KComboBox
#include <KComboBox>
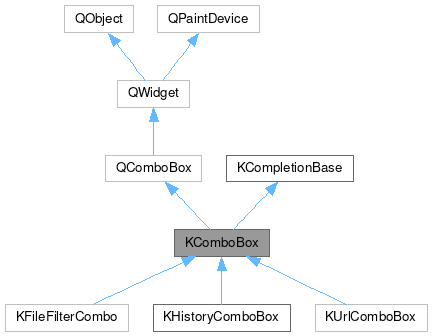
Signals | |
void | aboutToShowContextMenu (QMenu *contextMenu) |
void | completion (const QString &) |
void | completionModeChanged (KCompletion::CompletionMode) |
void | returnPressed (const QString &text) |
void | substringCompletion (const QString &) |
void | textRotation (KCompletionBase::KeyBindingType) |
Public Slots | |
void | rotateText (KCompletionBase::KeyBindingType type) |
void | setCompletedItems (const QStringList &items, bool autoSuggest=true) override |
void | setCompletedText (const QString &) override |
void | setCurrentItem (const QString &item, bool insert=false, int index=-1) |
Public Member Functions | |
KComboBox (bool rw, QWidget *parent=nullptr) | |
KComboBox (QWidget *parent=nullptr) | |
~KComboBox () override | |
void | addUrl (const QIcon &icon, const QUrl &url) |
void | addUrl (const QUrl &url) |
bool | autoCompletion () const |
void | changeUrl (int index, const QIcon &icon, const QUrl &url) |
void | changeUrl (int index, const QUrl &url) |
KCompletionBox * | completionBox (bool create=true) |
bool | contains (const QString &text) const |
QMenu * | contextMenu () const |
int | cursorPosition () const |
void | insertUrl (int index, const QIcon &icon, const QUrl &url) |
void | insertUrl (int index, const QUrl &url) |
QSize | minimumSizeHint () const override |
virtual void | setAutoCompletion (bool autocomplete) |
void | setEditable (bool editable) |
void | setEditUrl (const QUrl &url) |
virtual void | setLineEdit (QLineEdit *) |
void | setTrapReturnKey (bool trap) |
bool | trapReturnKey () const |
bool | urlDropsEnabled () const |
![]() | |
QComboBox (QWidget *parent) | |
void | activated (int index) |
void | addItem (const QIcon &icon, const QString &text, const QVariant &userData) |
void | addItem (const QString &text, const QVariant &userData) |
void | addItems (const QStringList &texts) |
void | clear () |
void | clearEditText () |
QCompleter * | completer () const const |
int | count () const const |
QVariant | currentData (int role) const const |
int | currentIndex () const const |
void | currentIndexChanged (int index) |
QString | currentText () const const |
void | currentTextChanged (const QString &text) |
bool | duplicatesEnabled () const const |
void | editTextChanged (const QString &text) |
virtual bool | event (QEvent *event) override |
int | findData (const QVariant &data, int role, Qt::MatchFlags flags) const const |
int | findText (const QString &text, Qt::MatchFlags flags) const const |
bool | hasFrame () const const |
virtual void | hidePopup () |
void | highlighted (int index) |
QSize | iconSize () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const override |
void | insertItem (int index, const QIcon &icon, const QString &text, const QVariant &userData) |
void | insertItem (int index, const QString &text, const QVariant &userData) |
void | insertItems (int index, const QStringList &list) |
InsertPolicy | insertPolicy () const const |
void | insertSeparator (int index) |
bool | isEditable () const const |
QVariant | itemData (int index, int role) const const |
QAbstractItemDelegate * | itemDelegate () const const |
QIcon | itemIcon (int index) const const |
QString | itemText (int index) const const |
QLineEdit * | lineEdit () const const |
int | maxCount () const const |
int | maxVisibleItems () const const |
int | minimumContentsLength () const const |
QAbstractItemModel * | model () const const |
int | modelColumn () const const |
QString | placeholderText () const const |
void | removeItem (int index) |
QModelIndex | rootModelIndex () const const |
void | setCompleter (QCompleter *completer) |
void | setCurrentIndex (int index) |
void | setCurrentText (const QString &text) |
void | setDuplicatesEnabled (bool enable) |
void | setEditable (bool editable) |
void | setEditText (const QString &text) |
void | setFrame (bool) |
void | setIconSize (const QSize &size) |
void | setInsertPolicy (InsertPolicy policy) |
void | setItemData (int index, const QVariant &value, int role) |
void | setItemDelegate (QAbstractItemDelegate *delegate) |
void | setItemIcon (int index, const QIcon &icon) |
void | setItemText (int index, const QString &text) |
void | setLineEdit (QLineEdit *edit) |
void | setMaxCount (int max) |
void | setMaxVisibleItems (int maxItems) |
