KWindowSystem
#include <kwindowsystem.h>
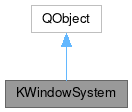
Public Types | |
enum class | Platform { Unknown , X11 , Wayland } |
![]() | |
typedef | QObjectList |
Properties | |
bool | isPlatformWayland |
bool | isPlatformX11 |
bool | showingDesktop |
![]() | |
objectName | |
Signals | |
void | showingDesktopChanged (bool showing) |
Static Public Member Functions | |
static Q_INVOKABLE void | activateWindow (QWindow *window, long time=0) |
static bool | isPlatformWayland () |
static bool | isPlatformX11 () |
static Platform | platform () |
static KWindowSystem * | self () |
static Q_INVOKABLE void | setCurrentXdgActivationToken (const QString &token) |
static void | setMainWindow (QWindow *subwindow, const QString &mainwindow) |
static void | setMainWindow (QWindow *subwindow, WId mainwindow) |
static void | setShowingDesktop (bool showing) |
static bool | showingDesktop () |
static void | updateStartupId (QWindow *window) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Convenience access to certain properties and features of window systems.
Definition at line 26 of file kwindowsystem.h.
Member Enumeration Documentation
◆ Platform
|
strong |
Enum describing the windowing system platform used by the QGuiApplication.
- See also
- platform
- Since
- 5.25
Enumerator | |
---|---|
Unknown | A platform unknown to the application is used. |
X11 | The X11 window system. |
Wayland | The Wayland window system. |
Definition at line 132 of file kwindowsystem.h.
Property Documentation
◆ isPlatformWayland
|
read |
Definition at line 29 of file kwindowsystem.h.
◆ isPlatformX11
|
read |
Definition at line 30 of file kwindowsystem.h.
◆ showingDesktop
|
readwrite |
Whether "show desktop" is currently active.
Definition at line 35 of file kwindowsystem.h.
Member Function Documentation
◆ activateWindow()
|
static |
Requests that window window
is activated.
Applications shouldn't make attempts to explicitly activate their windows, and instead let the user activate them. In the special cases where this may be needed, applications can use activateWindow(). The window manager may consider whether this request wouldn't result in focus stealing, which would be obtrusive, and may refuse the request.
In case of problems, consult KWin's README.md file, or ask on the kwin@.nosp@m.kde..nosp@m.org mailing list.
- Parameters
-
window the window to make active time X server timestamp of the user activity that caused this request
- Since
- 5.89
Definition at line 81 of file kwindowsystem.cpp.
◆ isPlatformWayland()
|
static |
Convenience method to check whether the Platform is Wayland.
- See also
- platform
- isPlatformX11
- Since
- 5.25
◆ isPlatformX11()
|
static |
Convenience method to check whether the Platform is X11.
- See also
- platform
- isPlatformWayland
- Since
- 5.25
◆ platform()
|
static |
Returns the Platform used by the QGuiApplication.
The Platform gets resolved the first time the method is invoked and cached for further usages.
- Returns
- The Platform used by the QGuiApplication.
- Since
- 5.25
Definition at line 150 of file kwindowsystem.cpp.
◆ self()
|
static |
Access to the singleton instance.
Useful mainly for connecting to signals.
Definition at line 71 of file kwindowsystem.cpp.
◆ setCurrentXdgActivationToken()
|
static |
Sets the token
that will be used when activateWindow is called next.
- Since
- 5.83
Definition at line 190 of file kwindowsystem.cpp.
◆ setMainWindow() [1/2]
Sets the parent window of subwindow
to be mainwindow
.
This function should be used before a dialog is shown for a window that belongs to another application.
- Parameters
-
window the sub window mainwindow The main window ID or XDG Foreign token
- Since
- 6.0
Definition at line 98 of file kwindowsystem.cpp.
◆ setMainWindow() [2/2]
|
static |
Sets the parent window of subwindow
to be mainwindow
.
This overrides the parent set the usual way as the QWidget or QWindow parent, but only for the window manager - e.g. stacking order and window grouping will be affected, but features like automatic deletion of children when the parent is deleted are unaffected and normally use the QObject parent.
This function should be used before a dialog is shown for a window that belongs to another application.
On Wayland, use the QString overload to provide an XDG Foreign token.
Definition at line 87 of file kwindowsystem.cpp.
◆ setShowingDesktop()
|
static |
Sets the state of the "showing desktop" mode of the window manager.
If on, windows are hidden and desktop background is shown and focused.
- Parameters
-
showing if true, the window manager is put in "showing desktop" mode. If false, the window manager is put out of that mode.
- Since
- 5.7.0
Definition at line 123 of file kwindowsystem.cpp.
◆ showingDesktop()
|
static |
Returns the state of showing the desktop.
◆ showingDesktopChanged
|
signal |
The state of showing the desktop has changed.
◆ updateStartupId()
|
static |
Updates the platform-specific startup id, if any.
This method is to be called when a running application instance is reused for handling the request to start this application. A typical use would be in the handler of the KDBusService activation signal.
For X11, this updates the id for the Startup Notification protocol, taking the id from QX11Info::nextStartupId(), if not empty. For Wayland, this updates the token for the XDG Activation protocol, taking the token from the "XDG_ACTIVATION_TOKEN" environment variable and then unsetting it, if not empty.
- Parameters
-
window the main window (needed by X11 platform)
- Since
- 5.91
Definition at line 166 of file kwindowsystem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:58:55 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.