KServiceGroup
#include <KServiceGroup>
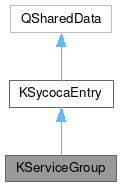
Public Types | |
enum | EntriesOption { NoOptions = 0x0 , SortEntries = 0x1 , ExcludeNoDisplay = 0x2 , AllowSeparators = 0x4 , SortByGenericName = 0x8 } |
typedef QFlags< EntriesOption > | EntriesOptions |
typedef QList< SPtr > | List |
typedef QExplicitlySharedDataPointer< KServiceGroup > | Ptr |
typedef QExplicitlySharedDataPointer< KSycocaEntry > | SPtr |
![]() | |
typedef QList< Ptr > | List |
typedef QExplicitlySharedDataPointer< KSycocaEntry > | Ptr |
Public Member Functions | |
KServiceGroup (const QString &_fullpath, const QString &_relpath) | |
KServiceGroup (const QString &name) | |
bool | allowInline () const |
QString | baseGroupName () const |
QString | caption () const |
int | childCount () const |
QString | comment () const |
QString | directoryEntryPath () const |
List | entries (bool sorted, bool excludeNoDisplay) |
List | entries (bool sorted, bool excludeNoDisplay, bool allowSeparators, bool sortByGenericName=false) |
List | entries (bool sorted=false) |
QList< Ptr > | groupEntries (EntriesOptions options=ExcludeNoDisplay) |
QString | icon () const |
bool | inlineAlias () const |
int | inlineValue () const |
QStringList | layoutInfo () const |
bool | noDisplay () const |
QString | relPath () const |
KService::List | serviceEntries (EntriesOptions options=ExcludeNoDisplay) |
void | setAllowInline (bool _b) |
void | setInlineAlias (bool _b) |
void | setInlineValue (int _val) |
void | setLayoutInfo (const QStringList &layout) |
void | setShowEmptyMenu (bool b) |
void | setShowInlineHeader (bool _b) |
bool | showEmptyMenu () const |
bool | showInlineHeader () const |
QStringList | suppressGenericNames () const |
![]() | |
QString | entryPath () const |
bool | isDeleted () const |
bool | isSeparator () const |
bool | isType (KSycocaType t) const |
bool | isValid () const |
QString | name () const |
void | setDeleted (bool deleted) |
QString | storageId () const |
KSycocaType | sycocaType () const |
![]() | |
QSharedData (const QSharedData &) | |
Static Public Member Functions | |
static Ptr | childGroup (const QString &parent) |
static Ptr | group (const QString &relPath) |
static Ptr | root () |
Protected Member Functions | |
void | addEntry (const KSycocaEntry::Ptr &entry) |
![]() | |
KSERVICE_NO_EXPORT | KSycocaEntry (KSycocaEntryPrivate &d) |
Additional Inherited Members | |
![]() | |
std::unique_ptr< KSycocaEntryPrivate > const | d_ptr |
![]() | |
enum | KSycocaType |
Detailed Description
KServiceGroup represents a group of service, for example screensavers.
This class is typically used like this:
Represents a group of services
Definition at line 52 of file kservicegroup.h.
Member Typedef Documentation
◆ EntriesOptions
typedef QFlags< EntriesOption > KServiceGroup::EntriesOptions |
Stores a combination of EntriesOption values.
Definition at line 210 of file kservicegroup.h.
◆ List
typedef QList<SPtr> KServiceGroup::List |
A list of shared data pointers for KSycocaEntry.
Definition at line 68 of file kservicegroup.h.
◆ Ptr
A shared data pointer for KServiceGroup.
Definition at line 60 of file kservicegroup.h.
◆ SPtr
A shared data pointer for KSycocaEntry.
Definition at line 64 of file kservicegroup.h.
Member Enumeration Documentation
◆ EntriesOption
options for groupEntries and serviceEntries
- See also
- EntriesOptions
Definition at line 200 of file kservicegroup.h.
Constructor & Destructor Documentation
◆ KServiceGroup() [1/2]
KServiceGroup::KServiceGroup | ( | const QString & | name | ) |
Construct a dummy servicegroup indexed with name
.
- Parameters
-
name the name of the service group
Definition at line 19 of file kservicegroup.cpp.
◆ KServiceGroup() [2/2]
Construct a service and take all information from a config file.
- Parameters
-
_fullpath full path to the config file _relpath relative path to the config file
Definition at line 24 of file kservicegroup.cpp.
◆ ~KServiceGroup()
|
override |
Definition at line 77 of file kservicegroup.cpp.
Member Function Documentation
◆ addEntry()
|
protected |
Add a service to this group
Definition at line 238 of file kservicegroup.cpp.
◆ allowInline()
bool KServiceGroup::allowInline | ( | ) | const |
- Returns
- true if we allow to inline menu.
Definition at line 178 of file kservicegroup.cpp.
◆ baseGroupName()
QString KServiceGroup::baseGroupName | ( | ) | const |
Returns a non-empty string if the group is a special base group.
By default, "Settings/" is the kcontrol base group ("settings") and "System/Screensavers/" is the screensavers base group ("screensavers"). This allows moving the groups without breaking those apps.
The base group is defined by the X-KDE-BaseGroup key in the .directory file.
- Returns
- the base group name, or null if no base group
Definition at line 661 of file kservicegroup.cpp.
◆ caption()
QString KServiceGroup::caption | ( | ) | const |
Returns the caption of this group.
- Returns
- the caption of this group
Definition at line 86 of file kservicegroup.cpp.
◆ childCount()
int KServiceGroup::childCount | ( | ) | const |
Returns the total number of displayable services in this group and any of its subgroups.
- Returns
- the number of child services
Definition at line 104 of file kservicegroup.cpp.
