KStatusNotifierItem
#include <KStatusNotifierItem>
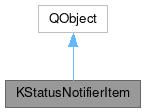
Public Types | |
enum | ItemCategory { ApplicationStatus = 1 , Communications = 2 , SystemServices = 3 , Hardware = 4 , Reserved = 129 } |
enum | ItemStatus { Passive = 1 , Active = 2 , NeedsAttention = 3 } |
Properties | |
QString | attentionIconName |
ItemCategory | category |
QString | iconName |
QString | overlayIconName |
ItemStatus | status |
QString | title |
QString | toolTipIconName |
QString | toolTipSubTitle |
QString | toolTipTitle |
![]() | |
objectName | |
Signals | |
void | activateRequested (bool active, const QPoint &pos) |
void | quitRequested () |
void | scrollRequested (int delta, Qt::Orientation orientation) |
void | secondaryActivateRequested (const QPoint &pos) |
Public Slots | |
virtual void | activate (const QPoint &pos=QPoint()) |
void | hideAssociatedWindow () |
Public Member Functions | |
KStatusNotifierItem (const QString &id, QObject *parent=nullptr) | |
KStatusNotifierItem (QObject *parent=nullptr) | |
void | abortQuit () |
QWindow * | associatedWindow () const |
QString | attentionIconName () const |
QIcon | attentionIconPixmap () const |
QString | attentionMovieName () const |
ItemCategory | category () const |
QMenu * | contextMenu () const |
QString | iconName () const |
QIcon | iconPixmap () const |
QString | id () const |
bool | isMenu () const |
QString | overlayIconName () const |
QIcon | overlayIconPixmap () const |
QString | providedToken () const |
void | setAssociatedWindow (QWindow *window) |
void | setAttentionIconByName (const QString &name) |
void | setAttentionIconByPixmap (const QIcon &icon) |
void | setAttentionMovieByName (const QString &name) |
void | setCategory (const ItemCategory category) |
void | setContextMenu (QMenu *menu) |
void | setIconByName (const QString &name) |
void | setIconByPixmap (const QIcon &icon) |
void | setIsMenu (bool isMenu) |
void | setOverlayIconByName (const QString &name) |
void | setOverlayIconByPixmap (const QIcon &icon) |
void | setStandardActionsEnabled (bool enabled) |
void | setStatus (const ItemStatus status) |
void | setTitle (const QString &title) |
void | setToolTip (const QIcon &icon, const QString &title, const QString &subTitle) |
void | setToolTip (const QString &iconName, const QString &title, const QString &subTitle) |
void | setToolTipIconByName (const QString &name) |
void | setToolTipIconByPixmap (const QIcon &icon) |
void | setToolTipSubTitle (const QString &subTitle) |
void | setToolTipTitle (const QString &title) |
void | showMessage (const QString &title, const QString &message, const QString &icon, int timeout=10000) |
bool | standardActionsEnabled () const |
ItemStatus | status () const |
QString | title () const |
QString | toolTipIconName () const |
QIcon | toolTipIconPixmap () const |
QString | toolTipSubTitle () const |
QString | toolTipTitle () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | eventFilter (QObject *watched, QEvent *event) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
KDE Status notifier Item protocol implementation
This class implements the Status notifier Item D-Bus specification. It provides an icon similar to the classical systemtray icons, with some key differences:
- the actual representation is done by the systemtray (or the app behaving like it) itself, not by this app. Since 4.5 this also includes the menu, which means you cannot use embed widgets in the menu.
- there is communication between the systemtray and the icon owner, so the system tray can know if the application is in a normal or in a requesting attention state.
- icons are divided in categories, so the systemtray can represent in a different way the icons from normal applications and for instance the ones about hardware status.
Whenever possible you should prefer passing icon by name rather than by pixmap because:
- it is much lighter on D-Bus (no need to pass all image pixels).
- it makes it possible for the systemtray to load an icon of the appropriate size or to replace your icon with a systemtray specific icon which matches with the desktop theme.
- some implementations of the system tray do not support passing icons by pixmap and will show a blank icon instead.
