KCompletionMatches
#include <KCompletionMatches>
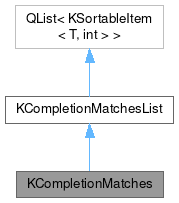
Public Member Functions | |
KCompletionMatches (bool sort) | |
KCompletionMatches (const KCompletionMatches &) | |
KCompletionMatches (const KCompletionMatchesWrapper &matches) | |
~KCompletionMatches () | |
QStringList | list (bool sort=true) const |
KCompletionMatches & | operator= (const KCompletionMatches &) |
void | removeDuplicates () |
bool | sorting () const |
![]() | |
void | insert (int i, const QString &t) |
void | insert (int i, const QString &t) |
QString & | operator[] (int i) |
QString & | operator[] (int i) |
const QString & | operator[] (int i) const |
const QString & | operator[] (int i) const |
void | sort () |
void | sort () |
![]() | |
QList (const QList< T > &other) | |
QList (InputIterator first, InputIterator last) | |
QList (QList< T > &&other) | |
QList (qsizetype size) | |
QList (qsizetype size, parameter_type value) | |
QList (std::initializer_list< T > args) | |
void | append (const QList< T > &value) |
void | append (parameter_type value) |
void | append (QList< T > &&value) |
void | append (rvalue_ref value) |
const_reference | at (qsizetype i) const const |
reference | back () |
const_reference | back () const const |
iterator | begin () |
const_iterator | begin () const const |
qsizetype | capacity () const const |
const_iterator | cbegin () const const |
const_iterator | cend () const const |
void | clear () |
const_iterator | constBegin () const const |
const_pointer | constData () const const |
const_iterator | constEnd () const const |
const T & | constFirst () const const |
const T & | constLast () const const |
bool | contains (const AT &value) const const |
qsizetype | count () const const |
qsizetype | count (const AT &value) const const |
const_reverse_iterator | crbegin () const const |
const_reverse_iterator | crend () const const |
pointer | data () |
const_pointer | data () const const |
iterator | emplace (const_iterator before, Args &&... args) |
iterator | emplace (qsizetype i, Args &&... args) |
reference | emplace_back (Args &&... args) |
reference | emplaceBack (Args &&... args) |
bool | empty () const const |
iterator | end () |
const_iterator | end () const const |
bool | endsWith (parameter_type value) const const |
iterator | erase (const_iterator begin, const_iterator end) |
iterator | erase (const_iterator pos) |
qsizetype | erase (QList< T > &list, const AT &t) |
qsizetype | erase_if (QList< T > &list, Predicate pred) |
QList< T > & | fill (parameter_type value, qsizetype size) |
T & | first () |
const T & | first () const const |
QList< T > | first (qsizetype n) const const |
reference | front () |
const_reference | front () const const |
qsizetype | indexOf (const AT &value, qsizetype from) const const |
iterator | insert (const_iterator before, parameter_type value) |
iterator | insert (const_iterator before, qsizetype count, parameter_type value) |
iterator | insert (const_iterator before, rvalue_ref value) |
iterator | insert (qsizetype i, parameter_type value) |
iterator | insert (qsizetype i, qsizetype count, parameter_type value) |
iterator | insert (qsizetype i, rvalue_ref value) |
bool | isEmpty () const const |
T & | last () |
const T & | last () const const |
QList< T > | last (qsizetype n) const const |
qsizetype | lastIndexOf (const AT &value, qsizetype from) const const |
qsizetype | length () const const |
QList< T > | mid (qsizetype pos, qsizetype length) const const |
void | move (qsizetype from, qsizetype to) |
bool | operator!= (const QList< T > &other) const const |
QList< T > | operator+ (const QList< T > &other) && |
QList< T > | operator+ (const QList< T > &other) const &const |
QList< T > | operator+ (QList< T > &&other) && |
QList< T > | operator+ (QList< T > &&other) const &const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (parameter_type value) |
QList< T > & | operator+= (QList< T > &&other) |
QList< T > & | operator+= (rvalue_ref value) |
bool | operator< (const QList< T > &other) const const |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator<< (parameter_type value) |
QDataStream & | operator<< (QDataStream &out, const QList< T > &list) |
QList< T > & | operator<< (QList< T > &&other) |
QList< T > & | operator<< (rvalue_ref value) |
bool | operator<= (const QList< T > &other) const const |
QList< T > & | operator= (const QList< T > &other) |
QList< T > & | operator= (QList< T > &&other) |
QList< T > & | operator= (std::initializer_list< T > args) |
bool | operator== (const QList< T > &other) const const |
bool | operator> (const QList< T > &other) const const |
