KCoreDirLister
#include <KCoreDirLister>
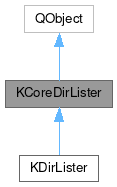
Public Types | |
enum | OpenUrlFlag { NoFlags = 0x0 , Keep = 0x1 , Reload = 0x2 } |
typedef QFlags< OpenUrlFlag > | OpenUrlFlags |
enum | WhichItems { AllItems = 0 , FilteredItems = 1 } |
Properties | |
bool | autoErrorHandlingEnabled |
bool | autoUpdate |
bool | delayedMimeTypes |
bool | dirOnlyMode |
QStringList | mimeFilter |
QString | nameFilter |
bool | requestMimeTypeWhileListing |
bool | showHiddenFiles |
![]() | |
objectName | |
Signals | |
void | canceled () |
void | clear () |
void | clearDir (const QUrl &dirUrl) |
void | completed () |
void | infoMessage (const QString &msg) |
void | itemsAdded (const QUrl &directoryUrl, const KFileItemList &items) |
void | itemsDeleted (const KFileItemList &items) |
void | itemsFilteredByMime (const KFileItemList &items) |
void | jobError (KIO::Job *job) |
void | listingDirCanceled (const QUrl &dirUrl) |
void | listingDirCompleted (const QUrl &dirUrl) |
void | newItems (const KFileItemList &items) |
void | percent (int percent) |
void | processedSize (KIO::filesize_t size) |
void | redirection (const QUrl &oldUrl, const QUrl &newUrl) |
void | refreshItems (const QList< QPair< KFileItem, KFileItem > > &items) |
void | speed (int bytes_per_second) |
void | started (const QUrl &dirUrl) |
void | totalSize (KIO::filesize_t size) |
Public Member Functions | |
KCoreDirLister (QObject *parent=nullptr) | |
~KCoreDirLister () override | |
bool | autoErrorHandlingEnabled () const |
bool | autoUpdate () const |
void | clearMimeFilter () |
bool | delayedMimeTypes () const |
QList< QUrl > | directories () const |
bool | dirOnlyMode () const |
void | emitChanges () |
KFileItem | findByName (const QString &name) const |
KFileItem | findByUrl (const QUrl &url) const |
void | forgetDirs (const QUrl &dirUrl) |
bool | isFinished () const |
KFileItemList | items (WhichItems which=FilteredItems) const |
KFileItemList | itemsForDir (const QUrl &dirUrl, WhichItems which=FilteredItems) const |
QStringList | mimeFilters () const |
QString | nameFilter () const |
bool | openUrl (const QUrl &dirUrl, OpenUrlFlags flags=NoFlags) |
bool | requestMimeTypeWhileListing () const |
KFileItem | rootItem () const |
void | setAutoErrorHandlingEnabled (bool enable) |
void | setAutoUpdate (bool enable) |
void | setDelayedMimeTypes (bool delayedMimeTypes) |
void | setDirOnlyMode (bool dirsOnly) |
void | setMimeExcludeFilter (const QStringList &mimeList) |
void | setMimeFilter (const QStringList &mimeList) |
void | setNameFilter (const QString &filter) |
void | setRequestMimeTypeWhileListing (bool request) |
void | setShowHiddenFiles (bool showHiddenFiles) |
bool | showHiddenFiles () const |
void | stop () |
void | stop (const QUrl &dirUrl) |
void | updateDirectory (const QUrl &dirUrl) |
QUrl | url () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static KFileItem | cachedItemForUrl (const QUrl &url) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual void | jobStarted (KIO::ListJob *) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
Detailed Description
Helper class for the kiojob used to list and update a directory.
The dir lister deals with the kiojob used to list and update a directory and has signals for the user of this class (e.g. konqueror view or kdesktop) to create/destroy its items when asked.
This class is independent from the graphical representation of the dir (icon container, tree view, ...) and it stores the items (as KFileItems).
Typical usage :
- Create an instance.
- Connect to at least update, clear, itemsAdded, and itemsDeleted.
- Call openUrl - the signals will be called.
