SpellcheckHighlighter
#include <spellcheckhighlighter.h>
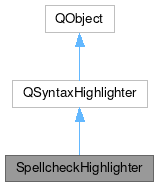
Properties | |
bool | active |
bool | autoDetectLanguageDisabled |
bool | automatic |
QString | currentLanguage |
int | cursorPosition |
QML_ELEMENTQQuickTextDocument * | document |
QColor | misspelledColor |
int | selectionEnd |
int | selectionStart |
bool | spellCheckerFound |
bool | wordIsMisspelled |
QString | wordUnderMouse |
![]() | |
objectName | |
Signals | |
void | activeChanged () |
void | activeChanged (const QString &description) |
void | autoDetectLanguageDisabledChanged () |
void | automaticChanged () |
void | changeCursorPosition (int start, int end) |
void | currentLanguageChanged () |
void | cursorPositionChanged () |
void | documentChanged () |
void | misspelledColorChanged () |
void | selectionEndChanged () |
void | selectionStartChanged () |
void | wordIsMisspelledChanged () |
void | wordUnderMouseChanged () |
Public Slots | |
void | setCurrentLanguage (const QString &language) |
void | slotAutoDetection () |
void | slotRehighlight () |
Public Member Functions | |
SpellcheckHighlighter (QObject *parent=nullptr) | |
bool | active () const |
Q_INVOKABLE void | addWordToDictionary (const QString &word) |
bool | autoDetectLanguageDisabled () const |
bool | automatic () const |
QString | currentLanguage () const |
int | cursorPosition () const |
Q_INVOKABLE void | ignoreWord (const QString &word) |
Q_INVOKABLE bool | isWordMisspelled (const QString &word) |
QColor | misspelledColor () const |
QQuickTextDocument * | quickDocument () const |
QColor | quoteColor () const |
Q_INVOKABLE void | replaceWord (const QString &word, int at=-1) |
int | selectionEnd () const |
int | selectionStart () const |
void | setActive (bool active) |
void | setAutoDetectLanguageDisabled (bool autoDetectDisabled) |
void | setAutomatic (bool automatic) |
void | setCursorPosition (int position) |
void | setDocument (QTextDocument *document) |
void | setMisspelledColor (const QColor &color) |
void | setQuickDocument (QQuickTextDocument *document) |
void | setQuoteColor (const QColor &color) |
void | setSelectionEnd (int position) |
void | setSelectionStart (int position) |
bool | spellCheckerFound () const |
Q_INVOKABLE QStringList | suggestions (int position, int max=5) |
bool | wordIsMisspelled () const |
QString | wordUnderMouse () const |
![]() | |
QSyntaxHighlighter (QObject *parent) | |
QSyntaxHighlighter (QTextDocument *parent) | |
QTextDocument * | document () const const |
void | rehighlight () |
void | rehighlightBlock (const QTextBlock &block) |
void | setDocument (QTextDocument *doc) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | eventFilter (QObject *o, QEvent *e) override |
void | highlightBlock (const QString &text) override |
bool | intraWordEditing () const |
void | setIntraWordEditing (bool editing) |
virtual void | setMisspelled (int start, int count) |
virtual void | setMisspelledSelected (int start, int count) |
virtual void | unsetMisspelled (int start, int count) |
![]() | |
QTextBlock | currentBlock () const const |
int | currentBlockState () const const |
QTextBlockUserData * | currentBlockUserData () const const |
QTextCharFormat | format (int position) const const |
int | previousBlockState () const const |
void | setCurrentBlockState (int newState) |
void | setCurrentBlockUserData (QTextBlockUserData *data) |
void | setFormat (int start, int count, const QColor &color) |
void | setFormat (int start, int count, const QFont &font) |
void | setFormat (int start, int count, const QTextCharFormat &format) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The Sonnet Highlighter class, used for drawing red lines in text fields when detecting spelling mistakes.
SpellcheckHighlighter is adapted for QML applications. In usual Kirigami/QQC2-desktop-style applications, this can be used directly by adding Kirigami.SpellCheck.enabled: true
on a TextArea.
On other QML applications, you can add the SpellcheckHighlighter as a child of a TextArea.
Note: TextField is not supported, as it lacks QTextDocument API that Sonnet relies on.
Additionally SpellcheckHighlighter provides some convenient methods to create a context menu with suggestions.
- See also
- suggestions
- Since
- 5.88
Definition at line 53 of file spellcheckhighlighter.h.
Property Documentation
◆ active
|
readwrite |
This property holds whether spell checking is enabled.
If active
is true then spell checking is enabled; otherwise it is disabled. Note that you have to disable automatic (de)activation with automatic before you change the state of spell checking if you want to persistently enable/disable spell checking.
- See also
- automatic
- Since
- 5.88
Definition at line 104 of file spellcheckhighlighter.h.
◆ autoDetectLanguageDisabled
|
readwrite |
This property holds whether the automatic language detection is disabled overriding the Sonnet global settings.
- Since
- 5.88
Definition at line 114 of file spellcheckhighlighter.h.
◆ automatic
|
readwrite |
This property holds whether spell checking is automatically disabled if there's too many errors.
- Since
- 5.88
Definition at line 109 of file spellcheckhighlighter.h.
◆ currentLanguage
|
read |
This property holds the current language used for spell checking.
- Since
- 5.88
Definition at line 87 of file spellcheckhighlighter.h.
◆ cursorPosition
|
readwrite |
This property holds the current cursor position.
- Since
- 5.88
Definition at line 63 of file spellcheckhighlighter.h.
◆ document
|
readwrite |
This property holds the underneath document from a QML TextEdit.
- Since
- 5.88
Definition at line 59 of file spellcheckhighlighter.h.
◆ misspelledColor
|
readwrite |
This property holds the spell color.
By default, it's red.
- Since
- 5.88
Definition at line 83 of file spellcheckhighlighter.h.
◆ selectionEnd
|
readwrite |
This property holds the end of the selection.
- Since
- 5.88
Definition at line 71 of file spellcheckhighlighter.h.
◆ selectionStart
|
readwrite |
This property holds the start of the selection.
- Since
- 5.88
Definition at line 67 of file spellcheckhighlighter.h.
◆ spellCheckerFound
|
read |
This property holds whether a spell checking backend with support for the currentLanguage was found.
- Since
- 5.88
Definition at line 92 of file spellcheckhighlighter.h.
◆ wordIsMisspelled
|
read |
This property holds whether the current word under the mouse is misspelled.
- Since
- 5.88
Definition at line 75 of file spellcheckhighlighter.h.
◆ wordUnderMouse
|
read |
This property holds the current word under the mouse.
- Since
- 5.88
Definition at line 79 of file spellcheckhighlighter.h.
Constructor & Destructor Documentation
◆ SpellcheckHighlighter()
|
explicit |
Definition at line 158 of file spellcheckhighlighter.cpp.
◆ ~SpellcheckHighlighter()
|
override |
Definition at line 164 of file spellcheckhighlighter.cpp.
Member Function Documentation
◆ active()
bool SpellcheckHighlighter::active | ( | ) | const |
Definition at line 283 of file spellcheckhighlighter.cpp.
◆ activeChanged
|
signal |
Emitted when as-you-type spell checking is enabled or disabled.
- Parameters
-
description is a i18n description of the new state, with an optional reason
- Since
- 5.88
◆ addWordToDictionary()
void SpellcheckHighlighter::addWordToDictionary | ( | const QString & | word | ) |
Adds the given word permanently to the dictionary.
It will never be marked as misspelled again, even after restarting the application.
- Parameters
-
word the word which will be added to the dictionary
- Since
- 5.88
Definition at line 494 of file spellcheckhighlighter.cpp.
◆ autoDetectLanguageDisabled()
bool SpellcheckHighlighter::autoDetectLanguageDisabled | ( | ) | const |
Definition at line 200 of file spellcheckhighlighter.cpp.
◆ automatic()
bool SpellcheckHighlighter::automatic | ( | ) | const |
Definition at line 195 of file spellcheckhighlighter.cpp.
◆ currentLanguage()
QString SpellcheckHighlighter::currentLanguage | ( | ) | const |
Definition at line 457 of file spellcheckhighlighter.cpp.
◆ cursorPosition()
int SpellcheckHighlighter::cursorPosition | ( | ) | const |
Definition at line 560 of file spellcheckhighlighter.cpp.
◆ eventFilter()
Reimplemented from QObject.
Definition at line 661 of file spellcheckhighlighter.cpp.
◆ highlightBlock()
|
overrideprotectedvirtual |
Implements QSyntaxHighlighter.
Definition at line 312 of file spellcheckhighlighter.cpp.
◆ ignoreWord()
void SpellcheckHighlighter::ignoreWord | ( | const QString & | word | ) |
Ignores the given word.
This word will not be marked misspelled for this session. It will again be marked as misspelled when creating new highlighters.
- Parameters
-
word the word which will be ignored
- Since
- 5.88
Definition at line 500 of file spellcheckhighlighter.cpp.
◆ intraWordEditing()
|
protected |
Definition at line 205 of file spellcheckhighlighter.cpp.
◆ isWordMisspelled()
bool SpellcheckHighlighter::isWordMisspelled | ( | const QString & | word | ) |
Checks if a given word is marked as misspelled by the highlighter.
- Parameters
-
word the word to be checked
- Returns
- true if the given word is misspelled.
- Since
- 5.88
Definition at line 656 of file spellcheckhighlighter.cpp.
