KPIMTextEdit::AbstractMarkupBuilder
#include <grantlee/abstractmarkupbuilder.h>
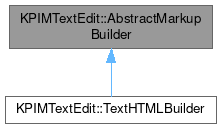
Public Member Functions | |
virtual | ~AbstractMarkupBuilder ()=default |
virtual void | addNewline ()=0 |
virtual void | addSingleBreakLine ()=0 |
virtual void | appendLiteralText (const QString &text)=0 |
virtual void | appendRawText (const QString &text)=0 |
virtual void | beginAnchor (const QString &href={}, const QString &name={})=0 |
virtual void | beginBackground (const QBrush &brush)=0 |
virtual void | beginEmph ()=0 |
virtual void | beginFontFamily (const QString &family)=0 |
virtual void | beginFontPointSize (int size)=0 |
virtual void | beginForeground (const QBrush &brush)=0 |
virtual void | beginHeader (int level)=0 |
virtual void | beginList (QTextListFormat::Style style)=0 |
virtual void | beginListItem ()=0 |
virtual void | beginParagraph (Qt::Alignment a=Qt::AlignLeft, qreal top=0.0, qreal bottom=0.0, qreal left=0.0, qreal right=0.0, bool leftToRightText=false)=0 |
virtual void | beginStrikeout ()=0 |
virtual void | beginStrong ()=0 |
virtual void | beginSubscript ()=0 |
virtual void | beginSuperscript ()=0 |
virtual void | beginTable (qreal cellpadding, qreal cellspacing, const QString &width)=0 |
virtual void | beginTableCell (const QString &width, int colSpan, int rowSpan)=0 |
virtual void | beginTableHeaderCell (const QString &width, int colSpan, int rowSpan)=0 |
virtual void | beginTableRow ()=0 |
virtual void | beginUnderline ()=0 |
virtual void | endAnchor ()=0 |
virtual void | endBackground ()=0 |
virtual void | endEmph ()=0 |
virtual void | endFontFamily ()=0 |
virtual void | endFontPointSize ()=0 |
virtual void | endForeground ()=0 |
virtual void | endHeader (int level)=0 |
virtual void | endList ()=0 |
virtual void | endListItem ()=0 |
virtual void | endParagraph ()=0 |
virtual void | endStrikeout ()=0 |
virtual void | endStrong ()=0 |
virtual void | endSubscript ()=0 |
virtual void | endSuperscript ()=0 |
virtual void | endTable ()=0 |
virtual void | endTableCell ()=0 |
virtual void | endTableHeaderCell ()=0 |
virtual void | endTableRow ()=0 |
virtual void | endUnderline ()=0 |
virtual QString | getResult ()=0 |
virtual void | insertHorizontalRule (int width=-1)=0 |
virtual void | insertImage (const QString &url, qreal width, qreal height)=0 |
Detailed Description
Interface for creating marked-up text output.
The AbstractMarkupBuilder is used by a MarkupDirector to create marked-up output such as html or markdown.
See PlainTextMarkupBuilder and TextHTMLBuilder for example implementations.
This interface can be extended to handle custom format types in a QTextDocument.
- See also
- custom_qtextobject
Definition at line 36 of file abstractmarkupbuilder.h.
Constructor & Destructor Documentation
◆ ~AbstractMarkupBuilder()
|
virtualdefault |
Destructor.
Member Function Documentation
◆ addNewline()
|
pure virtual |
Add a newline to the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ appendLiteralText()
|
pure virtual |
Append the plain text text
to the markup.
- Parameters
-
text The text to append.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ appendRawText()
|
pure virtual |
Append the raw text text
to the markup.
text
is added unescaped
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginAnchor()
|
pure virtual |
Begin a url anchor element in the markup.
- Parameters
-
href The href of the anchor. name The name of the anchor.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginBackground()
|
pure virtual |
Begin a decorarated background element in the markup (A text background color) using brush
.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginEmph()
|
pure virtual |
Begin an emphasised element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginFontFamily()
|
pure virtual |
Begin a new font family element in the markup.
- Parameters
-
family The name of the font family to begin.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginFontPointSize()
|
pure virtual |
Begin a new font point size element in the markup.
- Parameters
-
size The point size to begin.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginForeground()
|
pure virtual |
Begin a decorarated foreground element in the markup (A text color) using brush
.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginHeader()
|
pure virtual |
Begin a level level
header.
- Parameters
-
level An integer between 1 and 6
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginList()
|
pure virtual |
Begin a new list element in the markup.
A list element contains list items, and may contain other lists.
- Parameters
-
style The style of list to create.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginListItem()
|
pure virtual |
Begin a new list item in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginParagraph()
|
pure virtual |
Begin a new paragraph in the markup.
- Parameters
-
a The alignment of the new paragraph. top The top margin of the new paragraph. bottom The bottom margin of the new paragraph. left The left margin of the new paragraph. right The right margin of the new paragraph.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginStrikeout()
|
pure virtual |
Begin a struck out element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginStrong()
|
pure virtual |
Begin a bold element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginSubscript()
|
pure virtual |
Begin a subscript element.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginSuperscript()
|
pure virtual |
Begin a superscript element.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginTable()
|
pure virtual |
Begin a table element.
- Parameters
-
cellpadding The padding attribute for the table. cellspacing The spacing attribute for the table. width The width of the table. May be either an integer, or a percentage value.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginTableCell()
|
pure virtual |
Begin a new table cell.
- Parameters
-
width The width of the cell. colSpan The column span of the cell. rowSpan The row span of the cell.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginTableHeaderCell()
|
pure virtual |
Begin a new table header cell.
- Parameters
-
width The width of the cell. colSpan The column span of the cell. rowSpan The row span of the cell.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginTableRow()
|
pure virtual |
Begin a new table row.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ beginUnderline()
|
pure virtual |
Begin an underlined element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endAnchor()
|
pure virtual |
Close the anchor element.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endBackground()
|
pure virtual |
Close the decorarated background element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endEmph()
|
pure virtual |
Close the emphasised element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endFontFamily()
|
pure virtual |
End font family element.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endFontPointSize()
|
pure virtual |
End font point size element.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endForeground()
|
pure virtual |
Close the decorarated foreground element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endHeader()
|
pure virtual |
End a level level
header.
- Parameters
-
level An integer between 1 and 6
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endList()
|
pure virtual |
Close the list.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endListItem()
|
pure virtual |
End the list item.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endParagraph()
|
pure virtual |
Close the paragraph in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endStrikeout()
|
pure virtual |
Close the struck out element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endStrong()
|
pure virtual |
Close the bold element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endSubscript()
|
pure virtual |
End subscript element.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endSuperscript()
|
pure virtual |
End superscript element.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endTable()
|
pure virtual |
End a table element.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endTableCell()
|
pure virtual |
End a table cell.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endTableHeaderCell()
|
pure virtual |
End a table header cell.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endTableRow()
|
pure virtual |
End a table row.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ endUnderline()
|
pure virtual |
Close the underlined element in the markup.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ getResult()
|
nodiscardpure virtual |
Return the fully marked up result of the building process.
This may contain metadata etc, such as a head element in html.
- Returns
- The fully marked up text.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ insertHorizontalRule()
|
pure virtual |
Insert a horizontal rule into the markup.
- Parameters
-
width The width of the rule. Default is full width.
Implemented in KPIMTextEdit::TextHTMLBuilder.
◆ insertImage()
|
pure virtual |
Insert a new image element into the markup.
- Parameters
-
url The url of the image width The width of the image height The height of the image.
Implemented in KPIMTextEdit::TextHTMLBuilder.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 12:00:02 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.