- Note
- When used inside a Flatpak it is important to request explicit support in the Flatpak manifest with the following line. –talk-name=org.kde.StatusNotifierWatcher
- Since
- 4.4
Definition at line 65 of file kstatusnotifieritem.h.
Member Enumeration Documentation
◆ ItemCategory
Different kinds of applications announce their type to the systemtray, so can be drawn in a different way or in a different place.
Definition at line 103 of file kstatusnotifieritem.h.
◆ ItemStatus
All the possible status this icon can have, depending on the importance of the events that happens in the parent application.
Definition at line 87 of file kstatusnotifieritem.h.
Property Documentation
◆ attentionIconName
|
readwrite |
Definition at line 74 of file kstatusnotifieritem.h.
◆ category
|
readwrite |
Definition at line 69 of file kstatusnotifieritem.h.
◆ iconName
|
readwrite |
Definition at line 72 of file kstatusnotifieritem.h.
◆ overlayIconName
|
readwrite |
Definition at line 73 of file kstatusnotifieritem.h.
◆ status
|
readwrite |
Definition at line 71 of file kstatusnotifieritem.h.
◆ title
|
readwrite |
Definition at line 70 of file kstatusnotifieritem.h.
◆ toolTipIconName
|
readwrite |
Definition at line 75 of file kstatusnotifieritem.h.
◆ toolTipSubTitle
|
readwrite |
Definition at line 77 of file kstatusnotifieritem.h.
◆ toolTipTitle
|
readwrite |
Definition at line 76 of file kstatusnotifieritem.h.
Constructor & Destructor Documentation
◆ KStatusNotifierItem() [1/2]
|
explicit |
Construct a new status notifier item.
- Parameters
-
parent the parent object for this object. If the object passed in as a parent is also a QWidget, it will be used as the main application window represented by this icon and will be shown/hidden when an activation is requested.
- See also
- associatedWindow
Definition at line 59 of file kstatusnotifieritem.cpp.
◆ KStatusNotifierItem() [2/2]
|
explicit |
Construct a new status notifier item with a unique identifier.
If your application has more than one status notifier item and the user should be able to manipulate them separately (e.g. mark them for hiding in a user interface), the id can be used to differentiate between them.
The id should remain consistent even between application restarts. Status notifier items without ids default to the application's name for the id. This id may be used, for instance, by hosts displaying status notifier items to associate configuration information with this item in a way that can persist between sessions or application restarts.
- Parameters
-
id the unique id for this icon parent the parent object for this object. If the object passed in as a parent is also a QWidget, it will be used as the main application window represented by this icon and will be shown/hidden when an activation is requested.
- See also
- associatedWindow
Definition at line 66 of file kstatusnotifieritem.cpp.
◆ ~KStatusNotifierItem()
|
override |
Definition at line 73 of file kstatusnotifieritem.cpp.
Member Function Documentation
◆ abortQuit()
void KStatusNotifierItem::abortQuit | ( | ) |
Cancelles an ongoing quit operation.
Call this in a slot connected to quitRequested().
- See also
- quitRequested()
- Since
- 6.5
Definition at line 1153 of file kstatusnotifieritem.cpp.
◆ activate
Shows the main window and try to position it on top of the other windows, if the window is already visible, hide it.
- Parameters
-
pos if it's a valid position it represents the mouse coordinates when the event was triggered
Definition at line 639 of file kstatusnotifieritem.cpp.
◆ activateRequested
|
signal |
Inform the host application that an activation has been requested, for instance left mouse click, but this is not guaranteed since it's dependent from the visualization.
- Parameters
-
active if it's true the application asked for the activation of the main window, if it's false it asked for hiding pos the position in the screen where the user clicked to trigger this signal, QPoint() if it's not the consequence of a mouse click.
◆ associatedWindow()
QWindow * KStatusNotifierItem::associatedWindow | ( | ) | const |
Access the main window associated with this StatusNotifierItem.
- Since
- 6.0
Definition at line 542 of file kstatusnotifieritem.cpp.
