KPtyProcess
#include <kptyprocess.h>
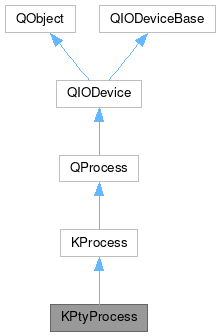
Public Types | |
enum | PtyChannelFlag { NoChannels = 0 , StdinChannel = 1 , StdoutChannel = 2 , StderrChannel = 4 , AllOutputChannels = 6 , AllChannels = 7 } |
typedef QFlags< PtyChannelFlag > | PtyChannels |
![]() | |
enum | OutputChannelMode |
![]() | |
enum | ExitStatus |
enum | InputChannelMode |
enum | ProcessChannel |
enum | ProcessChannelMode |
enum | ProcessError |
enum | ProcessState |
enum | UnixProcessFlag |
![]() | |
enum | OpenModeFlag |
Public Member Functions | |
KPtyProcess (int ptyMasterFd, QObject *parent=nullptr) | |
KPtyProcess (QObject *parent=nullptr) | |
~KPtyProcess () override | |
bool | isUseUtmp () const |
KPtyDevice * | pty () const |
PtyChannels | ptyChannels () const |
void | setPtyChannels (PtyChannels channels) |
void | setUseUtmp (bool value) |
![]() | |
KProcess (QObject *parent=nullptr) | |
void | clearEnvironment () |
void | clearProgram () |
int | execute (int msecs=-1) |
KProcess & | operator<< (const QString &arg) |
KProcess & | operator<< (const QStringList &args) |
OutputChannelMode | outputChannelMode () const |
QStringList | program () const |
void | setEnv (const QString &name, const QString &value, bool overwrite=true) |
void | setNextOpenMode (QIODevice::OpenMode mode) |
void | setOutputChannelMode (OutputChannelMode mode) |
void | setProgram (const QString &exe, const QStringList &args=QStringList()) |
void | setProgram (const QStringList &argv) |
void | setShellCommand (const QString &cmd) |
void | start () |
int | startDetached () |
void | unsetEnv (const QString &name) |
![]() | |
QProcess (QObject *parent) | |
QStringList | arguments () const const |
virtual qint64 | bytesToWrite () const const override |
std::function< void()> | childProcessModifier ()> childProcessModifier() const const |
virtual void | close () override |
void | closeReadChannel (ProcessChannel channel) |
void | closeWriteChannel () |
CreateProcessArgumentModifier | createProcessArgumentsModifier () const const |
QStringList | environment () const const |
QProcess::ProcessError | error () const const |
void | errorOccurred (QProcess::ProcessError error) |
int | exitCode () const const |
QProcess::ExitStatus | exitStatus () const const |
void | finished (int exitCode, QProcess::ExitStatus exitStatus) |
InputChannelMode | inputChannelMode () const const |
virtual bool | isSequential () const const override |
void | kill () |
QString | nativeArguments () const const |
virtual bool | open (OpenMode mode) override |
ProcessChannelMode | processChannelMode () const const |
QProcessEnvironment | processEnvironment () const const |
qint64 | processId () const const |
QString | program () const const |
QByteArray | readAllStandardError () |
QByteArray | readAllStandardOutput () |
ProcessChannel | readChannel () const const |
void | readyReadStandardError () |
void | readyReadStandardOutput () |
void | setArguments (const QStringList &arguments) |
void | setChildProcessModifier (const std::function< void()> &modifier) |
void | setCreateProcessArgumentsModifier (CreateProcessArgumentModifier modifier) |
void | setEnvironment (const QStringList &environment) |
void | setInputChannelMode (InputChannelMode mode) |
void | setNativeArguments (const QString &arguments) |
void | setProcessChannelMode (ProcessChannelMode mode) |
void | setProcessEnvironment (const QProcessEnvironment &environment) |
void | setProgram (const QString &program) |
void | setReadChannel (ProcessChannel channel) |
void | setStandardErrorFile (const QString &fileName, OpenMode mode) |
void | setStandardInputFile (const QString &fileName) |
void | setStandardOutputFile (const QString &fileName, OpenMode mode) |
void | setStandardOutputProcess (QProcess *destination) |
void | setUnixProcessParameters (const UnixProcessParameters ¶ms) |
void | setUnixProcessParameters (UnixProcessFlags flagsOnly) |
void | setWorkingDirectory (const QString &dir) |
void | start (const QString &program, const QStringList &arguments, OpenMode mode) |
void | start (OpenMode mode) |
void | startCommand (const QString &command, OpenMode mode) |
bool | startDetached (qint64 *pid) |
void | started () |
QProcess::ProcessState | state () const const |
void | stateChanged (QProcess::ProcessState newState) |
