NETWinInfo
#include <netwm.h>
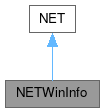
Public Types | |
enum | { PROTOCOLS , PROTOCOLS2 , PROPERTIES_SIZE } |
![]() | |
enum | { OnAllDesktops = -1 } |
enum | Action { ActionMove = 1u << 0 , ActionResize = 1u << 1 , ActionMinimize = 1u << 2 , ActionShade = 1u << 3 , ActionStick = 1u << 4 , ActionMaxVert = 1u << 5 , ActionMaxHoriz = 1u << 6 , ActionMax = ActionMaxVert | ActionMaxHoriz , ActionFullScreen = 1u << 7 , ActionChangeDesktop = 1u << 8 , ActionClose = 1u << 9 } |
typedef QFlags< Action > | Actions |
enum | DesktopLayoutCorner { DesktopLayoutCornerTopLeft = 0 , DesktopLayoutCornerTopRight = 1 , DesktopLayoutCornerBottomLeft = 2 , DesktopLayoutCornerBottomRight = 3 } |
enum | Direction { TopLeft = 0 , Top = 1 , TopRight = 2 , Right = 3 , BottomRight = 4 , Bottom = 5 , BottomLeft = 6 , Left = 7 , Move = 8 , KeyboardSize = 9 , KeyboardMove = 10 , MoveResizeCancel = 11 } |
enum | MappingState { Visible = 1 , Withdrawn = 0 , Iconic = 3 } |
enum | Orientation { OrientationHorizontal = 0 , OrientationVertical = 1 } |
typedef QFlags< Property > | Properties |
typedef QFlags< Property2 > | Properties2 |
enum | Property { Supported = 1u << 0 , ClientList = 1u << 1 , ClientListStacking = 1u << 2 , NumberOfDesktops = 1u << 3 , DesktopGeometry = 1u << 4 , DesktopViewport = 1u << 5 , CurrentDesktop = 1u << 6 , DesktopNames = 1u << 7 , ActiveWindow = 1u << 8 , WorkArea = 1u << 9 , SupportingWMCheck = 1u << 10 , VirtualRoots = 1u << 11 , CloseWindow = 1u << 13 , WMMoveResize = 1u << 14 , WMName = 1u << 15 , WMVisibleName = 1u << 16 , WMDesktop = 1u << 17 , WMWindowType = 1u << 18 , WMState = 1u << 19 , WMStrut = 1u << 20 , WMIconGeometry = 1u << 21 , WMIcon = 1u << 22 , WMPid = 1u << 23 , WMHandledIcons = 1u << 24 , WMPing = 1u << 25 , XAWMState = 1u << 27 , WMFrameExtents = 1u << 28 , WMIconName = 1u << 29 , WMVisibleIconName = 1u << 30 , WMGeometry = 1u << 31 , WMAllProperties = ~0u } |
enum | Property2 { WM2UserTime = 1u << 0 , WM2StartupId = 1u << 1 , WM2TransientFor = 1u << 2 , WM2GroupLeader = 1u << 3 , WM2AllowedActions = 1u << 4 , WM2RestackWindow = 1u << 5 , WM2MoveResizeWindow = 1u << 6 , WM2ExtendedStrut = 1u << 7 , WM2KDETemporaryRules = 1u << 8 , WM2WindowClass = 1u << 9 , WM2WindowRole = 1u << 10 , WM2ClientMachine = 1u << 11 , WM2ShowingDesktop = 1u << 12 , WM2Opacity = 1u << 13 , WM2DesktopLayout = 1u << 14 , WM2FullPlacement = 1u << 15 , WM2FullscreenMonitors = 1u << 16 , WM2FrameOverlap = 1u << 17 , WM2Activities = 1u << 18 , WM2BlockCompositing = 1u << 19 , WM2KDEShadow = 1u << 20 , WM2Urgency = 1u << 21 , WM2Input = 1u << 22 , WM2Protocols = 1u << 23 , WM2InitialMappingState = 1u << 24 , WM2IconPixmap = 1u << 25 , WM2OpaqueRegion = 1u << 25 , WM2DesktopFileName = 1u << 26 , WM2GTKFrameExtents = 1u << 27 , WM2AppMenuServiceName = 1u << 28 , WM2AppMenuObjectPath = 1u << 29 , WM2GTKApplicationId = 1u << 30 , WM2GTKShowWindowMenu = 1u << 31 , WM2AllProperties = ~0u } |
enum | Protocol { NoProtocol = 0 , TakeFocusProtocol = 1 << 0 , DeleteWindowProtocol = 1 << 1 , PingProtocol = 1 << 2 , SyncRequestProtocol = 1 << 3 , ContextHelpProtocol = 1 << 4 } |
typedef QFlags< Protocol > | Protocols |
enum | RequestSource { FromUnknown = 0 , FromApplication = 1 , FromTool = 2 } |
enum | Role { Client , WindowManager } |
enum | State { Modal = 1u << 0 , Sticky = 1u << 1 , MaxVert = 1u << 2 , MaxHoriz = 1u << 3 , Max = MaxVert | MaxHoriz , Shaded = 1u << 4 , SkipTaskbar = 1u << 5 , KeepAbove = 1u << 6 , SkipPager = 1u << 7 , Hidden = 1u << 8 , FullScreen = 1u << 9 , KeepBelow = 1u << 10 , DemandsAttention = 1u << 11 , SkipSwitcher = 1u << 12 , Focused = 1u << 13 } |
typedef QFlags< State > | States |