- Reuse the instance when opening a new url (openUrl).
- Destroy the instance when not needed anymore (usually destructor).
Advanced usage : call openUrl with OpenUrlFlag::Keep to list directories without forgetting the ones previously read (e.g. for a tree view)
Definition at line 53 of file kcoredirlister.h.
Member Typedef Documentation
◆ OpenUrlFlags
typedef QFlags< OpenUrlFlag > KCoreDirLister::OpenUrlFlags |
Stores a combination of OpenUrlFlag values.
Definition at line 89 of file kcoredirlister.h.
Member Enumeration Documentation
◆ OpenUrlFlag
- See also
- OpenUrlFlags
Enumerator | |
---|---|
NoFlags | No additional flags specified. |
Keep | Previous directories aren't forgotten. (they are still watched by kdirwatch and their items are kept for this KCoreDirLister). This is useful for e.g. a treeview. |
Reload | Indicates whether to use the cache or to reread the directory from the disk. Use only when opening a dir not yet listed by this lister without using the cache. Otherwise use updateDirectory. |
Definition at line 72 of file kcoredirlister.h.
◆ WhichItems
Used by items() and itemsForDir() to specify whether you want all items for a directory or just the filtered ones.
Definition at line 393 of file kcoredirlister.h.
Property Documentation
◆ autoErrorHandlingEnabled
|
readwrite |
Definition at line 66 of file kcoredirlister.h.
◆ autoUpdate
|
readwrite |
Definition at line 59 of file kcoredirlister.h.
◆ delayedMimeTypes
|
readwrite |
Definition at line 62 of file kcoredirlister.h.
◆ dirOnlyMode
|
readwrite |
Definition at line 61 of file kcoredirlister.h.
◆ mimeFilter
|
readwrite |
Definition at line 65 of file kcoredirlister.h.
◆ nameFilter
|
readwrite |
Definition at line 64 of file kcoredirlister.h.
◆ requestMimeTypeWhileListing
|
readwrite |
Definition at line 63 of file kcoredirlister.h.
◆ showHiddenFiles
|
readwrite |
Definition at line 60 of file kcoredirlister.h.
Constructor & Destructor Documentation
◆ KCoreDirLister()
KCoreDirLister::KCoreDirLister | ( | QObject * | parent = nullptr | ) |
Create a directory lister.
Definition at line 2098 of file kcoredirlister.cpp.
◆ ~KCoreDirLister()
|
override |
Destroy the directory lister.
Definition at line 2111 of file kcoredirlister.cpp.
Member Function Documentation
◆ autoErrorHandlingEnabled()
bool KCoreDirLister::autoErrorHandlingEnabled | ( | ) | const |
Checks whether auto error handling is enabled.
If enabled, it will show an error dialog to the user when an error occurs (assuming the application links to KIOWidgets). It is turned on by default.
- Returns
true
if auto error handling is enabled,false
otherwise
- See also
- setAutoErrorHandlingEnabled()
- Since
- 5.82
Definition at line 2758 of file kcoredirlister.cpp.
◆ autoUpdate()
bool KCoreDirLister::autoUpdate | ( | ) | const |
Checks whether KDirWatch will automatically update directories.
This is enabled by default.
- Returns
true
if KDirWatch is used to automatically update directories
Definition at line 2150 of file kcoredirlister.cpp.
◆ cachedItemForUrl()
Return the KFileItem for the given URL, if it was listed recently and it's still in the cache, which is always the case if a directory view is currently showing this item.
If not, then it might be in the cache; if not in the cache a a null KFileItem will be returned.
If you really need a KFileItem for this URL in all cases, then use KIO::stat() instead.
Definition at line 2749 of file kcoredirlister.cpp.
◆ canceled
|
signal |
Tell the view that the user canceled the listing.
No running jobs are left.
◆ clear
|
signal |
Signals to the view to remove all items (when e.g. going from dirA to dirB).
Make sure to connect to this signal to avoid having duplicate items in the view.
◆ clearDir
|
signal |
Signals to the view to clear all items from directory dirUrl
.
