KGlobalAccel
#include <kglobalaccel.h>
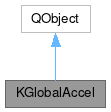
Public Types | |
enum | actionIdFields { ComponentUnique = 0 , ActionUnique = 1 , ComponentFriendly = 2 , ActionFriendly = 3 } |
enum | GlobalShortcutLoading { Autoloading = 0x0 , NoAutoloading = 0x4 } |
enum | MatchType { Equal , Shadows , Shadowed } |
Signals | |
void | globalShortcutActiveChanged (QAction *action, bool active) |
void | globalShortcutChanged (QAction *action, const QKeySequence &seq) |
Public Member Functions | |
QList< QKeySequence > | defaultShortcut (const QAction *action) const |
QList< QKeySequence > | globalShortcut (const QString &componentName, const QString &actionId) const |
bool | hasShortcut (const QAction *action) const |
void | removeAllShortcuts (QAction *action) |
bool | setDefaultShortcut (QAction *action, const QList< QKeySequence > &shortcut, GlobalShortcutLoading loadFlag=Autoloading) |
bool | setShortcut (QAction *action, const QList< QKeySequence > &shortcut, GlobalShortcutLoading loadFlag=Autoloading) |
QList< QKeySequence > | shortcut (const QAction *action) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static bool | cleanComponent (const QString &componentUnique) |
static QList< KGlobalShortcutInfo > | globalShortcutsByKey (const QKeySequence &seq, MatchType type=Equal) |
static bool | isComponentActive (const QString &componentName) |
static bool | isGlobalShortcutAvailable (const QKeySequence &seq, const QString &component=QString()) |
static bool | promptStealShortcutSystemwide (QWidget *parent, const QList< KGlobalShortcutInfo > &shortcuts, const QKeySequence &seq) |
static KGlobalAccel * | self () |
static bool | setGlobalShortcut (QAction *action, const QKeySequence &shortcut) |
static bool | setGlobalShortcut (QAction *action, const QList< QKeySequence > &shortcut) |
static void | stealShortcutSystemwide (const QKeySequence &seq) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Configurable global shortcut support.
KGlobalAccel allows you to have global accelerators that are independent of the focused window. Unlike regular shortcuts, the application's window does not need focus for them to be activated.
- See also
- KKeyChooser
- KKeyDialog
Definition at line 33 of file kglobalaccel.h.
Member Enumeration Documentation
◆ actionIdFields
Index for actionId QStringLists.
Enumerator | |
---|---|
ComponentUnique | Components Unique Name (ID) |
ActionUnique | Actions Unique Name(ID) |
ComponentFriendly | Components Friendly Translated Name. |
ActionFriendly | Actions Friendly Translated Name. |
Definition at line 53 of file kglobalaccel.h.
◆ GlobalShortcutLoading
An enum about global shortcut setter semantics.
Enumerator | |
---|---|
Autoloading | Look up the action in global settings (using its main component's name and text()) and set the shortcut as saved there.
|
NoAutoloading | Prevent autoloading of saved global shortcut for action. |
Definition at line 41 of file kglobalaccel.h.
◆ MatchType
Keysequence match semantics.
Assuming we have an Emacs-style shortcut, for example (Alt+B, Alt+F, Alt+G) already assigned, how a new shortcut is compared depends on which value of the enum is used: Equal
: Exact matching: (Alt+B, Alt+F, Alt+G) Shadows
: Sequence shadowing: (Alt+B, Alt+F), (Alt+F, Alt+G) Shadowed
: Sequence being shadowed: (Alt+B, Alt+F, Alt+G, <any key>), (<any key>, Alt+B, Alt+F, Alt+G)
- Since
- 5.90
Definition at line 71 of file kglobalaccel.h.
Member Function Documentation
◆ cleanComponent()
|
static |
Clean the shortcuts for component componentUnique.
If the component is not active all global shortcut registrations are purged and the component is removed completely.
If the component is active all global shortcut registrations not in use will be purged. If there is no shortcut registration left the component is purged too.
If a purged component or shortcut is activated the next time it will reregister itself. All you probably will lose on wrong usage are the user's set shortcuts.
If you make sure your component is running and all global shortcuts it has are active this function can be used to clean up the registry.
Handle with care!
If the method return true
at least one shortcut was purged so handle all previously acquired information with care.
Definition at line 152 of file kglobalaccel.cpp.
◆ defaultShortcut()
QList< QKeySequence > KGlobalAccel::defaultShortcut | ( | const QAction * | action | ) | const |
Get the global default shortcut for this action, if one exists.
Global shortcuts allow your actions to respond to accellerators independently of the focused window. Unlike regular shortcuts, the application's window does not need focus for them to be activated.
- See also
- setDefaultShortcut()
- Since
- 5.0
Definition at line 626 of file kglobalaccel.cpp.
◆ globalShortcut()
QList< QKeySequence > KGlobalAccel::globalShortcut | ( | const QString & | componentName, |
const QString & | actionId ) const |
Retrieves the shortcut as defined in global settings by componentName (e.g.
"kwin") and actionId (e.g. "Kill Window").
- Since
- 5.10
Definition at line 636 of file kglobalaccel.cpp.
◆ globalShortcutChanged
|
signal |
Emitted when the global shortcut is changed.
A global shortcut is subject to be changed by the global shortcuts kcm.
- Parameters
-
action pointer to the action for which the changed shortcut was registered seq the key sequence that corresponds to the changed shortcut
- See also
- setGlobalShortcut
- setDefaultShortcut
- Since
- 5.0
◆ globalShortcutsByKey()
|
static |
Returns a list of global shortcuts registered for the shortcut seq
.
