KToolTipHelper
#include <KToolTipHelper>
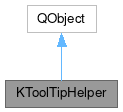
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An event filter used to enhance tooltips.
Example: Without this class, a tooltip of a QToolButton of a "New" action will read something like "New File". Using this class, the tooltip can be enhanced to read something like "New File (Ctrl+N)" and in the next line smaller "Press Shift for help.". Pressing Shift will open the "What's This" context help for that widget. If a hyperlink in that help is clicked, the corresponding event will also be filtered by this class and open the linked location.
The extra text added to tooltips is only shown when available and where it makes sense. If a QToolButton has no associated shortcut and an empty QWidget::whatsThis(), this class won't tamper with the requested tooltip at all.
This class also activates tooltips for actions in QMenus but only when it makes sense like when the tooltip isn't equal to the already displayed text.
If you want the "Press Shift for help." line to be displayed for a widget that has whatsThis() but no toolTip() take a look at KToolTipHelper::whatsThisHintOnly().
The enhanced tooltips can be enabled at any time after the QApplication was constructed with
Therefore, to de-activate them you can call
any time later.
If you want KToolTipHelper to not tamper with certain QEvents, e.g. you want to handle some tooltips differently or you want to change what happens when a QWhatsThisClickedEvent is processed, first remove KToolTipHelper as an event filter just like in the line of code above. Then create your own custom EventFilter that handles those QEvents differently and for all cases that you don't want to handle differently call
KMainWindow will have this EventFilter installed by default from framework version 5.84 onward so if you want to opt out of that, remove the EventFilter in the constructor of your MainWindow class inheriting from KMainWindow.
- See also
- QToolTip
- Since
- 5.84
Definition at line 68 of file ktooltiphelper.h.
Member Function Documentation
◆ eventFilter()
Filters QEvent::ToolTip if an enhanced tooltip is available for the widget.
Filters the QEvent::KeyPress that is used to expand an expandable tooltip. Filters QEvent::WhatsThisClicked so hyperlinks in whatsThis() texts work.
- See also
- QObject::eventFilter()
- QHelpEvent
Reimplemented from QObject.
Definition at line 58 of file ktooltiphelper.cpp.
◆ instance()
|
static |
Definition at line 28 of file ktooltiphelper.cpp.
◆ whatsThisHintOnly()
Use this to have a widget show "Press Shift for help." as its tooltip.
KToolTipHelper won't show that tooltip if the widget's whatsThis() is empty.
- Returns
- a QString that is interpreted by this class to show the expected tooltip.
Definition at line 79 of file ktooltiphelper.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 3 2024 11:52:03 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.