bool | operator>= (const QList< T > &other) const const |
QDataStream & | operator>> (QDataStream &in, QList< T > &list) |
reference | operator[] (qsizetype i) |
const_reference | operator[] (qsizetype i) const const |
void | pop_back () |
void | pop_front () |
void | prepend (parameter_type value) |
void | prepend (rvalue_ref value) |
void | push_back (parameter_type value) |
void | push_back (rvalue_ref value) |
void | push_front (parameter_type value) |
void | push_front (rvalue_ref value) |
size_t | qHash (const QList< T > &key, size_t seed) |
reverse_iterator | rbegin () |
const_reverse_iterator | rbegin () const const |
void | remove (qsizetype i, qsizetype n) |
qsizetype | removeAll (const AT &t) |
void | removeAt (qsizetype i) |
void | removeFirst () |
qsizetype | removeIf (Predicate pred) |
void | removeLast () |
bool | removeOne (const AT &t) |
reverse_iterator | rend () |
const_reverse_iterator | rend () const const |
void | replace (qsizetype i, parameter_type value) |
void | replace (qsizetype i, rvalue_ref value) |
void | reserve (qsizetype size) |
void | resize (qsizetype size) |
void | resize (qsizetype size, parameter_type c) |
void | shrink_to_fit () |
qsizetype | size () const const |
QList< T > | sliced (qsizetype pos) const const |
QList< T > | sliced (qsizetype pos, qsizetype n) const const |
void | squeeze () |
bool | startsWith (parameter_type value) const const |
void | swap (QList< T > &other) |
void | swapItemsAt (qsizetype i, qsizetype j) |
T | takeAt (qsizetype i) |
value_type | takeFirst () |
value_type | takeLast () |
QList< T > | toList () const const |
QList< T > | toVector () const const |
T | value (qsizetype i) const const |
T | value (qsizetype i, parameter_type defaultValue) const const |
Additional Inherited Members | |
![]() | |
QList< T > | fromList (const QList< T > &list) |
QList< T > | fromVector (const QList< T > &list) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | const_reverse_iterator |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | parameter_type |
typedef | pointer |
typedef | reference |
typedef | reverse_iterator |
typedef | rvalue_ref |
typedef | size_type |
typedef | value_type |
Detailed Description
This structure is returned by KCompletion::allWeightedMatches().
It also keeps the weight of the matches, allowing you to modify some matches or merge them with matches from another call to allWeightedMatches(), and sort the matches after that in order to have the matches ordered correctly.
Example (a simplified example of what Konqueror's completion does):
List for keeping matches returned from KCompletion
Definition at line 41 of file kcompletionmatches.h.
Constructor & Destructor Documentation
◆ KCompletionMatches() [1/3]
KCompletionMatches::KCompletionMatches | ( | bool | sort | ) |
Default constructor.
- Parameters
-
sort if false, the matches won't be sorted before the conversion, use only if you're sure the sorting is not needed
Definition at line 47 of file kcompletionmatches.cpp.
◆ KCompletionMatches() [2/3]
KCompletionMatches::KCompletionMatches | ( | const KCompletionMatches & | o | ) |
copy constructor.
Definition at line 28 of file kcompletionmatches.cpp.
◆ KCompletionMatches() [3/3]
KCompletionMatches::KCompletionMatches | ( | const KCompletionMatchesWrapper & | matches | ) |
Definition at line 52 of file kcompletionmatches.cpp.
◆ ~KCompletionMatches()
KCompletionMatches::~KCompletionMatches | ( | ) |
default destructor.
Definition at line 66 of file kcompletionmatches.cpp.
Member Function Documentation
◆ list()
QStringList KCompletionMatches::list | ( | bool | sort = true | ) | const |
Returns the matches as a QStringList.
- Parameters
-
sort if false, the matches won't be sorted before the conversion, use only if you're sure the sorting is not needed
- Returns
- the list of matches
Definition at line 70 of file kcompletionmatches.cpp.
◆ operator=()
KCompletionMatches & KCompletionMatches::operator= | ( | const KCompletionMatches & | o | ) |
assignment operator.
Definition at line 35 of file kcompletionmatches.cpp.
◆ removeDuplicates()
void KCompletionMatches::removeDuplicates | ( | ) |
Removes duplicate matches.
Needed only when you merged several matches results and there's a possibility of duplicates.
Definition at line 91 of file kcompletionmatches.cpp.
◆ sorting()
bool KCompletionMatches::sorting | ( | ) | const |
If sorting() returns false, the matches aren't sorted by their weight, even if true is passed to list().
- Returns
- true if the matches won't be sorted
Definition at line 85 of file kcompletionmatches.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:19 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.