◆ attentionIconName()
QString KStatusNotifierItem::attentionIconName | ( | ) | const |
- Returns
- the name of the icon to be displayed when the application is requesting the user's attention if attentionImage() is not empty this will always return an empty string
Definition at line 253 of file kstatusnotifieritem.cpp.
◆ attentionIconPixmap()
QIcon KStatusNotifierItem::attentionIconPixmap | ( | ) | const |
- Returns
- a pixmap of the requesting attention icon
Definition at line 273 of file kstatusnotifieritem.cpp.
◆ attentionMovieName()
QString KStatusNotifierItem::attentionMovieName | ( | ) | const |
- Returns
- the name of the movie to be displayed when the application is requesting the user attention
Definition at line 299 of file kstatusnotifieritem.cpp.
◆ category()
KStatusNotifierItem::ItemCategory KStatusNotifierItem::category | ( | ) | const |
- Returns
- the application category
Definition at line 104 of file kstatusnotifieritem.cpp.
◆ contextMenu()
QMenu * KStatusNotifierItem::contextMenu | ( | ) | const |
Access the context menu associated to this status notifier item.
Definition at line 489 of file kstatusnotifieritem.cpp.
◆ eventFilter()
|
overrideprotectedvirtual |
Reimplemented from QObject.
Definition at line 795 of file kstatusnotifieritem.cpp.
◆ hideAssociatedWindow
|
slot |
Hides the main window, if not already hidden.
Stores some information about the window which otherwise would be lost due to unmapping from the window system. Use when toggling the main window via activate(const QPoint &) is not wanted, but instead the hidden state should be reached in any case.
- Since
- 6.0
Definition at line 666 of file kstatusnotifieritem.cpp.
◆ iconName()
QString KStatusNotifierItem::iconName | ( | ) | const |
- Returns
- the name of the main icon to be displayed if image() is not empty this will always return an empty string
Definition at line 151 of file kstatusnotifieritem.cpp.
◆ iconPixmap()
QIcon KStatusNotifierItem::iconPixmap | ( | ) | const |
- Returns
- a pixmap of the icon
Definition at line 175 of file kstatusnotifieritem.cpp.
◆ id()
QString KStatusNotifierItem::id | ( | ) | const |
- Returns
- The id which was specified in the constructor. This should be guaranteed to be consistent between application starts and untranslated, as host applications displaying items may use it for storing configuration related to this item.
Definition at line 88 of file kstatusnotifieritem.cpp.
◆ isMenu()
bool KStatusNotifierItem::isMenu | ( | ) | const |
- Returns
- if the item indicates that it only supports the context menu.
- Since
- 6.14
Definition at line 688 of file kstatusnotifieritem.cpp.
◆ overlayIconName()
QString KStatusNotifierItem::overlayIconName | ( | ) | const |
- Returns
- the name of the icon to be used as overlay fr the main one
Definition at line 202 of file kstatusnotifieritem.cpp.
◆ overlayIconPixmap()
QIcon KStatusNotifierItem::overlayIconPixmap | ( | ) | const |
- Returns
- a pixmap of the icon
Definition at line 232 of file kstatusnotifieritem.cpp.
◆ providedToken()
QString KStatusNotifierItem::providedToken | ( | ) | const |
- Returns
- the last provided token to be used with Wayland's xdg_activation_v1
Definition at line 674 of file kstatusnotifieritem.cpp.
◆ quitRequested
|
signal |
Emitted when the Quit action is triggered.
If abortQuit() is called from the slot the quit is cancelled. This allows to e.g. display a custom confirmation prompt.
- Since
- 6.5
◆ scrollRequested
|
signal |
Inform the host application that the mouse wheel (or another mean of scrolling that the visualization provides) has been used.
- Parameters
-
delta the amount of scrolling, can be either positive or negative orientation direction of the scrolling, can be either horizontal or vertical
◆ secondaryActivateRequested
|
signal |
Alternate activate action, for instance right mouse click, but this is not guaranteed since it's dependent from the visualization.
- Parameters
-
pos the position in the screen where the user clicked to trigger this signal, QPoint() if it's not the consequence of a mouse click.
◆ setAssociatedWindow()
void KStatusNotifierItem::setAssociatedWindow | ( | QWindow * | window | ) |
Sets the main window associated with this StatusNotifierItem.
