a nested event-loop (with QEventLoop::ExcludeUserInputEvents, which blocks the GUI), otherwise returns false
which indicates this job was started asynchronously with start().
This is useful for code that for example shows a dialog to ask the user a question, and that would be no-op since the user cannot interact with the dialog.
- Since
- 5.95
◆ isSuspended()
bool KJob::isSuspended | ( | ) | const |
◆ kill
|
slot |
Aborts this job.
This kills and deletes the job.
- Parameters
-
verbosity if equals to EmitResult, Job will emit signal result and ask uiserver to close the progress window. verbosity
is set to EmitResult for subjobs. Whether applications should call with Quietly or EmitResult depends on whether they rely on result being emitted or not. Please notice that ifverbosity
is set to Quietly, signal result will NOT be emitted.
- Returns
- true if the operation is supported and succeeded, false otherwise
◆ percent()
unsigned long KJob::percent | ( | ) | const |
◆ percentChanged
|
signal |
Progress signal showing the overall progress of the job.
This is valid for any kind of job, and allows using a progress bar very easily. (see KProgressBar).
Note that this signal is not emitted for finished jobs.
- Note
- This is a private signal, it shouldn't be emitted directly by subclasses of KJob, use emitPercent(), setPercent() setTotalAmount() or setProcessedAmount() instead.
- Parameters
-
job the job that emitted this signal percent the percentage
- Since
- 5.80
◆ processedAmount()
qulonglong KJob::processedAmount | ( | Unit | unit | ) | const |
◆ processedAmountChanged
|
signal |
Regularly emitted to show the progress of this job by giving the current amount.
The unit of this amount is sent too. It can be emitted several times if the job manages several different units.
- Note
- This is a private signal, it shouldn't be emitted directly by subclasses of KJob, use setProcessedAmount() instead.
- Parameters
-
job the job that emitted this signal unit the unit of the processed amount amount the processed amount
- Since
- 5.80
◆ processedSize
|
signal |
Regularly emitted to show the progress of this job (current data size in bytes for transfers, entries listed, etc.).
- Note
- This is a private signal, it shouldn't be emitted directly by subclasses of KJob, use setProcessedAmount() instead.
- Parameters
-
job the job that emitted this signal size the processed size
◆ result
|
signal |
Emitted when the job is finished (except when killed with KJob::Quietly).
Since 5.75 this signal is guaranteed to be emitted at most once.
Use error to know if the job was finished with error.
This is a private signal, it can't be emitted directly by subclasses of KJob, use emitResult() instead.
Please connect to this signal instead of finished.
- Parameters
-
job the job that emitted this signal
- See also
- kill
◆ resume
|
slot |
◆ resumed
|
signal |
Emitted when the job is resumed.
This is a private signal, it can't be emitted directly by subclasses of KJob.
- Parameters
-
job the job that emitted this signal
◆ setAutoDelete()
void KJob::setAutoDelete | ( | bool | autodelete | ) |
Sets the auto-delete property of the job.
If autodelete
is set to false
the job will not delete itself once it is finished.
The default for any KJob is to automatically delete itself, which implies that the job was created on the heap (using new
). If the job is created on the stack (which isn't the typical use-case for a job) then you must set auto-delete to false
, otherwise you could get a crash when the job finishes and tries to delete itself.
- Note
- If you set auto-delete to
false
then you need to kill the job manually, ideally by calling kill().
- Parameters
-
autodelete set to false
to disable automatic deletion of the job.
◆ setCapabilities()
|
protected |
◆ setError()
|
protected |
Sets the error code.
It should be called when an error is encountered in the job, just before calling emitResult().
You should define an (anonymous) enum of error codes, with values starting at KJob::UserDefinedError, and use those. For example,
- Parameters
-
errorCode the error code
- See also
- emitResult()
◆ setErrorText()
|
protected |
Sets the error text.
It should be called when an error is encountered in the job, just before calling emitResult().
Provides extra information about the error that cannot be determined directly from the error code. For example, a URL or filename. This string is not normally translatable.
- Parameters
-
errorText the error text
- See also
- emitResult(), errorString(), setError()
◆ setFinishedNotificationHidden()
void KJob::setFinishedNotificationHidden | ( | bool | hide = true | ) |
This method can be used to indicate to classes listening to signals from a job that they should ideally show a progress bar, but not a finished notification.
For example when opening a remote URL, a job will emit the progress of the download, which can be used to show a progress dialog or a Plasma notification, then when the job is done it'll emit e.g. the finished signal. Showing the user the progress dialog is useful, however the dialog/notification about the download being finished isn't of much interest, because the user can see the application that invoked the job opening the actual file that was downloaded.
- Since
- 5.92
◆ setPercent()
|
protected |
Sets the overall progress of the job.
The percent() signal is emitted if the value changed.
The job takes care of this if you call setProcessedAmount in Bytes (or the unit set by setProgressUnit). This method allows you to set your own progress, as an alternative.
- Parameters
-
percentage the new overall progress
◆ setProcessedAmount()
|
protected |
Sets the processed size.
The processedAmount() and percent() signals are emitted if the values changed. The percent() signal is emitted only for the progress unit.
- Parameters
-
unit the unit of the new processed amount amount the new processed amount
◆ setProgressUnit()
|
protected |
◆ setTotalAmount()
|
protected |
Sets the total size.
