NetworkManager::Device
#include <device.h>
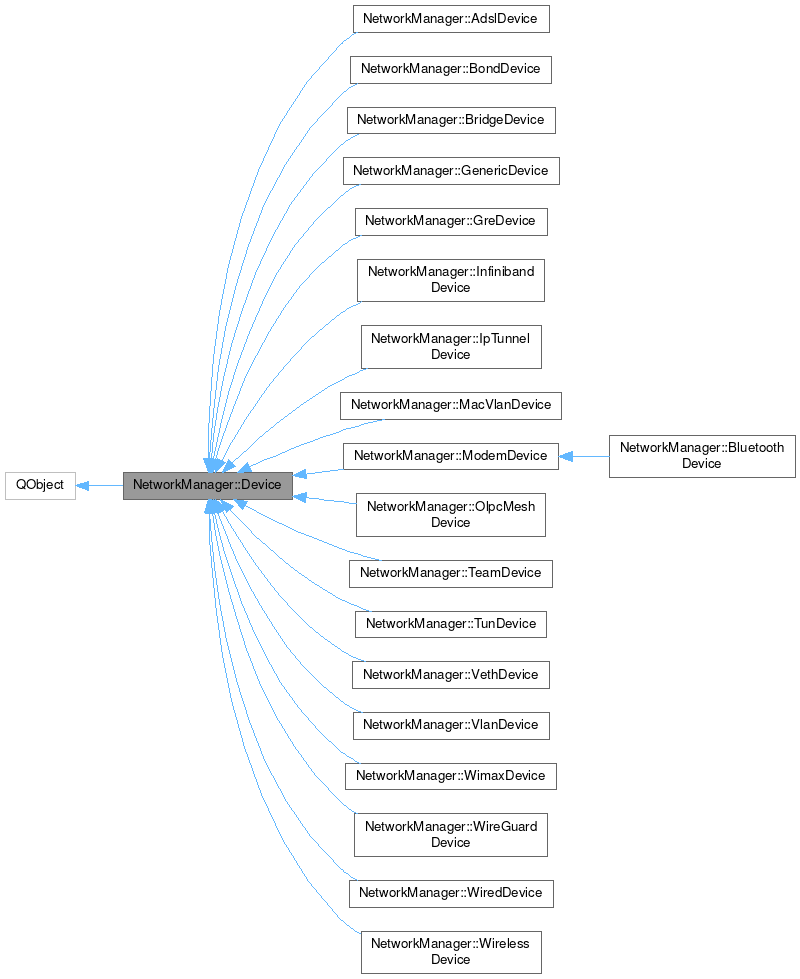
Public Types | |
typedef QFlags< Capability > | Capabilities |
enum | Capability { IsManageable = 0x1 , SupportsCarrierDetect = 0x2 } |
enum | Interfaceflag { None = NM_DEVICE_INTERFACE_FLAG_NONE , Up = NM_DEVICE_INTERFACE_FLAG_UP , LowerUp = NM_DEVICE_INTERFACE_FLAG_LOWER_UP , Carrier = NM_DEVICE_INTERFACE_FLAG_CARRIER } |
typedef QFlags< Interfaceflag > | Interfaceflags |
typedef QList< Ptr > | List |
enum | MeteredStatus { UnknownStatus = 0 , Yes = 1 , No = 2 , GuessYes = 3 , GuessNo = 4 } |
typedef QSharedPointer< Device > | Ptr |
enum | State { UnknownState = 0 , Unmanaged = 10 , Unavailable = 20 , Disconnected = 30 , Preparing = 40 , ConfiguringHardware = 50 , NeedAuth = 60 , ConfiguringIp = 70 , CheckingIp = 80 , WaitingForSecondaries = 90 , Activated = 100 , Deactivating = 110 , Failed = 120 } |
enum | StateChangeReason { UnknownReason = 0 , NoReason = 1 , NowManagedReason = 2 , NowUnmanagedReason = 3 , ConfigFailedReason = 4 , ConfigUnavailableReason = 5 , ConfigExpiredReason = 6 , NoSecretsReason = 7 , AuthSupplicantDisconnectReason = 8 , AuthSupplicantConfigFailedReason = 9 , AuthSupplicantFailedReason = 10 , AuthSupplicantTimeoutReason = 11 , PppStartFailedReason = 12 , PppDisconnectReason = 13 , PppFailedReason = 14 , DhcpStartFailedReason = 15 , DhcpErrorReason = 16 , DhcpFailedReason = 17 , SharedStartFailedReason = 18 , SharedFailedReason = 19 , AutoIpStartFailedReason = 20 , AutoIpErrorReason = 21 , AutoIpFailedReason = 22 , ModemBusyReason = 23 , ModemNoDialToneReason = 24 , ModemNoCarrierReason = 25 , ModemDialTimeoutReason = 26 , ModemDialFailedReason = 27 , ModemInitFailedReason = 28 , GsmApnSelectFailedReason = 29 , GsmNotSearchingReason = 30 , GsmRegistrationDeniedReason = 31 , GsmRegistrationTimeoutReason = 32 , GsmRegistrationFailedReason = 33 , GsmPinCheckFailedReason = 34 , FirmwareMissingReason = 35 , DeviceRemovedReason = 36 , SleepingReason = 37 , ConnectionRemovedReason = 38 , UserRequestedReason = 39 , CarrierReason = 40 , ConnectionAssumedReason = 41 , SupplicantAvailableReason = 42 , ModemNotFoundReason = 43 , BluetoothFailedReason = 44 , GsmSimNotInserted = 45 , GsmSimPinRequired = 46 , GsmSimPukRequired = 47 , GsmSimWrong = 48 , InfiniBandMode = 49 , DependencyFailed = 50 , Br2684Failed = 51 , ModemManagerUnavailable = 52 , SsidNotFound = 53 , SecondaryConnectionFailed = 54 , DcbFcoeFailed = 55 , TeamdControlFailed = 56 , ModemFailed = 57 , ModemAvailable = 58 , SimPinIncorrect = 59 , NewActivation = 60 , ParentChanged = 61 , ParentManagedChanged = 62 , Reserved = 65536 } |
