KIO::AskUserActionInterface
#include <KIO/AskUserActionInterface>
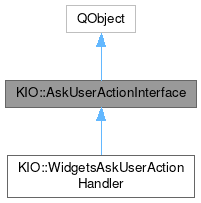
Public Types | |
enum | ConfirmationType { DefaultConfirmation , ForceConfirmation } |
enum | DeletionType { Delete , Trash , EmptyTrash , DeleteInsteadOfTrash } |
enum | MessageDialogType { QuestionTwoActions = 1 , QuestionTwoActionsCancel = 2 , WarningTwoActions = 3 , WarningTwoActionsCancel = 4 , WarningContinueCancel = 5 , Information = 7 , Error = 9 } |
Signals | |
void | askIgnoreSslErrorsResult (int result) |
void | askUserDeleteResult (bool allowDelete, const QList< QUrl > &urls, KIO::AskUserActionInterface::DeletionType deletionType, QWidget *parent) |
void | askUserRenameResult (KIO::RenameDialog_Result result, const QUrl &newUrl, KJob *parentJob) |
void | askUserSkipResult (KIO::SkipDialog_Result result, KJob *parentJob) |
void | messageBoxResult (int result) |
Public Member Functions | |
~AskUserActionInterface () override | |
virtual void | askIgnoreSslErrors (const QVariantMap &sslErrorData, QWidget *parent)=0 |
virtual void | askUserDelete (const QList< QUrl > &urls, DeletionType deletionType, ConfirmationType confirmationType, QWidget *parent=nullptr)=0 |
virtual void | requestUserMessageBox (MessageDialogType type, const QString &text, const QString &title, const QString &primaryActionText, const QString &secondatyActionText, const QString &primaryActionIconName={}, const QString &secondatyActionIconName={}, const QString &dontAskAgainName={}, const QString &details={}, QWidget *parent=nullptr)=0 |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
AskUserActionInterface (QObject *parent=nullptr) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Related Symbols | |
(Note that these are not member symbols.) | |
virtual void | askUserRename (KJob *job, const QString &title, const QUrl &src, const QUrl &dest, KIO::RenameDialog_Options options, KIO::filesize_t sizeSrc, KIO::filesize_t sizeDest, const QDateTime &ctimeSrc={}, const QDateTime &ctimeDest={}, const QDateTime &mtimeSrc={}, const QDateTime &mtimeDest={})=0 |
virtual void | askUserSkip (KJob *job, KIO::SkipDialog_Options options, const QString &errorText)=0 |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The AskUserActionInterface class allows a KIO::Job to prompt the user for a decision when e.g. copying directories/files and there is a conflict (e.g. a file with the same name already exists at the destination).
The methods in this interface are similar to their counterparts in KIO::JobUiDelegateExtension, the main difference is that AskUserActionInterface shows the dialogs using show() or open(), rather than exec(), the latter creates a nested event loop which could lead to crashes.
- See also
- KIO::JobUiDelegateExtension
- Since
- 5.78
Definition at line 43 of file askuseractioninterface.h.
Member Enumeration Documentation
◆ ConfirmationType
Deletion confirmation type.
Used by askUserDelete().
Enumerator | |
---|---|
DefaultConfirmation | Do not ask if the user has previously set the "Do not ask again" checkbox (which is is shown in the message dialog invoked by askUserDelete()) |
ForceConfirmation | Always ask the user for confirmation. |
Definition at line 132 of file askuseractioninterface.h.
◆ DeletionType
The type of deletion.
Used by askUserDelete().
Definition at line 114 of file askuseractioninterface.h.
◆ MessageDialogType
Enumerator | |
---|---|
QuestionTwoActions |
|
QuestionTwoActionsCancel |
|
WarningTwoActions |
|
WarningTwoActionsCancel |
|
Definition at line 161 of file askuseractioninterface.h.
Constructor & Destructor Documentation
◆ AskUserActionInterface()
|
explicitprotected |
Constructor.
Definition at line 18 of file askuseractioninterface.cpp.
◆ ~AskUserActionInterface()
|
override |
Destructor.
Definition at line 23 of file askuseractioninterface.cpp.
