KCodecs::Codec
#include <KCodecs>
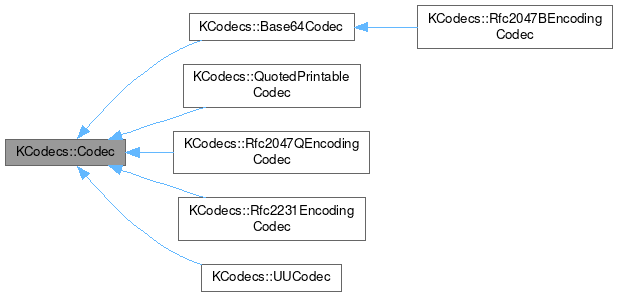
Public Types | |
enum | NewlineType { NewlineLF , NewlineCRLF } |
Public Member Functions | |
virtual | ~Codec () |
virtual bool | decode (const char *&scursor, const char *const send, char *&dcursor, const char *const dend, NewlineType newline=NewlineLF) const |
QByteArray | decode (QByteArrayView src, NewlineType newline=NewlineLF) const |
virtual bool | encode (const char *&scursor, const char *const send, char *&dcursor, const char *const dend, NewlineType newline=NewlineLF) const |
QByteArray | encode (QByteArrayView src, NewlineType newline=NewlineLF) const |
virtual Decoder * | makeDecoder (NewlineType newline=NewlineLF) const =0 |
virtual Encoder * | makeEncoder (NewlineType newline=NewlineLF) const =0 |
virtual qsizetype | maxDecodedSizeFor (qsizetype insize, NewlineType newline=NewlineLF) const =0 |
virtual qsizetype | maxEncodedSizeFor (qsizetype insize, NewlineType newline=NewlineLF) const =0 |
virtual const char * | name () const =0 |
Static Public Member Functions | |
static Codec * | codecForName (QByteArrayView name) |
Protected Member Functions | |
Codec () | |
Detailed Description
An abstract base class of codecs for common mail transfer encodings.
- Glossary
- MIME: Multipurpose Internet Mail Extensions or MIME is an Internet Standard that extends the format of e-mail to support text in character sets other than US-ASCII, non-text attachments, multi-part message bodies, and header information in non-ASCII character sets. Virtually all human-written Internet e-mail and a fairly large proportion of automated e-mail is transmitted via SMTP in MIME format. Internet e-mail is so closely associated with the SMTP and MIME standards that it is sometimes called SMTP/MIME e-mail. The content types defined by MIME standards are also of growing importance outside of e-mail, such as in communication protocols like HTTP for the World Wide Web. MIME is also a fundamental component of communication protocols such as HTTP, which requires that data be transmitted in the context of e-mail-like messages, even though the data may not actually be e-mail.
- Glossary
- codec: a program capable of performing encoding and decoding on a digital data stream. Codecs encode data for storage or encryption and decode it for viewing or editing.
- Glossary
- CRLF: a "Carriage Return (0x0D)" followed by a "Line Feed (0x0A)", two ASCII control characters used to represent a newline on some operating systems, notably DOS and Microsoft Windows.
- Glossary
- LF: a "Line Feed (0x0A)" ASCII control character used to represent a newline on some operating systems, notably Unix, Unix-like, and Linux.
Provides an abstract base class of codecs like base64 and quoted-printable. Implemented as a singleton.
- Since
- 5.5
Member Enumeration Documentation
◆ NewlineType
Constructor & Destructor Documentation
◆ ~Codec()
|
inlinevirtual |
◆ Codec()
|
inlineprotected |
Member Function Documentation
◆ codecForName()
|
static |
Returns a codec associated with the specified name
.
- Parameters
-
name is a valid codec name.
Definition at line 519 of file kcodecs.cpp.
◆ decode() [1/2]
|
virtual |
Convenience wrapper that can be used for small chunks of data when you can provide a large enough buffer.
The default implementation creates a Decoder and uses it.
Decodes a chunk of bytes starting at scursor
and extending to send
into the buffer described by dcursor
and dend
.
This function doesn't support chaining of blocks. The returned block cannot be added to, but you don't need to finalize it, too.
Example usage (in
contains the input data):
KCodecs::Codec *codec = KCodecs::Codec::codecForName("base64"); if (!codec) { qFatal() << "no base64 codec found!?"; } QByteArray out(in.size()); // good guess for any encoding... QByteArray::Iterator iit = in.begin(); QByteArray::Iterator oit = out.begin(); if (!codec->decode(iit, in.end(), oit, out.end())) { qDebug() << "output buffer too small"; return; } qDebug() << "Size of decoded data:" << oit - out.begin();
- Parameters
-
scursor is a pointer to the start of the input buffer. send is a pointer to the end of the input buffer. dcursor is a pointer to the start of the output buffer. dend is a pointer to the end of the output buffer. newline whether make new lines using CRLF, or LF (default is LF).
