Solid::Battery
#include <battery.h>
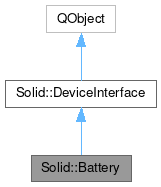
Public Types | |
enum | BatteryType { UnknownBattery , PdaBattery , UpsBattery , PrimaryBattery , MouseBattery , KeyboardBattery , KeyboardMouseBattery , CameraBattery , PhoneBattery , MonitorBattery , GamingInputBattery , BluetoothBattery , TabletBattery , HeadphoneBattery , HeadsetBattery , TouchpadBattery } |
enum | ChargeState { NoCharge , Charging , Discharging , FullyCharged } |
enum | Technology { UnknownTechnology = 0 , LithiumIon , LithiumPolymer , LithiumIronPhosphate , LeadAcid , NickelCadmium , NickelMetalHydride } |
![]() | |
enum | Type { Unknown = 0 , GenericInterface = 1 , Processor = 2 , Block = 3 , StorageAccess = 4 , StorageDrive = 5 , OpticalDrive = 6 , StorageVolume = 7 , OpticalDisc = 8 , Camera = 9 , PortableMediaPlayer = 10 , Battery = 12 , NetworkShare = 14 , Last = 0xffff } |
![]() | |
typedef | QObjectList |
Properties | |
int | capacity |
int | chargePercent |
ChargeState | chargeState |
int | cycleCount |
double | energy |
double | energyFull |
double | energyFullDesign |
double | energyRate |
bool | powerSupply |
bool | present |
bool | rechargeable |
qlonglong | remainingTime |
QString | serial |
Technology | technology |
double | temperature |
qlonglong | timeToEmpty |
qlonglong | timeToFull |
BatteryType | type |
double | voltage |
![]() | |
objectName | |
Signals | |
void | capacityChanged (int value, const QString &udi) |
void | chargePercentChanged (int value, const QString &udi) |
void | chargeStateChanged (int newState, const QString &udi=QString()) |
void | cycleCountChanged (int value, const QString &udi) |
void | energyChanged (double energy, const QString &udi) |
void | energyFullChanged (double energy, const QString &udi) |
void | energyFullDesignChanged (double energy, const QString &udi) |
void | energyRateChanged (double energyRate, const QString &udi) |
void | powerSupplyStateChanged (bool newState, const QString &udi) |
void | presentStateChanged (bool newState, const QString &udi) |
void | remainingTimeChanged (qlonglong time, const QString &udi) |
void | temperatureChanged (double temperature, const QString &udi) |
void | timeToEmptyChanged (qlonglong time, const QString &udi) |
void | timeToFullChanged (qlonglong time, const QString &udi) |
void | voltageChanged (double voltage, const QString &udi) |
Public Member Functions | |
~Battery () override | |
int | capacity () const |
int | chargePercent () const |
Solid::Battery::ChargeState | chargeState () const |
int | cycleCount () const |
double | energy () const |
double | energyFull () const |
double | energyFullDesign () const |
double | energyRate () const |
bool | isPowerSupply () const |
bool | isPresent () const |
bool | isRechargeable () const |
qlonglong | remainingTime () const |
QString | serial () const |
Solid::Battery::Technology | technology () const |
double | temperature () const |
qlonglong | timeToEmpty () const |
qlonglong | timeToFull () const |
Solid::Battery::BatteryType | type () const |
double | voltage () const |
![]() | |
~DeviceInterface () override | |
bool | isValid () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static Type | deviceInterfaceType () |
![]() | |
static Type | stringToType (const QString &type) |
static QString | typeDescription (Type type) |
static QString | typeToString (Type type) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
SOLID_NO_EXPORT | DeviceInterface (DeviceInterfacePrivate &dd, QObject *backendObject) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
DeviceInterfacePrivate * | d_ptr |
Detailed Description
This device interface is available on batteries.
Definition at line 24 of file frontend/battery.h.
Member Enumeration Documentation
◆ BatteryType
This enum type defines the type of the device holding the battery.