- Parameters
-
window The window to be used.
- Since
- 6.0
Definition at line 494 of file kstatusnotifieritem.cpp.
◆ setAttentionIconByName()
void KStatusNotifierItem::setAttentionIconByName | ( | const QString & | name | ) |
Sets a new icon that should be used when the application wants to request attention (usually the systemtray will blink between this icon and the main one)
- Parameters
-
name QIcon::fromTheme compatible name of icon to use
Definition at line 239 of file kstatusnotifieritem.cpp.
◆ setAttentionIconByPixmap()
void KStatusNotifierItem::setAttentionIconByPixmap | ( | const QIcon & | icon | ) |
Sets the pixmap of the requesting attention icon.
Use setAttentionIcon(const QString) instead when possible.
- Parameters
-
icon QIcon to use for requesting attention.
Definition at line 258 of file kstatusnotifieritem.cpp.
◆ setAttentionMovieByName()
void KStatusNotifierItem::setAttentionMovieByName | ( | const QString & | name | ) |
Sets a movie as the requesting attention icon.
This overrides anything set in setAttentionIcon()
Definition at line 278 of file kstatusnotifieritem.cpp.
◆ setCategory()
void KStatusNotifierItem::setCategory | ( | const ItemCategory | category | ) |
Sets the category for this icon, usually it's needed to call this function only once.
- Parameters
-
category the new category for this icon
Definition at line 94 of file kstatusnotifieritem.cpp.
◆ setContextMenu()
void KStatusNotifierItem::setContextMenu | ( | QMenu * | menu | ) |
Sets a new context menu for this StatusNotifierItem.
the menu will be shown with a contextMenu(int,int) call by the systemtray over D-Bus
The KStatusNotifierItem instance takes ownership of the menu, and will delete it upon its destruction.
Definition at line 450 of file kstatusnotifieritem.cpp.
◆ setIconByName()
void KStatusNotifierItem::setIconByName | ( | const QString & | name | ) |
Sets a new main icon for the system tray.
- Parameters
-
name it must be a QIcon::fromTheme compatible name, this is the preferred way to set an icon
Definition at line 133 of file kstatusnotifieritem.cpp.
◆ setIconByPixmap()
void KStatusNotifierItem::setIconByPixmap | ( | const QIcon & | icon | ) |
Sets a new main icon for the system tray.
- Parameters
-
pixmap our icon, use setIcon(const QString) when possible
Definition at line 156 of file kstatusnotifieritem.cpp.
◆ setIsMenu()
void KStatusNotifierItem::setIsMenu | ( | bool | isMenu | ) |
Indictates that this item only supports the context menu.
Instead of sending activate the provided the menu will be shown.
- See also
- setContextMenu
- Since
- 6.14
Definition at line 683 of file kstatusnotifieritem.cpp.
◆ setOverlayIconByName()
void KStatusNotifierItem::setOverlayIconByName | ( | const QString & | name | ) |
Sets an icon to be used as overlay for the main one.
- Parameters
-
icon name, if name is and empty QString() (and overlayIconPixmap() is empty too) the icon will be removed
Definition at line 180 of file kstatusnotifieritem.cpp.
◆ setOverlayIconByPixmap()
void KStatusNotifierItem::setOverlayIconByPixmap | ( | const QIcon & | icon | ) |
Sets an icon to be used as overlay for the main one setOverlayIconByPixmap(QIcon()) will remove the overlay when overlayIconName() is empty too.
- Parameters
-
pixmap our overlay icon, use setOverlayIcon(const QString) when possible.
Definition at line 207 of file kstatusnotifieritem.cpp.
◆ setStandardActionsEnabled()
void KStatusNotifierItem::setStandardActionsEnabled | ( | bool | enabled | ) |
Sets whether to show the standard items in the menu, such as Quit.
Definition at line 575 of file kstatusnotifieritem.cpp.
◆ setStatus()
void KStatusNotifierItem::setStatus | ( | const ItemStatus | status | ) |
Sets a new status for this icon.
Definition at line 114 of file kstatusnotifieritem.cpp.
