KDBusService
#include <KDBusService>
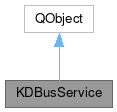
Public Types | |
enum | StartupOption { Unique = 1 , Multiple = 2 , NoExitOnFailure = 4 , Replace = 8 } |
typedef QFlags< StartupOption > | StartupOptions |
Signals | |
void | activateActionRequested (const QString &actionName, const QVariant ¶meter) |
void | activateRequested (const QStringList &arguments, const QString &workingDirectory) |
void | openRequested (const QList< QUrl > &uris) |
Public Slots | |
void | unregister () |
Public Member Functions | |
KDBusService (StartupOptions options=Multiple, QObject *parent=nullptr) | |
~KDBusService () override | |
QString | errorMessage () const |
bool | isRegistered () const |
QString | serviceName () const |
void | setExitValue (int value) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
KDBusService takes care of registering the current process with D-Bus.
This registers the application at a predictable location on D-Bus, registers the QCoreApplication (or subclass) object at /MainApplication, and assists in implementing the application side of D-Bus activation from the Desktop Entry Specification.
An application can either work in Multiple mode or Unique mode.
In Multiple mode, the application can be launched many times. The service name in the D-Bus registration will contain the PID to distinguish the various instances; for example: org.kde.konqueror-12345
.
In Unique mode, only one instance of this application can ever run. The first instance of the application registers with D-Bus without the PID, and any attempt to run the application again will cause the activateRequested() signal to be emitted in the already-running instance; the duplicate instance will then quit. The exit value can be set by the already running instance with setExitValue(), the default value is 0
.
Unique-mode applications should usually delay parsing command-line arguments until after creating a KDBusService object; that way they know they are the original instance of the application.
Applications that set the D-Bus activation entry (DBusActivatable=true) in their desktop files will use Unique mode and connect to the signals emitted by this class. Note that the D-Bus interface is exported for Multiple-mode applications as well, so it also makes sense for such applications to connect to the signals emitted by this class.
- Note
- In order to avoid a race, the application should export its objects to D-Bus before allowing the event loop to run (for example, by calling QCoreApplication::exec()). Otherwise, the application will appear on the bus before its objects are accessible via D-Bus, which could be a problem for other applications or scripts which start the application in order to talk D-Bus to it immediately.
Example usage:
- Since
- 5.0
Definition at line 80 of file kdbusservice.h.
Member Typedef Documentation
◆ StartupOptions
typedef QFlags< StartupOption > KDBusService::StartupOptions |
Stores a combination of StartupOption values.
Definition at line 128 of file kdbusservice.h.
Member Enumeration Documentation
◆ StartupOption
Options to control the behaviour of KDBusService.
- See also
- StartupOptions
Enumerator | |
---|---|
Unique | Indicates that only one instance of this application should ever exist. Cannot be combined with |
Multiple | Indicates that multiple instances of the application may exist. Cannot be combined with |
NoExitOnFailure | Indicates that the application should not exit if it failed to register with D-Bus. If not set, KDBusService will quit the application if it failed to register the service with D-Bus or a |
Replace | Indicates that if there's already a unique service running, to be quit and replaced with our own. If exported, it will try first quitting the service calling
|
Definition at line 89 of file kdbusservice.h.
Constructor & Destructor Documentation
◆ KDBusService()
|
explicit |
Tries to register the current process to D-Bus at an address based on the application name and organization domain.
The D-Bus service name is the reversed organization domain, followed by the application name. If options
includes the Multiple
flag, the application PID will be appended. For example,
will make KDBusService register as org.kde.kuiserver
in Unique
mode, and org.kde.kuiserver-1234
(if the process has PID 1234
) in Multiple
mode.
Definition at line 267 of file kdbusservice.cpp.
◆ ~KDBusService()
|
overridedefault |
Destroys this object (but does not unregister the application).
Deleting this object before unregister() could confuse clients, who will see the service on the bus but will be unable to use the activation methods.