void | setMinimumContentsLength (int characters) |
virtual void | setModel (QAbstractItemModel *model) |
void | setModelColumn (int visibleColumn) |
void | setPlaceholderText (const QString &placeholderText) |
void | setRootModelIndex (const QModelIndex &index) |
void | setSizeAdjustPolicy (SizeAdjustPolicy policy) |
void | setValidator (const QValidator *validator) |
void | setView (QAbstractItemView *itemView) |
virtual void | showPopup () |
SizeAdjustPolicy | sizeAdjustPolicy () const const |
virtual QSize | sizeHint () const const override |
void | textActivated (const QString &text) |
void | textHighlighted (const QString &text) |
const QValidator * | validator () const const |
QAbstractItemView * | view () const const |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
![]() | |
KCompletionBase () | |
virtual | ~KCompletionBase () |
KCompletion::CompletionMode | completionMode () const |
KCompletion * | completionObject (bool handleSignals=true) |
KCompletion * | compObj () const |
bool | emitSignals () const |
bool | handleSignals () const |
bool | isCompletionObjectAutoDeleted () const |
QList< QKeySequence > | keyBinding (KeyBindingType item) const |
void | setAutoDeleteCompletionObject (bool autoDelete) |
virtual void | setCompletedItems (const QStringList &items, bool autoSuggest=true)=0 |
virtual void | setCompletedText (const QString &text)=0 |
virtual void | setCompletionMode (KCompletion::CompletionMode mode) |
virtual void | setCompletionObject (KCompletion *completionObject, bool handleSignals=true) |
void | setEmitSignals (bool emitRotationSignals) |
void | setEnableSignals (bool enable) |
virtual void | setHandleSignals (bool handle) |
bool | setKeyBinding (KeyBindingType item, const QList< QKeySequence > &key) |
void | useGlobalKeyBindings () |
Protected Slots | |
virtual void | makeCompletion (const QString &) |
Protected Member Functions | |
KCOMPLETION_NO_EXPORT | KComboBox (KComboBoxPrivate &dd, QWidget *parent) |
virtual void | setCompletedText (const QString &text, bool marked) |
![]() | |
virtual void | changeEvent (QEvent *e) override |
virtual void | contextMenuEvent (QContextMenuEvent *e) override |
virtual void | focusInEvent (QFocusEvent *e) override |
virtual void | focusOutEvent (QFocusEvent *e) override |
virtual void | hideEvent (QHideEvent *e) override |
virtual void | initStyleOption (QStyleOptionComboBox *option) const const |
virtual void | inputMethodEvent (QInputMethodEvent *e) override |
virtual void | keyPressEvent (QKeyEvent *e) override |
virtual void | keyReleaseEvent (QKeyEvent *e) override |
virtual void | mousePressEvent (QMouseEvent *e) override |
virtual void | mouseReleaseEvent (QMouseEvent *e) override |
virtual void | paintEvent (QPaintEvent *e) override |
virtual void | resizeEvent (QResizeEvent *e) override |
virtual void | showEvent (QShowEvent *e) override |
virtual void | wheelEvent (QWheelEvent *e) override |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
bool | focusPreviousChild () |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
KCompletionBase * | delegate () const |
KeyBindingMap | keyBindingMap () const |
void | setDelegate (KCompletionBase *delegate) |
void | setKeyBindingMap (KeyBindingMap keyBindingMap) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
std::unique_ptr< KComboBoxPrivate > const | d_ptr |
Additional Inherited Members | |
![]() | |
enum | InsertPolicy |
enum | SizeAdjustPolicy |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
![]() | |
typedef QMap< KeyBindingType, QList< QKeySequence > > | KeyBindingMap |
enum | KeyBindingType { TextCompletion , PrevCompletionMatch , NextCompletionMatch , SubstringCompletion } |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
AdjustToContents | |
AdjustToContentsOnFirstShow | |
AdjustToMinimumContentsLengthWithIcon | |
InsertAfterCurrent | |
InsertAlphabetically | |
InsertAtBottom | |
InsertAtCurrent | |
InsertAtTop | |
InsertBeforeCurrent | |
NoInsert | |
![]() | |
DrawChildren | |
DrawWindowBackground | |
IgnoreMask | |
typedef | RenderFlags |
![]() | |
typedef | QObjectList |
![]() | |
PdmDepth | |
PdmDevicePixelRatio | |
PdmDevicePixelRatioScaled | |
PdmDpiX | |
PdmDpiY | |
PdmHeight | |
PdmHeightMM | |
PdmNumColors | |
PdmPhysicalDpiX | |
PdmPhysicalDpiY | |
PdmWidth | |
PdmWidthMM | |
Detailed Description
A combo box with completion support.