This is only emitted if the lister is holding more than one directory.
- Parameters
-
dirUrl the directory that the view should clear all items from
- Since
- 5.79
◆ clearMimeFilter()
void KCoreDirLister::clearMimeFilter | ( | ) |
Clears the MIME type based filter.
You need to call emitChanges() afterwards.
- See also
- setMimeFilter
Definition at line 2367 of file kcoredirlister.cpp.
◆ completed
|
signal |
Tell the view that listing is finished.
There are no jobs running anymore.
◆ delayedMimeTypes()
bool KCoreDirLister::delayedMimeTypes | ( | ) | const |
- Returns
true
if the "delayed MIME types" feature was enabled
- See also
- setDelayedMimeTypes
Definition at line 2683 of file kcoredirlister.cpp.
◆ directories()
Returns all URLs that are listed by this KCoreDirLister.
This is only useful if you called openUrl() with OpenUrlFlag::Keep, as it happens in a treeview, for example. (Note that the base url is included in the list as well, of course.)
- Returns
- a list of all listed URLs
Definition at line 2220 of file kcoredirlister.cpp.
◆ dirOnlyMode()
bool KCoreDirLister::dirOnlyMode | ( | ) | const |
Checks whether this KCoreDirLister only lists directories or all items (directories and files), by default all items are listed.
- Returns
true
if only directories are listed,false
otherwise
- See also
- setDirOnlyMode(bool)
Definition at line 2180 of file kcoredirlister.cpp.
◆ emitChanges()
void KCoreDirLister::emitChanges | ( | ) |
Actually emit the changes made with setShowHiddenFiles, setDirOnlyMode, setNameFilter and setMimeFilter.
Definition at line 2225 of file kcoredirlister.cpp.
◆ findByName()
Find an item by its name.
- Parameters
-
name the item name
- Returns
- the KFileItem
Definition at line 2314 of file kcoredirlister.cpp.
◆ findByUrl()
Find an item by its URL.
- Parameters
-
url the item URL
- Returns
- the KFileItem
Definition at line 2309 of file kcoredirlister.cpp.
◆ forgetDirs()
void KCoreDirLister::forgetDirs | ( | const QUrl & | dirUrl | ) |
Stop listening for further changes in the given directory.
When a new directory is opened with OpenUrlFlag::Keep the caller will keep being notified of file changes for all directories that were kept open. This call selectively removes a directory from sending future notifications to this KCoreDirLister.
- Parameters
-
dirUrl the directory URL.
- Since
- 5.91
Definition at line 2145 of file kcoredirlister.cpp.
◆ infoMessage
|
signal |
Emitted to display information about running jobs.
Examples of message are "Resolving host", "Connecting to host...", etc.
- Parameters
-
msg the info message
◆ isFinished()
bool KCoreDirLister::isFinished | ( | ) | const |
Returns true
if no I/O operation is currently in progress.
- Returns
true
if finished,false
otherwise
Definition at line 2299 of file kcoredirlister.cpp.
◆ items()
KFileItemList KCoreDirLister::items | ( | WhichItems | which = FilteredItems | ) | const |
Returns the items listed for the current url().
This method will not start listing a directory, you should only call this in a slot connected to the finished() signal.
The items in the KFileItemList are copies of the items used by KCoreDirLister.
- Parameters
-
which specifies whether the returned list will contain all entries or only the ones that passed the nameFilter(), mimeFilter(), etc. Note that the latter causes iteration over all the items, filtering them. If this is too slow for you, use the newItems() signal, sending out filtered items in chunks
- Returns
- the items listed for the current url()
Definition at line 2660 of file kcoredirlister.cpp.
◆ itemsAdded
|
signal |
Signal that new items were found during directory listing.
Alternative signal emitted at the same time as newItems(), but itemsAdded also passes the url of the parent directory.
- Parameters
-
items a list of new items
◆ itemsDeleted
|
signal |
Signal that items have been deleted.
- Since
- 4.1.2
- Parameters
-
items the list of deleted items
◆ itemsFilteredByMime
|
signal |
Send a list of items filtered-out by MIME type.
