AgentManager
#include <agentmanager.h>
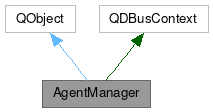
Signals | |
void | agentInstanceAdded (const QString &agentIdentifier) |
void | agentInstanceAdvancedStatusChanged (const QString &agentIdentifier, const QVariantMap &status) |
void | agentInstanceError (const QString &agentIdentifier, const QString &message) |
void | agentInstanceNameChanged (const QString &agentIdentifier, const QString &name) |
void | agentInstanceOnlineChanged (const QString &agentIdentifier, bool state) |
void | agentInstanceProgressChanged (const QString &agentIdentifier, uint progress, const QString &message) |
void | agentInstanceRemoved (const QString &agentIdentifier) |
void | agentInstanceStatusChanged (const QString &agentIdentifier, int status, const QString &message) |
void | agentInstanceWarning (const QString &agentIdentifier, const QString &message) |
void | agentTypeAdded (const QString &agentType) |
void | agentTypeRemoved (const QString &agentType) |
Public Member Functions | |
AgentManager (bool verbose, QObject *parent=nullptr) | |
~AgentManager () override | |
void | addSearch (const QString &query, const QString &queryLanguage, qint64 resultCollectionId) |
QStringList | agentCapabilities (const QString &identifier) const |
QString | agentComment (const QString &identifier) const |
QVariantMap | agentCustomProperties (const QString &identifier) const |
QString | agentIcon (const QString &identifier) const |
QStringList | agentInstanceActivities (const QString &identifier) |
bool | agentInstanceActivitiesEnabled (const QString &identifier) |
void | agentInstanceConfigure (const QString &identifier, qlonglong windowId) |
QString | agentInstanceName (const QString &identifier) const |
bool | agentInstanceOnline (const QString &identifier) |
uint | agentInstanceProgress (const QString &identifier) const |
QString | agentInstanceProgressMessage (const QString &identifier) const |
QStringList | agentInstances () const |
int | agentInstanceStatus (const QString &identifier) const |
QString | agentInstanceStatusMessage (const QString &identifier) const |
void | agentInstanceSynchronize (const QString &identifier) |
void | agentInstanceSynchronizeCollection (const QString &identifier, qint64 collection) |
void | agentInstanceSynchronizeCollection (const QString &identifier, qint64 collection, bool recursive) |
void | agentInstanceSynchronizeCollectionTree (const QString &identifier) |
void | agentInstanceSynchronizeTags (const QString &identifier) |
QString | agentInstanceType (const QString &identifier) |
QStringList | agentMimeTypes (const QString &identifier) const |
QString | agentName (const QString &identifier) const |
QStringList | agentTypes () const |
void | cleanup () |
QString | createAgentInstance (const QString &identifier) |
void | removeAgentInstance (const QString &identifier) |
void | removeSearch (quint64 resultCollectionId) |
void | restartAgentInstance (const QString &identifier) |
void | setAgentInstanceActivities (const QString &identifier, const QStringList &activities) |
void | setAgentInstanceActivitiesEnabled (const QString &identifier, bool enabled) |
void | setAgentInstanceName (const QString &identifier, const QString &name) |
void | setAgentInstanceOnline (const QString &identifier, bool state) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
bool | calledFromDBus () const const |
QDBusConnection | connection () const const |
bool | isDelayedReply () const const |
void | sendErrorReply (const QString &name, const QString &msg) const const |
void | sendErrorReply (QDBusError::ErrorType type, const QString &msg) const const |
void | setDelayedReply (bool enable) const const |
Detailed Description
The agent manager has knowledge about all available agents (it scans for .desktop files in the agent directory) and the available configured instances.
Definition at line 28 of file akonadicontrol/agentmanager.h.
Constructor & Destructor Documentation
◆ AgentManager()
|
explicit |
Creates a new agent manager.
- Parameters
-
parent The parent object.
Definition at line 100 of file akonadicontrol/agentmanager.cpp.
◆ ~AgentManager()
|
overridedefault |
Destroys the agent manager.
Definition at line 163 of file akonadicontrol/agentmanager.cpp.
Member Function Documentation
◆ addSearch()
void AgentManager::addSearch | ( | const QString & | query, |
const QString & | queryLanguage, | ||
qint64 | resultCollectionId ) |
Add a persistent search to remote search agents.
Definition at line 943 of file akonadicontrol/agentmanager.cpp.
◆ agentCapabilities()
|
nodiscard |
Returns a list of supported capabilities of the agent type for the given identifier
.
Definition at line 225 of file akonadicontrol/agentmanager.cpp.
◆ agentComment()
Returns the i18n'ed comment of the agent type for the given identifier
.
Definition at line 193 of file akonadicontrol/agentmanager.cpp.
◆ agentCustomProperties()
|
nodiscard |
Returns a list of Custom added properties of the agent type for the given identifier
.
- Since
- 1.11
Definition at line 233 of file akonadicontrol/agentmanager.cpp.
◆ agentIcon()
Returns the icon name of the agent type for the given identifier
.
Definition at line 202 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceActivities()
|
nodiscard |
Definition at line 424 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceActivitiesEnabled()
|
nodiscard |
Definition at line 445 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceAdded
|
signal |
This signal is emitted whenever a new agent instance was created.
- Parameters
-
agentIdentifier The identifier of the new agent instance.
◆ agentInstanceAdvancedStatusChanged
|
signal |
This signal is emitted whenever the status of an agent instance has changed.
- Parameters
-
agentIdentifier The identifier of the agent that has changed. status The object that describes the status change.
◆ agentInstanceConfigure()
void AgentManager::agentInstanceConfigure | ( | const QString & | identifier, |
qlonglong | windowId ) |
Triggers the agent instance with the given identifier
to show its configuration dialog.
- Parameters
-
windowId Parent window id for the configuration dialog.
Definition at line 388 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceError
|
signal |
This signal is emitted whenever an agent instance raised an error.
- Parameters
-
agentIdentifier The identifier of the agent instance. message The i18n'ed error message.
◆ agentInstanceName()
Returns the name of the agent instance with the given identifier
.
Definition at line 467 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceNameChanged
|
signal |
This signal is emitted whenever the name of the agent instance has changed.
- Parameters
-
agentIdentifier The identifier of the agent that has changed. name The new name of the agent instance.
◆ agentInstanceOnline()
bool AgentManager::agentInstanceOnline | ( | const QString & | identifier | ) |
Returns if the agent instance identifier
is in online mode.
Definition at line 397 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceOnlineChanged
|
signal |
Emitted when the online state of an agent changed.
◆ agentInstanceProgress()
|
nodiscard |
Returns the current progress of the agent with the given identifier
in percentage.
Definition at line 372 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceProgressChanged
|
signal |
This signal is emitted whenever the progress of an agent instance has changed.
- Parameters
-
agentIdentifier The identifier of the agent that has changed. progress The new progress in percentage. message The i18n'ed description of the new progress.
◆ agentInstanceProgressMessage()
Returns the i18n'ed description of the current progress of the agent with the given identifier
.
Definition at line 381 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceRemoved
|
signal |
This signal is emitted whenever an agent instance was removed.
- Parameters
-
agentIdentifier The identifier of the removed agent instance.
◆ agentInstances()
|
nodiscard |
Returns the list of identifiers of configured instances.
Definition at line 349 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceStatus()
int AgentManager::agentInstanceStatus | ( | const QString & | identifier | ) | const |
Returns the current status code of the agent with the given identifier
.
Definition at line 354 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceStatusChanged
|
signal |
This signal is emitted whenever the status of an agent instance has changed.
- Parameters
-
agentIdentifier The identifier of the agent that has changed. status The new status code. message The i18n'ed description of the new status.
◆ agentInstanceStatusMessage()
Returns the i18n'ed description of the current status of the agent with the given identifier
.
Definition at line 363 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceSynchronize()
void AgentManager::agentInstanceSynchronize | ( | const QString & | identifier | ) |
Triggers the agent instance with the given identifier
to start synchronization.
Definition at line 485 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceSynchronizeCollection() [1/2]
void AgentManager::agentInstanceSynchronizeCollection | ( | const QString & | identifier, |
qint64 | collection ) |
Trigger a synchronization of the given collection by its owning resource agent.
Definition at line 503 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceSynchronizeCollection() [2/2]
void AgentManager::agentInstanceSynchronizeCollection | ( | const QString & | identifier, |
qint64 | collection, | ||
bool | recursive ) |
Trigger a synchronization of the given collection by its owning resource agent.
- Parameters
-
recursive set it true to have sub-collection synchronized as well
Definition at line 508 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceSynchronizeCollectionTree()
void AgentManager::agentInstanceSynchronizeCollectionTree | ( | const QString & | identifier | ) |
Trigger a synchronization of the collection tree by the given resource agent.
- Parameters
-
identifier The resource agent identifier.
Definition at line 494 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceSynchronizeTags()
void AgentManager::agentInstanceSynchronizeTags | ( | const QString & | identifier | ) |
Trigger a synchronization of tags by the given resource agent.
- Parameters
-
identifier The resource agent identifier.
Definition at line 517 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceType()
Returns the type of the agent instance with the given identifier
.
Definition at line 338 of file akonadicontrol/agentmanager.cpp.
◆ agentInstanceWarning
|
signal |
This signal is emitted whenever an agent instance raised a warning.
- Parameters
-
agentIdentifier The identifier of the agent instance. message The i18n'ed warning message.
◆ agentMimeTypes()
|
nodiscard |
Returns a list of supported mimetypes of the agent type for the given identifier
.
Definition at line 216 of file akonadicontrol/agentmanager.cpp.
◆ agentName()
Returns the i18n'ed name of the agent type for the given identifier
.
Definition at line 184 of file akonadicontrol/agentmanager.cpp.
◆ agentTypeAdded
|
signal |
This signal is emitted whenever a new agent type was installed on the system.
- Parameters
-
agentType The identifier of the new agent type.
◆ agentTypeRemoved
|
signal |
This signal is emitted whenever an agent type was removed from the system.
- Parameters
-
agentType The identifier of the removed agent type.
◆ agentTypes()
|
nodiscard |
Returns the list of identifiers of all available agent types.
Definition at line 179 of file akonadicontrol/agentmanager.cpp.
◆ cleanup()
void AgentManager::cleanup | ( | ) |
Called by the crash handler and dtor to terminate the child processes.
Definition at line 168 of file akonadicontrol/agentmanager.cpp.
◆ createAgentInstance()
Creates a new agent of the given agent type identifier
.
- Returns
- The identifier of the new agent if created successfully, an empty string otherwise. The identifier consists of two parts, the type of the agent and an unique instance number, and looks like the following: 'file_1' or 'imap_267'.
Definition at line 257 of file akonadicontrol/agentmanager.cpp.
◆ removeAgentInstance()
void AgentManager::removeAgentInstance | ( | const QString & | identifier | ) |
Removes the agent with the given identifier
.
Definition at line 296 of file akonadicontrol/agentmanager.cpp.
◆ removeSearch()
void AgentManager::removeSearch | ( | quint64 | resultCollectionId | ) |
Removes a persistent search for the given result collection.
Definition at line 954 of file akonadicontrol/agentmanager.cpp.
◆ restartAgentInstance()
void AgentManager::restartAgentInstance | ( | const QString & | identifier | ) |
Restarts the agent instance identifier
.
This is supposed to be used as a development aid and not something to use during normal operations.
Definition at line 526 of file akonadicontrol/agentmanager.cpp.
◆ setAgentInstanceActivities()
void AgentManager::setAgentInstanceActivities | ( | const QString & | identifier, |
const QStringList & | activities ) |
Definition at line 415 of file akonadicontrol/agentmanager.cpp.
◆ setAgentInstanceActivitiesEnabled()
void AgentManager::setAgentInstanceActivitiesEnabled | ( | const QString & | identifier, |
bool | enabled ) |
Definition at line 436 of file akonadicontrol/agentmanager.cpp.
◆ setAgentInstanceName()
Sets the name
of the agent instance with the given identifier
.
Definition at line 458 of file akonadicontrol/agentmanager.cpp.
◆ setAgentInstanceOnline()
void AgentManager::setAgentInstanceOnline | ( | const QString & | identifier, |
bool | state ) |
Sets agent instance identifier
to online or offline mode.
Definition at line 406 of file akonadicontrol/agentmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:10 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.