KTextEditor::MovingCursor
#include <KTextEditor/MovingCursor>
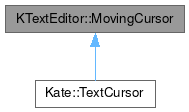
Public Types | |
enum | InsertBehavior { StayOnInsert = 0x0 , MoveOnInsert = 0x1 } |
enum | WrapBehavior { Wrap = 0x0 , NoWrap = 0x1 } |
Public Member Functions | |
MovingCursor (const MovingCursor &)=delete | |
virtual | ~MovingCursor () |
bool | atEndOfDocument () const |
bool | atEndOfLine () const |
bool | atStartOfDocument () const |
bool | atStartOfLine () const |
virtual int | column () const =0 |
virtual Document * | document () const =0 |
bool | gotoNextLine () |
bool | gotoPreviousLine () |
virtual InsertBehavior | insertBehavior () const =0 |
bool | isValid () const |
bool | isValidTextPosition () const |
virtual int | line () const =0 |
bool | move (int chars, WrapBehavior wrapBehavior=Wrap) |
operator Cursor () const | |
MovingCursor & | operator= (const MovingCursor &)=delete |
virtual MovingRange * | range () const =0 |
void | setColumn (int column) |
virtual void | setInsertBehavior (InsertBehavior insertBehavior)=0 |
void | setLine (int line) |
void | setPosition (int line, int column) |
virtual void | setPosition (KTextEditor::Cursor position)=0 |
const Cursor | toCursor () const |
Protected Member Functions | |
MovingCursor () | |
Detailed Description
A Cursor which is bound to a specific Document, and maintains its position.
A MovingCursor is an extension of the basic Cursor class. It maintains its position in the document. As a result of this, MovingCursors may not be copied, as they need to maintain a connection to the associated Document.
Create a new MovingCursor like this:
When finished with a MovingCursor, simply delete it. If the document the cursor belong to is deleted, it will get deleted automatically.
- See also
- Cursor, Range, MovingRange and MovingInterface.
- Since
- 4.5
Definition at line 54 of file movingcursor.h.
Member Enumeration Documentation
◆ InsertBehavior
Insert behavior of this cursor, should it stay if text is insert at its position or should it move.
Enumerator | |
---|---|
StayOnInsert | stay on insert |
MoveOnInsert | move on insert |
Definition at line 64 of file movingcursor.h.
◆ WrapBehavior
Wrap behavior for end of line treatement used in move().
Enumerator | |
---|---|
Wrap | wrap at end of line |
NoWrap | do not wrap at end of line |
Definition at line 72 of file movingcursor.h.
Constructor & Destructor Documentation
◆ ~MovingCursor()
|
virtual |
Destruct the moving cursor.
Definition at line 72 of file movingapi.cpp.
◆ MovingCursor() [1/2]
|
protected |
For inherited class only.
Definition at line 68 of file movingapi.cpp.
◆ MovingCursor() [2/2]
|
delete |
no copy constructor, don't allow this to be copied.
Member Function Documentation
◆ atEndOfDocument()
bool MovingCursor::atEndOfDocument | ( | ) | const |
Determine if this cursor is located at the end of the last line in the document.
- Returns
- true if the cursor is at the end of the document, otherwise false.
Definition at line 104 of file movingapi.cpp.
◆ atEndOfLine()
bool MovingCursor::atEndOfLine | ( | ) | const |
Determine if this cursor is located at the end of the current line.
- Returns
- true if the cursor is situated at the end of the line, otherwise false.
Definition at line 99 of file movingapi.cpp.
◆ atStartOfDocument()
bool MovingCursor::atStartOfDocument | ( | ) | const |
Determine if this cursor is located at line 0 and column 0.
- Returns
- true if the cursor is at start of the document, otherwise false.
Definition at line 109 of file movingapi.cpp.
◆ atStartOfLine()
bool MovingCursor::atStartOfLine | ( | ) | const |
Determine if this cursor is located at column 0 of a valid text line.
- Returns
- true if cursor is a valid text position and column()=0, otherwise false.
Definition at line 94 of file movingapi.cpp.