enum | Type { UnknownType = NM_DEVICE_TYPE_UNKNOWN , Ethernet = NM_DEVICE_TYPE_ETHERNET , Wifi = NM_DEVICE_TYPE_WIFI , Unused1 = NM_DEVICE_TYPE_UNUSED1 , Unused2 = NM_DEVICE_TYPE_UNUSED2 , Bluetooth = NM_DEVICE_TYPE_BT , OlpcMesh = NM_DEVICE_TYPE_OLPC_MESH , Wimax = NM_DEVICE_TYPE_WIMAX , Modem = NM_DEVICE_TYPE_MODEM , InfiniBand = NM_DEVICE_TYPE_INFINIBAND , Bond = NM_DEVICE_TYPE_BOND , Vlan = NM_DEVICE_TYPE_VLAN , Adsl = NM_DEVICE_TYPE_ADSL , Bridge = NM_DEVICE_TYPE_BRIDGE , Generic = NM_DEVICE_TYPE_GENERIC , Team = NM_DEVICE_TYPE_TEAM , Gre , MacVlan , Tun , Veth , IpTunnel , VxLan , MacSec , Dummy , Ppp , OvsInterface , OvsPort , OvsBridge , Wpan , Lowpan , WireGuard , WifiP2P } |
typedef QFlags< Type > | Types |
Properties | |
bool | autoconnect |
NetworkManager::DeviceStatistics::Ptr | deviceStatistics |
QString | driver |
QString | driverVersion |
bool | firmwareMissing |
QString | firmwareVersion |
QVariant | genericCapabilities |
Interfaceflags | InterfaceFlags |
QString | interfaceName |
QString | ipInterfaceName |
QHostAddress | ipV4Address |
bool | managed |
MeteredStatus | metered |
uint | mtu |
bool | nmPluginMissing |
State | state |
DeviceStateReason | stateReason |
QString | udi |
QString | uni |
![]() | |
objectName | |
Signals | |
void | activeConnectionChanged () |
void | autoconnectChanged () |
void | availableConnectionAppeared (const QString &connection) |
void | availableConnectionChanged () |
void | availableConnectionDisappeared (const QString &connection) |
void | capabilitiesChanged () |
void | connectionStateChanged () |
void | dhcp4ConfigChanged () |
void | dhcp6ConfigChanged () |
void | driverChanged () |
void | driverVersionChanged () |
void | firmwareMissingChanged () |
void | firmwareVersionChanged () |
void | interfaceFlagsChanged () |
void | interfaceNameChanged () |
void | ipInterfaceChanged () |
void | ipV4AddressChanged () |
void | ipV4ConfigChanged () |
void | ipV6ConfigChanged () |
void | managedChanged () |
void | meteredChanged (MeteredStatus metered) |
void | mtuChanged () |
void | nmPluginMissingChanged (bool nmPluginMissing) |
void | physicalPortIdChanged () |
void | stateChanged (NetworkManager::Device::State newstate, NetworkManager::Device::State oldstate, NetworkManager::Device::StateChangeReason reason) |
void | stateReasonChanged () |
void | udiChanged () |
Public Member Functions | |
Device (const QString &path, QObject *parent=nullptr) | |
~Device () override | |
NetworkManager::ActiveConnection::Ptr | activeConnection () const |
template<class DevIface > | |
DevIface * | as () |
template<class DevIface > | |
const DevIface * | as () const |
bool | autoconnect () const |
Connection::List | availableConnections () |
Capabilities | capabilities () const |
QVariant | capabilitiesV () const |
QDBusPendingReply | deleteInterface () |
int | designSpeed () const |
DeviceStatistics::Ptr | deviceStatistics () const |
Dhcp4Config::Ptr | dhcp4Config () const |
Dhcp6Config::Ptr | dhcp6Config () const |
QDBusPendingReply | disconnectInterface () |
QString | driver () const |
QString | driverVersion () const |
bool | firmwareMissing () const |
QString | firmwareVersion () const |
Interfaceflags | interfaceFlags () const |
QString | interfaceName () const |
QString | ipInterfaceName () const |
QHostAddress | ipV4Address () const |
IpConfig | ipV4Config () const |
IpConfig | ipV6Config () const |
bool | isActive () const |
bool | isValid () const |
bool | managed () const |
MeteredStatus | metered () const |
uint | mtu () const |
bool | nmPluginMissing () const |
QString | physicalPortId () const |
QDBusPendingReply | reapplyConnection (const NMVariantMapMap &connection, qulonglong version_id, uint flags) |
void | setAutoconnect (bool autoconnect) |
State | state () const |
DeviceStateReason | stateReason () const |
virtual Type | type () const |
QString | udi () const |
QString | uni () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
NETWORKMANAGERQT_NO_EXPORT | Device (DevicePrivate &dd, QObject *parent) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
DevicePrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
Member Typedef Documentation
◆ Capabilities
typedef QFlags< Capability > NetworkManager::Device::Capabilities |
◆ Interfaceflags
◆ List
typedef QList<Ptr> NetworkManager::Device::List |
◆ Ptr
◆ Types
typedef QFlags< Type > NetworkManager::Device::Types |
Member Enumeration Documentation
◆ Capability
◆ Interfaceflag
◆ MeteredStatus
◆ State
Device connection states describe the possible states of a network connection from the user's point of view.
For simplicity, states from several different layers are present - this is a high level view
Enumerator | |
---|---|
UnknownState | The device is in an unknown state. |
Unmanaged | The device is recognized but not managed by NetworkManager. |
Unavailable | The device cannot be used (carrier off, rfkill, etc) |
Disconnected | The device is not connected. |
Preparing | The device is preparing to connect. |
ConfiguringHardware | The device is being configured. |
NeedAuth | The device is awaiting secrets necessary to continue connection. |
ConfiguringIp | The IP settings of the device are being requested and configured. |
CheckingIp | The device's IP connectivity ability is being determined. |
WaitingForSecondaries | The device is waiting for secondary connections to be activated. |
Activated | The device is active. |
Deactivating | The device's network connection is being torn down. |
Failed | The device is in a failure state following an attempt to activate it. |
◆ StateChangeReason
◆ Type
Device type.