- Returns
- false if the decoded data didn't fit into the output buffer; true otherwise.
Definition at line 617 of file kcodecs.cpp.
◆ decode() [2/2]
QByteArray KCodecs::Codec::decode | ( | QByteArrayView | src, |
NewlineType | newline = NewlineLF ) const |
Even more convenient, but also a bit slower and more memory intensive, since it allocates storage for the worst case and then shrinks the result QByteArray to the actual size again.
For use with small src
.
- Parameters
-
src is the data to decode. newline whether make new lines using CRLF, or LF (default is LF).
Definition at line 594 of file kcodecs.cpp.
◆ encode() [1/2]
|
virtual |
Convenience wrapper that can be used for small chunks of data when you can provide a large enough buffer.
The default implementation creates an Encoder and uses it.
Encodes a chunk of bytes starting at scursor
and extending to send
into the buffer described by dcursor
and dend
.
This function doesn't support chaining of blocks. The returned block cannot be added to, but you don't need to finalize it, too.
Example usage (in
contains the input data):
KCodecs::Codec *codec = KCodecs::Codec::codecForName("base64"); if (!codec) { qFatal() << "no base64 codec found!?"; } QByteArray out(in.size() * 1.4); // crude maximal size of b64 encoding QByteArray::Iterator iit = in.begin(); QByteArray::Iterator oit = out.begin(); if (!codec->encode(iit, in.end(), oit, out.end())) { qDebug() << "output buffer too small"; return; } qDebug() << "Size of encoded data:" << oit - out.begin();
- Parameters
-
scursor is a pointer to the start of the input buffer. send is a pointer to the end of the input buffer. dcursor is a pointer to the start of the output buffer. dend is a pointer to the end of the output buffer. newline whether make new lines using CRLF, or LF (default is LF).
- Returns
- false if the encoded data didn't fit into the output buffer; true otherwise.
Definition at line 545 of file kcodecs.cpp.
◆ encode() [2/2]
QByteArray KCodecs::Codec::encode | ( | QByteArrayView | src, |
NewlineType | newline = NewlineLF ) const |
Even more convenient, but also a bit slower and more memory intensive, since it allocates storage for the worst case and then shrinks the result QByteArray to the actual size again.
For use with small src
.
- Parameters
-
src is the data to encode. newline whether make new lines using CRLF, or LF (default is LF).
Definition at line 571 of file kcodecs.cpp.
◆ makeDecoder()
|
pure virtual |
Creates the decoder for the codec.
- Returns
- a pointer to an instance of the codec's decoder.
Implemented in KCodecs::Base64Codec, KCodecs::QuotedPrintableCodec, KCodecs::Rfc2047QEncodingCodec, KCodecs::Rfc2231EncodingCodec, and KCodecs::UUCodec.
◆ makeEncoder()
|
pure virtual |
Creates the encoder for the codec.
- Returns
- a pointer to an instance of the codec's encoder.
Implemented in KCodecs::Base64Codec, KCodecs::QuotedPrintableCodec, KCodecs::Rfc2047BEncodingCodec, KCodecs::Rfc2047QEncodingCodec, KCodecs::Rfc2231EncodingCodec, and KCodecs::UUCodec.
◆ maxDecodedSizeFor()
|
pure virtual |
Computes the maximum size, in characters, needed for the deccoding.
- Parameters
-
insize is the number of input characters to be decoded. newline whether make new lines using CRLF, or LF (default is LF).
- Returns
- the maximum number of characters in the decoding.
Implemented in KCodecs::Base64Codec, KCodecs::QuotedPrintableCodec, KCodecs::Rfc2047BEncodingCodec, KCodecs::Rfc2047QEncodingCodec, KCodecs::Rfc2231EncodingCodec, and KCodecs::UUCodec.
◆ maxEncodedSizeFor()
|
pure virtual |
Computes the maximum size, in characters, needed for the encoding.
- Parameters
-
insize is the number of input characters to be encoded. newline whether make new lines using CRLF, or LF (default is LF).
- Returns
- the maximum number of characters in the encoding.
Implemented in KCodecs::Base64Codec, KCodecs::QuotedPrintableCodec, KCodecs::Rfc2047BEncodingCodec, KCodecs::Rfc2047QEncodingCodec, KCodecs::Rfc2231EncodingCodec, and KCodecs::UUCodec.
◆ name()
|
pure virtual |
Returns the name of the encoding.
Guaranteed to be lowercase.
Implemented in KCodecs::Base64Codec, KCodecs::QuotedPrintableCodec, KCodecs::Rfc2047BEncodingCodec, KCodecs::Rfc2047QEncodingCodec, KCodecs::Rfc2231EncodingCodec, and KCodecs::UUCodec.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:59:08 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.