Member Function Documentation
◆ activateActionRequested
|
signal |
Signals that an application action should be triggered.
This signal is emitted to request handling of the respective method of the D-Bus activation. For GUI applications, the signal handler also needs to deal with any platform-specific startup ids and make sure the mainwindow is shown as well as request its activation from the window manager. See documentation of activateRequested(const QStringList &arguments, const QString &) for details.
See the desktop entry specification for more information about action activation.
◆ activateRequested
|
signal |
Signals that the application is to be activated.
If this is a Unique
application, when KDBusService is constructed in subsequent instances of the application (ie: when the executable is run when an instance is already running), it will cause this signal to be emitted in the already-running instance (with the arguments passed to the duplicate instance), and the duplicate instance will then exit.
If this application's desktop file indicates that it supports D-Bus activation (DBusActivatable=true), a command launcher may also call the Activate() D-Bus method to trigger this signal. In this case, args
will be empty.
In single-window applications, the connected signal should typically raise the window.
- Parameters
-
arguments The arguments the executable was called with, starting with the executable file name. See QCoreApplication::arguments(). This can also be empty.
A typical implementation of the signal handler would be:
For GUI applications, the handler also needs to deal with any platform-specific startup ids and make sure the mainwindow is shown as well as request its activation from the window manager. For X11, KDBusService makes the id for the Startup Notification protocol available from QX11Info::nextStartupId(), if there is one. For Wayland, KDBusService provides the token for the XDG Activation protocol in the "XDG_ACTIVATION_TOKEN" environment variable and unsets it again after the signal, if there is one. The util method KWindowSystem::updateStartupId(QWindow *window)
(since KF 5.91) takes care of that. A typical implementation in the signal handler would be:
If you're using the builtin handling of --help
and --version
in QCommandLineParser, you should call parser.process(arguments) before creating the KDBusService instance, since parse() doesn't handle those (and exiting the already-running instance would be wrong anyway).
- See also
- setExitValue()
◆ errorMessage()
QString KDBusService::errorMessage | ( | ) | const |
Returns the error message from the D-Bus registration if it failed.
Note that this is only useful when specifying the option NoExitOnFailure. Otherwise the process has quit by the time you can get a chance to call this.
Definition at line 285 of file kdbusservice.cpp.
◆ isRegistered()
bool KDBusService::isRegistered | ( | ) | const |
Returns true if the D-Bus registration succeeded.
Note that this is only useful when specifying the option NoExitOnFailure. Otherwise, the simple fact that this process is still running indicates that the registration succeeded.
Definition at line 280 of file kdbusservice.cpp.
◆ openRequested
Signals that one or more files should be opened in the application.
This signal is emitted to request handling of the respective method of the D-Bus activation. For GUI applications, the signal handler also needs to deal with any platform-specific startup ids and make sure the mainwindow is shown as well as request its activation from the window manager. See documentation of activateRequested(const QStringList &arguments, const QString &) for details.
- Parameters
-
uris The URLs of the files to open.
◆ serviceName()
QString KDBusService::serviceName | ( | ) | const |
Returns the name of the D-Bus service registered by this class.
Mostly useful when using the option Multiple.
- Since
- 5.33
Definition at line 295 of file kdbusservice.cpp.
◆ setExitValue()
void KDBusService::setExitValue | ( | int | value | ) |
Sets the exit value to be used for a duplicate instance.
If this is a Unique
application, a slot connected to activateRequested() can use this to specify a non-zero exit value for the duplicate instance. This would typically be done if invalid command-line arguments are passed.
Note that this will only work if the signal-slot connection type is Qt::DirectConnection.
- Parameters
-
value The exit value for the duplicate instance.
Definition at line 290 of file kdbusservice.cpp.
◆ unregister
|
slot |
Manually unregister the given serviceName from D-Bus.
Definition at line 300 of file kdbusservice.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:52:34 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.