This widget inherits from QComboBox and implements the following additional features:
- a completion object that provides both automatic and manual text completion as well as text rotation
- configurable key bindings to activate these features
- a popup menu item that can be used to allow the user to change the text completion mode on the fly.
To support these additional features, KComboBox emits a few additional signals such as completion(const QString&) and textRotation(KeyBindingType).
The completion signal can be connected to a slot that will assist the user in filling out the remaining text while the rotation signal can be used to traverse through all possible matches whenever text completion results in multiple matches. Additionally, the returnPressed(const QString &) signal is emitted when the user presses the Return or Enter key.
KCombobox by default creates a completion object when you invoke the completionObject(bool) member function for the first time or explicitly use setCompletionObject(KCompletion*, bool) to assign your own completion object. Additionally, to make this widget more functional, KComboBox will by default handle text rotation and completion events internally whenever a completion object is created through either one of the methods mentioned above. If you do not need this functionality, simply use KCompletionBase::setHandleSignals(bool) or alternatively set the boolean parameter in the setCompletionObject()
call to false
.
Beware: The completion object can be deleted on you, especially if a call such as setEditable(false) is made. Store the pointer at your own risk, and consider using QPointer<KCompletion>.
The default key bindings for completion and rotation are determined from the global settings in KStandardShortcut. These values, however, can be overridden locally by invoking KCompletionBase::setKeyBinding(). The values can easily be reverted back to the default settings by calling useGlobalSettings(). An alternate method would be to default individual key bindings by using setKeyBinding() with the default second argument.
A non-editable combo box only has one completion mode, CompletionAuto
. Unlike an editable combo box, the CompletionAuto mode works by matching any typed key with the first letter of entries in the combo box. Please note that if you call setEditable(false) to change an editable combo box to a non-editable one, the text completion object associated with the combo box will no longer exist unless you created the completion object yourself and assigned it to this widget or you called setAutoDeleteCompletionObject(false). In other words do not do the following:
A read-only KComboBox will have the same background color as a disabled KComboBox, but its foreground color will be the one used for the editable mode. This differs from QComboBox's implementation and is done to give visual distinction between the three different modes: disabled, read-only, and editable.
Usage
To enable the basic completion feature:
To use your own completion object:
Miscellaneous function calls:
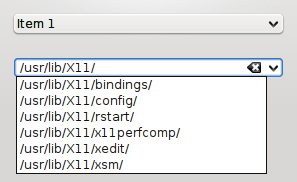
Definition at line 135 of file kcombobox.h.
Property Documentation
◆ autoCompletion
|
readwrite |
Definition at line 138 of file kcombobox.h.
◆ trapReturnKey
|
readwrite |
Definition at line 139 of file kcombobox.h.
Constructor & Destructor Documentation
◆ KComboBox() [1/3]
|
explicit |
Constructs a read-only (or rather select-only) combo box.
- Parameters
-
parent The parent object of this widget
Definition at line 38 of file kcombobox.cpp.
◆ KComboBox() [2/3]
|
explicit |
Constructs an editable or read-only combo box.
- Parameters
-
rw When true
, widget will be editable.parent The parent object of this widget.
Definition at line 52 of file kcombobox.cpp.
◆ ~KComboBox()
|
override |
Destructor.
Definition at line 58 of file kcombobox.cpp.
◆ KComboBox() [3/3]
|
protected |
Definition at line 43 of file kcombobox.cpp.
Member Function Documentation
◆ aboutToShowContextMenu
|
signal |
Emitted before the context menu is displayed.
