libkcal
KCal::Incidence Class Reference
This class provides the base class common to all calendar components. More...
#include <incidence.h>
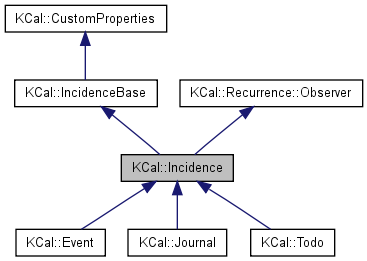
Classes | |
class | AddVisitor |
This class implements a visitor for adding an Incidence to a resource supporting addEvent(), addTodo() and addJournal() calls. More... | |
class | DeleteVisitor |
This class implements a visitor for deleting an Incidence from a resource supporting deleteEvent(), deleteTodo() and deleteJournal() calls. More... | |
Public Types | |
enum | { SecrecyPublic = 0, SecrecyPrivate = 1, SecrecyConfidential = 2 } |
typedef ListBase< Incidence > | List |
enum | Status { StatusNone, StatusTentative, StatusConfirmed, StatusCompleted, StatusNeedsAction, StatusCanceled, StatusInProcess, StatusDraft, StatusFinal, StatusX } |
Public Member Functions | |
void | addAlarm (Alarm *) |
void | addAttachment (Attachment *attachment) |
void | addRelation (Incidence *) |
const Alarm::List & | alarms () const |
Attachment::List | attachments (const QString &mime) const |
Attachment::List | attachments () const |
QStringList | categories () const |
QString | categoriesStr () const |
void | clearAlarms () |
void | clearAttachments () |
void | clearRecurrence () |
virtual Incidence * | clone ()=0 |
QDateTime | created () const |
void | deleteAttachment (Attachment *attachment) |
void | deleteAttachments (const QString &mime) |
QString | description () const |
bool | doesRecur () const |
virtual QDateTime | dtEnd () const |
virtual QDateTime | endDateForStart (const QDateTime &startDt) const |
Incidence (const Incidence &) | |
Incidence () | |
bool | isAlarmEnabled () const |
QString | location () const |
Alarm * | newAlarm () |
Incidence & | operator= (const Incidence &i) |
bool | operator== (const Incidence &) const |
int | priority () const |
void | recreate () |
Recurrence * | recurrence () const |
uint | recurrenceType () const |
virtual void | recurrenceUpdated (Recurrence *) |
bool | recursAt (const QDateTime &qdt) const |
virtual bool | recursOn (const QDate &qd) const |
Incidence * | relatedTo () const |
QString | relatedToUid () const |
Incidence::List | relations () const |
void | removeAlarm (Alarm *) |
void | removeRelation (Incidence *) |
QStringList | resources () const |
int | revision () const |
QString | schedulingID () const |
int | secrecy () const |
QString | secrecyStr () const |
void | setCategories (const QString &catStr) |
void | setCategories (const QStringList &categories) |
void | setCreated (const QDateTime &) |
void | setCustomStatus (const QString &status) |
void | setDescription (const QString &description) |
virtual void | setDtStart (const QDateTime &dtStart) |
void | setFloats (bool f) |
void | setLocation (const QString &location) |
void | setPriority (int priority) |
void | setReadOnly (bool readonly) |
void | setRelatedTo (Incidence *relatedTo) |
void | setRelatedToUid (const QString &) |
void | setResources (const QStringList &resources) |
void | setRevision (int rev) |
void | setSchedulingID (const QString &sid) |
void | setSecrecy (int) |
void | setStatus (Status status) |
void | setSummary (const QString &summary) |
virtual QValueList< QDateTime > | startDateTimesForDate (const QDate &date) const |
virtual QValueList< QDateTime > | startDateTimesForDateTime (const QDateTime &datetime) const |
Status | status () const |
QString | statusStr () const |
QString | summary () const |
~Incidence () | |
Static Public Member Functions | |
static QStringList | secrecyList () |
static QString | secrecyName (int) |
static QString | statusName (Status) |
Protected Member Functions | |
virtual QDateTime | endDateRecurrenceBase () const |
Detailed Description
This class provides the base class common to all calendar components.Definition at line 43 of file incidence.h.
Member Typedef Documentation
typedef ListBase<Incidence> KCal::Incidence::List |
Reimplemented in KCal::Event, KCal::Journal, and KCal::Todo.
Definition at line 93 of file incidence.h.
Member Enumeration Documentation
anonymous enum |
Enumeration for describing an event's status.
- Enumerator:
-
StatusNone StatusTentative StatusConfirmed StatusCompleted StatusNeedsAction StatusCanceled StatusInProcess StatusDraft StatusFinal StatusX
Definition at line 83 of file incidence.h.
Constructor & Destructor Documentation
Incidence::Incidence | ( | ) |
Definition at line 33 of file incidence.cpp.
Incidence::Incidence | ( | const Incidence & | i | ) |
Definition at line 44 of file incidence.cpp.
