libkcal
KCal::Attendee Class Reference
This class represents information related to an attendee of an event. More...
#include <attendee.h>
Inheritance diagram for KCal::Attendee:
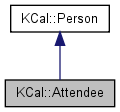
Public Types | |
typedef ListBase< Attendee > | List |
enum | PartStat { NeedsAction, Accepted, Declined, Tentative, Delegated, Completed, InProcess } |
enum | Role { ReqParticipant, OptParticipant, NonParticipant, Chair } |
Public Member Functions | |
Attendee (const QString &name, const QString &email, bool rsvp=false, PartStat status=NeedsAction, Role role=ReqParticipant, const QString &u=QString::null) | |
QString | delegate () const |
QString | delegator () const |
Role | role () const |
QString | roleStr () const |
bool | RSVP () const |
void | setDelegate (const QString &delegate) |
void | setDelegator (const QString &delegator) |
void | setRole (Role) |
void | setRSVP (bool r) |
void | setStatus (PartStat s) |
void | setUid (const QString &) |
PartStat | status () const |
QString | statusStr () const |
QString | uid () const |
virtual | ~Attendee () |
Static Public Member Functions | |
static QStringList | roleList () |
static QString | roleName (Role) |
static QStringList | statusList () |
static QString | statusName (PartStat) |
Detailed Description
This class represents information related to an attendee of an event.Definition at line 36 of file attendee.h.
Member Typedef Documentation
typedef ListBase<Attendee> KCal::Attendee::List |
Definition at line 43 of file attendee.h.
Member Enumeration Documentation
Definition at line 39 of file attendee.h.
enum KCal::Attendee::Role |
Definition at line 41 of file attendee.h.
Constructor & Destructor Documentation
Attendee::Attendee | ( | const QString & | name, | |
const QString & | email, | |||
bool | rsvp = false , |
|||
Attendee::PartStat | s = NeedsAction , |
|||
Attendee::Role | r = ReqParticipant , |
|||
const QString & | u = QString::null | |||
) |
Create Attendee.
- Parameters:
-
name Name email Email address rsvp Request for reply status Status (see enum for list) role Role u the uid for the attendee
Definition at line 31 of file attendee.cpp.
Attendee::~Attendee | ( | ) | [virtual] |
Member Function Documentation
QString KCal::Attendee::delegate | ( | ) | const [inline] |
QString KCal::Attendee::delegator | ( | ) | const [inline] |
Attendee::Role Attendee::role | ( | ) | const |
QStringList Attendee::roleList | ( | ) | [static] |
QString Attendee::roleName | ( | Attendee::Role | r | ) | [static] |
QString Attendee::roleStr | ( | ) | const |
bool KCal::Attendee::RSVP | ( | ) | const [inline] |
void KCal::Attendee::setDelegate | ( | const QString & | delegate | ) | [inline] |
void KCal::Attendee::setDelegator | ( | const QString & | delegator | ) | [inline] |
void Attendee::setRole | ( | Attendee::Role | r | ) |
void KCal::Attendee::setRSVP | ( | bool | r | ) | [inline] |
void Attendee::setStatus | ( | Attendee::PartStat | s | ) |
Set status.
See enum for definitions of possible values.
Definition at line 56 of file attendee.cpp.
void Attendee::setUid | ( | const QString & | uid | ) |
Attendee::PartStat Attendee::status | ( | ) | const |
QStringList Attendee::statusList | ( | ) | [static] |
Return string representations of all available attendee status values.
Definition at line 99 of file attendee.cpp.
QString Attendee::statusName | ( | Attendee::PartStat | s | ) | [static] |
QString Attendee::statusStr | ( | ) | const |
QString Attendee::uid | ( | ) | const |
The documentation for this class was generated from the following files: