KApplicationScope
#include <kapplicationscope.h>
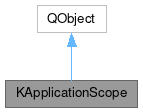
Public Types | |
enum | ErrorCode { NoError , SetFailedError , CacheFillError , StopFailedError } |
Properties | |
QString | cgroup |
OptionalQULongLong | cpuQuota |
OptionalQULongLong | cpuQuotaPeriod |
OptionalQULongLong | cpuWeight |
QString | description |
QString | desktopFilePath |
QString | desktopName |
QString | id |
QString | instance |
OptionalQULongLong | ioWeight |
ErrorCode | lastError |
OptionalQULongLong | memoryHigh |
OptionalQULongLong | memoryLow |
OptionalQULongLong | memoryMax |
OptionalQULongLong | memoryMin |
OptionalQULongLong | memorySwapMax |
QString | path |
![]() | |
objectName | |
Signals | |
void | cgroupChanged (const QString &cgroup) |
void | cpuQuotaChanged (const OptionalQULongLong "a) |
void | cpuQuotaPeriodChanged (const OptionalQULongLong &period) |
void | cpuWeightChanged (const OptionalQULongLong &weight) |
void | descriptionChanged (const QString &description) |
void | desktopFilePathChanged (const QString &desktopFilePath) |
void | desktopNameChanged (const QString &desktopName) |
void | errorOccurred (KApplicationScope::ErrorCode lastError) |
void | idChanged (const QString &id) |
void | instanceChanged (const QString &instance) |
void | ioWeightChanged (const OptionalQULongLong &weight) |
void | memoryHighChanged (const OptionalQULongLong &memoryHigh) |
void | memoryLowChanged (const OptionalQULongLong &memoryLow) |
void | memoryMaxChanged (const OptionalQULongLong &memoryMax) |
void | memoryMinChanged (const OptionalQULongLong &memoryMin) |
void | memorySwapMaxChanged (const OptionalQULongLong &memorySwapMax) |
void | propertyChanged (const QString &propertyName) |
Public Slots | |
void | setCpuQuota (const OptionalQULongLong "a) |
void | setCpuQuotaPeriod (const OptionalQULongLong &period) |
void | setCpuWeight (const OptionalQULongLong &weight) |
void | setIoWeight (const OptionalQULongLong &weight) |
void | setMemoryHigh (const OptionalQULongLong &memoryHigh) |
void | setMemoryLow (const OptionalQULongLong &memoryLow) |
void | setMemoryMax (const OptionalQULongLong &memoryMax) |
void | setMemoryMin (const OptionalQULongLong &memoryMin) |
void | setMemorySwapMax (const OptionalQULongLong &memorySwapMax) |
void | stop () |
Public Member Functions | |
KApplicationScope (const QString &path, const QString &id, QObject *parent=nullptr) | |
KApplicationScope (const QString &path, QObject *parent=nullptr) | |
QString | cgroup () const |
OptionalQULongLong | cpuQuota () const |
OptionalQULongLong | cpuQuotaPeriod () const |
OptionalQULongLong | cpuWeight () const |
QString | description () const |
QString | desktopFilePath () const |
QString | desktopName () const |
QString | id () const |
QString | instance () const |
OptionalQULongLong | ioWeight () const |
ErrorCode | lastError () const |
OptionalQULongLong | memoryHigh () const |
OptionalQULongLong | memoryLow () const |
OptionalQULongLong | memoryMax () const |
OptionalQULongLong | memoryMin () const |
OptionalQULongLong | memorySwapMax () const |
QString | path () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static KApplicationScope * | fromPid (uint pid, QObject *parent=nullptr) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A desktop application in a systemd transient scope.
Definition at line 19 of file kapplicationscope.h.
Member Enumeration Documentation
◆ ErrorCode
The types of errors that can occur.
Enumerator | |
---|---|
NoError | Default value. No error has occurred |
SetFailedError | A property set operation failed. |
CacheFillError | Initial loading of property values failed. |
StopFailedError | Error during stop() operation. |
Definition at line 145 of file kapplicationscope.h.
Property Documentation
◆ cgroup
|
read |
file path of the control group in /sys/fs/cgroup @accessors cgroup() @notifySignal cgroupChanged()
Definition at line 41 of file kapplicationscope.h.
◆ cpuQuota
|
readwrite |
cpu quota for cpu controller, in microseconds per second (1000000 means 100%).
Can be unset @accessors cpuQuota(), setCpuQuota() @notifySignal cpuQuotaChanged()
Definition at line 83 of file kapplicationscope.h.
◆ cpuQuotaPeriod
|
readwrite |
duration in micoseconds over which the CPU time quota is measured.
(default when unset is 100ms) @accessors cpuQuotaPeriod(), setCpuQuotaPeriod() @notifySignal cpuQuotaPeriodChanged()
Definition at line 90 of file kapplicationscope.h.
◆ cpuWeight
|
readwrite |
cpu time weight.
Between 1 and 10000. Defaults to 100. @accessors cpuQuotaPeriod(), setCpuQuotaPeriod() @notifySignal cpuQuotaPeriodChanged()
Definition at line 97 of file kapplicationscope.h.
◆ description
|
read |
the systemd unit description.
Read-only. @accessors description() @notifySignal descriptionChanged()
Definition at line 48 of file kapplicationscope.h.
◆ desktopFilePath
|
read |
the application .desktop file if available.
Read-only. @accessors desktopFilePath() @notifySignal desktopFilePathChanged()
Definition at line 62 of file kapplicationscope.h.
◆ desktopName
|
read |
the .desktop application name.
Read-only. @accessors desktopName() @notifySignal desktopNameChanged()
Definition at line 55 of file kapplicationscope.h.
◆ id
|
read |
the systemd unit id.
Read-only. Will be set asynchronously if not specified in constructor. @accessors id() @notifySignal idChanged()
Definition at line 34 of file kapplicationscope.h.
◆ instance
|
read |
the app instance random identifier.
Can be empty if this is a singleton application. @accessors instance() @notifySignal instanceChanged()
Definition at line 69 of file kapplicationscope.h.
◆ ioWeight
|
readwrite |
Overall block I/O weight.
Between 1 and 10000. Defaults to 100. @accessors ioWeight(), setIoWeight() @notifySignal ioWeightChanged()
Definition at line 104 of file kapplicationscope.h.
◆ lastError
|
read |
code of the last error that occurred (NoError if none) @accessors lastError() @notifySignal errorOccurred()
Definition at line 76 of file kapplicationscope.h.
◆ memoryHigh
|
readwrite |
throttling limit on memory usage (in bytes) of all executed processes within the application.
@accessors memoryHigh(), setMemoryHigh() @notifySignal memoryHighChanged()
Definition at line 118 of file kapplicationscope.h.
◆ memoryLow
|
readwrite |
best-effort memory usage protection (in bytes) of all executed processes within the application.
@accessors memoryLow(), setMemoryLow() @notifySignal memoryLowChanged()
Definition at line 111 of file kapplicationscope.h.
◆ memoryMax
|
readwrite |
absolute limit on memory usage (in bytes) of all executed processes within the application.
@accessors memoryMax(), setMemoryMax() @notifySignal memoryMaxChanged()
Definition at line 132 of file kapplicationscope.h.
◆ memoryMin
|
readwrite |
memory usage protection (in bytes) of all executed processes within the application.
@accessors memoryMin(), setMemoryMin() @notifySignal memoryMinChanged()
Definition at line 125 of file kapplicationscope.h.
◆ memorySwapMax
|
readwrite |
absolute limit on swap usage (in bytes) of all executed processes within the application.
@accessors memorySwapMax(), setMemorySwapMax() @notifySignal memorySwapMaxChanged()
Definition at line 139 of file kapplicationscope.h.
◆ path
|
read |
the dbus path of the application.
Same as constructor parameter. Read-only, constant @accessors path()
Definition at line 27 of file kapplicationscope.h.
Constructor & Destructor Documentation
◆ KApplicationScope() [1/2]
Use when only path is known.
Incurs an extra DBus call to get unit id.
- Parameters
-
path dbus path of the application parent parent QObject
Definition at line 84 of file kapplicationscope.cpp.
◆ KApplicationScope() [2/2]
|
explicit |
Use when unit id is known in advance (such as when using KApplicationScopeLister)
- Parameters
-
path dbus path of the application id systemd unit id parent parent QObject
Definition at line 78 of file kapplicationscope.cpp.
◆ ~KApplicationScope()
|
override |
Definition at line 224 of file kapplicationscope.cpp.
Member Function Documentation
◆ cgroup()
QString KApplicationScope::cgroup | ( | ) | const |
Definition at line 99 of file kapplicationscope.cpp.
◆ cgroupChanged
|
signal |
emitted when cgroup has been loaded asynchronously
- Parameters
-
cgroup the filesystem cgroup path
◆ cpuQuota()
OptionalQULongLong KApplicationScope::cpuQuota | ( | ) | const |
Definition at line 129 of file kapplicationscope.cpp.
◆ cpuQuotaChanged
|
signal |
emitted when the cpu quota has changed
- Parameters
-
quota the new quota value
◆ cpuQuotaPeriod()
OptionalQULongLong KApplicationScope::cpuQuotaPeriod | ( | ) | const |
Definition at line 134 of file kapplicationscope.cpp.
◆ cpuQuotaPeriodChanged
|
signal |
emitted when the cpu quota period has changed
- Parameters
-
period the new period value
◆ cpuWeight()
OptionalQULongLong KApplicationScope::cpuWeight | ( | ) | const |
Definition at line 139 of file kapplicationscope.cpp.
◆ cpuWeightChanged
|
signal |
emitted when the cpu weight has changed
- Parameters
-
weight the new weight value
◆ description()
QString KApplicationScope::description | ( | ) | const |
Definition at line 104 of file kapplicationscope.cpp.