void | terminate () |
UnixProcessParameters | unixProcessParameters () const const |
virtual bool | waitForBytesWritten (int msecs) override |
bool | waitForFinished (int msecs) |
virtual bool | waitForReadyRead (int msecs) override |
bool | waitForStarted (int msecs) |
QString | workingDirectory () const const |
![]() | |
QIODevice (QObject *parent) | |
void | aboutToClose () |
virtual bool | atEnd () const const |
virtual qint64 | bytesAvailable () const const |
void | bytesWritten (qint64 bytes) |
virtual bool | canReadLine () const const |
void | channelBytesWritten (int channel, qint64 bytes) |
void | channelReadyRead (int channel) |
void | commitTransaction () |
int | currentReadChannel () const const |
int | currentWriteChannel () const const |
QString | errorString () const const |
bool | getChar (char *c) |
bool | isOpen () const const |
bool | isReadable () const const |
bool | isTextModeEnabled () const const |
bool | isTransactionStarted () const const |
bool | isWritable () const const |
virtual bool | open (QIODeviceBase::OpenMode mode) |
QIODeviceBase::OpenMode | openMode () const const |
qint64 | peek (char *data, qint64 maxSize) |
QByteArray | peek (qint64 maxSize) |
virtual qint64 | pos () const const |
bool | putChar (char c) |
qint64 | read (char *data, qint64 maxSize) |
QByteArray | read (qint64 maxSize) |
QByteArray | readAll () |
int | readChannelCount () const const |
void | readChannelFinished () |
qint64 | readLine (char *data, qint64 maxSize) |
QByteArray | readLine (qint64 maxSize) |
void | readyRead () |
virtual bool | reset () |
void | rollbackTransaction () |
virtual bool | seek (qint64 pos) |
void | setCurrentReadChannel (int channel) |
void | setCurrentWriteChannel (int channel) |
void | setTextModeEnabled (bool enabled) |
virtual qint64 | size () const const |
qint64 | skip (qint64 maxSize) |
void | startTransaction () |
void | ungetChar (char c) |
qint64 | write (const char *data) |
qint64 | write (const char *data, qint64 maxSize) |
qint64 | write (const QByteArray &data) |
int | writeChannelCount () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
static int | execute (const QString &exe, const QStringList &args=QStringList(), int msecs=-1) |
static int | execute (const QStringList &argv, int msecs=-1) |
static int | startDetached (const QString &exe, const QStringList &args=QStringList()) |
static int | startDetached (const QStringList &argv) |
![]() | |
int | execute (const QString &program, const QStringList &arguments) |
QString | nullDevice () |
QStringList | splitCommand (QStringView command) |
bool | startDetached (const QString &program, const QStringList &arguments, const QString &workingDirectory, qint64 *pid) |
QStringList | systemEnvironment () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
ForwardedChannels | |
MergedChannels | |
OnlyStderrChannel | |
OnlyStdoutChannel | |
SeparateChannels | |
![]() | |
CloseFileDescriptors | |
Crashed | |
CrashExit | |
typedef | CreateProcessArgumentModifier |
FailedToStart | |
ForwardedChannels | |
ForwardedErrorChannel | |
ForwardedInputChannel | |
ForwardedOutputChannel | |
IgnoreSigPipe | |
ManagedInputChannel | |
MergedChannels | |
NormalExit | |
NotRunning | |
ReadError | |
ResetSignalHandlers | |
Running | |
SeparateChannels | |
StandardError | |
StandardOutput | |
Starting | |
Timedout | |
typedef | UnixProcessFlags |
UnknownError | |
UseVFork | |
WriteError | |
![]() | |
typedef | QObjectList |
![]() | |
Append | |
ExistingOnly | |
NewOnly | |
NotOpen | |
typedef | OpenMode |
ReadOnly | |
ReadWrite | |
Text | |
Truncate | |
Unbuffered | |
WriteOnly | |
![]() | |
KCOREADDONS_NO_EXPORT | KProcess (KProcessPrivate *d, QObject *parent) |
![]() | |
virtual qint64 | readData (char *data, qint64 maxlen) override |
void | setProcessState (ProcessState state) |
![]() | |
virtual qint64 | readLineData (char *data, qint64 maxSize) |
void | setErrorString (const QString &str) |
void | setOpenMode (QIODeviceBase::OpenMode openMode) |
virtual qint64 | skipData (qint64 maxSize) |
virtual qint64 | writeData (const char *data, qint64 maxSize)=0 |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
std::unique_ptr< KProcessPrivate > const | d_ptr |
Detailed Description
This class extends KProcess by support for PTYs (pseudo TTYs).