Enumerator | |
---|---|
UnknownType | Unknown device type. |
Ethernet | Ieee8023 wired ethernet. |
Wifi | the Ieee80211 family of wireless networks |
Unused1 | Currently unused. |
Unused2 | Currently unused. |
Bluetooth | network bluetooth device (usually a cell phone) |
OlpcMesh | OLPC Mesh networking device. |
Wimax | WiMax WWAN technology. |
Modem | POTS, GSM, CDMA or LTE modems. |
InfiniBand | Infiniband network device. |
Bond | Bond virtual device. |
Vlan | Vlan virtual device. |
Adsl | ADSL modem device. |
Bridge | Bridge virtual device. |
Generic | Generic device.
|
Team | Team master device.
|
Gre | Gre virtual device.
|
MacVlan | MacVlan virtual device.
|
Tun | Tun virtual device.
|
Veth | Veth virtual device.
|
IpTunnel | IP Tunneling Device.
|
VxLan | Vxlan Device.
|
MacSec | MacSec Device.
|
Dummy | Dummy Device.
|
Ppp | Ppp Device.
|
OvsInterface | OvsInterface Device.
|
OvsPort | OvsPort Device.
|
OvsBridge | OvsBridge Device.
|
Wpan | Wpan Device.
|
Lowpan | Lowpan Device.
|
WireGuard | WireGuard Device.
|
WifiP2P | WifiP2P Device.
|
Property Documentation
◆ autoconnect
◆ deviceStatistics
|
read |
◆ driver
◆ driverVersion
◆ firmwareMissing
◆ firmwareVersion
◆ genericCapabilities
◆ InterfaceFlags
|
read |
◆ interfaceName
◆ ipInterfaceName
◆ ipV4Address
|
read |
◆ managed
◆ metered
|
read |
◆ mtu
◆ nmPluginMissing
◆ state
◆ stateReason
◆ udi
◆ uni
Constructor & Destructor Documentation
◆ Device() [1/2]
Creates a new device object.
- Parameters
-
path UNI of the device
Definition at line 220 of file device.cpp.
◆ ~Device()
|
override |
Destroys a device object.
Definition at line 375 of file device.cpp.
◆ Device() [2/2]
|
protected |
Definition at line 229 of file device.cpp.
Member Function Documentation
◆ activeConnection()
NetworkManager::ActiveConnection::Ptr Device::activeConnection | ( | ) | const |
The current active connection for this device.
- Returns
- A valid ActiveConnection object or NULL if no active connection was found
Definition at line 417 of file device.cpp.
◆ activeConnectionChanged
|
signal |
Emitted when the autoconnect of this network has changed.
◆ as() [1/2]
|
inline |
◆ as() [2/2]
|
inline |
◆ autoconnect()
bool Device::autoconnect | ( | ) | const |
If the device is allowed to autoconnect.
Definition at line 444 of file device.cpp.
◆ autoconnectChanged
|
signal |
Emitted when the autoconnect of this network has changed.
◆ availableConnectionAppeared
|
signal |
Emitted when a new connection is available.
◆ availableConnectionChanged
|
signal |
Emitted when the list of avaiable connections of this network has changed.
◆ availableConnectionDisappeared
|
signal |
Emitted when the connection is no longer available.
◆ availableConnections()
NetworkManager::Connection::List Device::availableConnections | ( | ) |
Returns available connections for this device.
- Returns
- List of availables connection
Definition at line 423 of file device.cpp.
◆ capabilities()
NetworkManager::Device::Capabilities Device::capabilities | ( | ) | const |
Retrieves the capabilities supported by this device.
- Returns
- the capabilities of the device
Definition at line 597 of file device.cpp.
◆ capabilitiesChanged
|
signal |
Emitted when the capabilities of this network has changed.
◆ capabilitiesV()
QVariant Device::capabilitiesV | ( | ) | const |
Definition at line 603 of file device.cpp.
◆ connectionStateChanged
|
signal |
Emitted when the connection state of this network has changed.
◆ deleteInterface()
QDBusPendingReply Device::deleteInterface | ( | ) |
Deletes a software device from NetworkManager and removes the interface from the system.
The method returns an error when called for a hardware device.
- Since
- 5.8.0
Definition at line 575 of file device.cpp.
◆ designSpeed()
int Device::designSpeed | ( | ) | const |
Retrieves the maximum speed as reported by the device.
Note that this is only a design related piece of information, and that the device might not reach this maximum.
- Returns
- the device's maximum speed
Definition at line 591 of file device.cpp.
◆ deviceStatistics()
NetworkManager::DeviceStatistics::Ptr Device::deviceStatistics | ( | ) | const |
Returns Device Statistics interface.
Definition at line 609 of file device.cpp.
◆ dhcp4Config()
NetworkManager::Dhcp4Config::Ptr Device::dhcp4Config | ( | ) | const |
Get the DHCP options returned by the DHCP server or a null pointer if the device is not Activated or does not use DHCP configuration.