Incidence::~Incidence | ( | ) |
Definition at line 90 of file incidence.cpp.
Member Function Documentation
void Incidence::addAlarm | ( | Alarm * | alarm | ) |
void Incidence::addAttachment | ( | Attachment * | attachment | ) |
void Incidence::addRelation | ( | Incidence * | event | ) |
const Alarm::List & Incidence::alarms | ( | ) | const |
Attachment::List Incidence::attachments | ( | const QString & | mime | ) | const |
Attachment::List Incidence::attachments | ( | ) | const |
QStringList Incidence::categories | ( | ) | const |
QString Incidence::categoriesStr | ( | ) | const |
void Incidence::clearAlarms | ( | ) |
Remove all alarms that are associated with this incidence.
Definition at line 799 of file incidence.cpp.
void Incidence::clearAttachments | ( | ) |
void Incidence::clearRecurrence | ( | ) |
virtual Incidence* KCal::Incidence::clone | ( | ) | [pure virtual] |
Return copy of this object.
The returned object is owned by the caller.
Implemented in KCal::Event, KCal::Journal, and KCal::Todo.
QDateTime Incidence::created | ( | ) | const |
void Incidence::deleteAttachment | ( | Attachment * | attachment | ) |
void Incidence::deleteAttachments | ( | const QString & | mime | ) |
Remove and delete all attachments with this mime type.
Definition at line 632 of file incidence.cpp.
QString Incidence::description | ( | ) | const |
bool Incidence::doesRecur | ( | ) | const |
virtual QDateTime KCal::Incidence::dtEnd | ( | ) | const [inline, virtual] |
Return the incidence's ending date/time as a QDateTime.
Reimplemented in KCal::Event.
Definition at line 150 of file incidence.h.
Return the end time of the occurrence if it starts at the given date/time.
Definition at line 519 of file incidence.cpp.
virtual QDateTime KCal::Incidence::endDateRecurrenceBase | ( | ) | const [inline, protected, virtual] |
Return the end date/time of the base incidence (e.g.
due date/time for to-dos, end date/time for events). This method needs to be reimplemented by derived classes.
Reimplemented in KCal::Event, and KCal::Todo.
Definition at line 451 of file incidence.h.
bool Incidence::isAlarmEnabled | ( | ) | const |
Return whether any alarm associated with this incidence is enabled.
Definition at line 805 of file incidence.cpp.
QString Incidence::location | ( | ) | const |
Return the event's/todo's location.
Do _not_ use it with journal.
Definition at line 821 of file incidence.cpp.
Alarm * Incidence::newAlarm | ( | ) |
Create a new alarm which is associated with this incidence.
Definition at line 779 of file incidence.cpp.
Definition at line 108 of file incidence.cpp.
bool Incidence::operator== | ( | const Incidence & | i2 | ) | const |
Definition at line 154 of file incidence.cpp.
int Incidence::priority | ( | ) | const |
Return priority.
The priority is a number between 1 and 9. 1 is highest priority. If the priority is undefined 0 is returned.
Definition at line 682 of file incidence.cpp.
void Incidence::recreate | ( | ) |
Recreate event.
The event is made a new unique event, but already stored event information is preserved. Sets uniquie id, creation date, last modification date and revision number.
Definition at line 200 of file incidence.cpp.
Recurrence * Incidence::recurrence | ( | ) | const |
Return the recurrence rule associated with this incidence.
If there is none, returns an appropriate (non-0) object.
Definition at line 382 of file incidence.cpp.
uint Incidence::recurrenceType | ( | ) | const |
Definition at line 402 of file incidence.cpp.
void Incidence::recurrenceUpdated | ( | Recurrence * | recurrence | ) | [virtual] |
Observer interface for the recurrence class.
If the recurrence is changed, this method will be called for the incidence the recurrence object belongs to.
Implements KCal::Recurrence::Observer.
Definition at line 842 of file incidence.cpp.
bool Incidence::recursAt | ( | const QDateTime & | qdt | ) | const |
Returns true if the date/time specified is one on which the incidence will recur.
Definition at line 419 of file incidence.cpp.
bool Incidence::recursOn | ( | const QDate & | qd | ) | const [virtual] |
Returns true if the date specified is one on which the incidence will recur.
Reimplemented in KCal::Todo.
Definition at line 414 of file incidence.cpp.
Incidence * Incidence::relatedTo | ( | ) | const |
QString Incidence::relatedToUid | ( | ) | const |
What event does this one relate to? This function should only be used when constructing a calendar before the related Incidence exists.
Definition at line 332 of file incidence.cpp.
Incidence::List Incidence::relations | ( | ) | const |
void Incidence::removeAlarm | ( | Alarm * | alarm | ) |
Remove an alarm that is associated with this incidence.
Definition at line 793 of file incidence.cpp.
void Incidence::removeRelation | ( | Incidence * | event | ) |
QStringList Incidence::resources | ( | ) | const |
int Incidence::revision | ( | ) | const |
QString Incidence::schedulingID | ( | ) | const |
Return the event's/todo's scheduling ID.