- PdaBattery : A battery in a Personal Digital Assistant
- UpsBattery : A battery in an Uninterruptible Power Supply
- PrimaryBattery : A primary battery for the system (for example laptop battery)
- MouseBattery : A battery in a mouse
- KeyboardBattery : A battery in a keyboard
- KeyboardMouseBattery : A battery in a combined keyboard and mouse
- CameraBattery : A battery in a camera
- PhoneBattery : A battery in a phone
- MonitorBattery : A battery in a monitor
- GamingInputBattery : A battery in a gaming input device (for example a wireless game pad)
- BluetoothBattery: A generic Bluetooth device battery (if its type isn't known, a Bluetooth mouse would normally show up as a MouseBattery),
- Since
- 5.54
- TabletBattery : A battery in a graphics tablet or pen,
- Since
- 5.88
- HeadphoneBattery : A battery in a headphone,
- Since
- 6.0
- HeadsetBattery : A battery in a headset,
- Since
- 6.0
- TouchpadBattery : A battery in a touchpad. This is how the Dualsense Wireless Controller is categorized
- Since
- 6.0
- UnknownBattery : A battery in an unknown device
Definition at line 71 of file frontend/battery.h.
◆ ChargeState
This enum type defines charge state of a battery.
- NoCharge : Battery charge is stable, not charging or discharging or the state is Unknown
- Charging : Battery is charging
- Discharging : Battery is discharging
- FullyCharged: The battery is fully charged; a battery not necessarily charges up to 100%
Definition at line 101 of file frontend/battery.h.
◆ Technology
Technology used in the battery.
0: Unknown 1: Lithium ion 2: Lithium polymer 3: Lithium iron phosphate 4: Lead acid 5: Nickel cadmium 6: Nickel metal hydride
Definition at line 115 of file frontend/battery.h.
Property Documentation
◆ capacity
|
read |
Definition at line 30 of file frontend/battery.h.
◆ chargePercent
|
read |
Definition at line 29 of file frontend/battery.h.
◆ chargeState
|
read |
Definition at line 34 of file frontend/battery.h.
◆ cycleCount
|
read |
Definition at line 31 of file frontend/battery.h.
◆ energy
|
read |
Definition at line 37 of file frontend/battery.h.
◆ energyFull
|
read |
Definition at line 38 of file frontend/battery.h.
◆ energyFullDesign
|
read |
Definition at line 39 of file frontend/battery.h.
◆ energyRate
|
read |
Definition at line 40 of file frontend/battery.h.
◆ powerSupply
|
read |
Definition at line 33 of file frontend/battery.h.
◆ present
|
read |
Definition at line 27 of file frontend/battery.h.
◆ rechargeable
|
read |
Definition at line 32 of file frontend/battery.h.
◆ remainingTime
|
read |
Definition at line 45 of file frontend/battery.h.
◆ serial
|
read |
Definition at line 44 of file frontend/battery.h.
◆ technology
|
read |
Definition at line 43 of file frontend/battery.h.
◆ temperature
|
read |
Definition at line 42 of file frontend/battery.h.
◆ timeToEmpty
|
read |
Definition at line 35 of file frontend/battery.h.
◆ timeToFull
|
read |
Definition at line 36 of file frontend/battery.h.
◆ type
|
read |
Definition at line 28 of file frontend/battery.h.
◆ voltage
|
read |
Definition at line 41 of file frontend/battery.h.
Constructor & Destructor Documentation
◆ ~Battery()
|
override |
Destroys a Battery object.
Definition at line 49 of file frontend/battery.cpp.
Member Function Documentation
◆ capacity()
int Solid::Battery::capacity | ( | ) | const |
Retrieves the battery capacity normalised to percent, meaning how much energy can it hold compared to what it is designed to.
The capacity of the battery will reduce with age. A capacity value less than 75% is usually a sign that you should renew your battery.
- Since
- 4.11
- Returns
- the battery capacity normalised to percent
Definition at line 71 of file frontend/battery.cpp.
◆ capacityChanged
|
signal |
This signal is emitted when the capacity of this battery has changed.
- Parameters
-
value the new capacity of the battery udi the UDI of the battery with the new capacity
- Since
- 4.11
◆ chargePercent()
int Solid::Battery::chargePercent | ( | ) | const |
Retrieves the current charge level of the battery normalised to percent.
- Returns
- the current charge level normalised to percent
Definition at line 65 of file frontend/battery.cpp.
◆ chargePercentChanged
|
signal |
This signal is emitted when the charge percent value of this battery has changed.
- Parameters
-
value the new charge percent value of the battery udi the UDI of the battery with the new charge percent
◆ chargeState()
Solid::Battery::ChargeState Solid::Battery::chargeState | ( | ) | const |
Retrieves the current charge state of the battery.
It can be in a stable state (no charge), charging or discharging.
- Returns
- the current battery charge state
- See also
- Solid::Battery::ChargeState
Definition at line 95 of file frontend/battery.cpp.
◆ chargeStateChanged
This signal is emitted when the charge state of this battery has changed.
- Parameters
-
newState the new charge state of the battery, it's one of the type Solid::Battery::ChargeState
- See also
- Solid::Battery::ChargeState
- Parameters
-
udi the UDI of the battery with the new charge state
◆ cycleCount()
int Solid::Battery::cycleCount | ( | ) | const |
Retrieves the number of charge cycles this battery has experienced so far, or -1 if this information is unavailable.
- Since
- 6.9
- Returns
- the number of charge cycles
Definition at line 77 of file frontend/battery.cpp.
◆ cycleCountChanged
|
signal |
This signal is emitted when the number of charge cycles of the battery has changed.
- Parameters
-
value the new number of charge cycles of the battery udi the UDI of the battery with the new number of charge cycles
- Since
- 6.9
◆ deviceInterfaceType()
|
inlinestatic |
Get the Solid::DeviceInterface::Type of the Battery device interface.
- Returns
- the Battery device interface type
- See also
- Solid::DeviceInterface::Type
Definition at line 149 of file frontend/battery.h.
◆ energy()
double Solid::Battery::energy | ( | ) | const |
Amount of energy (measured in Wh) currently available in the power source.
- Returns
- amount of battery energy in Wh
Definition at line 119 of file frontend/battery.cpp.
◆ energyChanged
|
signal |
This signal is emitted when the energy value of this battery has changed.
- Parameters
-
energy the new energy value of the battery udi the UDI of the battery with the new energy value
◆ energyFull()
double Solid::Battery::energyFull | ( | ) | const |
Amount of energy (measured in Wh) the battery has when it is full.
- Returns
- amount of battery energy when full in Wh
- Since
- 5.7
Definition at line 125 of file frontend/battery.cpp.
◆ energyFullChanged
|
signal |
This signal is emitted when the energy full value of this battery has changed.
- Parameters
-
energy the new energy full value of the battery udi the UDI of the battery with the new energy full value
◆ energyFullDesign()
double Solid::Battery::energyFullDesign | ( | ) | const |
Amount of energy (measured in Wh) the battery should have by design hen it is full.
- Returns
- amount of battery energy when full by design in Wh
- Since
- 5.7
Definition at line 131 of file frontend/battery.cpp.
◆ energyFullDesignChanged
|
signal |
This signal is emitted when the energy full design value of this battery has changed.
- Parameters
-
energy the new energy full design value of the battery udi the UDI of the battery with the new energy full design value
◆ energyRate()
double Solid::Battery::energyRate | ( | ) | const |
Amount of energy being drained from the source, measured in W.
If positive, the source is being discharged, if negative it's being charged.
- Returns
- battery rate in Watts
Definition at line 137 of file frontend/battery.cpp.
◆ energyRateChanged
|
signal |
This signal is emitted when the energy rate value of this battery has changed.
If positive, the source is being discharged, if negative it's being charged.
- Parameters
-
energyRate the new energy rate value of the battery udi the UDI of the battery with the new charge percent
◆ isPowerSupply()
bool Solid::Battery::isPowerSupply | ( | ) | const |
Indicates if the battery is powering the machine.
- Returns
- true if the battery is powersupply, false otherwise
Definition at line 89 of file frontend/battery.cpp.
