Solid::StorageAccess
#include <Solid/StorageAccess>
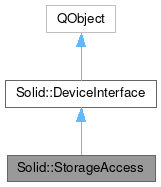
Properties | |
bool | accessible |
bool | encrypted |
QString | filePath |
bool | ignored |
![]() | |
objectName | |
Signals | |
void | accessibilityChanged (bool accessible, const QString &udi) |
void | repairDone (Solid::ErrorType error, QVariant errorData, const QString &udi) |
void | repairRequested (const QString &udi) |
void | setupDone (Solid::ErrorType error, QVariant errorData, const QString &udi) |
void | setupRequested (const QString &udi) |
void | teardownDone (Solid::ErrorType error, QVariant errorData, const QString &udi) |
void | teardownRequested (const QString &udi) |
Public Member Functions | |
~StorageAccess () override | |
bool | canCheck () const |
bool | canRepair () const |
bool | check () |
QString | filePath () const |
bool | isAccessible () const |
bool | isEncrypted () const |
bool | isIgnored () const |
bool | repair () |
bool | setup () |
bool | teardown () |
![]() | |
~DeviceInterface () override | |
bool | isValid () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static Type | deviceInterfaceType () |
![]() | |
static Type | stringToType (const QString &type) |
static QString | typeDescription (Type type) |
static QString | typeToString (Type type) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
SOLID_NO_EXPORT | StorageAccess (StorageAccessPrivate &dd, QObject *backendObject) |
![]() | |
SOLID_NO_EXPORT | DeviceInterface (DeviceInterfacePrivate &dd, QObject *backendObject) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | Type { Unknown = 0 , GenericInterface = 1 , Processor = 2 , Block = 3 , StorageAccess = 4 , StorageDrive = 5 , OpticalDrive = 6 , StorageVolume = 7 , OpticalDisc = 8 , Camera = 9 , PortableMediaPlayer = 10 , Battery = 12 , NetworkShare = 14 , Last = 0xffff } |
![]() | |
typedef | QObjectList |
![]() | |
DeviceInterfacePrivate * | d_ptr |
Detailed Description
This device interface is available on volume devices to access them (i.e.
mount or unmount them).
A volume is anything that can contain data (partition, optical disc, memory card). It's a particular kind of block device.
Property Documentation
◆ accessible
|
read |
Definition at line 33 of file frontend/storageaccess.h.
◆ encrypted
|
read |
Definition at line 36 of file frontend/storageaccess.h.
◆ filePath
|
read |
Definition at line 34 of file frontend/storageaccess.h.
◆ ignored
|
read |
Definition at line 35 of file frontend/storageaccess.h.
Constructor & Destructor Documentation
◆ ~StorageAccess()
|
override |
Destroys a StorageAccess object.
Definition at line 47 of file frontend/storageaccess.cpp.
◆ StorageAccess()
|
protected |
Definition at line 27 of file frontend/storageaccess.cpp.
Member Function Documentation
◆ accessibilityChanged
|
signal |
This signal is emitted when the accessiblity of this device has changed.
- Parameters
-
accessible true if the volume is accessible, false otherwise udi the UDI of the volume
◆ canCheck()
bool Solid::StorageAccess::canCheck | ( | ) | const |
Indicates if this volume can check for filesystem errors.
- Returns
- true if the volume is can be checked
Definition at line 87 of file frontend/storageaccess.cpp.
◆ canRepair()
bool Solid::StorageAccess::canRepair | ( | ) | const |
Indicates if the filesystem of this volume supports repair attempts.
It does not indicate if such an attempt will succeed.
- Returns
- true if the volume is can be repaired
Definition at line 99 of file frontend/storageaccess.cpp.
◆ check()
bool Solid::StorageAccess::check | ( | ) |
Checks the filesystem for consistency avoiding any modifications or repairs.
Mounted or unsupported filesystems will result in an error.
- Returns
- Whether the filesystem is undamaged.
Definition at line 93 of file frontend/storageaccess.cpp.
◆ deviceInterfaceType()
|
inlinestatic |
Get the Solid::DeviceInterface::Type of the StorageAccess device interface.
- Returns
- the StorageVolume device interface type
- See also
- Solid::Ifaces::Enums::DeviceInterface::Type
Definition at line 63 of file frontend/storageaccess.h.
◆ filePath()
QString Solid::StorageAccess::filePath | ( | ) | const |
Retrieves the absolute path of this volume mountpoint.
- Returns
- the absolute path to the mount point if the volume is mounted, QString() otherwise
Definition at line 57 of file frontend/storageaccess.cpp.
◆ isAccessible()
bool Solid::StorageAccess::isAccessible | ( | ) | const |
Indicates if this volume is mounted.
- Returns
- true if the volume is mounted
Definition at line 51 of file frontend/storageaccess.cpp.
◆ isEncrypted()
bool Solid::StorageAccess::isEncrypted | ( | ) | const |
Checks if source of the storage is encrypted.
- Returns
- true if storage is encrypted one
- Since
- 5.80
Definition at line 81 of file frontend/storageaccess.cpp.
◆ isIgnored()
bool Solid::StorageAccess::isIgnored | ( | ) | const |
Indicates if this volume should be ignored by applications.
If it should be ignored, it generally means that it should be invisible to the user. It's useful for firmware partitions or OS reinstall partitions on some systems.
- Returns
- true if the volume should be ignored
Definition at line 75 of file frontend/storageaccess.cpp.
◆ repair()
bool Solid::StorageAccess::repair | ( | ) |
Tries to repair the filesystem.
Mounted or unsupported filesystems will result in an error.
- Returns
- Whether the filesystem could be successfully repaired
Definition at line 105 of file frontend/storageaccess.cpp.
◆ repairDone
|
signal |
This signal is emitted when the attempted repaired of this device is completed.
The signal might be spontaneous i.e. it can be triggered by another process.
- Parameters
-
error type of error that occurred, if any errorData more information about the error, if any udi the UDI of the volume
◆ repairRequested
|
signal |
This signal is emitted when a repair of this device is requested.
The signal might be spontaneous i.e. it can be triggered by another process.
- Parameters
-
udi the UDI of the volume
◆ setup()
bool Solid::StorageAccess::setup | ( | ) |
Mounts the volume.
- Returns
- false if the operation is not supported, true if the operation is attempted
Definition at line 63 of file frontend/storageaccess.cpp.
◆ setupDone
|
signal |
This signal is emitted when the attempted setting up of this device is completed.
The signal might be spontaneous i.e. it can be triggered by another process.
- Parameters
-
error type of error that occurred, if any errorData more information about the error, if any udi the UDI of the volume
◆ setupRequested
|
signal |
This signal is emitted when a setup of this device is requested.
The signal might be spontaneous i.e. it can be triggered by another process.
- Parameters
-
udi the UDI of the volume
◆ teardown()
bool Solid::StorageAccess::teardown | ( | ) |
Unmounts the volume.
- Returns
- false if the operation is not supported, true if the operation is attempted
Definition at line 69 of file frontend/storageaccess.cpp.
◆ teardownDone
|
signal |
This signal is emitted when the attempted tearing down of this device is completed.
The signal might be spontaneous i.e. it can be triggered by another process.
- Parameters
-
error type of error that occurred, if any errorData more information about the error, if any udi the UDI of the volume
◆ teardownRequested
|
signal |
This signal is emitted when a teardown of this device is requested.
The signal might be spontaneous i.e. it can be triggered by another process
- Parameters
-
udi the UDI of the volume
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:57:03 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.