KIconEffect
#include <KIconEffect>
Public Types | |
enum | Effects { NoEffect , ToGray , Colorize , ToGamma , DeSaturate , ToMonochrome , LastEffect } |
Public Member Functions | |
KIconEffect () | |
KIconEffect (const KIconEffect &)=delete | |
QImage | apply (const QImage &src, int effect, float value, const QColor &rgb, bool trans) const |
QImage | apply (const QImage &src, int effect, float value, const QColor &rgb, const QColor &rgb2, bool trans) const |
QImage | apply (const QImage &src, int group, int state) const |
QPixmap | apply (const QPixmap &src, int effect, float value, const QColor &rgb, bool trans) const |
QPixmap | apply (const QPixmap &src, int effect, float value, const QColor &rgb, const QColor &rgb2, bool trans) const |
QPixmap | apply (const QPixmap &src, int group, int state) const |
QImage | doublePixels (const QImage &src) const |
QString | fingerprint (int group, int state) const |
bool | hasEffect (int group, int state) const |
void | init () |
KIconEffect & | operator= (const KIconEffect &)=delete |
Static Public Member Functions | |
static void | colorize (QImage &image, const QColor &col, float value) |
static void | deSaturate (QImage &image, float value) |
static void | overlay (QImage &src, QImage &overlay) |
static void | semiTransparent (QImage &image) |
static void | semiTransparent (QPixmap &pixmap) |
static void | toActive (QImage &image) |
static void | toActive (QPixmap &pixmap) |
static void | toDisabled (QImage &image) |
static void | toDisabled (QPixmap &pixmap) |
static void | toGamma (QImage &image, float value) |
static void | toGray (QImage &image, float value) |
static void | toMonochrome (QImage &image, const QColor &black, const QColor &white, float value) |
Detailed Description
Applies effects to icons.
This class applies effects to icons depending on their state and group. For example, it can be used to make all disabled icons in a toolbar gray.
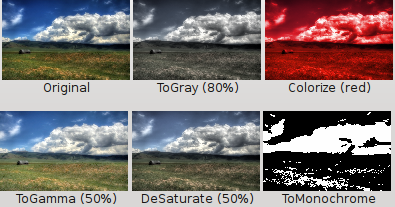
- See also
- QIcon::fromTheme
Definition at line 39 of file kiconeffect.h.
Member Enumeration Documentation
◆ Effects
enum KIconEffect::Effects |
This is the enumeration of all possible icon effects.
Note that 'LastEffect' is no valid icon effect but only used internally to check for invalid icon effects.
- NoEffect: Do not apply any icon effect
- ToGray: Tints the icon gray
- Colorize: Tints the icon with a specific color
- ToGamma: Change the gamma value of the icon
- DeSaturate: Reduce the saturation of the icon
- ToMonochrome: Produces a monochrome icon
- Deprecated
- since 6.5, use the static API
Definition at line 77 of file kiconeffect.h.
Constructor & Destructor Documentation
◆ KIconEffect()
KIconEffect::KIconEffect | ( | ) |
Create a new KIconEffect.
You will most likely never have to use this to create a new KIconEffect yourself, as you can use the KIconEffect provided by the global KIconLoader (which itself is accessible by KIconLoader::global()) through its iconEffect() function.
- Deprecated
- since 6.5, use the static API
Definition at line 47 of file kiconeffect.cpp.
Member Function Documentation
◆ apply() [1/6]
QImage KIconEffect::apply | ( | const QImage & | src, |
int | effect, | ||
float | value, | ||
const QColor & | rgb, | ||
bool | trans ) const |
Applies an effect to an image.
- Parameters
-
src The image. effect The effect to apply, one of KIconEffect::Effects. value Strength of the effect. 0 <= value
<= 1.rgb Color parameter for effects that need one. trans Add Transparency if trans = true.
- Returns
- An image with the effect applied.
- Deprecated
- since 6.5, use the static API
Definition at line 144 of file kiconeffect.cpp.
◆ apply() [2/6]
QImage KIconEffect::apply | ( | const QImage & | src, |
int | effect, | ||
float | value, | ||
const QColor & | rgb, | ||
const QColor & | rgb2, | ||
bool | trans ) const |
Definition at line 151 of file kiconeffect.cpp.
◆ apply() [3/6]
Applies an effect to an image.
The effect to apply depends on the group
and state
parameters, and is configured by the user.
- Parameters
-
src The image. group The group for the icon, see KIconLoader::Group state The icon's state, see KIconLoader::States
- Returns
- An image with the effect applied.
- Deprecated
- since 6.5, use the static API
Definition at line 129 of file kiconeffect.cpp.
◆ apply() [4/6]
QPixmap KIconEffect::apply | ( | const QPixmap & | src, |
int | effect, | ||
float | value, | ||
const QColor & | rgb, | ||
bool | trans ) const |
Applies an effect to a pixmap.