Member Function Documentation
◆ askUserDelete()
|
pure virtual |
Ask for confirmation before moving urls
(files/directories) to the Trash, emptying the Trash, or directly deleting files (i.e.
without moving to Trash).
Note that this method is not called automatically by KIO jobs. It's the application's responsibility to ask the user for confirmation before calling KIO::del() or KIO::trash().
You need to connect to the askUserDeleteResult signal to get the dialog's result (exit code).
- Parameters
-
urls the urls about to be moved to the Trash or deleted directly deletionType the type of deletion (Delete for real deletion, Trash otherwise), see the DeletionType enum confirmationType The type of deletion confirmation, see the ConfirmationType enum. Normally set to DefaultConfirmation parent the parent widget of the message box
Implemented in KIO::WidgetsAskUserActionHandler.
◆ askUserDeleteResult
|
signal |
Implementations of this interface must emit this signal when the dialog invoked by askUserDelete() finishes, to notify the caller of the user's decision.
- Parameters
-
allowDelete set to true if the user confirmed the delete operation, otherwise set to false urls a list of urls to delete/trash deletionType the deletion type to use, one of KIO::AskUserActionInterface::DeletionType parent the parent widget passed to askUserDelete(), for request identification
◆ askUserRenameResult
|
signal |
Implementations of this interface must emit this signal when the rename dialog finishes, to notify the caller of the dialog's result.
- Parameters
-
result the exit code from the rename dialog, one of the KIO::RenameDialog_Result enum newUrl the new destination URL set by the user parentJob the job that invoked the dialog
◆ askUserSkipResult
|
signal |
Implementations of this interface must emit this signal when the skip dialog finishes, to notify the caller of the dialog's result.
- Parameters
-
result the exit code from the skip dialog, one of the KIO::SkipDialog_Result enum parentJob the job that invoked the dialog
◆ messageBoxResult
|
signal |
Implementations of this interface must emit this signal when the dialog invoked by requestUserMessageBox() finishes, to notify the caller of the dialog's result (exit code).
- Parameters
-
result the exit code of the dialog, one of KIO::WorkerBase::ButtonCode enum
◆ requestUserMessageBox()
|
pure virtual |
This function allows for the delegation of user prompts from the KIO worker.
- Parameters
-
type the desired type of message box, see the MessageDialogType enum text the message to show to the user title the title of the message dialog box primaryActionText the text for the primary action secondatyActionText the text for the secondary action primaryActionIconName the icon to show on the primary action secondatyActionIconName the icon to show on the secondary action dontAskAgainName the config key name used to store the result from 'Do not ask again' checkbox details more details about the message shown to the user parent the parent widget of the dialog
Implemented in KIO::WidgetsAskUserActionHandler.
Friends And Related Symbol Documentation
◆ askUserRename()
|
related |
Constructs a modal, parent-less "rename" dialog, to prompt the user for a decision in case of conflicts, while copying/moving files.
The dialog is shown using open(), rather than exec() (the latter creates a nested eventloop which could lead to crashes). You will need to connect to the askUserRenameResult() signal to get the dialog's result (exit code). The exit code is one of KIO::RenameDialog_Result.
- See also
- KIO::RenameDialog_Result enum.
- Parameters
-
job the job that called this method title the title for the dialog box src the URL of the file/dir being copied/moved dest the URL of the destination file/dir, i.e. the one that already exists options parameters for the dialog (which buttons to show... etc), OR'ed values from the KIO::RenameDialog_Options enum sizeSrc size of the source file sizeDest size of the destination file ctimeSrc creation time of the source file ctimeDest creation time of the destination file mtimeSrc modification time of the source file mtimeDest modification time of the destination file
Implemented in KIO::WidgetsAskUserActionHandler.
◆ askUserSkip()
|
related |
You need to connect to the askUserSkipResult signal to get the dialog's result.
- Parameters
-
job the job that called this method options parameters for the dialog (which buttons to show... etc), OR'ed values from the KIO::SkipDialog_Options enum error_text the error text to show to the user (usually the string returned by KJob::errorText())
Implemented in KIO::WidgetsAskUserActionHandler.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:18:52 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.