The signal allows you to add your own entries into the context menu. Note that you must not store the pointer to the QPopupMenu since it is created and deleted on demand. Otherwise, you can crash your app.
- Parameters
-
contextMenu the context menu about to be displayed
◆ addUrl() [1/2]
Appends url
with the icon
to the combo box.
QUrl::toDisplayString() is used so that the url is properly decoded for displaying.
Definition at line 177 of file kcombobox.cpp.
◆ addUrl() [2/2]
void KComboBox::addUrl | ( | const QUrl & | url | ) |
Appends url
to the combo box.
QUrl::toDisplayString() is used so that the url is properly decoded for displaying.
Definition at line 172 of file kcombobox.cpp.
◆ autoCompletion()
bool KComboBox::autoCompletion | ( | ) | const |
Reimplemented from QComboBox.
Returns true
if the current completion mode is set to automatic. See its more comprehensive replacement completionMode().
- Returns
true
when completion mode is automatic.
Definition at line 98 of file kcombobox.cpp.
◆ changeUrl() [1/2]
Replaces the item at position index
with url
and icon
.
QUrl::toDisplayString() is used so that the url is properly decoded for displaying.
Definition at line 197 of file kcombobox.cpp.
◆ changeUrl() [2/2]
void KComboBox::changeUrl | ( | int | index, |
const QUrl & | url ) |
Replaces the item at position index
with url
.
QUrl::toDisplayString() is used so that the url is properly decoded for displaying.
Definition at line 192 of file kcombobox.cpp.
◆ completion
|
signal |
Emitted when the completion key is pressed.
The argument is the current text being edited.
Note that this signal is not available when the widget is non-editable or the completion mode is set to CompletionNone
.
◆ completionBox()
KCompletionBox * KComboBox::completionBox | ( | bool | create = true | ) |
This method will create a completion box by calling KLineEdit::completionBox, if none is there yet.
- Parameters
-
create Set this to false if you don't want the box to be created i.e. to test if it is available.
- Returns
- the completion box that is used in completion mode CompletionPopup and CompletionPopupAuto.
Definition at line 211 of file kcombobox.cpp.
◆ completionModeChanged
|
signal |
Emitted whenever the completion mode is changed by the user through the context menu.
◆ contains()
bool KComboBox::contains | ( | const QString & | text | ) | const |
Convenience method which iterates over all items and checks if any of them is equal to text
.
If text
is an empty string, false
is returned.
- Returns
true
if an item with the stringtext
is in the combo box.
Definition at line 64 of file kcombobox.cpp.
◆ contextMenu()
QMenu * KComboBox::contextMenu | ( | ) | const |
Pointer to KLineEdit's context menu, or nullptr if it does not exist at the given moment.
- Since
- 5.78
Definition at line 300 of file kcombobox.cpp.
◆ cursorPosition()
int KComboBox::cursorPosition | ( | ) | const |
Returns the current cursor position.
This method always returns a -1 if the combo box is not editable (read-only).
- Returns
- Current cursor position.
Definition at line 79 of file kcombobox.cpp.
◆ insertUrl() [1/2]
Inserts url
with the icon
at position index
into the combo box.
QUrl::toDisplayString() is used so that the url is properly decoded for displaying.
Definition at line 187 of file kcombobox.cpp.
◆ insertUrl() [2/2]
void KComboBox::insertUrl | ( | int | index, |
const QUrl & | url ) |
Inserts url
at position index
into the combo box.
QUrl::toDisplayString() is used so that the url is properly decoded for displaying.
Definition at line 182 of file kcombobox.cpp.
◆ makeCompletion
|
protectedvirtualslot |
Completes text according to the completion mode.
- Note
- This method is not invoked if the completion mode is set to
CompletionNone
. Also if the mode is set toCompletionShell
and multiple matches are found, this method will complete the text to the first match with a beep to indicate that there are more matches. Then any successive completion key event iterates through the remaining matches. This way the rotation functionality is left to iterate through the list as usual.
Definition at line 125 of file kcombobox.cpp.
◆ minimumSizeHint()
|
overridevirtual |
Reimplemented from QComboBox.
Definition at line 220 of file kcombobox.cpp.
◆ returnPressed
|
signal |
Emitted when the user presses the Return or Enter key.
The argument is the current text being edited.
- Note
- This signal is only emitted when the widget is editable.
◆ rotateText
|
slot |
Iterates through all possible matches of the completed text or the history list.
