KWallet::Wallet
#include <kwallet.h>
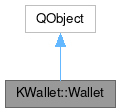
Public Types | |
enum | EntryType { Unknown = 0 , Password , Stream , Map , Unused = 0xffff } |
enum | OpenType { Synchronous = 0 , Asynchronous , Path , OpenTypeUnused = 0xff } |
Signals | |
void | folderListUpdated () |
void | folderRemoved (const QString &folder) |
void | folderUpdated (const QString &folder) |
void | walletClosed () |
void | walletOpened (bool success) |
Public Member Functions | |
~Wallet () override | |
virtual bool | createFolder (const QString &f) |
virtual const QString & | currentFolder () const |
QMap< QString, QByteArray > | entriesList (bool *ok) const |
virtual QStringList | entryList () |
virtual EntryType | entryType (const QString &key) |
virtual QStringList | folderList () |
virtual bool | hasEntry (const QString &key) |
virtual bool | hasFolder (const QString &f) |
virtual bool | isOpen () const |
virtual int | lockWallet () |
QMap< QString, QMap< QString, QString > > | mapList (bool *ok) const |
QMap< QString, QString > | passwordList (bool *ok) const |
virtual int | readEntry (const QString &key, QByteArray &value) |
virtual int | readMap (const QString &key, QMap< QString, QString > &value) |
virtual int | readPassword (const QString &key, QString &value) |
virtual int | removeEntry (const QString &key) |
virtual bool | removeFolder (const QString &f) |
virtual int | renameEntry (const QString &oldName, const QString &newName) |
virtual void | requestChangePassword (WId w) |
virtual bool | setFolder (const QString &f) |
virtual int | sync () |
virtual const QString & | walletName () const |
virtual int | writeEntry (const QString &key, const QByteArray &value) |
virtual int | writeEntry (const QString &key, const QByteArray &value, EntryType entryType) |
virtual int | writeMap (const QString &key, const QMap< QString, QString > &value) |
virtual int | writePassword (const QString &key, const QString &value) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static void | changePassword (const QString &name, WId w) |
static int | closeWallet (const QString &name, bool force) |
static int | deleteWallet (const QString &name) |
static bool | disconnectApplication (const QString &wallet, const QString &app) |
static bool | folderDoesNotExist (const QString &wallet, const QString &folder) |
static const QString | FormDataFolder () |
static bool | isEnabled () |
static bool | isOpen (const QString &name) |
static bool | keyDoesNotExist (const QString &wallet, const QString &folder, const QString &key) |
static const QString | LocalWallet () |
static const QString | NetworkWallet () |
static Wallet * | openWallet (const QString &name, WId w, OpenType ot=Synchronous) |
static const QString | PasswordFolder () |
static QStringList | users (const QString &wallet) |
static QStringList | walletList () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
Wallet (const Wallet &) | |
Wallet (int handle, const QString &name) | |
virtual void | virtual_hook (int id, void *data) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
KDE Wallet.
This class implements a generic system-wide Wallet for KDE. This is the ONLY public interface.
KDE Wallet Class
Member Enumeration Documentation
◆ EntryType
◆ OpenType
Constructor & Destructor Documentation
◆ Wallet() [1/2]
|
protected |
Construct a KWallet object.
- Parameters
-
handle The handle for the wallet. name The name of the wallet.
Definition at line 118 of file kwallet.cpp.
◆ Wallet() [2/2]
|
protected |
Copy a KWallet object.
◆ ~Wallet()
|
override |
Member Function Documentation
◆ changePassword()
|
static |
Request to the wallet service to change the password of the wallet name
.
- Parameters
-
name The wallet to change the password of. w The window id to associate any dialogs with. You can pass 0 if you don't have a window the password dialog should associate with.
Definition at line 174 of file kwallet.cpp.
◆ closeWallet()
|
static |
Close the wallet name
.
The wallet will only be closed if it is open but not in use (rare), or if it is forced closed.
- Parameters
-
name The name of the wallet to close. force Set true to force the wallet closed even if it is in use by others.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 206 of file kwallet.cpp.
◆ createFolder()
|
virtual |
Created the folder f
.
- Parameters
-
f the name of the folder to create
- Returns
- Returns true if the folder was successfully created.
Definition at line 449 of file kwallet.cpp.
◆ currentFolder()
|
virtual |
Determine the current working folder in the wallet.
If the folder name is empty, it is working in the global folder, which is valid but discouraged.
- Returns
- Returns the current working folder.
Definition at line 511 of file kwallet.cpp.
◆ deleteWallet()
|
static |
Delete the wallet name
.
The wallet will be forced closed first.
- Parameters
-
name The name of the wallet to delete.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 222 of file kwallet.cpp.
◆ disconnectApplication()
Disconnect the application app
from wallet
.
- Parameters
-
wallet The name of the wallet to disconnect. app The name of the application to disconnect.
- Returns
- Returns true on success, false on error.
Definition at line 312 of file kwallet.cpp.