- Parameters
-
items the list of filtered items
◆ itemsForDir()
KFileItemList KCoreDirLister::itemsForDir | ( | const QUrl & | dirUrl, |
WhichItems | which = FilteredItems ) const |
Returns the items listed for the given dirUrl
.
This method will not start listing dirUrl
, you should only call this in a slot connected to the finished() signal.
The items in the KFileItemList are copies of the items used by KCoreDirLister.
- Parameters
-
dirUrl specifies the url for which the items should be returned. This is only useful if you use KCoreDirLister with multiple URLs i.e. using bool OpenUrlFlag::Keep in openUrl() which specifies whether the returned list will contain all entries or only the ones that passed the nameFilter, mimeFilter, etc. Note that the latter causes iteration over all the items, filtering them. If this is too slow for you, use the newItems() signal, sending out filtered items in chunks
- Returns
- the items listed for
dirUrl
Definition at line 2665 of file kcoredirlister.cpp.
◆ jobError
|
signal |
Emitted if listing a directory fails with an error.
A typical implementation in a widgets-based application would show a message box by calling this in a slot connected to this signal: job->uiDelegate()->showErrorMessage()
Many applications might prefer to embed the error message though (e.g. by using the KMessageWidget class, from the KWidgetsAddons Framework).
- Parameters
-
the job with an error
- Since
- 5.82
◆ jobStarted()
|
protectedvirtual |
Reimplemented by KDirLister to associate windows with jobs.
- Since
- 5.0
Reimplemented in KDirLister.
Definition at line 2627 of file kcoredirlister.cpp.
◆ listingDirCanceled
|
signal |
Tell the view that the listing of the directory dirUrl
was canceled.
There might be other running jobs left.
- Parameters
-
dirUrl the directory URL
- Since
- 5.79
◆ listingDirCompleted
|
signal |
Tell the view that the listing of the directory dirUrl
is finished.
There might be other running jobs left.
- Parameters
-
dirUrl the directory URL
- Since
- 5.79
◆ mimeFilters()
QStringList KCoreDirLister::mimeFilters | ( | ) | const |
Returns the list of MIME type based filters, as set via setMimeFilter().
- Returns
- the list of MIME type based filters. Empty, when no MIME type filter is set.
Definition at line 2374 of file kcoredirlister.cpp.
◆ nameFilter()
QString KCoreDirLister::nameFilter | ( | ) | const |
Returns the current name filter, as set via setNameFilter()
- Returns
- the current name filter, can be QString() if filtering is turned off
Definition at line 2338 of file kcoredirlister.cpp.
◆ newItems
|
signal |
Signal new items.
- Parameters
-
items a list of new items
◆ openUrl()
bool KCoreDirLister::openUrl | ( | const QUrl & | dirUrl, |
OpenUrlFlags | flags = NoFlags ) |
Run the directory lister on the given url.
This method causes KCoreDirLister to emit all the items of dirUrl
, in any case. Depending on flags
, either clear() or clearDir(const QUrl &) will be emitted first.
The newItems() signal may be emitted more than once to supply you with KFileItems, up until the signal completed() is emitted (and isFinished() returns true
).
- Parameters
-
dirUrl the directory URL. flags whether to keep previous directories, and whether to reload, see OpenUrlFlags
- Returns
true
if successful,false
otherwise (e.g. ifdirUrl
is invalid)
Definition at line 2123 of file kcoredirlister.cpp.
◆ percent
|
signal |
Progress signal showing the overall progress of the KCoreDirLister.
This allows using a progress bar very easily. (see QProgressBar)
- Parameters
-
percent the progress in percent
◆ processedSize
|
signal |
Regularly emitted to show the progress of this KCoreDirLister.
- Parameters
-
size the processed size in bytes
◆ redirection
Signals a redirection.
- Parameters
-
oldUrl the original URL newUrl the new URL
◆ refreshItems
Signal an item to refresh (its MIME-type/icon/name has changed).
Note: KFileItem::refresh has already been called on those items.
