KPty
#include <kpty.h>
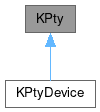
Public Member Functions | |
KPty () | |
KPty (const KPty &)=delete | |
~KPty () | |
void | close () |
void | closeSlave () |
void | login (const char *user=nullptr, const char *remotehost=nullptr) |
void | logout () |
int | masterFd () const |
bool | open () |
bool | open (int fd) |
bool | openSlave () |
KPty & | operator= (const KPty &)=delete |
void | setCTty () |
void | setCTtyEnabled (bool enable) |
bool | setEcho (bool echo) |
bool | setWinSize (int lines, int columns) |
bool | setWinSize (int lines, int columns, int height, int width) |
int | slaveFd () const |
bool | tcGetAttr (struct ::termios *ttmode) const |
bool | tcSetAttr (struct ::termios *ttmode) |
const char * | ttyName () const |
Protected Member Functions | |
KPTY_NO_EXPORT | KPty (KPtyPrivate *d) |
Protected Attributes | |
std::unique_ptr< KPtyPrivate > const | d_ptr |
Detailed Description
Provides primitives for opening & closing a pseudo TTY pair, assigning the controlling TTY, utmp registration and setting various terminal attributes.
Constructor & Destructor Documentation
◆ KPty() [1/2]
◆ ~KPty()
KPty::~KPty | ( | ) |
◆ KPty() [2/2]
Member Function Documentation
◆ close()
◆ closeSlave()
void KPty::closeSlave | ( | ) |
Close the pty slave descriptor.
When creating the pty, KPty also opens the slave and keeps it open. Consequently the master will never receive an EOF notification. Usually this is the desired behavior, as a closed pty slave can be reopened any time - unlike a pipe or socket. However, in some cases pipe-alike behavior might be desired.
After this function was called, slaveFd() and setCTty() cannot be used.
◆ login()
void KPty::login | ( | const char * | user = nullptr, |
const char * | remotehost = nullptr ) |
Creates an utmp entry for the tty.
This function must be called after calling setCTty and making this pty the stdin.
- Parameters
-
user the user to be logged on remotehost the host from which the login is coming. This is not the local host. For remote logins it should be the hostname of the client. For local logins from inside an X session it should be the name of the X display. Otherwise it should be empty.
◆ logout()
◆ masterFd()
int KPty::masterFd | ( | ) | const |
◆ open() [1/2]
bool KPty::open | ( | ) |
◆ open() [2/2]
bool KPty::open | ( | int | fd | ) |
◆ openSlave()
bool KPty::openSlave | ( | ) |
Open the pty slave descriptor.
This undoes the effect of closeSlave().
- Returns
- true if the pty slave was successfully opened
◆ setCTty()
void KPty::setCTty | ( | ) |
◆ setCTtyEnabled()
void KPty::setCTtyEnabled | ( | bool | enable | ) |
Whether this will be a controlling terminal.
This is on by default. Disabling the controllig aspect only makes sense if another process will take over control or there is nothing to control or for technical reasons control cannot be set (this notably is the case with flatpak-spawn when used inside a sandbox).
- Parameters
-
enable whether to enable ctty set up
◆ setEcho()
bool KPty::setEcho | ( | bool | echo | ) |
Set whether the pty should echo input.
Echo is on by default. If the output of automatically fed (non-interactive) PTY clients needs to be parsed, disabling echo often makes it much simpler.
This function can be used only while the PTY is open.
- Parameters
-
echo true if input should be echoed.
- Returns
true
on success, false otherwise
◆ setWinSize() [1/2]
bool KPty::setWinSize | ( | int | lines, |
int | columns ) |
◆ setWinSize() [2/2]
bool KPty::setWinSize | ( | int | lines, |
int | columns, | ||
int | height, | ||
int | width ) |
Change the logical (screen) size of the pty.
The default is 24 lines by 80 columns in characters, and zero pixels.
This function can be used only while the PTY is open.
- Parameters
-
lines the number of character rows columns the number of character columns height the view height in pixels width the view width in pixels
- Returns
true
on success, false otherwise
- Since
- 5.93
◆ slaveFd()
int KPty::slaveFd | ( | ) | const |
◆ tcGetAttr()
bool KPty::tcGetAttr | ( | struct ::termios * | ttmode | ) | const |
Wrapper around tcgetattr(3).
This function can be used only while the PTY is open. You will need an #include <termios.h> to do anything useful with it.
- Parameters
-
ttmode a pointer to a termios structure. Note: when declaring ttmode, struct
::termios
must be used - without the '::' some version of HP-UX thinks, this declares the struct in your class, in your method.
- Returns
true
on success, false otherwise
◆ tcSetAttr()
bool KPty::tcSetAttr | ( | struct ::termios * | ttmode | ) |
◆ ttyName()
const char * KPty::ttyName | ( | ) | const |
Member Data Documentation
◆ d_ptr
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 3 2024 11:45:13 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.