Definition at line 498 of file device.cpp.
◆ dhcp4ConfigChanged
|
signal |
Emitted when the DHCP configuration for IPv4 of this network has changed.
◆ dhcp6Config()
NetworkManager::Dhcp6Config::Ptr Device::dhcp6Config | ( | ) | const |
Get the DHCP options returned by the DHCP server or a null pointer if the device is not Activated or does not use DHCP configuration.
Definition at line 507 of file device.cpp.
◆ dhcp6ConfigChanged
|
signal |
Emitted when the DHCP configuration for IPv6 of this network has changed.
◆ disconnectInterface()
QDBusPendingReply Device::disconnectInterface | ( | ) |
Disconnects a device and prevents the device from automatically activating further connections without user intervention.
Definition at line 569 of file device.cpp.
◆ driver()
QString Device::driver | ( | ) | const |
Handle for the system driver controlling this network interface.
Definition at line 399 of file device.cpp.
◆ driverChanged
|
signal |
Emitted when the driver of this network has changed.
◆ driverVersion()
QString Device::driverVersion | ( | ) | const |
The driver version.
Definition at line 405 of file device.cpp.
◆ driverVersionChanged
|
signal |
Emitted when the driver version of this network has changed.
◆ firmwareMissing()
bool Device::firmwareMissing | ( | ) | const |
Is the firmware needed by the device missing?
Definition at line 438 of file device.cpp.
◆ firmwareMissingChanged
|
signal |
Emitted when the firmware missing state of this network has changed.
◆ firmwareVersion()
QString Device::firmwareVersion | ( | ) | const |
The firmware version.
Definition at line 411 of file device.cpp.
◆ firmwareVersionChanged
|
signal |
Emitted when the firmware version of this network has changed.
◆ interfaceFlags()
NetworkManager::Device::Interfaceflags Device::interfaceFlags | ( | ) | const |
The up or down flag for the device.
Definition at line 539 of file device.cpp.
◆ interfaceFlagsChanged
|
signal |
Emitted when the up or down state of the device.
- Since
- 1.22
- Note
- will always return NM_DEVICE_INTERFACE_FLAG_NONE when runtime NM < 1.22
◆ interfaceName()
QString Device::interfaceName | ( | ) | const |
The system name for the network device.
Definition at line 387 of file device.cpp.
◆ interfaceNameChanged
|
signal |
Emitted when the interface name of this network has changed.
◆ ipInterfaceChanged
|
signal |
Emitted when the ip interface name of this network has changed.
◆ ipInterfaceName()
QString Device::ipInterfaceName | ( | ) | const |
The name of the device's data interface when available.
This property may not refer to the actual data interface until the device has successfully established a data connection, indicated by the device's state() becoming ACTIVATED.
Definition at line 393 of file device.cpp.
◆ ipV4Address()
QHostAddress Device::ipV4Address | ( | ) | const |
returns the current IPv4 address without the prefix
- See also
- ipV4Config()
- ipV6Config()
Definition at line 468 of file device.cpp.
◆ ipV4AddressChanged
|
signal |
Emitted when the IPv4 address of this network has changed.
◆ ipV4Config()
NetworkManager::IpConfig Device::ipV4Config | ( | ) | const |
Get the current IPv4 configuration of this device.
Only valid when device is Activated.
Definition at line 480 of file device.cpp.
◆ ipV4ConfigChanged
|
signal |
Emitted when the IPv4 configuration of this network has changed.
◆ ipV6Config()
NetworkManager::IpConfig Device::ipV6Config | ( | ) | const |
Get the current IPv6 configuration of this device.
Only valid when device is Activated.
Definition at line 489 of file device.cpp.
◆ ipV6ConfigChanged
|
signal |
Emitted when the IPv6 configuration of this network has changed.
◆ isActive()
bool Device::isActive | ( | ) | const |
Retrieves the activation status of this network interface.
- Returns
- true if this network interface is active, false otherwise
Definition at line 516 of file device.cpp.
◆ isValid()
bool Device::isValid | ( | ) | const |
Retrieves the device is valid.
- Returns
- true if this device interface is valid, false otherwise
Definition at line 527 of file device.cpp.
◆ managed()
bool Device::managed | ( | ) | const |
Is the device currently being managed by NetworkManager?
Definition at line 533 of file device.cpp.
◆ managedChanged
|
signal |
Emitted when the managed state of this network has changed.