Depending on the value of the argument, this function either iterates through the history list of this widget or all the possible matches in whenever multiple matches result from a text completion request. Note that the all-possible-match iteration will not work if there are no previous matches, i.e. no text has been completed and the *nix shell history list rotation is only available if the insertion policy for this widget is set either QComobBox::AtTop
or QComboBox::AtBottom
. For other insertion modes whatever has been typed by the user when the rotation event was initiated will be lost.
- Parameters
-
type The key binding invoked.
Definition at line 141 of file kcombobox.cpp.
◆ setAutoCompletion()
|
virtual |
Reimplemented from QComboBox.
If true
, the completion mode will be set to automatic. Otherwise, it is defaulted to the global setting. This method has been replaced by the more comprehensive setCompletionMode().
- Parameters
-
autocomplete Flag to enable/disable automatic completion mode.
Definition at line 84 of file kcombobox.cpp.
◆ setCompletedItems
|
overrideslot |
Sets items
into the completion box if completionMode() is CompletionPopup.
The popup will be shown immediately.
Definition at line 203 of file kcombobox.cpp.
◆ setCompletedText [1/2]
|
overrideslot |
Sets the completed text in the line edit appropriately.
This function is an implementation for KCompletionBase::setCompletedText.
Definition at line 117 of file kcombobox.cpp.
◆ setCompletedText() [2/2]
|
protectedvirtual |
This function sets the line edit text and highlights the text appropriately if the boolean value is set to true.
- Parameters
-
text The text to be set in the line edit marked Whether the text inserted should be highlighted
Definition at line 109 of file kcombobox.cpp.
◆ setCurrentItem
|
slot |
Selects the first item that matches item
.
If there is no such item, it is inserted at position index
if insert
is true. Otherwise, no item is selected.
Definition at line 305 of file kcombobox.cpp.
◆ setEditable()
void KComboBox::setEditable | ( | bool | editable | ) |
Reimplemented so that setEditable(true) creates a KLineEdit instead of QLineEdit.
Note that QComboBox::setEditable is not virtual, so do not use a KComboBox in a QComboBox pointer.
Definition at line 329 of file kcombobox.cpp.
◆ setEditUrl()
void KComboBox::setEditUrl | ( | const QUrl & | url | ) |
Sets url
into the edit field of the combo box.
It uses QUrl::toDisplayString() so that the url is properly decoded for displaying.
Definition at line 167 of file kcombobox.cpp.
◆ setLineEdit()
|
virtual |
Reimplemented for internal reasons.
API remains unaffected. Note that QComboBox::setLineEdit is not virtual in Qt4, do not use a KComboBox in a QComboBox pointer.
NOTE: Only editable combo boxes can have a line editor. As such any attempt to assign a line edit to a non-editable combo box will simply be ignored.
Definition at line 237 of file kcombobox.cpp.
◆ setTrapReturnKey()
void KComboBox::setTrapReturnKey | ( | bool | trap | ) |
By default, KComboBox recognizes Key_Return and Key_Enter and emits the returnPressed(const QString &) signal, but it also lets the event pass, for example causing a dialog's default button to be called.
Call this method with trap
set to true to make KComboBox stop these events. The signals will still be emitted of course.
- Note
- This only affects editable combo boxes.
- See also
- setTrapReturnKey()
Definition at line 149 of file kcombobox.cpp.
◆ substringCompletion
|
signal |
Emitted when the shortcut for substring completion is pressed.
◆ textRotation
|
signal |
Emitted when the text rotation key bindings are pressed.
The argument indicates which key binding was pressed. In this case this can be either one of four values: PrevCompletionMatch
, NextCompletionMatch
, RotateUp
or RotateDown
.
Note that this signal is not emitted if the completion mode is set to CompletionNone.
- See also
- KCompletionBase::setKeyBinding() for details
◆ trapReturnKey()
bool KComboBox::trapReturnKey | ( | ) | const |
- Returns
true
if Key_Return or Key_Enter input events will be stopped orfalse
if they will be propagated.
- See also
- setTrapReturnKey()
Definition at line 161 of file kcombobox.cpp.
◆ urlDropsEnabled()
bool KComboBox::urlDropsEnabled | ( | ) | const |
Returns true
when decoded URL drops are enabled.
Definition at line 103 of file kcombobox.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 461 of file kcombobox.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:53:18 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.