◆ entriesList()
QMap< QString, QByteArray > Wallet::entriesList | ( | bool * | ok | ) | const |
Get a list of all the entries in the current folder.
The entry format is unknown except that it is either a QByteArray or a QDataStream, which effectively means that it could be anything.
- Parameters
-
ok if not nullptr, the object this parameter points to will be set to true to indicate success or false otherwise
- Returns
- a map of key/value pairs where the key in the map is the entry key
- Since
- 5.72
Definition at line 533 of file kwallet.cpp.
◆ entryList()
|
virtual |
Return the list of keys of all entries in this folder.
- Returns
- Returns an empty list if the wallet is not open, or if the folder is empty.
Definition at line 419 of file kwallet.cpp.
◆ entryType()
|
virtual |
Determine the type of the entry key
in this folder.
- Parameters
-
key The key to look up.
- Returns
- Returns an enumerated type representing the type of the entry.
Definition at line 781 of file kwallet.cpp.
◆ folderDoesNotExist()
Determine if a folder does not exist in a wallet.
This does not require decryption of the wallet. This is a handy optimization to avoid prompting the user if your data is certainly not in the wallet.
- Parameters
-
wallet The wallet to look in. folder The folder to look up.
- Returns
- Returns true if the folder does NOT exist in the wallet, or the wallet does not exist.
Definition at line 848 of file kwallet.cpp.
◆ folderList()
|
virtual |
Obtain the list of all folders contained in the wallet.
- Returns
- Returns an empty list if the wallet is not open.
Definition at line 404 of file kwallet.cpp.
◆ folderListUpdated
|
signal |
Emitted when the folder list is changed in this wallet.
◆ folderRemoved
|
signal |
Emitted when a folder in this wallet is removed.
- Parameters
-
folder The folder that was removed.
◆ folderUpdated
|
signal |
Emitted when a folder in this wallet is updated.
- Parameters
-
folder The folder that was updated.
◆ FormDataFolder()
|
static |
The standardized name of the form data folder.
It is automatically created when a wallet is created, but the user may still delete it so you should check for its existence and recreate it if necessary and desired.
Definition at line 92 of file kwallet.cpp.
◆ hasEntry()
|
virtual |
Determine if the current folder has they entry key
.
- Parameters
-
key The key to search for.
- Returns
- Returns true if the folder contains
key
.
Definition at line 750 of file kwallet.cpp.
◆ hasFolder()
|
virtual |
Determine if the folder f
exists in the wallet.
- Parameters
-
f the name of the folder to check for
- Returns
- Returns true if the folder exists in the wallet.
Definition at line 434 of file kwallet.cpp.
◆ isEnabled()
|
static |
Determine if the KDE wallet is enabled.
Normally you do not need to use this because openWallet() will just fail.
- Returns
- Returns true if the wallet enabled, else false.
Definition at line 185 of file kwallet.cpp.
◆ isOpen() [1/2]
|
virtual |
Determine if the current wallet is open, and is a valid wallet handle.
- Returns
- Returns true if the wallet handle is valid and open.
Definition at line 376 of file kwallet.cpp.
◆ isOpen() [2/2]
|
static |
Determine if the wallet name
is open by any application.
- Parameters
-
name The name of the wallet to check.
- Returns
- Returns true if the wallet is open, else false.
Definition at line 190 of file kwallet.cpp.
◆ keyDoesNotExist()
|
static |
Determine if an entry in a folder does not exist in a wallet.
This does not require decryption of the wallet. This is a handy optimization to avoid prompting the user if your data is certainly not in the wallet.
- Parameters
-
wallet The wallet to look in. folder The folder to look in. key The key to look up.
- Returns
- Returns true if the key does NOT exist in the wallet, or the folder or wallet does not exist.
Definition at line 863 of file kwallet.cpp.
◆ LocalWallet()
|
static |
The name of the wallet used to store local passwords.
Definition at line 58 of file kwallet.cpp.
◆ lockWallet()
|
virtual |
This closes and locks the current wallet.
It will disconnect all applications using the wallet.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 353 of file kwallet.cpp.
◆ mapList()
Get a list of all the maps in the current folder.
- Parameters
-
ok if not nullptr, the object this parameter points to will be set to true to indicate success or false otherwise. Note that if any of the keys was not originally written as a map, the object will be set to false
- Returns
- a map of key/value pairs where the key in the map is the entry key
- Since
- 5.72
Definition at line 604 of file kwallet.cpp.
◆ NetworkWallet()
|
static |
The name of the wallet used to store network passwords.
Definition at line 76 of file kwallet.cpp.
◆ openWallet()
Open the wallet name
.
The user will be prompted to allow your application to open the wallet, and may be prompted for a password. You are responsible for deleting this object when you are done with it.
- Parameters
-
name The name of the wallet to open. ot If Asynchronous, the call will return immediately with a non-null pointer to an invalid wallet. You must immediately connect the walletOpened() signal to a slot so that you will know when it is opened, or when it fails. w The window id to associate any dialogs with. You can pass 0 if you don't have a window the password dialog should associate with.