- Parameters
-
items the items to refresh. This is a list of pairs, where the first item in the pair is the OLD item, and the second item is the NEW item. This allows to track which item has changed, especially after a renaming.
◆ requestMimeTypeWhileListing()
bool KCoreDirLister::requestMimeTypeWhileListing | ( | ) | const |
Checks whether this KCoreDirLister requests the MIME type of files from the worker.
Enabling this will tell the worker used for listing that it should try to determine the mime type of entries while listing them. This potentially reduces the speed at which entries are listed but ensures mime types are immediately available when an entry is added, greatly speeding up things like mime type filtering.
By default this is disabled.
- Returns
true
if the worker is asked for MIME types,false
otherwise.
- Since
- 5.82
Definition at line 2195 of file kcoredirlister.cpp.
◆ rootItem()
KFileItem KCoreDirLister::rootItem | ( | ) | const |
Returns the file item of the URL.
Can return an empty KFileItem.
- Returns
- the file item for url() itself (".")
Definition at line 2304 of file kcoredirlister.cpp.
◆ setAutoErrorHandlingEnabled()
void KCoreDirLister::setAutoErrorHandlingEnabled | ( | bool | enable | ) |
Enable or disable auto error handling.
If enabled, it will show an error dialog to the user when an error occurs. It is turned on by default.
- Parameters
-
enable true to enable auto error handling, false to disable parent the parent widget for the error dialogs, can be nullptr
for top-level
- See also
- autoErrorHandlingEnabled()
- Since
- 5.82
Definition at line 2763 of file kcoredirlister.cpp.
◆ setAutoUpdate()
void KCoreDirLister::setAutoUpdate | ( | bool | enable | ) |
Toggle automatic directory updating, when a directory changes (using KDirWatch).
- Parameters
-
enable set to true
to enable orfalse
to disable
Definition at line 2155 of file kcoredirlister.cpp.
◆ setDelayedMimeTypes()
void KCoreDirLister::setDelayedMimeTypes | ( | bool | delayedMimeTypes | ) |
Delayed MIME types feature: If enabled, MIME types will be fetched on demand, which leads to a faster initial directory listing, where icons get progressively replaced with the correct one while KMimeTypeResolver is going through the items with unknown or imprecise MIME type (e.g.
files with no extension or an unknown extension).
Definition at line 2688 of file kcoredirlister.cpp.
◆ setDirOnlyMode()
void KCoreDirLister::setDirOnlyMode | ( | bool | dirsOnly | ) |
Call this to list only directories (by default all items (directories and files) are listed).
You need to call emitChanges() afterwards.
- Parameters
-
dirsOnly set to true
to list only directories
Definition at line 2185 of file kcoredirlister.cpp.
◆ setMimeExcludeFilter()
void KCoreDirLister::setMimeExcludeFilter | ( | const QStringList & | mimeList | ) |
Filtering should be done with KFileFilter.
This will be implemented in a later revision of KCoreDirLister. This method may be removed then.
Set MIME type based exclude filter to only list items not matching the given MIME types
NOTE: setting the filter does not automatically reload directory. Also calling this function will not affect any named filter already set.
- Parameters
-
mimeList a list of MIME types
- See also
- clearMimeFilter
- matchesMimeFilter
Definition at line 2357 of file kcoredirlister.cpp.
◆ setMimeFilter()
void KCoreDirLister::setMimeFilter | ( | const QStringList & | mimeList | ) |
Set MIME type based filter to only list items matching the given MIME types.
NOTE: setting the filter does not automatically reload directory. Also calling this function will not affect any named filter already set.
You need to call emitChanges() afterwards.
- Parameters
-
mimeList a list of MIME types
- See also
- clearMimeFilter
- matchesMimeFilter
Definition at line 2343 of file kcoredirlister.cpp.
◆ setNameFilter()
void KCoreDirLister::setNameFilter | ( | const QString & | filter | ) |
Set a name filter to only list items matching this name, e.g. "*.cpp".
You can set more than one filter by separating them with whitespace, e.g "*.cpp *.h". Note: the directory is not automatically reloaded. You need to call emitChanges() afterwards.