Does not make sense for journals If this is not set, it will return uid().
Definition at line 831 of file incidence.cpp.
int Incidence::secrecy | ( | ) | const |
QStringList Incidence::secrecyList | ( | ) | [static] |
Return list of all available secrecy states as list of translated strings.
Definition at line 763 of file incidence.cpp.
QString Incidence::secrecyName | ( | int | secrecy | ) | [static] |
Return human-readable translated name of secrecy class.
Definition at line 749 of file incidence.cpp.
QString Incidence::secrecyStr | ( | ) | const |
void Incidence::setCategories | ( | const QString & | catStr | ) |
void Incidence::setCategories | ( | const QStringList & | categories | ) |
void Incidence::setCreated | ( | const QDateTime & | created | ) |
void Incidence::setCustomStatus | ( | const QString & | status | ) |
Sets the incidence status to a non-standard status value.
- Parameters:
-
status non-standard status string. If empty, the incidence status will be set to StatusNone.
Definition at line 695 of file incidence.cpp.
void Incidence::setDescription | ( | const QString & | description | ) |
void Incidence::setDtStart | ( | const QDateTime & | dtStart | ) | [virtual] |
Set starting date/time.
Reimplemented from KCal::IncidenceBase.
Reimplemented in KCal::Todo.
Definition at line 256 of file incidence.cpp.
void Incidence::setFloats | ( | bool | f | ) |
Set whether the incidence floats, i.e.
has a date but no time attached to it.
Reimplemented from KCal::IncidenceBase.
Definition at line 221 of file incidence.cpp.
void Incidence::setLocation | ( | const QString & | location | ) |
Set the event's/todo's location.
Do _not_ use it with journal.
Definition at line 814 of file incidence.cpp.
void Incidence::setPriority | ( | int | priority | ) |
Set the incidences priority.
The priority has to be a value between 0 and 9, 0 is undefined, 1 the highest, 9 the lowest priority (decreasing order).
Definition at line 675 of file incidence.cpp.
void Incidence::setReadOnly | ( | bool | readonly | ) | [virtual] |
Set readonly state of incidence.
- Parameters:
-
readonly If true, the incidence is set to readonly, if false the incidence is set to readwrite.
Reimplemented from KCal::IncidenceBase.
Definition at line 214 of file incidence.cpp.
void Incidence::setRelatedTo | ( | Incidence * | relatedTo | ) |
void Incidence::setRelatedToUid | ( | const QString & | relatedToUid | ) |
Point at some other event to which the event relates.
This function should only be used when constructing a calendar before the related Incidence exists.
Definition at line 325 of file incidence.cpp.
void Incidence::setResources | ( | const QStringList & | resources | ) |
void Incidence::setRevision | ( | int | rev | ) |
void Incidence::setSchedulingID | ( | const QString & | sid | ) |
Set the event's/todo's scheduling ID.
Does not make sense for journals. This is used for accepted invitations as the place to store the UID of the invitation. It is later used again if updates to the invitation comes in. If we did not set a new UID on incidences from invitations, we can end up with more than one resource having events with the same UID, if you have access to other peoples resources.
Definition at line 826 of file incidence.cpp.
void Incidence::setSecrecy | ( | int | sec | ) |
Sets secrecy status.
This can be Public, Private or Confidential. See separate enum.
Definition at line 732 of file incidence.cpp.
void Incidence::setStatus | ( | Incidence::Status | status | ) |
Sets the incidence status to a standard status value.
See separate enum. Note that StatusX cannot be specified.
Definition at line 687 of file incidence.cpp.
void Incidence::setSummary | ( | const QString & | summary | ) |
QValueList< QDateTime > Incidence::startDateTimesForDate | ( | const QDate & | date | ) | const [virtual] |
Calculates the start date/time for all recurrences that happen at some time on the given date (might start before that date, but end on or after the given date).
- Parameters:
-
date the date where the incidence should occur
- Returns:
- the start date/time of all occurences that overlap with the given date. Empty list if the incidence does not overlap with the date at all
Definition at line 432 of file incidence.cpp.
QValueList< QDateTime > Incidence::startDateTimesForDateTime | ( | const QDateTime & | datetime | ) | const [virtual] |
Calculates the start date/time for all recurrences that happen at the given time.
- Parameters:
-
datetime the date/time where the incidence should occur
- Returns:
- the start date/time of all occurences that overlap with the given date/time. Empty list if the incidence does not happen at the given time at all.
Definition at line 479 of file incidence.cpp.
Incidence::Status Incidence::status | ( | ) | const |
QString Incidence::statusName | ( | Incidence::Status | status | ) | [static] |
Return human-readable translated name of status value.
Definition at line 715 of file incidence.cpp.
QString Incidence::statusStr | ( | ) | const |
QString Incidence::summary | ( | ) | const |
The documentation for this class was generated from the following files: