KLDAPCore::LdapUrl
#include <ldapurl.h>
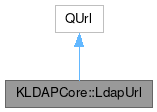
Public Types | |
using | Extension |
using | Scope |
![]() | |
enum | AceProcessingOption |
enum | ComponentFormattingOption |
enum | ParsingMode |
enum | UrlFormattingOption |
enum | UserInputResolutionOption |
Public Member Functions | |
LdapUrl () | |
LdapUrl (const LdapUrl &other) | |
LdapUrl (const QUrl &url) | |
~LdapUrl () | |
QStringList | attributes () const |
LdapDN | dn () const |
Extension | extension (const QString &extension) const |
QString | extension (const QString &extension, bool &critical) const |
QString | filter () const |
bool | hasExtension (const QString &extension) const |
LdapUrl & | operator= (const LdapUrl &other) |
void | parseQuery () |
void | removeExtension (const QString &extension) |
Scope | scope () const |
void | setAttributes (const QStringList &attributes) |
void | setDn (const LdapDN &dn) |
void | setExtension (const QString &key, const Extension &extension) |
void | setExtension (const QString &key, const QString &value, bool critical=false) |
void | setExtension (const QString &key, int value, bool critical=false) |
void | setFilter (const QString &filter) |
void | setScope (Scope scope) |
void | updateQuery () |
![]() | |
QUrl (const QString &url, ParsingMode parsingMode) | |
QUrl (const QUrl &other) | |
QUrl (QUrl &&other) | |
QUrl | adjusted (FormattingOptions options) const const |
QString | authority (ComponentFormattingOptions options) const const |
void | clear () |
QString | errorString () const const |
QString | fileName (ComponentFormattingOptions options) const const |
QString | fragment (ComponentFormattingOptions options) const const |
bool | hasFragment () const const |
bool | hasQuery () const const |
QString | host (ComponentFormattingOptions options) const const |
bool | isEmpty () const const |
bool | isLocalFile () const const |
bool | isParentOf (const QUrl &childUrl) const const |
bool | isRelative () const const |
bool | isValid () const const |
bool | matches (const QUrl &url, FormattingOptions options) const const |
bool | operator!= (const QUrl &url) const const |
QDataStream & | operator<< (QDataStream &out, const QUrl &url) |
QUrl & | operator= (const QString &url) |
QUrl & | operator= (const QUrl &url) |
QUrl & | operator= (QUrl &&other) |
bool | operator== (const QUrl &url) const const |
QDataStream & | operator>> (QDataStream &in, QUrl &url) |
QString | password (ComponentFormattingOptions options) const const |
QString | path (ComponentFormattingOptions options) const const |
int | port (int defaultPort) const const |
QT_NO_URL_CAST_FROM_STRING QT_NO_URL_CAST_FROM_STRING | |
QString | query (ComponentFormattingOptions options) const const |
QUrl | resolved (const QUrl &relative) const const |
QString | scheme () const const |
void | setAuthority (const QString &authority, ParsingMode mode) |
void | setFragment (const QString &fragment, ParsingMode mode) |
void | setHost (const QString &host, ParsingMode mode) |
void | setPassword (const QString &password, ParsingMode mode) |
void | setPath (const QString &path, ParsingMode mode) |
void | setPort (int port) |
void | setQuery (const QString &query, ParsingMode mode) |
void | setQuery (const QUrlQuery &query) |
void | setScheme (const QString &scheme) |
void | setUrl (const QString &url, ParsingMode parsingMode) |
void | setUserInfo (const QString &userInfo, ParsingMode mode) |
void | setUserName (const QString &userName, ParsingMode mode) |
void | swap (QUrl &other) |
CFURLRef | toCFURL () const const |
QString | toDisplayString (FormattingOptions options) const const |
QByteArray | toEncoded (FormattingOptions options) const const |
QString | toLocalFile () const const |
NSURL * | toNSURL () const const |
QString | toString (FormattingOptions options) const const |
QString | url (FormattingOptions options) const const |
QString | userInfo (ComponentFormattingOptions options) const const |
QString | userName (ComponentFormattingOptions options) const const |
Detailed Description
A special url class for LDAP.
