Sonnet::Highlighter
#include <highlighter.h>
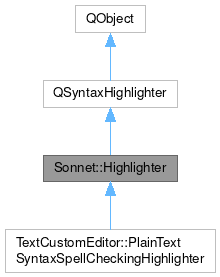
Signals | |
void | activeChanged (const QString &description) |
Public Slots | |
void | setCurrentLanguage (const QString &language) |
void | slotAutoDetection () |
void | slotRehighlight () |
Public Member Functions | |
Highlighter (QPlainTextEdit *textEdit, const QColor &col=QColor()) | |
Highlighter (QTextEdit *textEdit, const QColor &col=QColor()) | |
void | addWordToDictionary (const QString &word) |
bool | autoDetectLanguageDisabled () const |
bool | automatic () const |
bool | checkerEnabledByDefault () const |
QString | currentLanguage () const |
void | ignoreWord (const QString &word) |
bool | isActive () const |
bool | isWordMisspelled (const QString &word) |
void | setActive (bool active) |
void | setAutoDetectLanguageDisabled (bool autoDetectDisabled) |
void | setAutomatic (bool automatic) |
void | setDocument (QTextDocument *document) |
void | setMisspelledColor (const QColor &color) |
bool | spellCheckerFound () const |
QStringList | suggestionsForWord (const QString &word, const QTextCursor &cursor, int max=10) |
QStringList | suggestionsForWord (const QString &word, int max=10) |
![]() | |
QSyntaxHighlighter (QObject *parent) | |
QSyntaxHighlighter (QTextDocument *parent) | |
QTextDocument * | document () const const |
void | rehighlight () |
void | rehighlightBlock (const QTextBlock &block) |
void | setDocument (QTextDocument *doc) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | eventFilter (QObject *o, QEvent *e) override |
void | highlightBlock (const QString &text) override |
bool | intraWordEditing () const |
void | setIntraWordEditing (bool editing) |
virtual void | setMisspelled (int start, int count) |
virtual void | unsetMisspelled (int start, int count) |
![]() | |
QTextBlock | currentBlock () const const |
int | currentBlockState () const const |
QTextBlockUserData * | currentBlockUserData () const const |
QTextCharFormat | format (int position) const const |
int | previousBlockState () const const |
void | setCurrentBlockState (int newState) |
void | setCurrentBlockUserData (QTextBlockUserData *data) |
void | setFormat (int start, int count, const QColor &color) |
void | setFormat (int start, int count, const QFont &font) |
void | setFormat (int start, int count, const QTextCharFormat &format) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The Sonnet Highlighter class, used for drawing pretty red lines in text fields.
Definition at line 25 of file highlighter.h.
Constructor & Destructor Documentation
◆ Highlighter() [1/2]
Definition at line 142 of file highlighter.cpp.
◆ Highlighter() [2/2]
|
explicit |
- Parameters
-
textEdit col define spellchecking color.
- Since
- 5.12
Definition at line 151 of file highlighter.cpp.
◆ ~Highlighter()
|
override |
Definition at line 161 of file highlighter.cpp.
Member Function Documentation
◆ activeChanged
|
signal |
Emitted when as-you-type spell checking is enabled or disabled.
- Parameters
-
description is a i18n description of the new state, with an optional reason
◆ addWordToDictionary()
void Sonnet::Highlighter::addWordToDictionary | ( | const QString & | word | ) |
Adds the given word permanently to the dictionary.
It will never be marked as misspelled again, even after restarting the application.
- Parameters
-
word the word which will be added to the dictionary
- Since
- 4.1
Definition at line 469 of file highlighter.cpp.
◆ autoDetectLanguageDisabled()
bool Sonnet::Highlighter::autoDetectLanguageDisabled | ( | ) | const |
Returns whether the automatic language detection is disabled, overriding the Sonnet settings.
- Returns
- true if the automatic language detection is disabled
- Since
- 5.71
Definition at line 204 of file highlighter.cpp.
◆ automatic()
bool Sonnet::Highlighter::automatic | ( | ) | const |
Returns the state of the automatic disabling of spell checking.
- Returns
- true if spell checking is automatically disabled if there's too many errors
Definition at line 199 of file highlighter.cpp.
◆ checkerEnabledByDefault()
bool Sonnet::Highlighter::checkerEnabledByDefault | ( | ) | const |
Return true if checker is enabled by default.
- Since
- 4.5
Definition at line 514 of file highlighter.cpp.
◆ currentLanguage()
QString Sonnet::Highlighter::currentLanguage | ( | ) | const |
Returns the current language used for spell checking.
- Returns
- the language code for the current language.
Definition at line 375 of file highlighter.cpp.
◆ eventFilter()
Reimplemented from QObject.
Definition at line 411 of file highlighter.cpp.
◆ highlightBlock()
|
overrideprotectedvirtual |
Implements QSyntaxHighlighter.
Reimplemented in TextCustomEditor::PlainTextSyntaxSpellCheckingHighlighter.
Definition at line 315 of file highlighter.cpp.
◆ ignoreWord()
void Sonnet::Highlighter::ignoreWord | ( | const QString & | word | ) |
Ignores the given word.