◆ column()
|
pure virtual |
Retrieve the column on which this cursor is situated.
- Returns
- column number, where 0 is the first column.
Implemented in Kate::TextCursor.
◆ document()
|
pure virtual |
Gets the document to which this cursor is bound.
- Returns
- a pointer to the document
Implemented in Kate::TextCursor.
◆ gotoNextLine()
bool MovingCursor::gotoNextLine | ( | ) |
Moves the cursor to the next line and sets the column to 0.
If the cursor position is already in the last line of the document, the cursor position remains unchanged and the return value is false.
- Returns
- true on success, otherwise false
Definition at line 114 of file movingapi.cpp.
◆ gotoPreviousLine()
bool MovingCursor::gotoPreviousLine | ( | ) |
Moves the cursor to the previous line and sets the column to 0.
If the cursor position is already in line 0, the cursor position remains unchanged and the return value is false.
- Returns
- true on success, otherwise false
Definition at line 126 of file movingapi.cpp.
◆ insertBehavior()
|
pure virtual |
◆ isValid()
|
inline |
Returns whether the current position of this cursor is a valid position, i.e.
whether line() >= 0 and column() >= 0.
- Returns
- true , if the cursor position is valid, otherwise false
Definition at line 159 of file movingcursor.h.
◆ isValidTextPosition()
bool MovingCursor::isValidTextPosition | ( | ) | const |
Check whether this MovingCursor is located at a valid text position.
A cursor position at (line, column) is valid, if
- line >= 0 and line < document()->lines() holds, and
- column >= 0 and column <= lineLength(column).
Further, the text position is also invalid if it is inside a Unicode surrogate (utf-32 character).
- Returns
- true, if the cursor is a valid text position, otherwise false
- See also
- Document::isValidTextPosition()
Definition at line 151 of file movingapi.cpp.
◆ line()
|
pure virtual |
Retrieve the line on which this cursor is situated.
- Returns
- line number, where 0 is the first line.
Implemented in Kate::TextCursor.
◆ move()
bool MovingCursor::move | ( | int | chars, |
WrapBehavior | wrapBehavior = Wrap ) |
Moves the cursor chars
character forward or backwards.
If wrapBehavior equals WrapBehavior::Wrap, the cursor is automatically wrapped to the next line at the end of a line.
When moving backwards, the WrapBehavior does not have any effect.
- Note
- If the cursor could not be moved the amount of chars requested, the cursor is not moved at all!
- Returns
- true on success, otherwise false
Definition at line 138 of file movingapi.cpp.
◆ operator Cursor()
|
inline |
Convert this clever cursor into a dumb one.
Equal to toCursor, allowing to use implicit conversion. Even if this cursor belongs to a range, the created one not.
- Returns
- normal cursor
Definition at line 276 of file movingcursor.h.
◆ operator=()
|
delete |
no assignment operator, no copying around clever cursors.
◆ range()
|
pure virtual |
Get range this cursor belongs to, if any.
- Returns
- range this pointer is part of, else 0
Implemented in Kate::TextCursor.
◆ setColumn()
void MovingCursor::setColumn | ( | int | column | ) |
Set the cursor column to column.
- Parameters
-
column new cursor column
Definition at line 88 of file movingapi.cpp.
◆ setInsertBehavior()
|
pure virtual |
◆ setLine()
void MovingCursor::setLine | ( | int | line | ) |
Set the cursor line to line.
- Parameters
-
line new cursor line
Definition at line 82 of file movingapi.cpp.
◆ setPosition() [1/2]
void MovingCursor::setPosition | ( | int | line, |
int | column ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.Set the cursor position to line and column.
- Parameters
-
line new cursor line column new cursor column
Definition at line 76 of file movingapi.cpp.
◆ setPosition() [2/2]
|
pure virtual |
Set the current cursor position to position.
- Parameters
-
position new cursor position
Implemented in Kate::TextCursor.
◆ toCursor()
|
inline |
Convert this clever cursor into a dumb one.
Even if this cursor belongs to a range, the created one not.
- Returns
- normal cursor
Definition at line 266 of file movingcursor.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 3 2024 11:46:29 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.