◆ descriptionChanged
|
signal |
emitted when description has been loaded asynchronously
- Parameters
-
description the systemd unit desciption
◆ desktopFilePath()
QString KApplicationScope::desktopFilePath | ( | ) | const |
Definition at line 114 of file kapplicationscope.cpp.
◆ desktopFilePathChanged
|
signal |
emitted when .desktop file path has been loaded asynchronously
- Parameters
-
description the .desktop file path
◆ desktopName()
QString KApplicationScope::desktopName | ( | ) | const |
Definition at line 109 of file kapplicationscope.cpp.
◆ desktopNameChanged
|
signal |
emitted when .desktop name has been loaded asynchronously
- Parameters
-
description the .desktop application name
◆ errorOccurred
|
signal |
emitted when there is an error setting or getting a value
- Parameters
-
lastError the error code
◆ fromPid()
|
static |
Use when only PID is known.
- Parameters
-
pid process ID parent parent QObject
- Returns
- a new KApplicationScope, or null in case of failure (such as if process is not managed by systemd)
Definition at line 236 of file kapplicationscope.cpp.
◆ id()
QString KApplicationScope::id | ( | ) | const |
Definition at line 94 of file kapplicationscope.cpp.
◆ idChanged
|
signal |
emitted after loading when id is not known at constructor time
- Parameters
-
id the systemd unit id
◆ instance()
QString KApplicationScope::instance | ( | ) | const |
Definition at line 119 of file kapplicationscope.cpp.
◆ instanceChanged
|
signal |
emitted when the instance random identifier has been loaded asynchronously
- Parameters
-
description the instance random identifier
◆ ioWeight()
OptionalQULongLong KApplicationScope::ioWeight | ( | ) | const |
Definition at line 144 of file kapplicationscope.cpp.
◆ ioWeightChanged
|
signal |
emitted when the io weight has changed
- Parameters
-
weight the new weight value
◆ lastError()
KApplicationScope::ErrorCode KApplicationScope::lastError | ( | ) | const |
Definition at line 124 of file kapplicationscope.cpp.
◆ memoryHigh()
OptionalQULongLong KApplicationScope::memoryHigh | ( | ) | const |
Definition at line 154 of file kapplicationscope.cpp.
◆ memoryHighChanged
|
signal |
emitted when memoryHigh has changed
- Parameters
-
memoryHigh the new memoryHigh value
◆ memoryLow()
OptionalQULongLong KApplicationScope::memoryLow | ( | ) | const |
Definition at line 149 of file kapplicationscope.cpp.
◆ memoryLowChanged
|
signal |
emitted when memoryLow has changed
- Parameters
-
memoryLow the new memoryLow value
◆ memoryMax()
OptionalQULongLong KApplicationScope::memoryMax | ( | ) | const |
Definition at line 164 of file kapplicationscope.cpp.
◆ memoryMaxChanged
|
signal |
emitted when memoryMax has changed
- Parameters
-
memoryMax the new memoryMax value
◆ memoryMin()
OptionalQULongLong KApplicationScope::memoryMin | ( | ) | const |
Definition at line 159 of file kapplicationscope.cpp.
◆ memoryMinChanged
|
signal |
emitted when memoryMin has changed
- Parameters
-
memoryMin the new memoryMin value
◆ memorySwapMax()
OptionalQULongLong KApplicationScope::memorySwapMax | ( | ) | const |
Definition at line 169 of file kapplicationscope.cpp.
◆ memorySwapMaxChanged
|
signal |
emitted when memorySwapMax has changed
- Parameters
-
memorySwapMax the new memorySwapMax value
◆ path()
QString KApplicationScope::path | ( | ) | const |
Definition at line 89 of file kapplicationscope.cpp.
◆ propertyChanged
|
signal |
emitted when any cgroup resource property has changed
- Parameters
-
propertyName the systemd name of the property
◆ setCpuQuota
|
slot |
◆ setCpuQuotaPeriod
|
slot |
set cpuQuotaPeriod
- Parameters
-
period value to set
Definition at line 179 of file kapplicationscope.cpp.
◆ setCpuWeight
|
slot |
◆ setIoWeight
|
slot |
◆ setMemoryHigh
|
slot |
set memoryHigh
- Parameters
-
memoryHigh value to set
Definition at line 199 of file kapplicationscope.cpp.
◆ setMemoryLow
|
slot |
set memoryLow
- Parameters
-
memoryLow value to set
Definition at line 194 of file kapplicationscope.cpp.
◆ setMemoryMax
|
slot |
set memoryMax
- Parameters
-
memoryMax value to set
Definition at line 209 of file kapplicationscope.cpp.
◆ setMemoryMin
|
slot |
set memoryMin
- Parameters
-
memoryMin value to set
Definition at line 204 of file kapplicationscope.cpp.
◆ setMemorySwapMax
|
slot |
set memorySwapMax
- Parameters
-
memorySwapMax value to set
Definition at line 214 of file kapplicationscope.cpp.
◆ stop
|
slot |
Stops the application.
Definition at line 219 of file kapplicationscope.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:58:47 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.