KPluginFactory
#include <KPluginFactory>
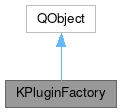
Classes | |
struct | InheritanceChecker |
struct | InheritanceWithMetaDataChecker |
class | Result |
Public Types | |
enum | ResultErrorReason { NO_PLUGIN_ERROR = 0 , INVALID_PLUGIN , INVALID_FACTORY , INVALID_KPLUGINFACTORY_INSTANTIATION } |
![]() | |
typedef | QObjectList |
Public Member Functions | |
KPluginFactory () | |
~KPluginFactory () override | |
template<typename T > | |
T * | create (QObject *parent=nullptr, const QVariantList &args={}) |
template<typename T > | |
T * | create (QWidget *parentWidget, QObject *parent, const QVariantList &args={}) |
KPluginMetaData | metaData () const |
void | setMetaData (const KPluginMetaData &metaData) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
template<typename T > | |
static Result< T > | instantiatePlugin (const KPluginMetaData &data, QObject *parent=nullptr, const QVariantList &args={}) |
static Result< KPluginFactory > | loadFactory (const KPluginMetaData &data) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Types | |
using | CreateInstanceWithMetaDataFunction = QObject *(*)(QWidget *, QObject *, const KPluginMetaData &, const QVariantList &) |
template<bool B, class T = void> | |
using | enable_if_t = typename std::enable_if<B, T>::type |
Protected Member Functions | |
virtual QObject * | create (const char *iface, QWidget *parentWidget, QObject *parent, const QVariantList &args) |
template<class T , enable_if_t< InheritanceChecker< T >::enabled, int > = 0> | |
void | registerPlugin () |
template<class T , enable_if_t< InheritanceWithMetaDataChecker< T >::enabled, int > = 0> | |
void | registerPlugin () |
template<class T > | |
void | registerPlugin (CreateInstanceWithMetaDataFunction instanceFunction) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Static Protected Member Functions | |
template<class impl , class ParentType > | |
static QObject * | createInstance (QWidget *, QObject *parent, const KPluginMetaData &, const QVariantList &args) |
template<class impl > | |
static QObject * | createPartWithMetaDataInstance (QWidget *parentWidget, QObject *parent, const KPluginMetaData &metaData, const QVariantList &args) |
template<class impl , class ParentType > | |
static QObject * | createWithMetaDataInstance (QWidget *, QObject *parent, const KPluginMetaData &metaData, const QVariantList &args) |
Related Symbols | |
(Note that these are not member symbols.) | |
#define | K_PLUGIN_CLASS(classname) |
#define | K_PLUGIN_CLASS_WITH_JSON(classname, jsonFile) |
#define | K_PLUGIN_FACTORY(name, pluginRegistrations) |
#define | K_PLUGIN_FACTORY_WITH_JSON(name, jsonFile, pluginRegistrations) |
Additional Inherited Members | |
![]() | |
objectName | |
Detailed Description
KPluginFactory provides a convenient way to provide factory-style plugins.
Qt plugins provide a singleton object, but a common pattern is for plugins to generate as many objects of a particular type as the application requires. By using KPluginFactory, you can avoid implementing the factory pattern yourself.
KPluginFactory also allows plugins to provide multiple different object types, indexed by keywords.
The objects created by KPluginFactory must inherit QObject, and must have a standard constructor pattern:
- if the object is a KPart::Part, it must be of the form QObject * parent() const constT(QWidget *parentWidget, QObject *parent, const KPluginMetaData &metaData, const QVariantList &args)This class allows easily accessing some standardized values from the JSON metadata that can be embedd...Definition kpluginmetadata.h:82
- if it is a QWidget, it must be of the form or
- otherwise it must be of the form or
You should typically use either K_PLUGIN_CLASS() or K_PLUGIN_CLASS_WITH_JSON() in your plugin code to generate a factory. The typical pattern is:
If you want to write a custom KPluginFactory not using the standard macro(s) you can reimplement the create(const char *iface, QWidget *parentWidget, QObject *parent, const QVariantList &args) method.
Example:
To load the KPluginFactory from an installed plugin you can use loadFactory and for directly creating a plugin instance from it instantiatePlugin
Definition at line 299 of file kpluginfactory.h.
Member Typedef Documentation
◆ CreateInstanceWithMetaDataFunction
|
protected |
Function pointer type to a function that instantiates a plugin For plugins that don't support a KPluginMetaData parameter it is discarded.
- Since
- 5.77
Definition at line 446 of file kpluginfactory.h.
◆ enable_if_t
|
protected |
Definition at line 501 of file kpluginfactory.h.
Member Enumeration Documentation
◆ ResultErrorReason
- Since
- 5.86
Definition at line 315 of file kpluginfactory.h.
Constructor & Destructor Documentation
◆ KPluginFactory()
|
explicit |
This constructor creates a factory for a plugin.
Definition at line 18 of file kpluginfactory.cpp.
◆ ~KPluginFactory()
|
overridedefault |
This destroys the PluginFactory.
Member Function Documentation
◆ create() [1/3]
|
protectedvirtual |
This function is called when the factory asked to create an Object.
You may reimplement it to provide a very flexible factory. This is especially useful to provide generic factories for plugins implemented using a scripting language.
- Parameters
-
iface the staticMetaObject::className() string identifying the plugin interface that was requested. E.g. for KCModule plugins this string will be "KCModule". parentWidget only used if the requested plugin is a KPart. parent the parent object for the plugin object. args a plugin specific list of arbitrary arguments.
Definition at line 113 of file kpluginfactory.cpp.
◆ create() [2/3]
|
inline |
Use this method to create an object.
It will try to create an object which inherits T
. If it has multiple choices it's not defined which object will be returned, so be careful to request a unique interface or use keywords.
- Template Parameters
-
T the interface for which an object should be created. The object will inherit T
.
- Parameters
-
parent the parent of the object. If parent
is a widget type, it will also passed to the parentWidget argument of the CreateInstanceFunction for the object.args additional arguments which will be passed to the object.
- Returns
- pointer to the created object is returned, or
nullptr
if an error occurred.
Definition at line 632 of file kpluginfactory.h.
◆ create() [3/3]
|
inline |
Use this method to create an object.
It will try to create an object which inherits T
This overload has an additional parentWidget
argument, which is used by some plugins (e.g. Parts).
- Template Parameters
-
T the interface for which an object should be created. The object will inherit T
.
- Parameters
-
parentWidget an additional parent widget. parent the parent of the object. If parent
is a widget type, it will also passed to the parentWidget argument of the CreateInstanceFunction for the object.args additional arguments which will be passed to the object. Since 5.93 this has a default arg.
- Returns
- pointer to the created object is returned, or
nullptr
if an error occurred.
Definition at line 644 of file kpluginfactory.h.
◆ createInstance()
|
inlinestaticprotected |
Definition at line 583 of file kpluginfactory.h.
◆ createPartWithMetaDataInstance()
|
inlinestaticprotected |
Definition at line 613 of file kpluginfactory.h.
◆ createWithMetaDataInstance()
|
inlinestaticprotected |
Definition at line 598 of file kpluginfactory.h.
◆ instantiatePlugin()
|
inlinestatic |
Attempts to load the KPluginFactory and create a T
instance from the given metadata KCoreAddons will log error messages automatically, meaning you only need to implement your own logging in case you want to give it more context info or have a custom category.
If there is no extra error handling needed the plugin can be directly accessed and checked if it is a nullptr
- Parameters
-
data KPluginMetaData from which the plugin should be loaded args arguments which get passed to the plugin's constructor
- Returns
- Result object which contains the plugin instance and potentially error information
- Since
- 5.86
Definition at line 373 of file kpluginfactory.h.
◆ loadFactory()
|
static |
Attempts to load the KPluginFactory from the given metadata.
The errors will be logged using the kf.coreaddons
debug category.
- Parameters
-
data KPluginMetaData from which the plugin should be loaded
- Returns
- Result object which contains the plugin instance and potentially error information
- Since
- 5.86
Definition at line 25 of file kpluginfactory.cpp.
◆ metaData()
KPluginMetaData KPluginFactory::metaData | ( | ) | const |
◆ registerPlugin() [1/3]
|
inlineprotected |
Uses a default instance creation function depending on the type of interface.
If the interface inherits from
KParts::Part
the function will callQWidget
the function will call- else the function will call
If those constructor methods are not callable this overload is not available.
Definition at line 522 of file kpluginfactory.h.