enum | WindowType { Unknown = -1 , Normal = 0 , Desktop = 1 , Dock = 2 , Toolbar = 3 , Menu = 4 , Dialog = 5 , Override = 6 , TopMenu = 7 , Utility = 8 , Splash = 9 , DropdownMenu = 10 , PopupMenu = 11 , Tooltip = 12 , Notification = 13 , ComboBox = 14 , DNDIcon = 15 , OnScreenDisplay = 16 , CriticalNotification = 17 , AppletPopup = 18 } |
enum | WindowTypeMask { NormalMask = 1u << 0 , DesktopMask = 1u << 1 , DockMask = 1u << 2 , ToolbarMask = 1u << 3 , MenuMask = 1u << 4 , DialogMask = 1u << 5 , OverrideMask = 1u << 6 , TopMenuMask = 1u << 7 , UtilityMask = 1u << 8 , SplashMask = 1u << 9 , DropdownMenuMask = 1u << 10 , PopupMenuMask = 1u << 11 , TooltipMask = 1u << 12 , NotificationMask = 1u << 13 , ComboBoxMask = 1u << 14 , DNDIconMask = 1u << 15 , OnScreenDisplayMask = 1u << 16 , CriticalNotificationMask = 1u << 17 , AppletPopupMask = 1u << 18 , AllTypesMask = 0U - 1 } |
typedef QFlags< WindowTypeMask > | WindowTypes |
Public Member Functions | |
NETWinInfo (const NETWinInfo &wininfo) | |
NETWinInfo (xcb_connection_t *connection, xcb_window_t window, xcb_window_t rootWindow, NET::Properties properties, NET::Properties2 properties2, Role role=Client) | |
virtual | ~NETWinInfo () |
const char * | activities () const |
NET::Actions | allowedActions () const |
const char * | appMenuObjectPath () const |
const char * | appMenuServiceName () const |
const char * | clientMachine () const |
int | desktop (bool ignore_viewport=false) const |
const char * | desktopFileName () const |
NET::Properties | event (xcb_generic_event_t *event) |
void | event (xcb_generic_event_t *event, NET::Properties *properties, NET::Properties2 *properties2=nullptr) |
NETExtendedStrut | extendedStrut () const |
NETStrut | frameExtents () const |
NETStrut | frameOverlap () const |
NETFullscreenMonitors | fullscreenMonitors () const |
xcb_window_t | groupLeader () const |
const char * | gtkApplicationId () const |
NETStrut | gtkFrameExtents () const |
bool | handledIcons () const |
bool | hasNETSupport () const |
bool | hasWindowType () const |
xcb_pixmap_t | icccmIconPixmap () const |
xcb_pixmap_t | icccmIconPixmapMask () const |
NETIcon | icon (int width=-1, int height=-1) const |
NETRect | iconGeometry () const |
const char * | iconName () const |
const int * | iconSizes () const |
MappingState | initialMappingState () const |
bool | input () const |
bool | isBlockingCompositing () const |
void | kdeGeometry (NETRect &frame, NETRect &window) |
MappingState | mappingState () const |
const char * | name () const |
unsigned long | opacity () const |
qreal | opacityF () const |
std::vector< NETRect > | opaqueRegion () const |
const NETWinInfo & | operator= (const NETWinInfo &wintinfo) |
NET::Properties | passedProperties () const |
NET::Properties2 | passedProperties2 () const |
int | pid () const |
NET::Protocols | protocols () const |
void | setActivities (const char *activities) |
void | setAllowedActions (NET::Actions actions) |
void | setAppMenuObjectPath (const char *path) |
void | setAppMenuServiceName (const char *name) |
void | setBlockingCompositing (bool active) |
void | setDesktop (int desktop, bool ignore_viewport=false) |
void | setDesktopFileName (const char *name) |
void | setExtendedStrut (const NETExtendedStrut &extended_strut) |
void | setFrameExtents (NETStrut strut) |
void | setFrameOverlap (NETStrut strut) |
void | setFullscreenMonitors (NETFullscreenMonitors topology) |
void | setGtkFrameExtents (NETStrut strut) |
void | setHandledIcons (bool handled) |
void | setIcon (NETIcon icon, bool replace=true) |
void | setIconGeometry (NETRect geometry) |
void | setIconName (const char *name) |
void | setName (const char *name) |
