Solid::GenericInterface
#include <Solid/GenericInterface>
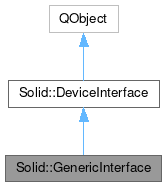
Public Types | |
enum | PropertyChange { PropertyModified , PropertyAdded , PropertyRemoved } |
![]() | |
enum | Type { Unknown = 0 , GenericInterface = 1 , Processor = 2 , Block = 3 , StorageAccess = 4 , StorageDrive = 5 , OpticalDrive = 6 , StorageVolume = 7 , OpticalDisc = 8 , Camera = 9 , PortableMediaPlayer = 10 , Battery = 12 , NetworkShare = 14 , Last = 0xffff } |
Signals | |
void | conditionRaised (const QString &condition, const QString &reason) |
void | propertyChanged (const QMap< QString, int > &changes) |
Public Member Functions | |
~GenericInterface () override | |
QMap< QString, QVariant > | allProperties () const |
QVariant | property (const QString &key) const |
bool | propertyExists (const QString &key) const |
![]() | |
~DeviceInterface () override | |
bool | isValid () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static Type | deviceInterfaceType () |
![]() | |
static Type | stringToType (const QString &type) |
static QString | typeDescription (Type type) |
static QString | typeToString (Type type) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
SOLID_NO_EXPORT | DeviceInterface (DeviceInterfacePrivate &dd, QObject *backendObject) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
DeviceInterfacePrivate * | d_ptr |
Detailed Description
Generic interface to deal with a device.
It exposes a set of properties and is organized as a key/value set.
Warning: Using this class could expose some backend specific details and lead to non portable code. Use it at your own risk, or during transitional phases when the provided device interfaces don't provide the necessary methods.
Definition at line 32 of file frontend/genericinterface.h.
Member Enumeration Documentation
◆ PropertyChange
This enum type defines the type of change that can occur to a GenericInterface property.
- PropertyModified : A property value has changed in the device
- PropertyAdded : A new property has been added to the device
- PropertyRemoved : A property has been removed from the device
Definition at line 47 of file frontend/genericinterface.h.
Constructor & Destructor Documentation
◆ ~GenericInterface()
|
override |
Destroys a Processor object.
Definition at line 22 of file frontend/genericinterface.cpp.
Member Function Documentation
◆ allProperties()
Retrieves a key/value map of all the known properties for the device.
Warning: Using this method could expose some backend specific details and lead to non portable code. Use it at your own risk, or during transitional phases when the provided device interfaces don't provide the necessary methods.
- Returns
- all the properties of the device
Definition at line 32 of file frontend/genericinterface.cpp.
◆ conditionRaised
|
signal |
This signal is emitted when an event occurred in the device.
For example when a button is pressed.
- Parameters
-
condition the condition name reason a message explaining why the condition has been raised
◆ deviceInterfaceType()
|
inlinestatic |
Get the Solid::DeviceInterface::Type of the GenericInterface device interface.
- Returns
- the Processor device interface type
- See also
- Solid::Ifaces::Enums::DeviceInterface::Type
Definition at line 77 of file frontend/genericinterface.h.
◆ property()
Retrieves a property of the device.
Warning: Using this method could expose some backend specific details and lead to non portable code. Use it at your own risk, or during transitional phases when the provided device interfaces don't provide the necessary methods.
- Parameters
-
key the property key
- Returns
- the actual value of the property, or QVariant() if the property is unknown
Definition at line 26 of file frontend/genericinterface.cpp.
◆ propertyChanged
This signal is emitted when a property is changed in the device.
- Parameters
-
changes the map describing the property changes that occurred in the device, keys are property name and values describe the kind of change done on the device property (added/removed/modified), it's one of the type Solid::Device::PropertyChange
◆ propertyExists()
bool Solid::GenericInterface::propertyExists | ( | const QString & | key | ) | const |
Tests if a property exist in the device.
Warning: Using this method could expose some backend specific details and lead to non portable code. Use it at your own risk, or during transitional phases when the provided device interfaces don't provide the necessary methods.
- Parameters
-
key the property key
- Returns
- true if the property is available in the device, false otherwise
Definition at line 38 of file frontend/genericinterface.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:55:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.