KSelectionOwner
#include <kselectionowner.h>
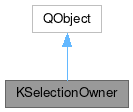
Signals | |
void | claimedOwnership () |
void | failedToClaimOwnership () |
void | lostOwnership () |
Public Member Functions | |
KSelectionOwner (const char *selection, int screen=-1, QObject *parent=nullptr) | |
KSelectionOwner (const char *selection, xcb_connection_t *c, xcb_window_t root, QObject *parent=nullptr) | |
KSelectionOwner (xcb_atom_t selection, int screen=-1, QObject *parent=nullptr) | |
KSelectionOwner (xcb_atom_t selection, xcb_connection_t *c, xcb_window_t root, QObject *parent=nullptr) | |
~KSelectionOwner () override | |
void | claim (bool force, bool force_kill=true) |
bool | filterEvent (void *ev_P) |
xcb_window_t | ownerWindow () const |
void | release () |
void | timerEvent (QTimerEvent *event) override |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
virtual bool | genericReply (xcb_atom_t target, xcb_atom_t property, xcb_window_t requestor) |
virtual void | getAtoms () |
virtual void | replyTargets (xcb_atom_t property, xcb_window_t requestor) |
void | setData (uint32_t extra1, uint32_t extra2) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
This class implements claiming and owning manager selections, as described in the ICCCM, section 2.8.
The selection atom is passed to the constructor, claim() attempts to claim ownership of the selection, release() gives up the selection ownership. Signal lostOwnership() is emitted when the selection is claimed by another owner.
ICCCM manager selection owner
This class is only useful on the xcb platform. On other platforms the code is only functional if the constructor overloads taking an xcb_connection_t are used. In case you inherit from this class ensure that you don't use xcb and/or XLib without verifying the platform.
Definition at line 29 of file kselectionowner.h.
Constructor & Destructor Documentation
◆ KSelectionOwner() [1/4]
|
explicit |
This constructor initializes the object, but doesn't perform any operation on the selection.
- Parameters
-
selection atom representing the manager selection screen X screen, or -1 for default parent parent object, or nullptr if there is none
Definition at line 133 of file kselectionowner.cpp.
◆ KSelectionOwner() [2/4]
|
explicit |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. This constructor accepts the selection name and creates the appropriate atom for it automatically.
- Parameters
-
selection name of the manager selection screen X screen, or -1 for default parent parent object, or nullptr if there is none
Definition at line 139 of file kselectionowner.cpp.
◆ KSelectionOwner() [3/4]
|
explicit |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. This constructor accepts the xcb_connection_t and root window and doesn't depend on running on the xcb platform.
Otherwise this constructor behaves like the similar one without the xcb_connection_t.
- Parameters
-
selection atom representing the manager selection c the xcb connection this KSelectionWatcher should use root the root window this KSelectionWatcher should use parent parent object, or nullptr if there is none
- Since
- 5.8
Definition at line 145 of file kselectionowner.cpp.
◆ KSelectionOwner() [4/4]
|
explicit |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. This constructor accepts the xcb_connection_t and root window and doesn't depend on running on the xcb platform.
Otherwise this constructor behaves like the similar one without the xcb_connection_t.
- Parameters
-
selection name of the manager selection c the xcb connection this KSelectionWatcher should use root the root window this KSelectionWatcher should use parent parent object, or nullptr if there is none
- Since
- 5.8
Definition at line 151 of file kselectionowner.cpp.
◆ ~KSelectionOwner()
|
override |
Member Function Documentation
◆ claim()
void KSelectionOwner::claim | ( | bool | force, |
bool | force_kill = true ) |
Try to claim ownership of the manager selection using the current X timestamp.
This function returns immediately, but it may take up to one second for the claim to succeed or fail, at which point either the claimedOwnership() or failedToClaimOwnership() signal is emitted. The claim will not be completed until the caller has returned to the event loop.
If force
is false, and the selection is already owned, the selection is not claimed, and failedToClaimOwnership() is emitted. If force
is true and the selection is owned by another client, the client will be given one second to relinquish ownership of the selection. If force_kill
is true, and the previous owner fails to disown the selection in time, it will be forcibly killed.
Definition at line 245 of file kselectionowner.cpp.
◆ claimedOwnership
|
signal |
This signal is emitted when claim() was successful in claiming ownership of the selection.
◆ failedToClaimOwnership
|
signal |
This signal is emitted when claim() failed to claim ownership of the selection.
◆ filterEvent()
bool KSelectionOwner::filterEvent | ( | void * | ev_P | ) |
Definition at line 341 of file kselectionowner.cpp.
◆ genericReply()
|
protectedvirtual |
Called for every X event received on the window used for owning the selection.
If true is returned, the event is filtered out. Called when a SelectionRequest event is received. A reply should be sent using the selection handling mechanism described in the ICCCM section 2.
- Parameters
-
target requested target type property property to use for the reply data requestor requestor window
Definition at line 571 of file kselectionowner.cpp.
◆ getAtoms()
|
protectedvirtual |
Called to create atoms needed for claiming the selection and communication using the selection handling mechanism.
The default implementation must be called if reimplemented. This method may be called repeatedly.
Definition at line 576 of file kselectionowner.cpp.
◆ lostOwnership
|
signal |
This signal is emitted if the selection was owned and the ownership has been lost due to another client claiming it, this signal is emitted.
IMPORTANT: It's not safe to delete the instance in a slot connected to this signal.
◆ ownerWindow()
xcb_window_t KSelectionOwner::ownerWindow | ( | ) | const |
If the selection is owned, returns the window used internally for owning the selection.
Definition at line 320 of file kselectionowner.cpp.
◆ release()
void KSelectionOwner::release | ( | ) |
If the selection is owned, the ownership is given up.
Definition at line 303 of file kselectionowner.cpp.
◆ replyTargets()
|
protectedvirtual |
Called to announce the supported targets, as described in the ICCCM section 2.6.
The default implementation announces the required targets MULTIPLE, TIMESTAMP and TARGETS.
Definition at line 552 of file kselectionowner.cpp.
◆ setData()
|
protected |
Sets extra data to be sent in the message sent to root window after successfully claiming a selection.
These extra data are in data.l[3] and data.l[4] fields of the XClientMessage.
Definition at line 332 of file kselectionowner.cpp.
◆ timerEvent()
|
overridevirtual |
Reimplemented from QObject.
Definition at line 425 of file kselectionowner.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:23 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.