◆ registerPlugin() [2/3]
|
inlineprotected |
Uses a default instance creation function depending on the type of interface.
If the interface inherits from
KParts::Part
the function will callnew T(QWidget *parentWidget, QObject *parent, const KPluginMetaData &metaData, const QVariantList &args)QWidget
the function will call- else the function will call
If those constructor methods are not callable this overload is not available.
Definition at line 547 of file kpluginfactory.h.
◆ registerPlugin() [3/3]
|
inlineprotected |
Registers a plugin with the factory.
Call this function from the constructor of the KPluginFactory subclass to make the create function able to instantiate the plugin when asked for an interface the plugin implements.
- Parameters
-
T the name of the plugin class instanceFunction A function pointer to a function that creates an instance of the plugin.
- Since
- 5.96
Definition at line 563 of file kpluginfactory.h.
◆ setMetaData()
void KPluginFactory::setMetaData | ( | const KPluginMetaData & | metaData | ) |
Set the metadata about the plugin this factory generates.
- Parameters
-
metaData the metadata about the plugin
- Since
- 5.77
Definition at line 70 of file kpluginfactory.cpp.
Friends And Related Symbol Documentation
◆ K_PLUGIN_CLASS
|
related |
Creates a KPluginFactory subclass and exports it as the root plugin object.
Unlike K_PLUGIN_CLASS_WITH_JSON, this macro does not require json meta data.
This macro does the same as K_PLUGIN_FACTORY, but you only have to pass the class name. The factory name and registerPlugin call are deduced from the class name. This is also useful if you want to use static plugins, see the kcoreaddons_add_plugin CMake method.
- Since
- 5.90
Definition at line 205 of file kpluginfactory.h.
◆ K_PLUGIN_CLASS_WITH_JSON
|
related |
Create a KPluginFactory subclass and export it as the root plugin object with JSON metadata.
This macro does the same as K_PLUGIN_FACTORY_WITH_JSON, but you only have to pass the class name and the json file. The factory name and registerPlugin call are deduced from the class name.
in the same source file when that one has the name "myplugin.cpp".
Example:
- See also
- K_PLUGIN_FACTORY_WITH_JSON
- Since
- 5.44
Definition at line 188 of file kpluginfactory.h.
◆ K_PLUGIN_FACTORY
|
related |
Create a KPluginFactory subclass and export it as the root plugin object.
- Parameters
-
name the name of the KPluginFactory derived class. pluginRegistrations code to be inserted into the constructor of the class. Usually a series of registerPlugin() calls.
- Note
- K_PLUGIN_FACTORY declares the subclass including a Q_OBJECT macro. So you need to make sure to have Qt's moc run also for the source file where you use the macro. E.g. in projects using CMake and it's automoc feature, as usual you need to have a line in the same source file when that one has the name "myplugin.cpp".#include <myplugin.moc>
Example:
If you want to compile a .json file into the plugin, use K_PLUGIN_FACTORY_WITH_JSON.
- See also
- K_PLUGIN_FACTORY_WITH_JSON
- K_PLUGIN_FACTORY_DECLARATION
- K_PLUGIN_FACTORY_DEFINITION
Definition at line 91 of file kpluginfactory.h.
◆ K_PLUGIN_FACTORY_WITH_JSON
|
related |
Create a KPluginFactory subclass and export it as the root plugin object with JSON metadata.
This macro does the same as K_PLUGIN_FACTORY, but adds a JSON file as plugin metadata. See Q_PLUGIN_METADATA() for more information.
- Parameters
-
name the name of the KPluginFactory derived class. pluginRegistrations code to be inserted into the constructor of the class. Usually a series of registerPlugin() calls. jsonFile name of the JSON file to be compiled into the plugin as metadata
- Note
- K_PLUGIN_FACTORY_WITH_JSON declares the subclass including a Q_OBJECT macro. So you need to make sure to have Qt's moc run also for the source file where you use the macro. E.g. in projects using CMake and its automoc feature, as usual you need to have a line in the same source file when that one has the name "myplugin.cpp".#include <myplugin.moc>
Example:
- See also
- K_PLUGIN_FACTORY
- K_PLUGIN_FACTORY_DECLARATION
- K_PLUGIN_FACTORY_DEFINITION
- Since
- 5.0
Definition at line 145 of file kpluginfactory.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:49:14 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.