◆ misspelledColor()
QColor SpellcheckHighlighter::misspelledColor | ( | ) | const |
Definition at line 642 of file spellcheckhighlighter.cpp.
◆ quickDocument()
QQuickTextDocument * SpellcheckHighlighter::quickDocument | ( | ) | const |
Definition at line 533 of file spellcheckhighlighter.cpp.
◆ replaceWord()
void SpellcheckHighlighter::replaceWord | ( | const QString & | word, |
int | at = -1 ) |
Replace word at the current cursor position, or.
- Parameters
-
at if at is not -1.
- Since
- 5.88
Definition at line 506 of file spellcheckhighlighter.cpp.
◆ selectionEnd()
int SpellcheckHighlighter::selectionEnd | ( | ) | const |
Definition at line 591 of file spellcheckhighlighter.cpp.
◆ selectionStart()
int SpellcheckHighlighter::selectionStart | ( | ) | const |
Definition at line 576 of file spellcheckhighlighter.cpp.
◆ setActive()
void SpellcheckHighlighter::setActive | ( | bool | active | ) |
Definition at line 267 of file spellcheckhighlighter.cpp.
◆ setAutoDetectLanguageDisabled()
void SpellcheckHighlighter::setAutoDetectLanguageDisabled | ( | bool | autoDetectDisabled | ) |
Definition at line 227 of file spellcheckhighlighter.cpp.
◆ setAutomatic()
void SpellcheckHighlighter::setAutomatic | ( | bool | automatic | ) |
Definition at line 215 of file spellcheckhighlighter.cpp.
◆ setCurrentLanguage
|
slot |
Set language to use for spell checking.
- Parameters
-
language the language code for the new language to use.
- Since
- 5.88
Definition at line 462 of file spellcheckhighlighter.cpp.
◆ setCursorPosition()
void SpellcheckHighlighter::setCursorPosition | ( | int | position | ) |
Definition at line 565 of file spellcheckhighlighter.cpp.
◆ setDocument()
void SpellcheckHighlighter::setDocument | ( | QTextDocument * | document | ) |
Set a new QTextDocument for this highlighter to operate on.
- Parameters
-
document the new document to operate on.
- Since
- 5.88
Definition at line 554 of file spellcheckhighlighter.cpp.
◆ setIntraWordEditing()
|
protected |
Definition at line 210 of file spellcheckhighlighter.cpp.
◆ setMisspelled()
|
protectedvirtual |
Definition at line 479 of file spellcheckhighlighter.cpp.
◆ setMisspelledColor()
void SpellcheckHighlighter::setMisspelledColor | ( | const QColor & | color | ) |
Definition at line 647 of file spellcheckhighlighter.cpp.
◆ setMisspelledSelected()
|
protectedvirtual |
Definition at line 484 of file spellcheckhighlighter.cpp.
◆ setQuickDocument()
void SpellcheckHighlighter::setQuickDocument | ( | QQuickTextDocument * | document | ) |
Definition at line 538 of file spellcheckhighlighter.cpp.
◆ setSelectionEnd()
void SpellcheckHighlighter::setSelectionEnd | ( | int | position | ) |
Definition at line 596 of file spellcheckhighlighter.cpp.
◆ setSelectionStart()
void SpellcheckHighlighter::setSelectionStart | ( | int | position | ) |
Definition at line 581 of file spellcheckhighlighter.cpp.
◆ slotAutoDetection
|
slot |
Run auto detection, disabling spell checking if too many errors are found.
- Since
- 5.88
Definition at line 232 of file spellcheckhighlighter.cpp.
◆ slotRehighlight
|
slot |
◆ spellCheckerFound()
bool SpellcheckHighlighter::spellCheckerFound | ( | ) | const |
Definition at line 171 of file spellcheckhighlighter.cpp.
◆ suggestions()
QStringList SpellcheckHighlighter::suggestions | ( | int | position, |
int | max = 5 ) |
Returns a list of suggested replacements for the given misspelled word.
If the word is not misspelled, the list will be empty.
- Parameters
-
word the misspelled word max at most this many suggestions will be returned. If this is -1, as many suggestions as the spell backend supports will be returned.
- Returns
- a list of suggested replacements for the word
- Since
- 5.88
Definition at line 390 of file spellcheckhighlighter.cpp.
◆ unsetMisspelled()
|
protectedvirtual |
Definition at line 489 of file spellcheckhighlighter.cpp.
◆ wordIsMisspelled()
bool SpellcheckHighlighter::wordIsMisspelled | ( | ) | const |
Definition at line 632 of file spellcheckhighlighter.cpp.
◆ wordUnderMouse()
QString SpellcheckHighlighter::wordUnderMouse | ( | ) | const |
Definition at line 637 of file spellcheckhighlighter.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:30 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.