- Returns
- Returns a pointer to the wallet if successful, or a null pointer on error or if rejected. A null pointer can also be returned if user choose to deactivate the wallet system.
Definition at line 238 of file kwallet.cpp.
◆ PasswordFolder()
|
static |
The standardized name of the password folder.
It is automatically created when a wallet is created, but the user may still delete it so you should check for its existence and recreate it if necessary and desired.
Definition at line 87 of file kwallet.cpp.
◆ passwordList()
Get a list of all the passwords in the current folder, which can be used to populate a list view in a password manager.
- Parameters
-
ok if not nullptr, the object this parameter points to will be set to true to indicate success or false otherwise. Note that the object will be set to false if any of the keys was not originally written as a password
- Returns
- a map of key/value pairs, where the key in the map is the entry key
- Since
- 5.72
Definition at line 654 of file kwallet.cpp.
◆ readEntry()
|
virtual |
Read the entry key
from the current folder.
The entry format is unknown except that it is either a QByteArray or a QDataStream, which effectively means that it is anything.
- Parameters
-
key The key of the entry to read. value A buffer to fill with the value.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 516 of file kwallet.cpp.
◆ readMap()
Read the map entry key
from the current folder.
- Parameters
-
key The key of the entry to read. value A map buffer to fill with the value.
- Returns
- Returns 0 on success, non-zero on error. Will return an error if the key was not originally written as a map.
Definition at line 581 of file kwallet.cpp.
◆ readPassword()
Read the password entry key
from the current folder.
- Parameters
-
key The key of the entry to read. value A password buffer to fill with the value.
- Returns
- Returns 0 on success, non-zero on error. Will return an error if the key was not originally written as a password.
Definition at line 637 of file kwallet.cpp.
◆ removeEntry()
|
virtual |
Remove the entry key
from the current folder.
- Parameters
-
key The key to remove.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 765 of file kwallet.cpp.
◆ removeFolder()
|
virtual |
Remove the folder f
and all its entries from the wallet.
- Parameters
-
f the name of the folder to remove
- Returns
- Returns true if the folder was successfully removed.
Definition at line 492 of file kwallet.cpp.
◆ renameEntry()
Rename the entry oldName
to newName
.
- Parameters
-
oldName The original key of the entry. newName The new key of the entry.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 561 of file kwallet.cpp.
◆ requestChangePassword()
|
virtual |
Request to the wallet service to change the password of the current wallet.
- Parameters
-
w The window id to associate any dialogs with. You can pass 0 if you don't have a window the password dialog should associate with.
Definition at line 381 of file kwallet.cpp.
◆ setFolder()
|
virtual |
Set the current working folder to f
.
The folder must exist, or this call will fail. Create a folder with createFolder().
- Parameters
-
f the name of the folder to make the working folder
- Returns
- Returns true if the folder was successfully set.
Definition at line 469 of file kwallet.cpp.
◆ sync()
|
virtual |
This syncs the wallet file on disk with what is in memory.
You don't normally need to use this. It happens automatically on close.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 343 of file kwallet.cpp.
◆ users()
|
static |
List the applications that are using the wallet wallet
.
- Parameters
-
wallet The wallet to query.
- Returns
- Returns a list of all D-Bus application IDs using the wallet.
Definition at line 328 of file kwallet.cpp.
◆ virtual_hook()
|
protectedvirtual |
Definition at line 878 of file kwallet.cpp.
◆ walletClosed
|
signal |
Emitted when this wallet is closed.
◆ walletList()
|
static |
List all the wallets available.
- Returns
- Returns a list of the names of all wallets that are open.
Definition at line 159 of file kwallet.cpp.
◆ walletName()
|
virtual |
The name of the current wallet.
Definition at line 371 of file kwallet.cpp.
◆ walletOpened
|
signal |
Emitted when a wallet is opened in asynchronous mode.
- Parameters
-
success True if the wallet was opened successfully.
◆ writeEntry() [1/2]
|
virtual |
Write key
= value
as a binary entry to the current folder.
- Parameters
-
key The key of the new entry. value The value of the entry.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 697 of file kwallet.cpp.
◆ writeEntry() [2/2]
|
virtual |
Write key
= value
as a binary entry to the current folder.
Be careful with this, it could cause inconsistency in the future since you can put an arbitrary entry type in place.
- Parameters
-
key The key of the new entry. value The value of the entry. entryType The type of the entry.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 681 of file kwallet.cpp.
◆ writeMap()
Write key
= value
as a map to the current folder.
- Parameters
-
key The key of the new entry. value The value of the map.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 713 of file kwallet.cpp.
◆ writePassword()
Write key
= value
as a password to the current folder.
- Parameters
-
key The key of the new entry. value The value of the password.
- Returns
- Returns 0 on success, non-zero on error.
Definition at line 734 of file kwallet.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:50 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.