◆ setTitle()
void KStatusNotifierItem::setTitle | ( | const QString & | title | ) |
Sets a title for this icon.
Definition at line 109 of file kstatusnotifieritem.cpp.
◆ setToolTip() [1/2]
void KStatusNotifierItem::setToolTip | ( | const QIcon & | icon, |
const QString & | title, | ||
const QString & | subTitle ) |
Sets a new toolTip or this status notifier item.
This is an overloaded member provided for convenience
Definition at line 353 of file kstatusnotifieritem.cpp.
◆ setToolTip() [2/2]
void KStatusNotifierItem::setToolTip | ( | const QString & | iconName, |
const QString & | title, | ||
const QString & | subTitle ) |
Sets a new toolTip or this icon, a toolTip is composed of an icon, a title and a text, all fields are optional.
- Parameters
-
iconName a QIcon::fromTheme compatible name for the tootip icon title tootip title subTitle subtitle for the toolTip
Definition at line 335 of file kstatusnotifieritem.cpp.
◆ setToolTipIconByName()
void KStatusNotifierItem::setToolTipIconByName | ( | const QString & | name | ) |
Set a new icon for the toolTip.
- Parameters
-
name the name for the icon
Definition at line 374 of file kstatusnotifieritem.cpp.
◆ setToolTipIconByPixmap()
void KStatusNotifierItem::setToolTipIconByPixmap | ( | const QIcon & | icon | ) |
Set a new icon for the toolTip.
Use setToolTipIconByName(QString) if possible.
- Parameters
-
pixmap representing the icon
Definition at line 392 of file kstatusnotifieritem.cpp.
◆ setToolTipSubTitle()
void KStatusNotifierItem::setToolTipSubTitle | ( | const QString & | subTitle | ) |
Sets a new subtitle for the toolTip.
Definition at line 431 of file kstatusnotifieritem.cpp.
◆ setToolTipTitle()
void KStatusNotifierItem::setToolTipTitle | ( | const QString & | title | ) |
Sets a new title for the toolTip.
Definition at line 412 of file kstatusnotifieritem.cpp.
◆ showMessage()
void KStatusNotifierItem::showMessage | ( | const QString & | title, |
const QString & | message, | ||
const QString & | icon, | ||
int | timeout = 10000 ) |
Shows the user a notification.
If possible use KNotify instead
- Parameters
-
title message title message the actual text shown to the user icon icon to be shown to the user timeout how much time will elapse before hiding the message
Definition at line 603 of file kstatusnotifieritem.cpp.
◆ standardActionsEnabled()
bool KStatusNotifierItem::standardActionsEnabled | ( | ) | const |
- Returns
- if the standard items in the menu, such as Quit
Definition at line 598 of file kstatusnotifieritem.cpp.
◆ status()
KStatusNotifierItem::ItemStatus KStatusNotifierItem::status | ( | ) | const |
- Returns
- the current application status
Definition at line 99 of file kstatusnotifieritem.cpp.
◆ title()
QString KStatusNotifierItem::title | ( | ) | const |
- Returns
- the title of this icon
Definition at line 634 of file kstatusnotifieritem.cpp.
◆ toolTipIconName()
QString KStatusNotifierItem::toolTipIconName | ( | ) | const |
- Returns
- the name of the toolTip icon if toolTipImage() is not empty this will always return an empty string
Definition at line 387 of file kstatusnotifieritem.cpp.
◆ toolTipIconPixmap()
QIcon KStatusNotifierItem::toolTipIconPixmap | ( | ) | const |
- Returns
- a serialization of the toolTip icon data
Definition at line 407 of file kstatusnotifieritem.cpp.
◆ toolTipSubTitle()
QString KStatusNotifierItem::toolTipSubTitle | ( | ) | const |
- Returns
- the subtitle of the main icon toolTip
Definition at line 445 of file kstatusnotifieritem.cpp.
◆ toolTipTitle()
QString KStatusNotifierItem::toolTipTitle | ( | ) | const |
- Returns
- the title of the main icon toolTip
Definition at line 426 of file kstatusnotifieritem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:58:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.