The PTY is opened as soon as the class is instantiated. Verify that it was opened successfully by checking that pty()->masterFd() is not -1.
The PTY is always made the process' controlling TTY. Utmp registration and connecting the stdio handles to the PTY are optional.
No attempt to integrate with QProcess' waitFor*() functions was made, for it is impossible. Note that execute() does not work with the PTY, too. Use the PTY device's waitFor*() functions or use it asynchronously.
- Note
- If you inherit from this class and use setChildProcessModifier() in the derived class, you must call the childProcessModifier() of KPtyProcess first (using setChildProcessModifier() in the derived class will "overwrite" the childProcessModifier() std::function that was previously set by KPtyProcess). For example: {MyProcess(){auto parentChildProcModifier = KPtyProcess::childProcessModifier();setChildProcessModifier([parentChildProcModifier]() {// First call the parent class modifier functionif (parentChildProcModifier) {parentChildProcModifier();}// Then whatever extra code you need to run........});}std::function< void()> childProcessModifier()> childProcessModifier() const constvoid setChildProcessModifier(const std::function< void()> &modifier)
Definition at line 59 of file kptyprocess.h.
Member Typedef Documentation
◆ PtyChannels
typedef QFlags< PtyChannelFlag > KPtyProcess::PtyChannels |
Stores a combination of PtyChannelFlag values.
Definition at line 80 of file kptyprocess.h.
Member Enumeration Documentation
◆ PtyChannelFlag
- See also
- PtyChannels
Definition at line 68 of file kptyprocess.h.
Constructor & Destructor Documentation
◆ KPtyProcess() [1/2]
|
explicit |
Constructor.
Definition at line 32 of file kptyprocess.cpp.
◆ KPtyProcess() [2/2]
KPtyProcess::KPtyProcess | ( | int | ptyMasterFd, |
QObject * | parent = nullptr ) |
Construct a process using an open pty master.
- Parameters
-
ptyMasterFd an open pty master file descriptor. The process does not take ownership of the descriptor; it will not be automatically closed at any point.
Definition at line 37 of file kptyprocess.cpp.
◆ ~KPtyProcess()
|
override |
Destructor.
Definition at line 79 of file kptyprocess.cpp.
Member Function Documentation
◆ isUseUtmp()
bool KPtyProcess::isUseUtmp | ( | ) | const |
Get whether to register the process as a TTY login in utmp.
- Returns
- whether to register in utmp
Definition at line 110 of file kptyprocess.cpp.
◆ pty()
KPtyDevice * KPtyProcess::pty | ( | ) | const |
Get the PTY device of this process.
- Returns
- the PTY device
Definition at line 117 of file kptyprocess.cpp.
◆ ptyChannels()
KPtyProcess::PtyChannels KPtyProcess::ptyChannels | ( | ) | const |
Query to which channels the PTY is assigned.
- Returns
- the output channel handling mode
Definition at line 96 of file kptyprocess.cpp.
◆ setPtyChannels()
void KPtyProcess::setPtyChannels | ( | PtyChannels | channels | ) |
Set to which channels the PTY should be assigned.
This function must be called before starting the process.
- Parameters
-
channels the output channel handling mode
Definition at line 89 of file kptyprocess.cpp.
◆ setUseUtmp()
void KPtyProcess::setUseUtmp | ( | bool | value | ) |
Set whether to register the process as a TTY login in utmp.
Utmp is disabled by default. It should enabled for interactively fed processes, like terminal emulations.
This function must be called before starting the process.
- Parameters
-
value whether to register in utmp.
Definition at line 103 of file kptyprocess.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:54:31 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.