Solid::Ifaces::Device
#include <device.h>
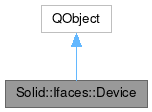
Public Member Functions | |
Device (QObject *parent=nullptr) | |
~Device () override | |
void | broadcastActionDone (const QString &actionName, int error=Solid::NoError, const QString &errorString=QString()) const |
void | broadcastActionRequested (const QString &actionName) const |
virtual QObject * | createDeviceInterface (const Solid::DeviceInterface::Type &type)=0 |
virtual QString | description () const =0 |
virtual QString | displayName () const |
virtual QStringList | emblems () const =0 |
virtual QString | icon () const =0 |
virtual QString | parentUdi () const |
virtual QString | product () const =0 |
virtual bool | queryDeviceInterface (const Solid::DeviceInterface::Type &type) const =0 |
void | registerAction (const QString &actionName, QObject *dest, const char *requestSlot, const char *doneSlot) const |
virtual QString | udi () const =0 |
virtual QString | vendor () const =0 |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class specifies the interface a device will have to comply to in order to be used in the system.
Backends will have to implement it to gather and modify data in the underlying system. Each device has a set of key/values pair describing its properties. It has also a list of interfaces describing what the device actually is (a cdrom drive, a portable media player, etc.)
Definition at line 32 of file ifaces/device.h.
Constructor & Destructor Documentation
◆ Device()
Solid::Ifaces::Device::Device | ( | QObject * | parent = nullptr | ) |
Constructs a Device.
Definition at line 14 of file ifaces/device.cpp.
◆ ~Device()
|
override |
Destruct the Device object.
Definition at line 19 of file ifaces/device.cpp.
Member Function Documentation
◆ broadcastActionDone()
void Solid::Ifaces::Device::broadcastActionDone | ( | const QString & | actionName, |
int | error = Solid::NoError, | ||
const QString & | errorString = QString() ) const |
Allows to broadcast that an action just completed in a device to all the corresponding devices in other processes.
- Parameters
-
actionName name of the action which just completed error error code if the action failed errorString message describing a potential error
Definition at line 39 of file ifaces/device.cpp.
◆ broadcastActionRequested()
void Solid::Ifaces::Device::broadcastActionRequested | ( | const QString & | actionName | ) | const |
Allows to broadcast that an action just got requested on a device to all the corresponding devices in other processes.
- Parameters
-
actionName name of the action which just completed
Definition at line 49 of file ifaces/device.cpp.
◆ createDeviceInterface()
|
pure virtual |
Create a specialized interface to interact with the device corresponding to a particular device interface.
- Parameters
-
type the device interface type
- Returns
- a pointer to the device interface if supported by the device, 0 otherwise
◆ description()
|
pure virtual |
Retrieves the description of device.
- Returns
- the description
◆ displayName()
|
virtual |
Retrieves the display name to use for this device.
Same as description when not defined.
- Returns
- the display name
- Since
- 5.71
Definition at line 63 of file ifaces/device.cpp.
◆ emblems()
|
pure virtual |
Retrieves the name of the emblems representing the state of this device.
The naming follows the freedesktop.org specification.
- Returns
- the emblem names
◆ icon()
|
pure virtual |
Retrieves the name of the icon representing this device.
The naming follows the freedesktop.org specification.
- Returns
- the icon name
◆ parentUdi()
|
virtual |
Retrieves the Universal Device Identifier (UDI) of the Device's parent.
- Returns
- the Universal Device Identifier of the parent device
Definition at line 23 of file ifaces/device.cpp.
◆ product()
|
pure virtual |
Retrieves the name of the product corresponding to this device.
- Returns
- the product name
◆ queryDeviceInterface()
|
pure virtual |
Tests if a property exist.
- Parameters
-
type the device interface type
- Returns
- true if the device interface is provided by this device, false otherwise
◆ registerAction()
void Solid::Ifaces::Device::registerAction | ( | const QString & | actionName, |
QObject * | dest, | ||
const char * | requestSlot, | ||
const char * | doneSlot ) const |
Register an action for the given device.
Each time the same device in another process broadcast the begin or the end of such action, the corresponding slots will be called in the current process.
- Parameters
-
actionName name of the action to register dest the object receiving the messages when the action begins and ends requestSlot the slot processing the message when the action begins doneSlot the slot processing the message when the action ends
Definition at line 28 of file ifaces/device.cpp.
◆ udi()
|
pure virtual |
◆ vendor()
|
pure virtual |
Retrieves the name of the device vendor.
- Returns
- the vendor name
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:58:27 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.