libkcal
recurrencerule.cpp File Reference
#include "recurrencerule.h"
#include <kdebug.h>
#include <kglobal.h>
#include <qdatetime.h>
#include <qstringlist.h>
#include <limits.h>
#include <math.h>
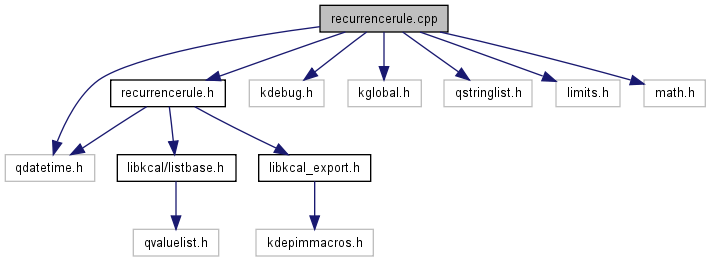
Go to the source code of this file.
Defines | |
#define | dumpByIntList(list, label) |
#define | fixConstraint(element, value) |
#define | intConstraint(list, element) |
#define | mergeConstraint(name, cmparison) |
Functions | |
long long | ownSecsTo (const QDateTime &dt1, const QDateTime &dt2) |
Define Documentation
#define dumpByIntList | ( | list, | |||
label | ) |
Value:
if ( !list.isEmpty() ) {\ QStringList lst;\ for ( QValueList<int>::ConstIterator it = list.begin();\ it != list.end(); ++it ) {\ lst.append( QString::number( *it ) );\ }\ kdDebug(5800) << " " << label << lst.join(", ") << endl;\ }
#define fixConstraint | ( | element, | |||
value | ) |
Value:
{ \
tmp.clear(); \
for ( it = mConstraints.constBegin(); it != mConstraints.constEnd(); ++it ) { \
con = (*it); con.element = value; tmp.append( con ); \
} \
mConstraints = tmp; \
}
#define intConstraint | ( | list, | |||
element | ) |
Value:
if ( !list.isEmpty() ) { \ for ( it = mConstraints.constBegin(); it != mConstraints.constEnd(); ++it ) { \ for ( intit = list.constBegin(); intit != list.constEnd(); ++intit ) { \ con = (*it); \ con.element = (*intit); \ tmp.append( con ); \ } \ } \ mConstraints = tmp; \ tmp.clear(); \ }
#define mergeConstraint | ( | name, | |||
cmparison | ) |
Value:
if ( conit.name cmparison ) { \ if ( !(result.name cmparison) || result.name == conit.name ) { \ result.name = conit.name; \ } else return false;\ }
Function Documentation
Workaround for broken QDateTime::secsTo (at least in Qt 3.3).
While QDateTime::secsTo does take time zones into account, QDateTime::addSecs does not, so we have a problem: QDateTime d1(QDate(2005, 10, 30), QTime(1, 30, 0) ); QDateTime d2(QDate(2005, 10, 30), QTime(3, 30, 0) );
kdDebug(5800) << "d1=" << d1 << ", d2=" << d2 << endl; kdDebug(5800) << "d1.secsTo(d2)=" << d1.secsTo(d2) << endl; kdDebug(5800) << "d1.addSecs(d1.secsTo(d2))=" << d1.addSecs(d1.secsTo(d2)) << endl; This code generates the following output: libkcal: d1=Son Okt 30 01:30:00 2005, d2=Son Okt 30 03:30:00 2005 libkcal: d1.secsTo(d2)=10800 libkcal: d1.addSecs(d1.secsTo(d2))=Son Okt 30 04:30:00 2005 Notice that secsTo counts the hour between 2:00 and 3:00 twice, while adddSecs doesn't and so has one additional hour. This basically makes it impossible to use QDateTime for *any* calculations, in local time zone as well as in UTC. Since we don't want to use time zones anyway, but do all secondsly/minutely/hourly calculations in UTC, we simply use our own secsTo, which ignores all time zone shifts.
Definition at line 58 of file recurrencerule.cpp.