void | setOpacity (unsigned long opacity) |
void | setOpacityF (qreal opacity) |
void | setPid (int pid) |
void | setStartupId (const char *startup_id) |
void | setState (NET::States state, NET::States mask) |
void | setStrut (NETStrut strut) |
void | setUserTime (xcb_timestamp_t time) |
void | setVisibleIconName (const char *name) |
void | setVisibleName (const char *visibleName) |
void | setWindowType (WindowType type) |
const char * | startupId () const |
NET::States | state () const |
NETStrut | strut () const |
bool | supportsProtocol (NET::Protocol protocol) const |
xcb_window_t | transientFor () const |
bool | urgency () const |
xcb_timestamp_t | userTime () const |
const char * | visibleIconName () const |
const char * | visibleName () const |
const char * | windowClassClass () const |
const char * | windowClassName () const |
const char * | windowRole () const |
WindowType | windowType (WindowTypes supported_types) const |
xcb_connection_t * | xcbConnection () const |
Static Public Attributes | |
static const int | OnAllDesktops = NET::OnAllDesktops |
Protected Member Functions | |
virtual void | changeDesktop (int desktop) |
virtual void | changeFullscreenMonitors (NETFullscreenMonitors topology) |
virtual void | changeState (NET::States state, NET::States mask) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
static int | timestampCompare (unsigned long time1, unsigned long time2) |
static int | timestampDiff (unsigned long time1, unsigned long time2) |
static bool | typeMatchesMask (WindowType type, WindowTypes mask) |
Detailed Description
Common API for application window properties/protocols.
The NETWinInfo class provides a common API for clients and window managers to set/read/change properties on an application window as defined by the NET Window Manager Specification.
Member Enumeration Documentation
◆ anonymous enum
Constructor & Destructor Documentation
◆ NETWinInfo() [1/2]
NETWinInfo::NETWinInfo | ( | xcb_connection_t * | connection, |
xcb_window_t | window, | ||
xcb_window_t | rootWindow, | ||
NET::Properties | properties, | ||
NET::Properties2 | properties2, | ||
Role | role = Client ) |
Create a NETWinInfo object, which will be used to set/read/change information stored on an application window.
- Parameters
-
connection XCB connection window The Window id of the application window. rootWindow The Window id of the root window. properties The NET::Properties flags properties2 The NET::Properties2 flags role Select the application role. If this argument is omitted, the role will default to Client.
◆ NETWinInfo() [2/2]
NETWinInfo::NETWinInfo | ( | const NETWinInfo & | wininfo | ) |
Creates a shared copy of the specified NETWinInfo object.
- Parameters
-
wininfo the NETWinInfo to copy
◆ ~NETWinInfo()
|
virtual |
Destroys the NETWinInfo object.
Member Function Documentation
◆ activities()
const char * NETWinInfo::activities | ( | ) | const |
◆ allowedActions()
NET::Actions NETWinInfo::allowedActions | ( | ) | const |
◆ appMenuObjectPath()
const char * NETWinInfo::appMenuObjectPath | ( | ) | const |
◆ appMenuServiceName()
const char * NETWinInfo::appMenuServiceName | ( | ) | const |
◆ changeDesktop()
|
inlineprotectedvirtual |
A Window Manager should subclass NETWinInfo and reimplement this function when it wants to know when a Client made a request to change desktops (ie.
move to another desktop).
- Parameters
-
desktop the number of the desktop
◆ changeFullscreenMonitors()
|
inlineprotectedvirtual |
◆ changeState()
|
inlineprotectedvirtual |
A Window Manager should subclass NETWinInfo and reimplement this function when it wants to know when a Client made a request to change state (ie.
to Shade / Unshade).
- Parameters
-
state the new state mask the mask for the state
◆ clientMachine()
const char * NETWinInfo::clientMachine | ( | ) | const |
◆ desktop()
int NETWinInfo::desktop | ( | bool | ignore_viewport = false | ) | const |
Returns the desktop where the window is residing.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. It is however mapped to virtual desktops if needed.
- Parameters
-
ignore_viewport if false, viewport is mapped to virtual desktops
- Returns
- the number of the window's desktop
- See also
- OnAllDesktops()
◆ desktopFileName()
const char * NETWinInfo::desktopFileName | ( | ) | const |
- Returns
- The desktop file name of the window's application if present.
- Since
- 5.28
- See also
- setDesktopFileName
◆ event() [1/2]
NET::Properties NETWinInfo::event | ( | xcb_generic_event_t * | event | ) |
This function takes the pass XEvent and returns an OR'ed list of NETWinInfo properties that have changed.
The new information will be read immediately by the class. This overloaded version returns only a single mask, and therefore cannot check state of all properties like the other variant.
- Parameters
-
event the event
- Returns
- the properties
◆ event() [2/2]
void NETWinInfo::event | ( | xcb_generic_event_t * | event, |
NET::Properties * | properties, | ||
NET::Properties2 * | properties2 = nullptr ) |
This function takes the passed in xcb_generic_event_t and returns the updated properties in the passed in arguments.
The new information will be read immediately by the class. It is possible to pass in a null pointer in the arguments. In that case the passed in argument will obviously not be updated, but the class will process the information nevertheless.
- Parameters
-
event the event properties The NET::Properties that changed properties2 The NET::Properties2 that changed
- Since
- 5.0
◆ extendedStrut()
NETExtendedStrut NETWinInfo::extendedStrut | ( | ) | const |
◆ frameExtents()
NETStrut NETWinInfo::frameExtents | ( | ) | const |
◆ frameOverlap()
NETStrut NETWinInfo::frameOverlap | ( | ) | const |
◆ fullscreenMonitors()
NETFullscreenMonitors NETWinInfo::fullscreenMonitors | ( | ) | const |
◆ groupLeader()
xcb_window_t NETWinInfo::groupLeader | ( | ) | const |
◆ gtkApplicationId()
const char * NETWinInfo::gtkApplicationId | ( | ) | const |
◆ gtkFrameExtents()
NETStrut NETWinInfo::gtkFrameExtents | ( | ) | const |
◆ handledIcons()
bool NETWinInfo::handledIcons | ( | ) | const |
◆ hasNETSupport()
bool NETWinInfo::hasNETSupport | ( | ) | const |
Returns true if the window has any window type set, even if the type itself is not known to this implementation.
Presence of a window type as specified by the NETWM spec is considered as the window supporting this specification.
- Returns
- true if the window has support for the NETWM spec
◆ hasWindowType()
bool NETWinInfo::hasWindowType | ( | ) | const |
This function returns false if the window has not window type specified at all.
Used by KWindowInfo::windowType() to return either NET::Normal or NET::Dialog as appropriate as a fallback.
◆ icccmIconPixmap()
xcb_pixmap_t NETWinInfo::icccmIconPixmap | ( | ) | const |
Returns the icon pixmap as set in WM_HINTS.
See ICCCM 4.1.2.4.
The default value is XCB_PIXMAP_NONE
.
Using the ICCCM variant for the icon is deprecated and only offers a limited functionality compared to icon
. Only use this variant as a fallback.
- See also
- icccmIconPixmapMask
- icon
- Since
- 5.7
◆ icccmIconPixmapMask()
xcb_pixmap_t NETWinInfo::icccmIconPixmapMask | ( | ) | const |
Returns the mask for the icon pixmap as set in WM_HINTS.
See ICCCM 4.1.2.4.
The default value is XCB_PIXMAP_NONE
.
- See also
- icccmIconPixmap
- Since
- 5.7
◆ icon()
NETIcon NETWinInfo::icon | ( | int | width = -1, |
int | height = -1 ) const |
Returns an icon.
If width and height are passed, the icon returned will be the closest it can find (the next biggest). If width and height are omitted, then the largest icon in the list is returned.
- Parameters
-
width the preferred width for the icon, -1 to ignore height the preferred height for the icon, -1 to ignore
- Returns
- the icon
◆ iconGeometry()
NETRect NETWinInfo::iconGeometry | ( | ) | const |
◆ iconName()
const char * NETWinInfo::iconName | ( | ) | const |
Returns the iconic name of the window in UTF-8 format.
Note that this has nothing to do with icons, but it's for "iconic" representations of the window (taskbars etc.), that should be shown when the window is in iconic state. See description of _NET_WM_ICON_NAME for details.
- Returns
- the iconic name
◆ iconSizes()
const int * NETWinInfo::iconSizes | ( | ) | const |
◆ initialMappingState()
NET::MappingState NETWinInfo::initialMappingState | ( | ) | const |
◆ input()
bool NETWinInfo::input | ( | ) | const |
◆ isBlockingCompositing()
bool NETWinInfo::isBlockingCompositing | ( | ) | const |
◆ kdeGeometry()
◆ mappingState()
NET::MappingState NETWinInfo::mappingState | ( | ) | const |
◆ name()
const char * NETWinInfo::name | ( | ) | const |
◆ opacity()
unsigned long NETWinInfo::opacity | ( | ) | const |
◆ opacityF()
qreal NETWinInfo::opacityF | ( | ) | const |
◆ opaqueRegion()
std::vector< NETRect > NETWinInfo::opaqueRegion | ( | ) | const |
◆ operator=()
const NETWinInfo & NETWinInfo::operator= | ( | const NETWinInfo & | wintinfo | ) |
◆ passedProperties()
NET::Properties NETWinInfo::passedProperties | ( | ) | const |
- Returns
- the properties argument passed to the constructor.
- See also
- passedProperties2()
◆ passedProperties2()
NET::Properties2 NETWinInfo::passedProperties2 | ( | ) | const |
◆ pid()
int NETWinInfo::pid | ( | ) | const |
◆ protocols()
NET::Protocols NETWinInfo::protocols | ( | ) | const |
◆ setActivities()
void NETWinInfo::setActivities | ( | const char * | activities | ) |
◆ setAllowedActions()
void NETWinInfo::setAllowedActions | ( | NET::Actions | actions | ) |
◆ setAppMenuObjectPath()
void NETWinInfo::setAppMenuObjectPath | ( | const char * | path | ) |
◆ setAppMenuServiceName()
void NETWinInfo::setAppMenuServiceName | ( | const char * | name | ) |
◆ setBlockingCompositing()
void NETWinInfo::setBlockingCompositing | ( | bool | active | ) |
◆ setDesktop()
void NETWinInfo::setDesktop | ( | int | desktop, |
bool | ignore_viewport = false ) |
Set which window the desktop is (should be) on.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. It is however mapped to virtual desktops if needed.
- Parameters
-
desktop the number of the new desktop ignore_viewport if false, viewport is mapped to virtual desktops
- See also
- OnAllDesktops()
◆ setDesktopFileName()
void NETWinInfo::setDesktopFileName | ( | const char * | name | ) |
Sets the name
as the desktop file name.
This is either the base name without full path and without file extension of the desktop file for the window's application (e.g. "org.kde.foo").
If the application's desktop file name is not at a standard location it should be the full path to the desktop file name (e.g. "/opt/kde/share/org.kde.foo.desktop").
If the window does not know the desktop file name, it should not set the name at all.
- Since
- 5.28
◆ setExtendedStrut()
void NETWinInfo::setExtendedStrut | ( | const NETExtendedStrut & | extended_strut | ) |
◆ setFrameExtents()
void NETWinInfo::setFrameExtents | ( | NETStrut | strut | ) |
◆ setFrameOverlap()
void NETWinInfo::setFrameOverlap | ( | NETStrut | strut | ) |
◆ setFullscreenMonitors()
void NETWinInfo::setFullscreenMonitors | ( | NETFullscreenMonitors | topology | ) |
Sets the desired multiple-monitor topology (4 monitor indices indicating the top, bottom, left, and right edges of the window) when the fullscreen state is enabled.
The indices are from the set returned by the Xinerama extension. See _NET_WM_FULLSCREEN_MONITORS for details.
- Parameters
-
topology A struct that models the desired monitor topology, namely: top is the monitor whose top edge defines the top edge of the fullscreen window, bottom is the monitor whose bottom edge defines the bottom edge of the fullscreen window, left is the monitor whose left edge defines the left edge of the fullscreen window, and right is the monitor whose right edge defines the right edge of the fullscreen window.
◆ setGtkFrameExtents()
void NETWinInfo::setGtkFrameExtents | ( | NETStrut | strut | ) |
◆ setHandledIcons()
void NETWinInfo::setHandledIcons | ( | bool | handled | ) |
◆ setIcon()
void NETWinInfo::setIcon | ( | NETIcon | icon, |
bool | replace = true ) |
Set icons for the application window.
If replace is True, then the specified icon is defined to be the only icon. If replace is False, then the specified icon is added to a list of icons.
- Parameters
-
icon the new icon replace true to replace, false to append to the list of icons
◆ setIconGeometry()
void NETWinInfo::setIconGeometry | ( | NETRect | geometry | ) |
◆ setIconName()
void NETWinInfo::setIconName | ( | const char * | name | ) |
◆ setName()
void NETWinInfo::setName | ( | const char * | name | ) |
◆ setOpacity()
void NETWinInfo::setOpacity | ( | unsigned long | opacity | ) |
◆ setOpacityF()
void NETWinInfo::setOpacityF | ( | qreal | opacity | ) |
◆ setPid()
void NETWinInfo::setPid | ( | int | pid | ) |
◆ setStartupId()
void NETWinInfo::setStartupId | ( | const char * | startup_id | ) |
◆ setState()
void NETWinInfo::setState | ( | NET::States | state, |
NET::States | mask ) |
◆ setStrut()
void NETWinInfo::setStrut | ( | NETStrut | strut | ) |
- Deprecated
- use setExtendedStrut() Set the strut for the application window.
- Parameters
-
strut the new strut
◆ setUserTime()
void NETWinInfo::setUserTime | ( | xcb_timestamp_t | time | ) |
Sets user timestamp time
on the window (property _NET_WM_USER_TIME).
The timestamp is expressed as XServer time. If a window is shown with user timestamp older than the time of the last user action, it won't be activated after being shown, with the special value 0 meaning not to activate the window after being shown.
◆ setVisibleIconName()
void NETWinInfo::setVisibleIconName | ( | const char * | name | ) |
◆ setVisibleName()
void NETWinInfo::setVisibleName | ( | const char * | visibleName | ) |
◆ setWindowType()
void NETWinInfo::setWindowType | ( | WindowType | type | ) |
◆ startupId()
const char * NETWinInfo::startupId | ( | ) | const |
◆ state()
NET::States NETWinInfo::state | ( | ) | const |
◆ strut()
NETStrut NETWinInfo::strut | ( | ) | const |
- Deprecated
- use strutPartial() Returns the strut specified by this client.
- Returns
- the strut of the window
◆ supportsProtocol()
bool NETWinInfo::supportsProtocol | ( | NET::Protocol | protocol | ) | const |
◆ transientFor()
xcb_window_t NETWinInfo::transientFor | ( | ) | const |
◆ urgency()
bool NETWinInfo::urgency | ( | ) | const |
◆ userTime()
xcb_timestamp_t NETWinInfo::userTime | ( | ) | const |
◆ virtual_hook()
|
protectedvirtual |
◆ visibleIconName()
const char * NETWinInfo::visibleIconName | ( | ) | const |
Returns the visible iconic name as set by the window manager in UTF-8 format.
Note that this has nothing to do with icons, but it's for "iconic" representations of the window (taskbars etc.), that should be shown when the window is in iconic state. See description of _NET_WM_VISIBLE_ICON_NAME for details.
- Returns
- the visible iconic name
◆ visibleName()
const char * NETWinInfo::visibleName | ( | ) | const |
◆ windowClassClass()
const char * NETWinInfo::windowClassClass | ( | ) | const |
◆ windowClassName()
const char * NETWinInfo::windowClassName | ( | ) | const |
◆ windowRole()
const char * NETWinInfo::windowRole | ( | ) | const |
◆ windowType()
NET::WindowType NETWinInfo::windowType | ( | WindowTypes | supported_types | ) | const |
Returns the window type for this client (see the NET base class documentation for a description of the various window types).
Since clients may specify several windows types for a window in order to support backwards compatibility and extensions not available in the NETWM spec, you should specify all window types you application supports (see the NET::WindowTypeMask mask values for various window types). This method will return the first window type that is listed in the supported types, or NET::Unknown if none of the window types is supported.
- Returns
- the type of the window
◆ xcbConnection()
xcb_connection_t * NETWinInfo::xcbConnection | ( | ) | const |
Member Data Documentation
◆ OnAllDesktops
|
static |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:51:07 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.