KSyntaxHighlighting::AbstractHighlighter
#include <abstracthighlighter.h>
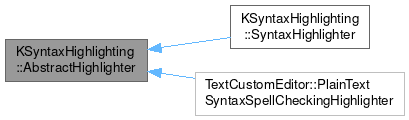
Public Member Functions | |
Definition | definition () const |
virtual void | setDefinition (const Definition &def) |
virtual void | setTheme (const Theme &theme) |
Theme | theme () const |
Protected Member Functions | |
KSYNTAXHIGHLIGHTING_NO_EXPORT | AbstractHighlighter (AbstractHighlighterPrivate *dd) |
virtual void | applyFolding (int offset, int length, FoldingRegion region) |
virtual void | applyFormat (int offset, int length, const Format &format)=0 |
State | highlightLine (QStringView text, const State &state) |
Protected Attributes | |
AbstractHighlighterPrivate * | d_ptr |
Detailed Description
Abstract base class for highlighters.
Introduction
The AbstractHighlighter provides an interface to highlight text.
The SyntaxHighlighting framework already ships with one implementation, namely the SyntaxHighlighter, which also derives from QSyntaxHighlighter, meaning that it can be used to highlight a QTextDocument or a QML TextEdit. In order to use the SyntaxHighlighter, just call setDefinition() and setTheme(), and the associated documents will automatically be highlighted.
However, if you want to use the SyntaxHighlighting framework to implement your own syntax highlighter, you need to sublcass from AbstractHighlighter.
Implementing your own Syntax Highlighter
In order to implement your own syntax highlighter, you need to inherit from AbstractHighlighter. Then, pass each text line that needs to be highlighted in order to highlightLine(). Internally, highlightLine() uses the Definition initially set through setDefinition() and the State of the previous text line to parse and highlight the given text line. For each visual highlighting change, highlightLine() will call applyFormat(). Therefore, reimplement applyFormat() to get notified of the Format that is valid in the range starting at the given offset with the specified length. Similarly, for each text part that starts or ends a code folding region, highlightLine() will call applyFolding(). Therefore, if you are interested in code folding, reimplement applyFolding() to get notified of the starting and ending code folding regions, again specified in the range starting at the given offset with the given length.
The Format class itself depends on the current Theme. A theme must be initially set once such that the Formats instances can be queried for concrete colors.
Optionally, you can also reimplement setTheme() and setDefinition() to get notified whenever the Definition or the Theme changes.
- See also
- SyntaxHighlighter
- Since
- 5.28
Definition at line 66 of file abstracthighlighter.h.
Constructor & Destructor Documentation
◆ ~AbstractHighlighter()
|
virtual |
Definition at line 59 of file abstracthighlighter.cpp.
◆ AbstractHighlighter() [1/2]
|
protected |
Definition at line 49 of file abstracthighlighter.cpp.
◆ AbstractHighlighter() [2/2]
|
explicitprotected |
Definition at line 54 of file abstracthighlighter.cpp.
Member Function Documentation
◆ applyFolding()
|
protectedvirtual |
Reimplement this to apply folding to your output.
The provided FoldingRegion region
either stars or ends a code folding region in the interval [offset
, offset
+ length
).
- Parameters
-
offset The start column of the FoldingRegion length The length of the matching text that starts / ends a folding region region The FoldingRegion that applies to the range [offset, offset + length)
- Note
- The FoldingRegion
region
is always either of type FoldingRegion::Type::Begin or FoldingRegion::Type::End.
- See also
- applyFormat(), highlightLine(), FoldingRegion
Reimplemented in KSyntaxHighlighting::SyntaxHighlighter.
Definition at line 433 of file abstracthighlighter.cpp.
◆ applyFormat()
|
protectedpure virtual |
Reimplement this to apply formats to your output.
The provided format
is valid for the interval [offset
, offset
+ length
).
- Parameters
-
offset The start column of the interval for which format
matcheslength The length of the matching text format The Format that applies to the range [offset, offset + length)
- Note
- Make sure to set a valid Definition, otherwise the parameter
format
is invalid for the entire line passed to highlightLine() (cf. Format::isValid()).
- See also
- applyFolding(), highlightLine()
Implemented in KSyntaxHighlighting::SyntaxHighlighter.
◆ definition()
Definition AbstractHighlighter::definition | ( | ) | const |
Returns the syntax definition used for highlighting.
- See also
- setDefinition()
Definition at line 64 of file abstracthighlighter.cpp.
◆ highlightLine()
|
protected |
Highlight the given line.
Call this from your derived class where appropriate. This will result in any number of applyFormat() and applyFolding() calls as a result.
- Parameters
-
text A string containing the text of the line to highlight. state The highlighting state handle returned by the call to highlightLine() for the previous line. For the very first line, just pass a default constructed State().
- Returns
- The state of the highlighting engine after processing the given line. This needs to passed into highlightLine() for the next line. You can store the state for efficient partial re-highlighting for example during editing.
- See also
- applyFormat(), applyFolding()
handle line empty context switches guard against endless loops see https://phabricator.kde.org/D18509
line empty context switches
end when trying to #pop the main context
for expensive rules like regexes we do:
- match them for the complete line, as this is faster than re-trying them at all positions
- store the result of the first position that matches (or -1 for no match in the full line) in the skipOffsets hash for re-use
- have capturesForLastDynamicSkipOffset as guard for dynamic regexes to invalidate the cache if they might have changed
current active format stored as pointer to avoid deconstruction/constructions inside the internal loop the pointers are stable, the formats are either in the contexts or rules
cached first non-space character, needs to be computed if < 0
avoid that we loop endless for some broken hl definitions
try to match all rules in the context in order of declaration in XML
filter out rules that require a specific column
filter out rules that only match for leading whitespace
compute the first non-space lazy avoids computing it for contexts without any such rules
can we skip?
shall we skip application of this rule? two cases:
- rule can't match at all => currentSkipOffset < 0
- rule will only match for some higher offset => currentSkipOffset > offset
we need to invalidate this if we are dynamic and have different captures then last time
update skip offset if new one rules out any later match or is larger than current one
apply folding. special cases:
- rule with endRegion + beginRegion: in endRegion, the length is 0
- rule with lookAhead: length is 0
if we arrive here, some new format has to be set!
on format change, apply the last one and switch to new one
we must have made progress if we arrive here!
apply format for remaining text, if any
handle line end context switches guard against endless loops see https://phabricator.kde.org/D18509
Definition at line 101 of file abstracthighlighter.cpp.
◆ setDefinition()
|
virtual |
Sets the syntax definition used for highlighting.
Subclasses can re-implement this method to e.g. trigger re-highlighting or clear internal data structures if needed.
Reimplemented in KSyntaxHighlighting::SyntaxHighlighter.
Definition at line 69 of file abstracthighlighter.cpp.
◆ setTheme()
|
virtual |
Sets the theme used for highlighting.
Subclasses can re-implement this method to e.g. trigger re-highlighing or to do general palette color setup.
Reimplemented in KSyntaxHighlighting::SyntaxHighlighter.
Definition at line 81 of file abstracthighlighter.cpp.
◆ theme()
Theme AbstractHighlighter::theme | ( | ) | const |
Returns the currently selected theme for highlighting.
- Note
- If no Theme was set through setTheme(), the returned Theme will be invalid, see Theme::isValid().
Definition at line 75 of file abstracthighlighter.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 157 of file abstracthighlighter.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri May 17 2024 11:50:00 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.