◆ metered()
NetworkManager::Device::MeteredStatus Device::metered | ( | ) | const |
- Returns
- Whether the amount of traffic flowing through the device is subject to limitations, for example set by service providers.
- Since
- 5.14.0
Definition at line 557 of file device.cpp.
◆ meteredChanged
|
signal |
Emitted when metered property has changed.
- Since
- 5.14.0
- See also
- metered
◆ mtu()
uint Device::mtu | ( | ) | const |
◆ mtuChanged
|
signal |
Emitted when the maximum transmission unit has changed.
- Since
- 0.9.9.0
◆ nmPluginMissing()
bool Device::nmPluginMissing | ( | ) | const |
- Returns
- If TRUE, indicates the NetworkManager plugin for the device is likely missing or misconfigured.
- Since
- 5.14.0
Definition at line 551 of file device.cpp.
◆ nmPluginMissingChanged
|
signal |
Emitted when NmPluginMissing property has changed.
- Since
- 5.14.0
- See also
- nmPluginMissing
◆ physicalPortId()
QString Device::physicalPortId | ( | ) | const |
- Returns
- If non-empty, an (opaque) indicator of the physical network port associated with the device. This can be used to recognize when two seemingly-separate hardware devices are actually just different virtual interfaces to the same physical port.
- Since
- 0.9.9.0
Definition at line 462 of file device.cpp.
◆ physicalPortIdChanged
|
signal |
◆ reapplyConnection()
QDBusPendingReply Device::reapplyConnection | ( | const NMVariantMapMap & | connection, |
qulonglong | version_id, | ||
uint | flags ) |
Reapplies connection settings on the interface.
Definition at line 563 of file device.cpp.
◆ setAutoconnect()
void Device::setAutoconnect | ( | bool | autoconnect | ) |
If true, indicates the device is allowed to autoconnect.
If false, manual intervention is required before the device will automatically connect to a known network, such as activating a connection using the device, or setting this property to true
.
Definition at line 450 of file device.cpp.
◆ state()
NetworkManager::Device::State Device::state | ( | ) | const |
Retrieves the current state of the device.
This is a high level view of the device. It is user oriented, so actually it provides state coming from different layers.
- Returns
- the current connection state
- See also
- Device::State
Definition at line 585 of file device.cpp.
◆ stateChanged
|
signal |
This signal is emitted when the device's link status changed.
- Parameters
-
newstate the new state of the connection oldstate the previous state of the connection reason the reason for the state change, if any. ReasonNone where the backend provides no reason.
◆ stateReason()
NetworkManager::DeviceStateReason Device::stateReason | ( | ) | const |
The current state and reason for changing to that state.
Definition at line 474 of file device.cpp.
◆ stateReasonChanged
|
signal |
Emitted when the state reason of this network has changed.
◆ type()
|
virtual |
Retrieves the interface type.
This is a virtual function that will return the proper type of all sub-classes.
- Returns
- the NetworkManager::Device::Type that corresponds to this device.
Reimplemented in NetworkManager::AdslDevice, NetworkManager::BluetoothDevice, NetworkManager::BondDevice, NetworkManager::BridgeDevice, NetworkManager::GenericDevice, NetworkManager::GreDevice, NetworkManager::InfinibandDevice, NetworkManager::IpTunnelDevice, NetworkManager::MacVlanDevice, NetworkManager::ModemDevice, NetworkManager::OlpcMeshDevice, NetworkManager::TeamDevice, NetworkManager::TunDevice, NetworkManager::VethDevice, NetworkManager::VlanDevice, NetworkManager::WimaxDevice, NetworkManager::WiredDevice, NetworkManager::WireGuardDevice, and NetworkManager::WirelessDevice.
Definition at line 652 of file device.cpp.
◆ udi()
QString Device::udi | ( | ) | const |
Retrieves the Unique Device Identifier (UDI) of the device.
This identifier is unique for each device in the system.
Definition at line 456 of file device.cpp.
◆ udiChanged
|
signal |
Emitted when the Unique Device Identifier of this device has changed.
◆ uni()
QString Device::uni | ( | ) | const |
Retrieves the Unique Network Identifier (UNI) of the device.
This identifier is unique for each network and network interface in the system.
- Returns
- the Unique Network Identifier of the current device
Definition at line 381 of file device.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:07:05 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.