This word will not be marked misspelled for this session. It will again be marked as misspelled when creating new highlighters.
- Parameters
-
word the word which will be ignored
- Since
- 4.1
Definition at line 474 of file highlighter.cpp.
◆ intraWordEditing()
|
protected |
Definition at line 209 of file highlighter.cpp.
◆ isActive()
bool Sonnet::Highlighter::isActive | ( | ) | const |
Returns the state of spell checking.
- Returns
- true if spell checking is active
- See also
- setActive()
Definition at line 286 of file highlighter.cpp.
◆ isWordMisspelled()
bool Sonnet::Highlighter::isWordMisspelled | ( | const QString & | word | ) |
Checks if a given word is marked as misspelled by the highlighter.
- Parameters
-
word the word to be checked
- Returns
- true if the given word is misspelled.
- Since
- 4.1
Definition at line 504 of file highlighter.cpp.
◆ setActive()
void Sonnet::Highlighter::setActive | ( | bool | active | ) |
Enable/Disable spell checking.
If active
is true then spell checking is enabled; otherwise it is disabled. Note that you have to disable automatic (de)activation with setAutomatic() before you change the state of spell checking if you want to persistently enable/disable spell checking.
- Parameters
-
active if true, then spell checking is enabled
- See also
- isActive(), setAutomatic()
Definition at line 271 of file highlighter.cpp.
◆ setAutoDetectLanguageDisabled()
void Sonnet::Highlighter::setAutoDetectLanguageDisabled | ( | bool | autoDetectDisabled | ) |
Sets whether to disable the automatic language detection.
- Parameters
-
autoDetectDisabled if true, the language will not be detected automatically by the spell checker, even if the option is enabled in the Sonnet settings.
- Since
- 5.71
Definition at line 231 of file highlighter.cpp.
◆ setAutomatic()
void Sonnet::Highlighter::setAutomatic | ( | bool | automatic | ) |
Sets whether to automatically disable spell checking if there's too many errors.
- Parameters
-
automatic if true, spell checking will be disabled if there's a significant amount of errors.
Definition at line 219 of file highlighter.cpp.
◆ setCurrentLanguage
|
slot |
Set language to use for spell checking.
- Parameters
-
language the language code for the new language to use.
Definition at line 380 of file highlighter.cpp.
◆ setDocument()
void Sonnet::Highlighter::setDocument | ( | QTextDocument * | document | ) |
Set a new QTextDocument for this highlighter to operate on.
- Parameters
-
document the new document to operate on.
Definition at line 519 of file highlighter.cpp.
◆ setIntraWordEditing()
|
protected |
Definition at line 214 of file highlighter.cpp.
◆ setMisspelled()
|
protectedvirtual |
Reimplemented in TextCustomEditor::PlainTextSyntaxSpellCheckingHighlighter.
Definition at line 397 of file highlighter.cpp.
◆ setMisspelledColor()
void Sonnet::Highlighter::setMisspelledColor | ( | const QColor & | color | ) |
Sets the color in which the highlighter underlines misspelled words.
- Since
- 4.2
Definition at line 509 of file highlighter.cpp.
◆ slotAutoDetection
|
slot |
Run auto detection, disabling spell checking if too many errors are found.
Definition at line 236 of file highlighter.cpp.
◆ slotRehighlight
|
slot |
Force a new highlighting.
Definition at line 175 of file highlighter.cpp.
◆ spellCheckerFound()
bool Sonnet::Highlighter::spellCheckerFound | ( | ) | const |
Returns whether a spell checking backend with support for the currentLanguage was found.
- Returns
- true if spell checking is supported for the current language.
Definition at line 170 of file highlighter.cpp.
◆ suggestionsForWord() [1/2]
QStringList Sonnet::Highlighter::suggestionsForWord | ( | const QString & | word, |
const QTextCursor & | cursor, | ||
int | max = 10 ) |
Returns a list of suggested replacements for the given misspelled word.
If the word is not misspelled, the list will be empty.
- Parameters
-
word the misspelled word cursor the cursor pointing to the beginning of that word. This is used to determine the language to use, when AutoDetectLanguage is enabled. max at most this many suggestions will be returned. If this is -1, as many suggestions as the spell backend supports will be returned.
- Returns
- a list of suggested replacements for the word
- Since
- 5.42
Definition at line 488 of file highlighter.cpp.
◆ suggestionsForWord() [2/2]
QStringList Sonnet::Highlighter::suggestionsForWord | ( | const QString & | word, |
int | max = 10 ) |
Returns a list of suggested replacements for the given misspelled word.
If the word is not misspelled, the list will be empty.
- Parameters
-
word the misspelled word max at most this many suggestions will be returned. If this is -1, as many suggestions as the spell backend supports will be returned.
- Returns
- a list of suggested replacements for the word
- Since
- 4.1
Definition at line 479 of file highlighter.cpp.
◆ unsetMisspelled()
|
protectedvirtual |
Reimplemented in TextCustomEditor::PlainTextSyntaxSpellCheckingHighlighter.
Definition at line 406 of file highlighter.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:59:24 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.