- Parameters
-
filter the new filter, QString() to disable filtering
- See also
- matchesFilter
Definition at line 2321 of file kcoredirlister.cpp.
◆ setRequestMimeTypeWhileListing()
void KCoreDirLister::setRequestMimeTypeWhileListing | ( | bool | request | ) |
Toggles whether to request MIME types from the worker or in-process.
- Parameters
-
request set to true
to request MIME types from the worker.
- Note
- If this is changed while the lister is already listing a directory, it will only have an effect the next time openUrl() is called.
- See also
- requestMimeTypeWhileListing()
- Since
- 5.82
Definition at line 2200 of file kcoredirlister.cpp.
◆ setShowHiddenFiles()
void KCoreDirLister::setShowHiddenFiles | ( | bool | showHiddenFiles | ) |
Toggles whether hidden files (e.g.
files whose name start with '.' on Unix) are shown/ By default hidden files are not shown.
You need to call emitChanges() afterwards.
- Parameters
-
showHiddenFiles set to true/false
to show/hide hidden files respectively
- See also
- showHiddenFiles()
- Since
- 5.100
Definition at line 2170 of file kcoredirlister.cpp.
◆ showHiddenFiles()
bool KCoreDirLister::showHiddenFiles | ( | ) | const |
Checks whether hidden files (e.g.
files whose name start with '.' on Unix) will be shown. By default this option is disabled (hidden files are not shown).
- Returns
true
if hidden files are shown,false
otherwise
- See also
- setShowHiddenFiles()
- Since
- 5.100
Definition at line 2165 of file kcoredirlister.cpp.
◆ speed
|
signal |
Emitted to display information about the speed of the jobs.
- Parameters
-
bytes_per_second the speed in bytes/s
◆ started
|
signal |
Tell the view that this KCoreDirLister has started to list dirUrl
.
Note that this does not imply that there is really a job running! I.e. KCoreDirLister::jobs() may return an empty list, in which case the items are taken from the cache.
The view knows that openUrl should start it, so this might seem useless, but the view also needs to know when an automatic update happens.
- Parameters
-
dirUrl the URL to list
◆ stop() [1/2]
void KCoreDirLister::stop | ( | ) |
Stop listing all directories currently being listed.
Emits canceled() if there was at least one job running. Emits listingDirCanceled(const QUrl &) for each stopped job if there is more than one directory being watched by this KCoreDirLister.
Definition at line 2135 of file kcoredirlister.cpp.
◆ stop() [2/2]
void KCoreDirLister::stop | ( | const QUrl & | dirUrl | ) |
Stop listing the given directory.
Emits canceled() if the killed job was the last running one. Emits listingDirCanceled(const QUrl &) for the killed job if there is more than one directory being watched by this KCoreDirLister.
No signal is emitted if there was no job running for dirUrl
.
- Parameters
-
dirUrl the directory URL
Definition at line 2140 of file kcoredirlister.cpp.
◆ totalSize
|
signal |
Emitted when we know the size of the jobs.
- Parameters
-
size the total size in bytes
◆ updateDirectory()
void KCoreDirLister::updateDirectory | ( | const QUrl & | dirUrl | ) |
Update the directory dirUrl
.
This method causes KCoreDirLister to only emit the items of dirUrl
that actually changed compared to the current state in the cache, and updates the cache.
The current implementation calls updateDirectory automatically for local files, using KDirWatch (if autoUpdate() is true
), but it might be useful to force an update manually.
- Parameters
-
dirUrl the directory URL
Definition at line 2294 of file kcoredirlister.cpp.
◆ url()
QUrl KCoreDirLister::url | ( | ) | const |
Returns the top level URL that is listed by this KCoreDirLister.
It might be different from the one given with openUrl() if there was a redirection. If you called openUrl() with OpenUrlFlag::Keep this is the first url opened (e.g. in a treeview this is the root).
- Returns
- the url used by this instance to list the files
Definition at line 2215 of file kcoredirlister.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:13:16 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.