If the list contains more that one entry it means the component that registered the shortcuts uses global shortcut contexts. All returned shortcuts belong to the same component.
- Parameters
-
type a value from the KGlobalAccel::MatchType enum
- Since
- 5.90
Definition at line 520 of file kglobalaccel.cpp.
◆ hasShortcut()
bool KGlobalAccel::hasShortcut | ( | const QAction * | action | ) | const |
Returns true if a shortcut or a default shortcut has been registered for the given action.
- Since
- 5.0
Definition at line 653 of file kglobalaccel.cpp.
◆ isComponentActive()
|
static |
Check if component is active.
- Parameters
-
componentUnique the components unique identifier
- Returns
true
if active, @false if not
Definition at line 163 of file kglobalaccel.cpp.
◆ isGlobalShortcutAvailable()
|
static |
Check if the shortcut @seq is available for the component
.
The component is only of interest if the current application uses global shortcut contexts. In that case a global shortcut by component
in an inactive global shortcut contexts does not block the seq
for us.
- Since
- 4.2
Definition at line 525 of file kglobalaccel.cpp.
◆ promptStealShortcutSystemwide()
|
static |
Show a messagebox to inform the user that a global shortcut is already occupied, and ask to take it away from its current action(s).
This is GUI only, so nothing will be actually changed.
- See also
- stealShortcutSystemwide()
- Since
- 4.2
Definition at line 531 of file kglobalaccel.cpp.
◆ removeAllShortcuts()
void KGlobalAccel::removeAllShortcuts | ( | QAction * | action | ) |
Unregister and remove all defined global shortcuts for the given action.
- Since
- 5.0
Definition at line 648 of file kglobalaccel.cpp.
◆ self()
|
static |
Returns (and creates if necessary) the singleton instance.
Definition at line 195 of file kglobalaccel.cpp.
◆ setDefaultShortcut()
bool KGlobalAccel::setDefaultShortcut | ( | QAction * | action, |
const QList< QKeySequence > & | shortcut, | ||
GlobalShortcutLoading | loadFlag = Autoloading ) |
Assign a default global shortcut for a given QAction.
For more information about global shortcuts
- See also
- setShortcut Upon shortcut change the globalShortcutChanged will be triggered so other applications get notified
- globalShortcutChanged
- Since
- 5.0
Definition at line 596 of file kglobalaccel.cpp.
◆ setGlobalShortcut() [1/2]
|
static |
Convenient method to set both active and default shortcut.
This method is suited for the case that only one shortcut is to be configured.
If more control for loading the shortcuts is needed use the variants offering more control.
- See also
- setShortcut
- setDefaultShortcut
- Since
- 5.0
Definition at line 664 of file kglobalaccel.cpp.
◆ setGlobalShortcut() [2/2]
|
static |
Convenient method to set both active and default shortcut.
If more control for loading the shortcuts is needed use the variants offering more control.
- See also
- setShortcut
- setDefaultShortcut
- Since
- 5.0
Definition at line 658 of file kglobalaccel.cpp.
◆ setShortcut()
bool KGlobalAccel::setShortcut | ( | QAction * | action, |
const QList< QKeySequence > & | shortcut, | ||
GlobalShortcutLoading | loadFlag = Autoloading ) |
Assign a global shortcut for the given action.
Global shortcuts allow an action to respond to key shortcuts independently of the focused window, i.e. the action will trigger if the keys were pressed no matter where in the X session.
The action must have a per main component unique action->objectName() to enable cross-application bookkeeping. If the action->objectName() is empty this method will do nothing and will return false.
It is mandatory that the action->objectName() doesn't change once the shortcut has been successfully registered.
- Note
- KActionCollection::insert(name, action) will set action's objectName to name so you often don't have to set an objectName explicitly.
When an action, identified by main component name and objectName(), is assigned a global shortcut for the first time on a KDE installation the assignment will be saved. The shortcut will then be restored every time setGlobalShortcut() is called with loading
== Autoloading.
If you actually want to change the global shortcut you have to set loading
to NoAutoloading. The new shortcut will be automatically saved again.
- Parameters
-
action the action for which the shortcut will be assigned shortcut global shortcut(s) to assign. Will be ignored unless loading
is set to NoAutoloading or this is the first time ever you call this method (see above).loadFlag if Autoloading, assign the global shortcut this action has previously had if any. That way user preferences and changes made to avoid clashes will be conserved. if NoAutoloading the given shortcut will be assigned without looking up old values. You should only do this if the user wants to change the shortcut or if you have another very good reason. Key combinations that clash with other shortcuts will be dropped.
- Note
- the default shortcut will never be influenced by autoloading - it will be set as given.
- See also
- shortcut()
- globalShortcutChanged
- Since
- 5.0
Definition at line 611 of file kglobalaccel.cpp.
◆ shortcut()
QList< QKeySequence > KGlobalAccel::shortcut | ( | const QAction * | action | ) | const |
Get the global shortcut for this action, if one exists.
Global shortcuts allow your actions to respond to accellerators independently of the focused window. Unlike regular shortcuts, the application's window does not need focus for them to be activated.
- Note
- that this method only works together with setShortcut() because the action pointer is used to retrieve the result. If you would like to retrieve the shortcut as stored in the global settings, use the globalShortcut(componentName, actionId) instead.
- See also
- setShortcut()
- Since
- 5.0
Definition at line 631 of file kglobalaccel.cpp.
◆ stealShortcutSystemwide()
|
static |
Take away the given shortcut from the named action it belongs to.
This applies to all actions with global shortcuts in any KDE application.
- See also
- promptStealShortcutSystemwide()
Definition at line 563 of file kglobalaccel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:08:57 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.