LdapUrl implements an RFC 2255 compliant LDAP Url parser, with minimal differences. LDAP Urls implemented by this class has the following format: ldap[s]://[user[:password]@]hostname[:port]["/" [dn ["?" [attributes] ["?" [scope] ["?" [filter] ["?" extensions]]]]]]
Member Typedef Documentation
◆ Extension
◆ Scope
Constructor & Destructor Documentation
◆ LdapUrl() [1/3]
LdapUrl::LdapUrl | ( | ) |
Constructs an empty LDAP url.
Definition at line 29 of file ldapurl.cpp.
◆ LdapUrl() [2/3]
|
explicit |
Constructs a LDAP url from a KUrl url
.
Definition at line 34 of file ldapurl.cpp.
◆ LdapUrl() [3/3]
LdapUrl::LdapUrl | ( | const LdapUrl & | other | ) |
Constructs a LDAP url from an other url.
Definition at line 41 of file ldapurl.cpp.
◆ ~LdapUrl()
|
default |
Destroys the LDAP url.
Member Function Documentation
◆ attributes()
QStringList LdapUrl::attributes | ( | ) | const |
Returns the attributes part of the LDAP url.
Definition at line 82 of file ldapurl.cpp.
◆ dn()
LdapDN LdapUrl::dn | ( | ) | const |
Returns the dn part of the LDAP url.
This is equal to path() with the slash removed from the beginning.
Definition at line 72 of file ldapurl.cpp.
◆ extension() [1/2]
LdapUrl::Extension LdapUrl::extension | ( | const QString & | extension | ) | const |
Returns the specified extension
.
Definition at line 120 of file ldapurl.cpp.
◆ extension() [2/2]
Returns the specified extension
.
Definition at line 135 of file ldapurl.cpp.
◆ filter()
QString LdapUrl::filter | ( | ) | const |
Returns the filter part of the LDAP url.
Definition at line 104 of file ldapurl.cpp.
◆ hasExtension()
bool LdapUrl::hasExtension | ( | const QString & | extension | ) | const |
Returns whether the specified extension
exists in the LDAP url.
Definition at line 115 of file ldapurl.cpp.
◆ operator=()
Overwrites the values of the LDAP url with values from an other
url.
Definition at line 48 of file ldapurl.cpp.
◆ parseQuery()
void LdapUrl::parseQuery | ( | ) |
Parses the query argument of the URL and makes it available via the attributes(), extension(), filter() and scope() methods.
Definition at line 220 of file ldapurl.cpp.
◆ removeExtension()
void LdapUrl::removeExtension | ( | const QString & | extension | ) |
Removes the specified extension
.
Definition at line 164 of file ldapurl.cpp.
◆ scope()
LdapUrl::Scope LdapUrl::scope | ( | ) | const |
Returns the scope part of the LDAP url.
Definition at line 93 of file ldapurl.cpp.
◆ setAttributes()
void LdapUrl::setAttributes | ( | const QStringList & | attributes | ) |
Sets the attributes
part of the LDAP url.
Definition at line 87 of file ldapurl.cpp.
◆ setDn()
void LdapUrl::setDn | ( | const LdapDN & | dn | ) |
Sets the dn
part of the LDAP url.
Definition at line 62 of file ldapurl.cpp.
◆ setExtension() [1/3]
Sets the specified extension key
with the value and criticality in extension
.
◆ setExtension() [2/3]
Sets the specified extension key
with the value
and criticality specified.
Definition at line 148 of file ldapurl.cpp.
◆ setExtension() [3/3]
void LdapUrl::setExtension | ( | const QString & | key, |
int | value, | ||
bool | critical = false ) |
Sets the specified extension key
with the value
and criticality specified.
Definition at line 156 of file ldapurl.cpp.
◆ setFilter()
void LdapUrl::setFilter | ( | const QString & | filter | ) |
Sets the filter part of the LDAP url.
Definition at line 109 of file ldapurl.cpp.
◆ setScope()
void LdapUrl::setScope | ( | Scope | scope | ) |
Sets the scope part of the LDAP url.
Definition at line 98 of file ldapurl.cpp.
◆ updateQuery()
void LdapUrl::updateQuery | ( | ) |
Updates the query component from the attributes, scope, filter and extensions.
Definition at line 170 of file ldapurl.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:12:58 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.