The totalSize() and percent() signals are emitted if the values changed. The percent() signal is emitted only for the progress unit.
- Parameters
-
unit the unit of the new total amount amount the new total amount
◆ setUiDelegate()
void KJob::setUiDelegate | ( | KJobUiDelegate * | delegate | ) |
Attach a UI delegate to this job.
If the job had another UI delegate, it's automatically deleted. Once attached to the job, the UI delegate will be deleted with the job.
- Parameters
-
delegate the new UI delegate to use
- See also
- KJobUiDelegate
◆ speed
|
signal |
Emitted to display information about the speed of this job.
- Note
- This is a private signal, it shouldn't be emitted directly by subclasses of KJob, use emitSpeed() instead.
- Parameters
-
job the job that emitted this signal speed the speed in bytes/s
◆ start()
|
pure virtual |
Starts the job asynchronously.
When the job is finished, result() is emitted.
Warning: Never implement any synchronous workload in this method. This method should just trigger the job startup, not do any work itself. It is expected to be non-blocking.
This is the method all subclasses need to implement. It should setup and trigger the workload of the job. It should not do any work itself. This includes all signals and terminating the job, e.g. by emitResult(). The workload, which could be another method of the subclass, is to be triggered using the event loop, e.g. by code like:
Implemented in Akonadi::AddContactJob, Akonadi::AddEmailAddressJob, Akonadi::AgentInstanceCreateJob, Akonadi::ContactGroupExpandJob, Akonadi::Job, Akonadi::MessageQueueJob, Akonadi::OpenEmailAddressJob, Akonadi::PartFetcher, Akonadi::RecursiveItemFetchJob, KAuth::ExecuteJob, KDAV2::DavCollectionCreateJob, KDAV2::DavCollectionDeleteJob, KDAV2::DavCollectionFetchJob, KDAV2::DavCollectionModifyJob, KDAV2::DavCollectionsFetchJob, KDAV2::DavCollectionsMultiFetchJob, KDAV2::DavDiscoveryJob, KDAV2::DavItemCreateJob, KDAV2::DavItemDeleteJob, KDAV2::DavItemFetchJob, KDAV2::DavItemModifyJob, KDAV2::DavItemsFetchJob, KDAV2::DavItemsListJob, KDAV2::DavPrincipalHomeSetsFetchJob, KDAV2::DavPrincipalSearchJob, KDAV::DavCollectionDeleteJob, KDAV::DavCollectionModifyJob, KDAV::DavCollectionsFetchJob, KDAV::DavCollectionsMultiFetchJob, KDAV::DavItemCreateJob, KDAV::DavItemDeleteJob, KDAV::DavItemFetchJob, KDAV::DavItemModifyJob, KDAV::DavItemsFetchJob, KDAV::DavItemsListJob, KDAV::DavPrincipalHomeSetsFetchJob, KDAV::DavPrincipalSearchJob, KEMailClientLauncherJob, KIO::ApplicationLauncherJob, KIO::CommandLauncherJob, KIO::DeleteOrTrashJob, KIO::FavIconRequestJob, KIO::MimeTypeFinderJob, KIO::NameFinderJob, KIO::OpenFileManagerWindowJob, KIO::OpenUrlJob, KListOpenFilesJob, KTerminalLauncherJob, MailTransport::PrecommandJob, MailTransport::TransportJob, MessageComposer::AliasesExpandJob, MessageComposer::ContentJobBase, MessageComposer::DistributionListExpandJob, MessageComposer::EmailAddressResolveJob, MessageCore::AttachmentCompressJob, MessageCore::AttachmentLoadJob, and Plasma5Support::ServiceJob.
◆ startElapsedTimer()
|
protected |
Starts the internal elapsed time measurement timer.
Sub-classes must call startElapsedTimer() from their start()
implementation, to get elapsedTime()
measurement. Otherwise elapsedTimer()
method will always return 0.
- Since
- 6.8
◆ suspend
|
slot |
◆ suspended
|
signal |
Emitted when the job is suspended.
This is a private signal, it can't be emitted directly by subclasses of KJob.
- Parameters
-
job the job that emitted this signal
◆ totalAmount()
qulonglong KJob::totalAmount | ( | Unit | unit | ) | const |
◆ totalAmountChanged
|
signal |
Emitted when we know the amount the job will have to process.
The unit of this amount is sent too. It can be emitted several times if the job manages several different units.
- Note
- This is a private signal, it shouldn't be emitted directly by subclasses of KJob, use setTotalAmount() instead.
- Parameters
-
job the job that emitted this signal unit the unit of the total amount amount the total amount
- Since
- 5.80
◆ totalSize
|
signal |
Emitted when we know the size of this job (data size in bytes for transfers, number of entries for listings, etc).
- Note
- This is a private signal, it shouldn't be emitted directly by subclasses of KJob, use setTotalAmount() instead.
- Parameters
-
job the job that emitted this signal size the total size
◆ uiDelegate()
KJobUiDelegate * KJob::uiDelegate | ( | ) | const |
◆ warning
Emitted to display a warning about this job.
- Parameters
-
job the job that emitted this signal message the warning message
Member Data Documentation
◆ d_ptr
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:00 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.