- Parameters
-
src The pixmap. effect The effect to apply, one of KIconEffect::Effects. value Strength of the effect. 0 <= value
<= 1.rgb Color parameter for effects that need one. trans Add Transparency if trans = true.
- Returns
- A pixmap with the effect applied.
- Deprecated
- since 6.5, use the static API
Definition at line 203 of file kiconeffect.cpp.
◆ apply() [5/6]
QPixmap KIconEffect::apply | ( | const QPixmap & | src, |
int | effect, | ||
float | value, | ||
const QColor & | rgb, | ||
const QColor & | rgb2, | ||
bool | trans ) const |
Definition at line 210 of file kiconeffect.cpp.
◆ apply() [6/6]
Applies an effect to a pixmap.
- Parameters
-
src The pixmap. group The group for the icon, see KIconLoader::Group state The icon's state, see KIconLoader::States
- Returns
- A pixmap with the effect applied.
- Deprecated
- since 6.5, use the static API
Definition at line 188 of file kiconeffect.cpp.
◆ colorize()
Colorizes an image with a specific color.
- Parameters
-
image The image col The color with which the image
is tintedvalue Strength of the effect. 0 <= value
<= 1
Definition at line 303 of file kiconeffect.cpp.
◆ deSaturate()
|
static |
Desaturates an image.
- Parameters
-
image The image value Strength of the effect. 0 <= value
<= 1
Definition at line 412 of file kiconeffect.cpp.
◆ doublePixels()
Returns an image twice as large, consisting of 2x2 pixels.
- Parameters
-
src the image.
- Returns
- the scaled image.
- Deprecated
- since 6.5, use the static API
Definition at line 542 of file kiconeffect.cpp.
◆ fingerprint()
QString KIconEffect::fingerprint | ( | int | group, |
int | state ) const |
Returns a fingerprint for the effect by encoding the given group
and state
into a QString.
This is useful for caching.
- Parameters
-
group the group, see KIconLoader::Group state the state, see KIconLoader::States
- Returns
- the fingerprint of the given
group+
state
- Deprecated
- since 6.5, use the static API
Definition at line 105 of file kiconeffect.cpp.
◆ hasEffect()
bool KIconEffect::hasEffect | ( | int | group, |
int | state ) const |
Tests whether an effect has been configured for the given icon group.
- Parameters
-
group the group to check, see KIconLoader::Group state the state to check, see KIconLoader::States
- Returns
- true if an effect is configured for the given
group
instate
, otherwise false.
- Deprecated
- since 6.5, use the static API
Definition at line 93 of file kiconeffect.cpp.
◆ init()
void KIconEffect::init | ( | ) |
Rereads configuration.
- Deprecated
- since 6.5, use the static API
Definition at line 57 of file kiconeffect.cpp.
◆ overlay()
Overlays an image with an other image.
- Parameters
-
src The image overlay The image to overlay src
with
Definition at line 587 of file kiconeffect.cpp.
◆ semiTransparent() [1/2]
|
static |
Renders an image semi-transparent.
- Parameters
-
image The image
Definition at line 451 of file kiconeffect.cpp.
◆ semiTransparent() [2/2]
|
static |
Renders a pixmap semi-transparent.
- Parameters
-
pixmap The pixmap
Definition at line 535 of file kiconeffect.cpp.
◆ toActive() [1/2]
|
static |
Applies an effect for an icon that is in an 'active' state.
- Parameters
-
image The image
- Since
- 6.5
Definition at line 714 of file kiconeffect.cpp.
◆ toActive() [2/2]
|
static |
Applies an effect for an icon that is in an 'active' state.
- Parameters
-
pixmap The image
- Since
- 6.5
Definition at line 719 of file kiconeffect.cpp.
◆ toDisabled() [1/2]
|
static |
Applies a disabled effect.
- Parameters
-
image The image
- Since
- 6.5
Definition at line 701 of file kiconeffect.cpp.
◆ toDisabled() [2/2]
|
static |
Applies a disabled effect.
- Parameters
-
pixmap The image
- Since
- 6.5
Definition at line 707 of file kiconeffect.cpp.
◆ toGamma()
|
static |
Changes the gamma value of an image.
- Parameters
-
image The image value Strength of the effect. 0 <= value
<= 1
Definition at line 435 of file kiconeffect.cpp.
◆ toGray()
|
static |
Tints an image gray.
- Parameters
-
image The image value Strength of the effect. 0 <= value
<= 1
Definition at line 273 of file kiconeffect.cpp.
◆ toMonochrome()
|
static |
Produces a monochrome icon with a given foreground and background color.
- Parameters
-
image The image white The color with which the white parts of image
are paintedblack The color with which the black parts of image
are paintedvalue Strength of the effect. 0 <= value
<= 1
Definition at line 345 of file kiconeffect.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:52:37 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.