◆ isPresent()
bool Solid::Battery::isPresent | ( | ) | const |
Indicates if this battery is currently present in its bay.
- Returns
- true if the battery is present, false otherwise
Definition at line 53 of file frontend/battery.cpp.
◆ isRechargeable()
bool Solid::Battery::isRechargeable | ( | ) | const |
Indicates if the battery is rechargeable.
- Returns
- true if the battery is rechargeable, false otherwise (one time usage)
Definition at line 83 of file frontend/battery.cpp.
◆ powerSupplyStateChanged
|
signal |
This signal is emitted when the power supply state of the battery changes.
- Parameters
-
newState the new power supply state, type is boolean udi the UDI of the battery with the new power supply state
- Since
- 4.11
◆ presentStateChanged
|
signal |
This signal is emitted if the battery gets plugged in/out of the battery bay.
- Parameters
-
newState the new plugging state of the battery, type is boolean udi the UDI of the battery with thew new plugging state
◆ remainingTime()
qlonglong Solid::Battery::remainingTime | ( | ) | const |
Retrieves the current estimated remaining time of the system batteries.
- Returns
- the current global estimated remaining time in seconds
- Since
- 5.8
Definition at line 161 of file frontend/battery.cpp.
◆ remainingTimeChanged
|
signal |
This signal is emitted when the estimated battery remaining time changes.
- Parameters
-
time the new remaining time udi the UDI of the battery with the new remaining time
- Since
- 5.8
◆ serial()
QString Solid::Battery::serial | ( | ) | const |
The serial number of the battery.
- Returns
- the serial number of the battery
- Since
- 5.0
Definition at line 155 of file frontend/battery.cpp.
◆ technology()
Solid::Battery::Technology Solid::Battery::technology | ( | ) | const |
Retrieves the technology used to manufacture the battery.
- Returns
- the battery technology
- See also
- Solid::Battery::Technology
Definition at line 113 of file frontend/battery.cpp.
◆ temperature()
double Solid::Battery::temperature | ( | ) | const |
The temperature of the battery in degrees Celsius.
- Returns
- the battery temperature in degrees Celsius
- Since
- 5.0
Definition at line 149 of file frontend/battery.cpp.
◆ temperatureChanged
|
signal |
This signal is emitted when the battery temperature has changed.
- Parameters
-
temperature the new temperature of the battery in degrees Celsius udi the UDI of the battery with the new temperature
- Since
- 5.0
◆ timeToEmpty()
qlonglong Solid::Battery::timeToEmpty | ( | ) | const |
Time (in seconds) until the battery is empty.
- Returns
- time until the battery is empty
- Since
- 5.0
Definition at line 101 of file frontend/battery.cpp.
◆ timeToEmptyChanged
|
signal |
This signal is emitted when the time until the battery is empty has changed.
- Parameters
-
time the new remaining time udi the UDI of the battery with the new remaining time
- Since
- 5.0
◆ timeToFull()
qlonglong Solid::Battery::timeToFull | ( | ) | const |
Time (in seconds) until the battery is full.
- Returns
- time until the battery is full
- Since
- 5.0
Definition at line 107 of file frontend/battery.cpp.
◆ timeToFullChanged
|
signal |
This signal is emitted when the time until the battery is full has changed.
- Parameters
-
time the new remaining time udi the UDI of the battery with the new remaining time
- Since
- 5.0
◆ type()
Solid::Battery::BatteryType Solid::Battery::type | ( | ) | const |
Retrieves the type of device holding this battery.
- Returns
- the type of device holding this battery
- See also
- Solid::Battery::BatteryType
Definition at line 59 of file frontend/battery.cpp.
◆ voltage()
double Solid::Battery::voltage | ( | ) | const |
Voltage in the Cell or being recorded by the meter.
- Returns
- voltage in Volts
Definition at line 143 of file frontend/battery.cpp.
◆ voltageChanged
|
signal |
This signal is emitted when the voltage in the cell has changed.
- Parameters
-
voltage the new voltage of the cell udi the UDI of the battery with the new voltage
- Since
- 5.0
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:57:03 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.