◆ childGroup()
|
static |
Returns the group of services that have X-KDE-ParentApp equal to parent
(siblings).
- Parameters
-
parent the name of the service's parent
- Returns
- the services group
Definition at line 655 of file kservicegroup.cpp.
◆ comment()
QString KServiceGroup::comment | ( | ) | const |
Returns the comment about this service group.
- Returns
- the descriptive comment for the group, if there is one, or QString() if not set
Definition at line 98 of file kservicegroup.cpp.
◆ directoryEntryPath()
QString KServiceGroup::directoryEntryPath | ( | ) | const |
Returns a path to the .directory file describing this service group.
The path is either absolute or relative to the "apps" resource.
Definition at line 666 of file kservicegroup.cpp.
◆ entries() [1/3]
KServiceGroup::List KServiceGroup::entries | ( | bool | sorted, |
bool | excludeNoDisplay ) |
Definition at line 315 of file kservicegroup.cpp.
◆ entries() [2/3]
KServiceGroup::List KServiceGroup::entries | ( | bool | sorted, |
bool | excludeNoDisplay, | ||
bool | allowSeparators, | ||
bool | sortByGenericName = false ) |
List of all Services and ServiceGroups within this ServiceGroup.
- Parameters
-
sorted true to sort items excludeNoDisplay true to exclude items marked "NoDisplay" allowSeparators true to allow separator items to be included sortByGenericName true to sort GenericName+Name instead of Name+GenericName
- Returns
- the list of entries
Definition at line 321 of file kservicegroup.cpp.
◆ entries() [3/3]
KServiceGroup::List KServiceGroup::entries | ( | bool | sorted = false | ) |
List of all Services and ServiceGroups within this ServiceGroup.
- Parameters
-
sorted true to sort items
- Returns
- the list of entried
Definition at line 309 of file kservicegroup.cpp.
◆ group()
|
static |
Returns the group with the given relative path.
- Parameters
-
relPath the path of the service group
- Returns
- the group with the given relative path name.
Definition at line 646 of file kservicegroup.cpp.
◆ groupEntries()
QList< KServiceGroup::Ptr > KServiceGroup::groupEntries | ( | EntriesOptions | options = ExcludeNoDisplay | ) |
subgroups for this service group
Definition at line 272 of file kservicegroup.cpp.
◆ icon()
QString KServiceGroup::icon | ( | ) | const |
Returns the name of the icon associated with the group.
- Returns
- the name of the icon associated with the group, or QString() if not set
Definition at line 92 of file kservicegroup.cpp.
◆ inlineAlias()
bool KServiceGroup::inlineAlias | ( | ) | const |
- Returns
- true to show an inline alias item into menu
Definition at line 142 of file kservicegroup.cpp.
◆ inlineValue()
int KServiceGroup::inlineValue | ( | ) | const |
- Returns
- inline limit value
Definition at line 166 of file kservicegroup.cpp.
◆ layoutInfo()
QStringList KServiceGroup::layoutInfo | ( | ) | const |
Returns information related to the layout of services in this group.
Definition at line 634 of file kservicegroup.cpp.
◆ noDisplay()
bool KServiceGroup::noDisplay | ( | ) | const |
Returns true if the NoDisplay flag was set, i.e.
if this group should be hidden from menus, while still being in ksycoca.
- Returns
- true to hide this service group, false to display it
Definition at line 190 of file kservicegroup.cpp.
◆ relPath()
QString KServiceGroup::relPath | ( | ) | const |
Returns the relative path of the service group.
- Returns
- the service group's relative path
Definition at line 81 of file kservicegroup.cpp.
◆ root()
|
static |
Returns the root service group.
- Returns
- the root service group
Definition at line 640 of file kservicegroup.cpp.
◆ serviceEntries()
KService::List KServiceGroup::serviceEntries | ( | EntriesOptions | options = ExcludeNoDisplay | ) |
entries of this service group
Definition at line 291 of file kservicegroup.cpp.
◆ setAllowInline()
void KServiceGroup::setAllowInline | ( | bool | _b | ) |
Definition at line 184 of file kservicegroup.cpp.
◆ setInlineAlias()
void KServiceGroup::setInlineAlias | ( | bool | _b | ) |
Definition at line 148 of file kservicegroup.cpp.
◆ setInlineValue()
void KServiceGroup::setInlineValue | ( | int | _val | ) |
Definition at line 172 of file kservicegroup.cpp.
◆ setLayoutInfo()
void KServiceGroup::setLayoutInfo | ( | const QStringList & | layout | ) |
Sets information related to the layout of services in this group.
Definition at line 628 of file kservicegroup.cpp.
◆ setShowEmptyMenu()
void KServiceGroup::setShowEmptyMenu | ( | bool | b | ) |
Definition at line 154 of file kservicegroup.cpp.
◆ setShowInlineHeader()
void KServiceGroup::setShowInlineHeader | ( | bool | _b | ) |
Definition at line 160 of file kservicegroup.cpp.
◆ showEmptyMenu()
bool KServiceGroup::showEmptyMenu | ( | ) | const |
Return true if we want to display empty menu entry.
- Returns
- true to show this service group as menu entry is empty, false to hide it
Definition at line 136 of file kservicegroup.cpp.
◆ showInlineHeader()
bool KServiceGroup::showInlineHeader | ( | ) | const |
- Returns
- true to show an inline header into menu
Definition at line 130 of file kservicegroup.cpp.
◆ suppressGenericNames()
QStringList KServiceGroup::suppressGenericNames | ( | ) | const |
Returns a list of untranslated generic names that should be be suppressed when showing this group.
E.g. The group "Games/Arcade" might want to suppress the generic name "Arcade Game" since it's redundant in this particular context.
Definition at line 196 of file kservicegroup.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:16:40 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.