KDNSSD::ServiceBrowser
#include <KDNSSD/ServiceBrowser>
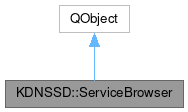
Public Types | |
enum | State { Working , Stopped , Unsupported } |
Signals | |
void | finished () |
void | serviceAdded (KDNSSD::RemoteService::Ptr service) |
void | serviceRemoved (KDNSSD::RemoteService::Ptr service) |
Public Member Functions | |
ServiceBrowser (const QString &type, bool autoResolve=false, const QString &domain=QString(), const QString &subtype=QString()) | |
bool | isAutoResolving () const |
QList< RemoteService::Ptr > | services () const |
virtual void | startBrowse () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QString | getLocalHostName () |
static State | isAvailable () |
static QHostAddress | resolveHostName (const QString &hostname) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual void | virtual_hook (int, void *) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
Browses for network services advertised over DNS-SD.
This is the central class in the KDNSSD library for applications that want to discover services on network.
Suppose that you need list of web servers running. Then you might do something like
In above example addService() will be called for every web server already running and for every web service that subsequently appears on the network and delService() will be called when a server previously advertised is stopped.
Because no domain was passed to constructor, the default domain will be searched. To find other domains to browse for services on, use DomainBrowser.
Definition at line 54 of file servicebrowser.h.
Member Enumeration Documentation
◆ State
Availability of DNS-SD services.
Enumerator | |
---|---|
Working | the service is available |
Stopped | not available because mDnsd or Avahi daemon is not running |
Unsupported | not available because KDE was compiled without DNS-SD support |
Definition at line 62 of file servicebrowser.h.
Constructor & Destructor Documentation
◆ ServiceBrowser()
|
explicit |
Create a ServiceBrowser for a particular service type.
DomainBrowser can be used to find other domains to browse on. If no domain is given, the default domain is used.
The service type is the high-level protocol type, followed by a dot, followed by the transport protocol type (_tcp
or _udp
). The DNS-SD website maintains a full list of service types.
The subtype
parameter allows you to do more fine-grained filtering on the services you are interested in. So you might request only FTP servers that allow anonymous access by passing "_ftp._tcp" as the type
and "_anon" as the subtype
. Subtypes are particularly important for types like _soap and _upnp, which are far too generic for most applications. In these cases, the subtype can be used to specify the particular SOAP or UPnP protocol they want.
- Warning
- Enabling
autoResolve
will increase network usage by resolving all services, so this feature should be used only when necessary.
- Parameters
-
type service types to browse for (example: "_http._tcp") autoResolve discovered services will be resolved before being reported with the serviceAdded() signal domain a domain to search on instead of the default one subtype only browse for a specific subtype
- See also
- startBrowse() and isAvailable()
Definition at line 20 of file avahi-servicebrowser.cpp.
◆ ~ServiceBrowser()
|
overridedefault |
Definition at line 34 of file dummy-servicebrowser.cpp.
Member Function Documentation
◆ finished
|
signal |
Emitted when the list of published services has settled.
This signal is emitted once after startBrowse() is called when all the services of the requested type that are currently published have been reported (even if none are available or the DNS-SD service is not available). It is emitted again when a new batch of services become available or disappear.
For example, if a new host is connected to network and announces some services watched for by this ServiceBrowser, they will be reported by one or more serviceAdded() signals and the whole batch will be concluded by finished().
This signal can be used by applications that just want to get a list of the currently available services (similar to a directory listing) and do not care about adding or removing services that appear or disappear later.
- Warning
- There is no guarantee any RemoteService pointers received by serviceAdded() will be valid by the time this signal is emitted, so you should either do all your work involving them in the slot receiving the serviceAdded() signal, or you should listen to serviceRemoved() as well.
- See also
- serviceAdded() and serviceRemoved()
◆ getLocalHostName()
|
static |
The mDNS hostname of the local machine.
Usually this will return the same as QHostInfo::localHostName(), but it may be changed to something different in the Avahi configuration file (if using the Avahi backend).
- Returns
- the hostname, or an empty string on failure
- Since
- 4.2
Definition at line 244 of file avahi-servicebrowser.cpp.
◆ isAutoResolving()
bool KDNSSD::ServiceBrowser::isAutoResolving | ( | ) | const |
Whether discovered services are resolved before being reported.
- Returns
- the value of the
autoResolve
parameter passed to the constructor
- Since
- 4.1
Definition at line 40 of file avahi-servicebrowser.cpp.
◆ isAvailable()
|
static |
Checks availability of DNS-SD services.
Although this method is part of ServiceBrowser, none of the classes in this library will be able to perform their intended function if this method does not return Working.
If this method does not return Working, it is still safe to call any of the methods in this library. However, no services will be found or published and no domains will be found.
If you use this function to report an error to the user, below is a suggestion on how to word the errors when publishing a service. The first line of each error message can also be used for reporting errors when browsing for services.
Definition at line 31 of file avahi-servicebrowser.cpp.
◆ resolveHostName()
|
static |
Resolves an mDNS hostname into an IP address.
This function is very rarely useful, since a properly configured system is able to resolve an mDNS-based host name using the system resolver (ie: you can just pass the mDNS hostname to KIO or other library).
- Parameters
-
hostname the hostname to be resolved
- Returns
- a QHostAddress containing the IP address, or QHostAddress() if resolution failed
- Since
- 4.2
Definition at line 225 of file avahi-servicebrowser.cpp.
◆ serviceAdded
|
signal |
Emitted when new service is discovered.
If isAutoResolving() returns true
, this will not be emitted until the service has been resolved.
- Parameters
-
service a RemoteService object describing the service
- See also
- serviceRemoved() and finished()
◆ serviceRemoved
|
signal |
Emitted when a service is no longer published over DNS-SD.
The RemoteService object is removed from the services() list and deleted immediately after this signal returns.
- Warning
- Do not use a delayed connection with this signal
- Parameters
-
service a RemoteService object describing the service
- See also
- serviceAdded() and finished()
◆ services()
QList< RemoteService::Ptr > KDNSSD::ServiceBrowser::services | ( | ) | const |
The currently known services of the specified type.
- Returns
- a list of RemoteService pointers
- See also
- serviceAdded() and serviceRemoved()
Definition at line 215 of file avahi-servicebrowser.cpp.
◆ startBrowse()
|
virtual |
Starts browsing for services.
Only the first call to this function will have any effect.
Browsing stops when the ServiceBrowser object is destroyed.
- Warning
- The serviceAdded() signal may be emitted before this function returns.
- See also
- serviceAdded(), serviceRemoved() and finished()
Definition at line 46 of file avahi-servicebrowser.cpp.
◆ virtual_hook()
|
protectedvirtual |
Definition at line 221 of file avahi-servicebrowser.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 3 2024 11:48:35 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.